Exploring the Linear Search Algorithm
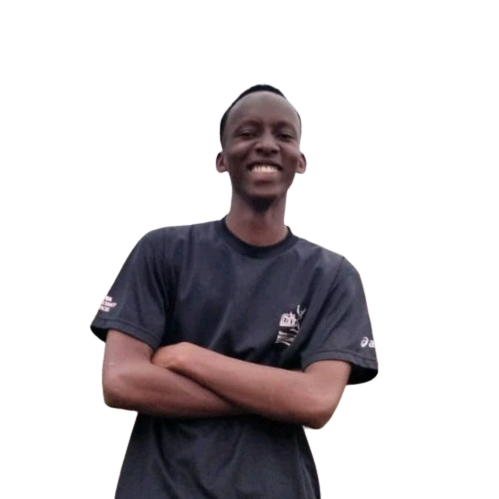
Introduction
In the realm of algorithms, the linear search algorithm holds a significant place as one of the simplest yet most powerful techniques for finding an element in a collection. In this blog post, we'll dive into the world of linear search, exploring its concepts, implementation, and time complexity analysis. Let's embark on this journey to understand the inner workings of the linear search algorithm.
Overview of Linear Search
The linear search algorithm sequentially checks each element in a collection until the desired element is found or the end of the collection is reached. It is a straightforward approach applicable to both sorted and unsorted arrays. Let's dissect the code snippet and understand each part in detail.
Code Breakdown
cppCopy code#include <iostream>
using namespace std;
int main()
{
int i, data;
bool isFound = false;
// declare array of elements
int arr[5] = {12, 22, 24, 44, 12};
int n = sizeof(arr) / sizeof(int);
cout << "Enter Element to check on the list: " << endl;
cin >> data;
// loop through elements in the array
for (i = 0; i < n; i++)
{
// check if the element is in the list
if (arr[i] == data)
{
cout << "Element found at position " << i + 1 << endl;
isFound = true; // confirm that the element is found
break;
}
cout << "Element not at position " << (i + 1) << endl;
cout << "Still more " << (n) - (i + 1) << " position(s) to go" << endl;
}
// Check if the element is not found
if (isFound == false || i == n)
{
cout << "______SORRY ELEMENT NOT FOUND!!_____________" << endl;
}
return 0;
}
Explanation of Code:
Variable Initialization:
i
: Loop counter variable.data
: Element to be searched in the array.isFound
: Flag variable to track if the element is found.
Array Declaration:
arr
: Array of elements to be searched.n
: Number of elements in the array.
User Input:
- Prompt the user to enter the element they want to search.
Linear Search Loop:
Iterate through each element in the array using a for loop.
Compare each element with the desired element (
data
).If a match is found, display the position and set
isFound
to true.If no match is found, display the current position and the remaining positions to search.
Element Not Found:
Check if the element is not found based on the flag
isFound
and loop termination condition.Display a message indicating that the element was not found.
Time Complexity Analysis
The time complexity of the linear search algorithm is O(n), where n represents the number of elements in the array. In the worst-case scenario, the algorithm may need to iterate through all the elements to find the desired element.
The space complexity is O(1).
Application
Here are a few examples of where linear search can be applicable:
Small Lists: Linear search is efficient for small lists or arrays where the number of elements is relatively small. Since it iterates through each element sequentially, the overhead of implementing complex search algorithms may not be justified.
Unsorted Lists: Linear search can be used to find an element in an unsorted list. Unlike binary search, which requires a sorted list, linear search does not rely on any particular order, making it suitable for unsorted data.
Partial Matches: In certain cases, you might need to find elements that partially match specific criteria. Linear search allows you to iterate through the entire collection, enabling you to compare elements based on partial matches or specific conditions.
Early Terminations: Linear search can be useful when you want to terminate the search as soon as you find the first occurrence of the desired element. This can be helpful in scenarios where you are looking for duplicates or specific instances of an element within a collection.
Sequential Data: Linear search is ideal for searching through sequential data structures, such as linked lists, where direct access to elements is not available. It allows you to traverse the data structure and find the desired element by comparing each node's value.
Conclusion
The linear search algorithm provides a simple yet effective solution for finding elements in a collection. By sequentially scanning each element, it enables us to locate the desired element efficiently. Understanding this algorithm and its time complexity empowers us to make informed decisions when choosing the appropriate search technique for different scenarios.
To explore more about the linear search algorithm and its variations, stay tuned for further articles on my Hashnode blog.
Happy coding and searching efficiently! 🚀💡
Subscribe to my newsletter
Read articles from Titus Kiplagat directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
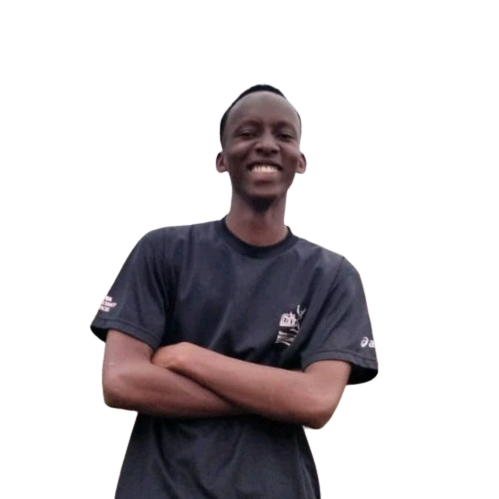
Titus Kiplagat
Titus Kiplagat
I happily spend late nights trying to build anything I think of, training AI models, or building SaaS tools that actually make people’s lives easier. With 5+ years in the game, I move across Python, JavaScript, LLMs, and backend systems like second nature — and I love blending deep tech with real-world impact. Always curious, always hands-on, always curious for the next breakthrough.