Array Manipulation in JavaScript

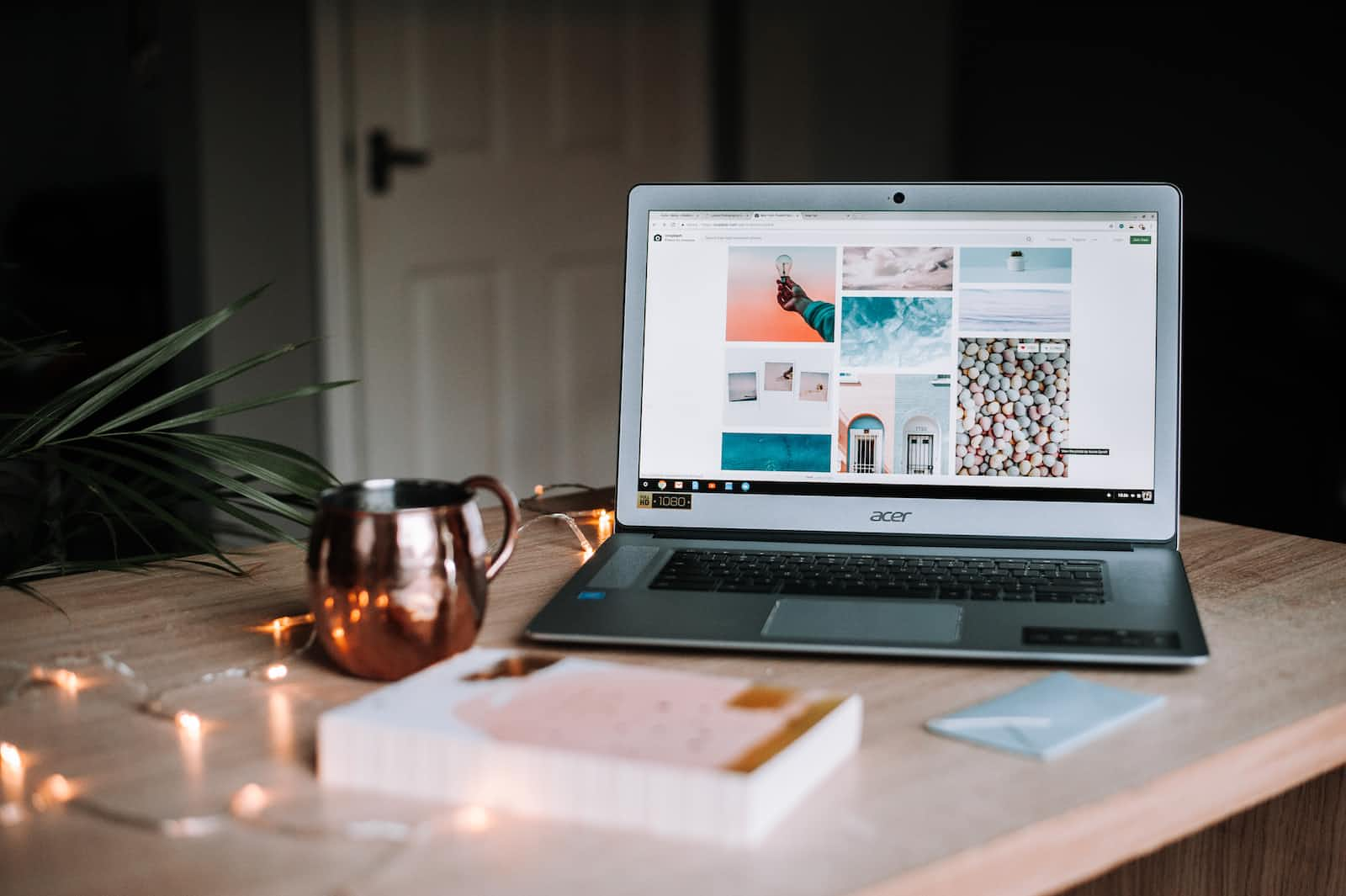
Introduction:
Arrays are a fundamental data structure in JavaScript, providing a flexible and efficient way to store and manage collections of values. JavaScript arrays come equipped with powerful built-in methods that allow for seamless manipulation, modification, and transformation of array data. Understanding how to manipulate arrays is essential for developers, as it enables them to efficiently organize, add, remove, or retrieve elements within an array. In this article, we will explore various techniques and methods for array manipulation in JavaScript, empowering you to harness the full potential of arrays in your coding endeavours. Whether you need to add, remove, or modify array elements, this article will equip you with the knowledge and tools to confidently manipulate arrays and leverage their capabilities in your JavaScript applications.
1. Adding Values from Both Ends
1.1 Introduction:
Arrays in JavaScript provide a versatile way to store and manipulate collections of data. One of the key features of arrays is the ability to add values from either the beginning or the end, depending on the specific requirements. In JavaScript, we have two methods for adding elements to arrays: unshift
and push
. This article will delve into these methods, explaining how they allow us to add elements at the start and end of an array, respectively.
1.2 Adding Elements at the Start: The unshift
Method
To insert an element at the beginning of an array, we use the unshift
method. It adds one or more elements to the front of the array, shifting the existing elements to higher indices. The newly added element will be located at index 0, pushing the rest of the array elements to higher indices.
const fruits = ['apple', 'banana', 'orange'];
console.log(fruits);//['apple', 'banana', 'orange']
fruits.unshift('mango', 'grape');
console.log(fruits); // ['mango', 'grape', 'apple', 'banana', 'orange']
1.3 Adding Elements at the End: The push
Method
To append an element to the end of an array, we utilize the push
method. It adds one or more elements to the back of the array, without affecting the existing elements. The new element will be inserted at the index array.length - 1
.
const fruits = ['apple', 'banana', 'orange'];
console.log(fruits);//['apple', 'banana', 'orange']
fruits.push('mango', 'grape');
console.log(fruits);// ['apple', 'banana', 'orange', 'mango', 'grape']
1.4 Conclusion:
Understanding how to add elements to arrays in JavaScript is crucial for manipulating data effectively. With the unshift
method, we can add elements to the beginning of an array, while the push
method allows us to append elements at the end. By employing these methods appropriately, JavaScript developers can efficiently modify arrays to suit their specific needs, making the language a powerful tool for managing collections of data.
2 Removing Values from Both Ends
2.1 Introduction:
Arrays in JavaScript provide a convenient way to store and manipulate collections of data. When working with arrays, we often need to remove elements from either the beginning or the end. In JavaScript, we have two methods for removing elements from arrays: shift
and pop
. In this article, we will explore these methods and understand how they allow us to remove elements from the start and end of an array, respectively.
2.2 Removing Elements from the Start: The shift
Method
The shift
method removes the first element from an array and returns it. This operation modifies the original array, shifting the remaining elements down by one position. The removed element is returned, and if the array is empty, the method returns undefined.
const fruits = ['apple', 'banana', 'orange'];
const firstFruit = fruits.shift();
console.log(firstFruit);//'apple'
console.log(fruits); //['banana', 'orange']
2.3 Removing Elements from the End: The pop
Method
The pop
method removes the last element from an array and returns it. This operation also modifies the original array, reducing its length by one. The removed element is returned, and if the array is empty, the method returns undefined
.
const fruits = ['apple', 'banana', 'orange']; const lastFruit = fruits.pop(); // lastFruit: 'orange' // fruits: ['apple', 'banana']
2.4 Conclusion:
Understanding how to remove elements from arrays in JavaScript is essential for manipulating array data effectively. By using the shift
method, we can remove elements from the start of an array, while the pop
method allows us to remove elements from the end. These methods enable developers to modify arrays dynamically, ensuring efficient data management in JavaScript applications.
Summary:
Array manipulation is a crucial aspect of working with data in JavaScript. Throughout this article, we have explored the various methods available for manipulating arrays, including push
, pop
, shift
, and unshift
. We learned that the push
method adds elements to the end of an array, while pop
removes elements from the end. On the other hand, the shift
method removes elements from the beginning, and unshift
adds elements to the start of an array. By understanding and utilizing these methods effectively, developers can easily modify arrays to suit their specific needs, whether it's adding or removing elements from either end. Arrays provide a versatile and efficient way to store and manage collections of data, making them an invaluable tool for JavaScript developers. With the knowledge gained from this article, you are now equipped to manipulate arrays with confidence, enabling you to create powerful and dynamic JavaScript applications.
Subscribe to my newsletter
Read articles from Kapil Chaudhary directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
