Building a very basic ToDo App with Flutter

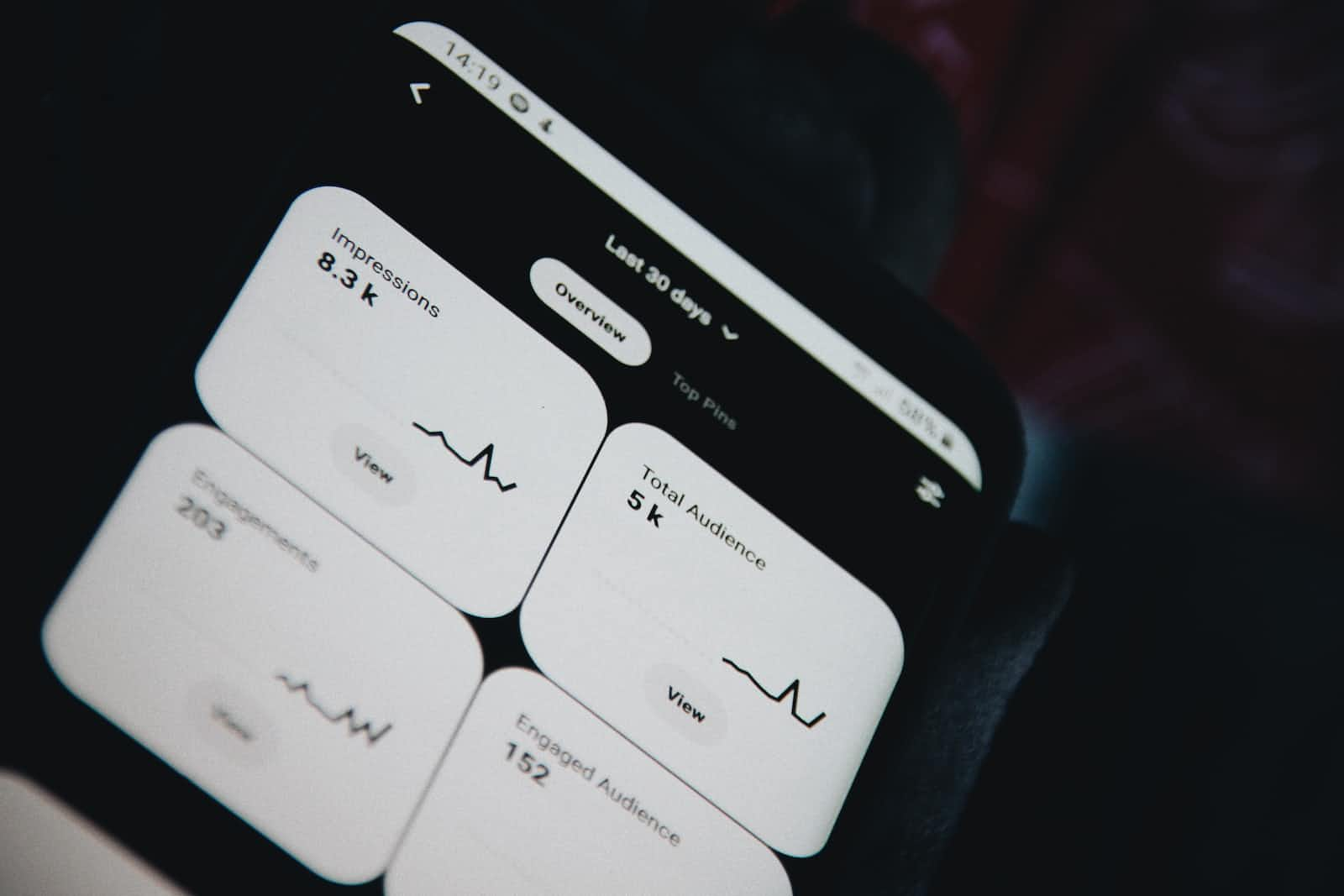
In this tutorial, we will build a simple ToDo application using the Flutter framework. The app will allow users to add and remove tasks from their to-do lists.
Prerequisites
Before we begin, make sure you have Flutter installed on your machine. You can follow the official Flutter installation guide for your operating system: Flutter Installation Guide
Setting up the Project
- Create a new Flutter project by running the following command in your terminal:
flutter create todo_app
Open the project in your preferred code editor.
Replace the contents of the
lib/main.dart
file with the following code:
import 'package:flutter/material.dart';
void main() => runApp(MaterialApp(
title: 'Testing todo application',
theme: ThemeData(
brightness: Brightness.dark,
primaryColor: Colors.green,
),
debugShowCheckedModeBanner: false,
home: const MyHomePage(),
));
class MyHomePage extends {
const MyHomePage({Key? key}) : super(key: key);
@override
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
final List<String> _todos = ['item1', 'item2', 'item2'];
void _addTodos() {
showDialog(
context: context,
builder: (BuildContext context) {
String _todo = '';
return AlertDialog(
title: const Text('Todo task'),
content: TextField(
decoration: const InputDecoration(
hintText: 'add your todos',
),
onChanged: (value) {
_todo = value;
},
),
actions: <Widget>[
TextButton(
onPressed: () {
Navigator.of(context).pop();
},
child: const Text('cancel'),
),
TextButton(
onPressed: () {
setState(() {
_todos.add(_todo);
});
Navigator.of(context).pop();
},
child: const Text('submit'),
),
],
);
},
);
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('ToDo App'),
centerTitle: true,
),
body: Center(
child: ListView.builder(
itemCount: _todos.length,
itemBuilder: (context, index) {
final newTodo = _todos[index];
return ListTile(
title: Text(newTodo),
subtitle: Text('You have added the new $newTodo'),
onTap: () {
setState(() {
_todos.removeAt(index);
});
},
);
},
),
),
floatingActionButton: FloatingActionButton(
onPressed: _addTodos,
child: const Icon(Icons.add),
),
);
}
}
Code Explanation
Let's go through the code and understand how our ToDo app works.
We start by importing the necessary packages. In this case, we are using
package:flutter/material.dart
for building the UI.The
main()
function is the entry point of our application. It sets up theMaterialApp
widget, which represents the root of our app. We provide a title, theme, and setdebugShowCheckedModeBanner
to false to hide the debug banner.We define a stateful widget called
MyHomePage
. This widget represents the home screen of our app.Inside the
MyHomePage
widget, we define a private list_todos
to store our todo items. Initially, we populate it with some dummy data.The
_addTodos()
method is responsible for showing a dialog to add new to-do items. It creates anAlertDialog
widget with a text field and two buttons: "cancel" and "submit". When the user taps the "submit" button, the entered to-do item is added to the_todos
list.In the
build()
method ofMyHomePage
, we create aScaffold
widget that provides the basic structure for our app. It contains anAppBar
with a title and a centered title.The body of the
Scaffold
is aCenter
widget that contains aListView.builder
. This builder generates aListTile
for each to-do item in the_todos
list. EachListTile
displays the to-do item's title and a subtitle indicating that it is a new item. Tapping on aListTile
removes the corresponding to-do item from the list.Finally, we add a
FloatingActionButton
at the bottom-right corner of the screen. When pressed, it calls the_addTodos()
method to show the add todo dialog.
Running the App
Save the changes and run the app using the following command:
flutter run
The app should launch on the connected device or emulator. You will see an app bar with the title "Alert Box Dialog" and a floating action button with an edit icon.
Subscribe to my newsletter
Read articles from Biplob Hossain Sheikh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Biplob Hossain Sheikh
Biplob Hossain Sheikh
I'm a Computer Science and Engineering graduate passionate about technology. I excel in web and mobile app development, DevOps, and cloud engineering. As a coding teacher, I love sharing knowledge and making complex concepts understandable. Skilled programmer and effective presenter, I stay up-to-date with emerging tech trends.