Two Sum - LeetCode's Solution in C#
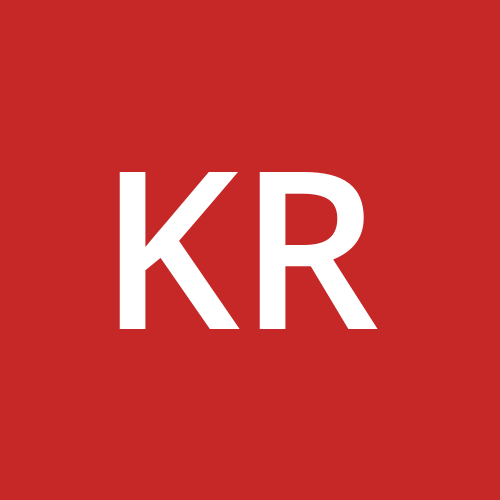
Problem: You have a function that takes the arguments of an array and a target. You have to return two values from the array that sum up to the target.
There would be exactly one solution, and using the same element twice is prohibited.
An example would be:
Input: nums = [2,7,11,15], target = 9
Output: [0,1]
This would be because the values in positions 0 and 1 sum up to the value 9.
(2+7 = 9)
One solution is by going with the brute force approach. This is O(n^2) and space complexity of O(1).
We first would want to have two indexes. One set at position 0, and one set at position 1. This would allow for a smooth scan of the entire array to compare each element to the desired target. An empty array to store the indices for return use.
From here, a while loop will be implemented, with proper return cases in the loop. Our first if statement will be verifying if we have found two values within the array that sum up to the target. If this sets as true, then we must store these values into the empty array (match) and then return the array.
What if idx2 scanned through the entire array to compare with idx1 and did not meet the desired summation? We will need to check that condition with another if statement. From there, we need to increment the idx1 to the next position, and set idx2 to be the value of idx1, then increment it. It would look something like this:
We then need to check if idx1 has finally reached the end of the array (meaning we did not find the desired summation). We would then simply have to return the empty array.
The entire solution would look like this:
And that's how you do it.
Summary:
We have covered the Two Sum coding problem that's one of LeetCode's coding challenges.
Link to the coding problem: https://leetcode.com/problems/two-sum/
Subscribe to my newsletter
Read articles from Kevin Rocha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
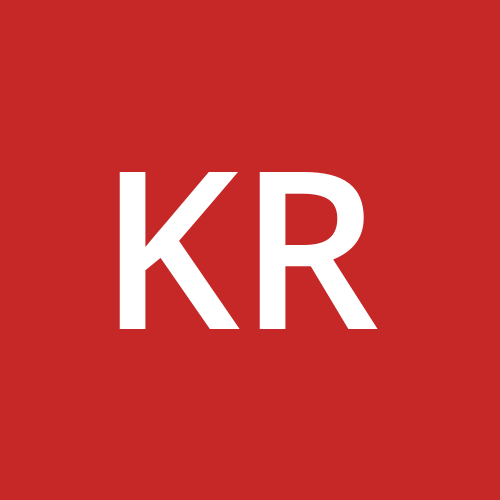