The Ultimate Beginner's Guide to React State Hook
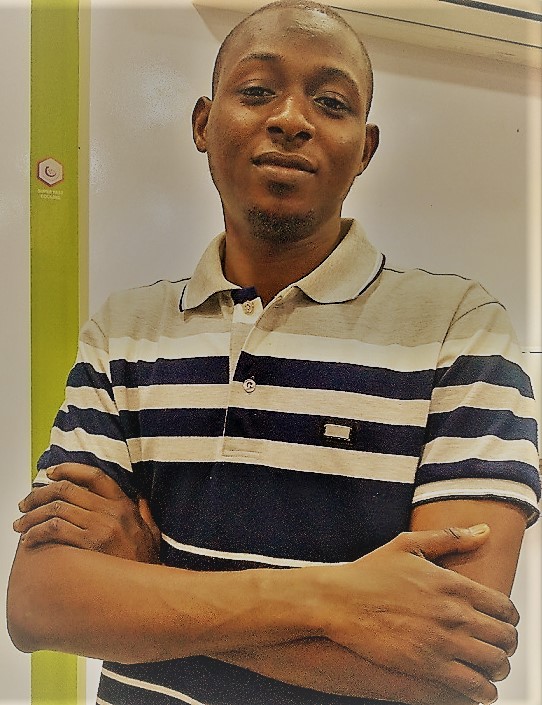
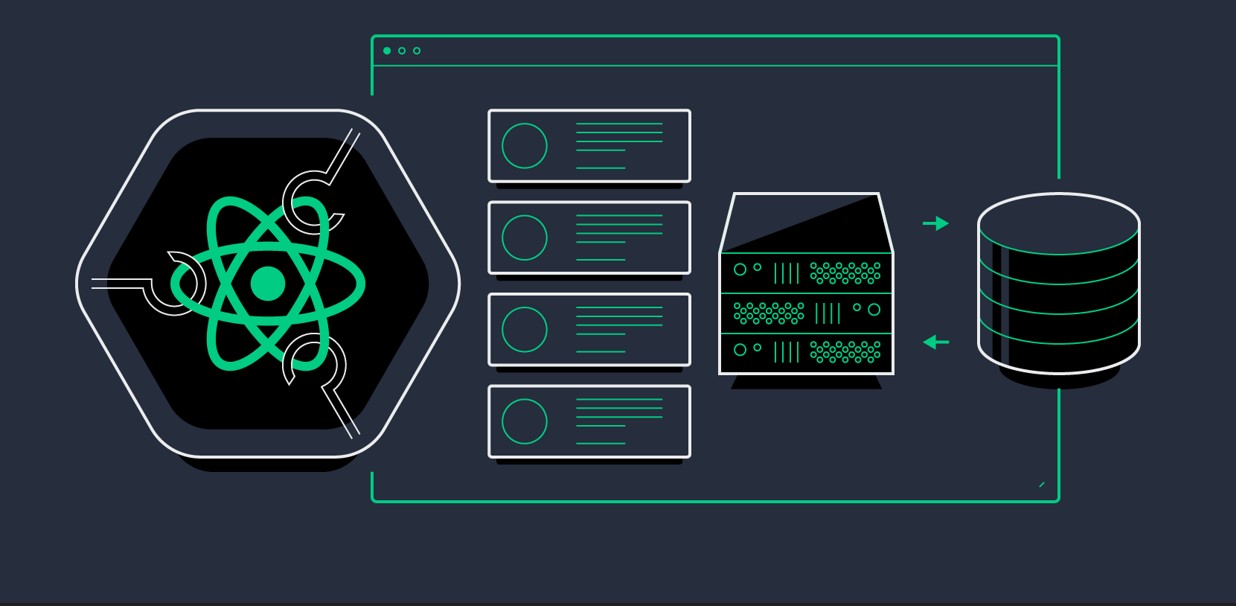
Introduction
State hook in React is not an easy concept to quickly understand and get hold of, but it gets better with time. This article breaks it down to fit into beginners understanding, it is well explained with simple terms for better understanding.
Prerequisite
You must have a good grasp of javascript and a basic understanding of React to be able to comprehend this write-up.
What is React State Hook
State hook in React is an object that lets you basically change some displays on the screen in response to a change of event(s).
For example, clicking on a social media like icon❤ (event) should change the color of the icon (state).
Components need to be able to "retain" things in their memory. the number of products in the cart, the data typed in a form field, the total cost of products bought, etc. In React this concept is called "State".
Why do we need the State Hook?
The State Hook is needed in our React projects to create and improve our project's interactivity, every website has a pinch of event changes here and there due to some set parameters. That's where State Hook comes in, to be able to "remember" these activities. So State Hook is highly essential in our React projects until state management starts getting complex, then state management tools will be employed at that level.
Local Variable vs State Variable
Changes made to the local variable will not reflect in the component when React renders.
Local variables are not "Sticky" in React, meaning that changes to them are not reflected in the rendered output.
Two things need to happen to update a component.
Preserve the data between renders.
Invoke React to re-renders the component with the new data.
The State Hook (useState
) provides the solution.
A State Variable to preserve data at re-rendering.
A State Function to set and update the variable and invokes re-rendering.
Using the State Hook
We begin with importing "useState"
at the top of the file.
import {useState} from 'react';
Then we create a function that'll hold the hook.
function arithmetic() {
const [add, setAdd] = useState(0)
}
add
is the state variable, which is referred to be the initialState
; while the setAdd
is the state setter function, which updates the initialState
to a new state. the square brackets [ ]
is called Array Destructuring.
Any object starting with the word use
in React is called a hook.
The state variable and the state setter function can be named anything, but the conventional way to name them is [anything, setAnything]
depending on their job in the code.
Example:
A simple example of increasing the initial state by 1 in the console, every time we click the "add" button.
import { useState } from "react";
const App = () => {
const [add, setAdd] = useState(0);
function handleclick() {
setAdd(add + 1);
console.log(add);
}
return <button onClick={handleclick}> Add </button>;
};
export default App;
Facts About the State Hook
The following facts are important to keep in mind while working with the State Hook, to understand how the hook works behind the hood.
React state is updated asynchronously: what this simply means is that updating the state in React is not done immediately. The state is updated after all event handlers have been completed.
The state is stored outside the components: State variables are tied to the component's instance, they stay in the memory provided the component remains visible on the screen.
Hooks can only be used at the top of the component: React relies on the order of the state to be able to map the values property to the state variable. this essentially means hooks cannot be used within a
"if"
statement,"loops"
or nested functions because they can impact the order in which, the state hook is invoked. so we can only call the state and every other hook at the top of the component to ensure a consistent order.
Choosing the State Structure
Below we'll discuss the best practices to employ when structuring the State Hook.
- Group related state variables: state variables that are closely related should be grouped together into an object to keep them organized.
Example:
import {useState} from 'react';
function App() {
const [firstName, setFirstName] = useState("");
const [lastName, setLastName] = useState("");
}
//Don't do this
import {useState} from 'react';
function App() {
const [person, setPerson] = useState({
firstName: "",
lastName: "",
});
}
//Do this
- Grouped state variables should not be deeply nested: deeply nested variables should be highly frowned upon, because it'll make our code hard to be updated or maintained. it's advisable to use a flat structure(minimal nested) as above.
Example:
import {useState} from 'react';
function App() {
const [person, setPerson] = useState({
firstName: "",
lastName: "",
contact: {
address: {
Street: ""
}
}
});
}
//This ia a bad practice, should be avoided.
Conclusion
React state hook allows you to change some components on the screen, it's needed whenever the need to "preserve" data arises, it's better than using local variables as it can't retain data.
We use "useState"
to define the State Hook at the top of the component, within a function. The hooks in React cannot be called in a loop or if statement as that would disturb its structure.
As a beginner, getting the hang of the State hook can be tough at first, but with consistency in using it, it'll become smooth.
Subscribe to my newsletter
Read articles from Adebakin Yusuf directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
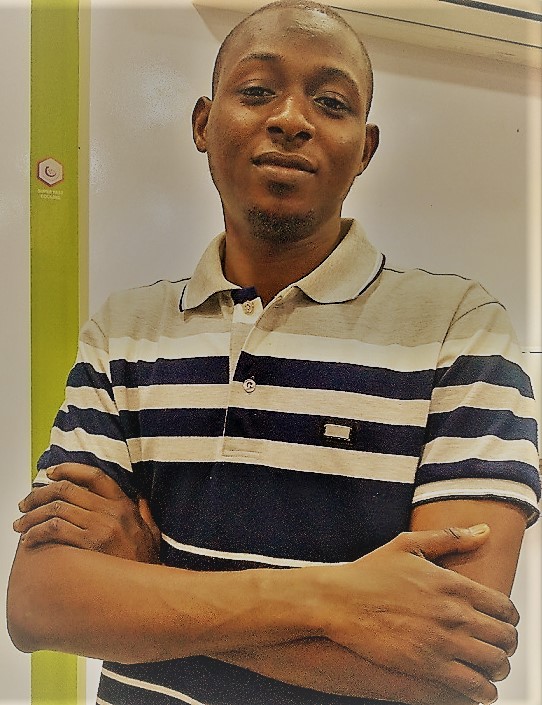
Adebakin Yusuf
Adebakin Yusuf
I'm a self-taught front-end developer and technical writer, experienced with JavaScript, React.js and Typescript.