Arrays in JavaScript 2/3

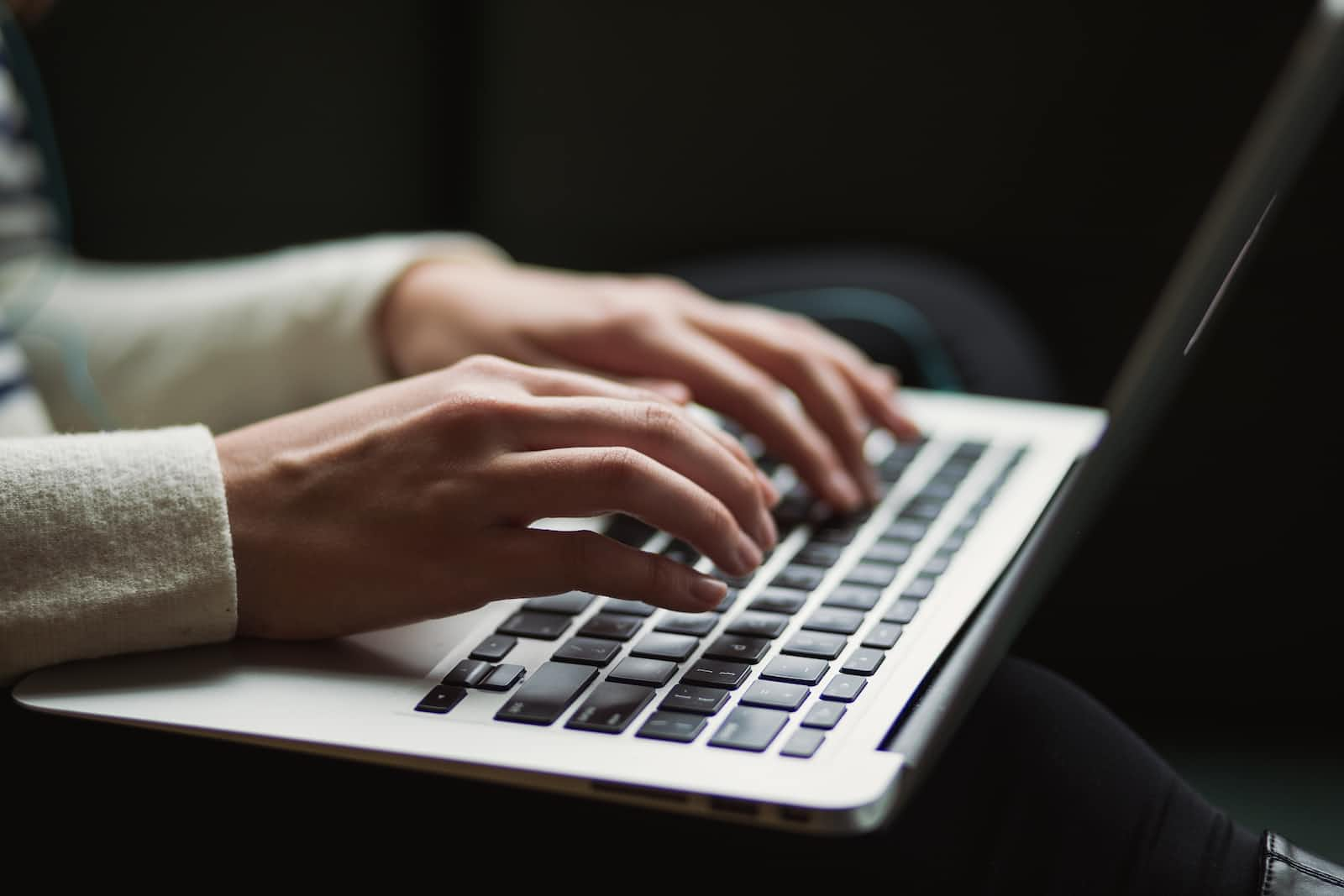
Recap
In our previous article, we delved into the fundamental concepts of arrays in JavaScript. We explored the significance of arrays, why they are essential, and examined how to create, access, and modify arrays. Building upon that foundation, this article will further expand our understanding by focusing on how we can leverage loops to manipulate arrays effectively. If you are new to this topic and haven't had the chance to read our previous article, we highly recommend starting there to ensure a comprehensive understanding of the concepts discussed. So, let's continue our exploration and enhance our proficiency in working with arrays using loops.
Here is the link of our previous blog
https://hashnode.com/post/clk0qd18z000209ms9wsu6iyk
Creating an Array using Loop
One of the powerful features of JavaScript is its ability to create arrays dynamically using loops. Loops allow us to iterate over a set of values and perform repetitive tasks, making them an ideal tool for creating arrays with a specific pattern or range of values
Let's explore some common ways to create arrays using loops in JavaScript:
Using for-loop
The for loop is commonly used when we know the number of iterations needed to create an array. We can initialize an empty array and then use the loop to push values into it. For example, let's create an array of numbers from 1 to 5:
let numbers = [];
for (let i = 1; i <= 5; i++) {
numbers.push(i);
}
console.log(numbers); // Output: [1, 2, 3, 4, 5]
Using while-loop
The while loop is useful when the number of iterations is unknown, and we have a condition to stop the loop. We can use it to create an array of even numbers. Here's an example:
let evenNumbers = [];
let i = 2;
while (i <= 10) {
evenNumbers.push(i);
i += 2;
}
console.log(evenNumbers); // Output: [2, 4, 6, 8, 10]
Using Array.from()
The Array.from() method creates a new array instance from an iterable object or array-like structure. We can use this method in combination with a mapping function to generate arrays with specific patterns. For instance, let's create an array of squares from 1 to 5:
let squares = Array.from({ length: 5 }, (_, index) => (index + 1) ** 2);
console.log(squares); // Output: [1, 4, 9, 16, 25]
By using loops, we can easily generate arrays of varying lengths and populate them with desired values. This flexibility allows us to efficiently create and manipulate arrays to suit our specific needs.
Conclusion
JavaScript provides multiple ways to create arrays using loops. Whether it's a simple range of numbers or more complex patterns, loops empower us to generate arrays dynamically, making JavaScript a versatile language for handling collections of data.
Accessing Arrays in JavaScript Using Loops
When working with arrays in JavaScript, loops provide a powerful mechanism for accessing and manipulating array elements. By using loops, we can iterate over the elements of an array and perform operations on each element individually. Let's explore some common ways to access array elements using loops, without relying on any array methods.
Using a for loop:
The for loop is a widely used construct for accessing array elements sequentially. By leveraging the loop variable as the index, we can access each element of the array one by one. Here's an example that demonstrates how to access and log each element of an array:
let fruits = ['apple', 'banana', 'orange', 'grape'];
for (let i = 0; i < fruits.length; i++) {
console.log(fruits[i]);
}
In this code snippet, the loop variable i
serves as the index to access each element of the fruits array. By incrementing
i` in each iteration, we traverse the entire array.
Using a while loop:
The while loop can also be utilized to access array elements. We can set a condition based on the array length to control the loop execution. Here's an example of accessing elements of an array using a while loop:
let numbers = [1, 2, 3, 4, 5];
let i = 0;
while (i < numbers.length) {
console.log(numbers[i]);
i++;
}
By initializing the loop variable i
as 0 and incrementing it after each iteration, we access each element of the numbers
array until the condition i < numbers.length
becomes false.
Using a for...of loop:
Introduced in ES6, the for...of loop provides a concise syntax for iterating over array elements without the need for an explicit index. It directly accesses the values rather than the indices of the array. Here's an example:
let colors = ['red', 'green', 'blue'];
for (let color of colors) {
console.log(color);
}
In this example, the loop variable color
represents each element of the colors array in each iteration. We can directly work with the value without accessing it via an index.
Using a for...in loop:
The for...in loop is used to iterate over the enumerable properties of an object. While it's not specifically designed for iterating over arrays, it can still be used to access array elements. However, caution should be exercised as it iterates over all enumerable properties, including non-index properties added to the array prototype. Here's an example:
let fruits = ['apple', 'banana', 'orange', 'grape'];
for (let index in fruits) {
console.log(fruits[index]);
}
In this code snippet, the loop variable index
represents the index of each element in the fruits
array. We can use this index to access the corresponding element.
By utilizing loops, such as the for loop, while loop, for...of loop, and for...in loop, we can dynamically access and work with the elements of an array in JavaScript. Each loop provides a different approach to iterating over array elements, allowing for efficient data manipulation and processing.
Conclusion
Loops offer a versatile approach to accessing array elements in JavaScript. By leveraging the power of loops, we can traverse arrays and perform operations on their elements without relying on any array methods. This flexibility empowers developers to handle and manipulate array data effectively, making JavaScript a powerful language for array-based operations.
Subscribe to my newsletter
Read articles from Kapil Chaudhary directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
