The Power of Array Concatenation in JavaScript: Combining Arrays with Ease

Table of contents
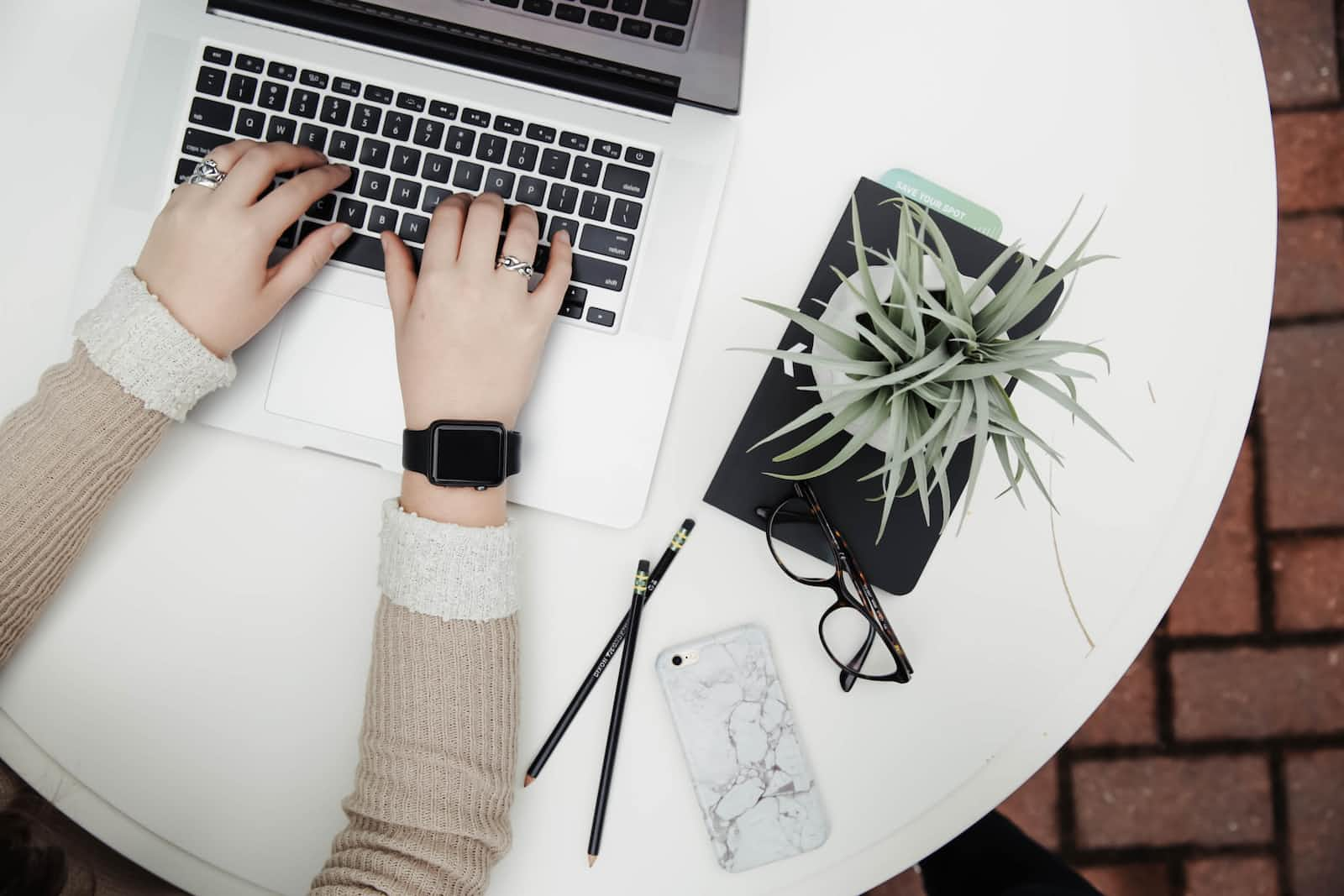
Introduction:
Arrays are a fundamental data structure in JavaScript, providing a powerful way to store and manipulate collections of elements. When working with arrays, there are scenarios where you need to combine multiple arrays into a single, cohesive unit. In such cases, the concat()
method comes to the rescue. In this article, we will explore the capabilities of array concatenation in JavaScript and uncover its potential in array manipulation. By understanding the concat()
method and examining practical examples, you'll be equipped to merge arrays seamlessly and efficiently.
Understanding Array Concatenation:
The concat()
method in JavaScript allows you to merge two or more arrays, creating a new array that contains all the elements from the original arrays. It does not modify the original arrays; instead, it returns a new array with the concatenated elements. The syntax for concat()
is as follows:
const newArray = array1.concat(array2, array3, ..., arrayN);
Here, array1
is the base array, and array2
to arrayN
are the arrays to be concatenated. The resulting newArray
contains all the elements from the original arrays in the order they were concatenated.
Practical Examples:
Let's explore practical examples to understand the array concatenation process and how it can be applied in JavaScript.
Example 1: Concatenating Two Arrays:
const array1 = [1, 2, 3];
const array2 = [4, 5, 6];
const newArray = array1.concat(array2);
console.log(newArray); // [1, 2, 3, 4, 5, 6]
Explanation: In this example, array1.concat(array2)
combines the elements from array1
and array2
into a new array, newArray
. The resulting array contains all the elements from both arrays, maintaining the order in which they were concatenated.
Example 2: Concatenating Multiple Arrays:
const array1 = ["a", "b"];
const array2 = ["c", "d"];
const array3 = ["e", "f"];
const newArray = array1.concat(array2, array3);
console.log(newArray); // ["a", "b", "c", "d", "e", "f"]
Explanation: Here, array1.concat(array2, array3)
combines the elements from array1
, array2
, and array3
into a single array, newArray
. The resulting array contains all the elements in the order they were concatenated.
Example 3: Concatenating Arrays with Different Data Types:
const array1 = [1, 2];
const array2 = ["a", "b"];
const array3 = [true, false];
const newArray = array1.concat(array2, array3);
console.log(newArray); // [1, 2, "a", "b", true, false]
Explanation: In this example, array1.concat(array2, array3)
combines arrays containing different data types. The resulting newArray
contains elements of numbers, strings, and booleans, providing a versatile way to merge arrays with heterogeneous data.
Example 4: Concatenating Arrays of Objects:
const array1 = [{ id: 1, name: "John" }];
const array2 = [{ id: 2, name: "Jane" }];
const array3 = [{ id: 3, name: "Bob" }];
const newArray = array1.concat(array2, array3);
console.log(newArray);
// [{ id: 1, name: "John" }, { id: 2, name: "Jane" }, { id: 3, name: "Bob" }]
Explanation: Here, array1.concat(array2, array3)
merges arrays of objects into a single array, newArray
. The resulting array contains all the object elements in the order they were concatenated, enabling you to combine and manipulate complex data structures.
Example 5: Concatenating an Empty Array:
const array1 = [1, 2, 3];
const emptyArray = [];
const newArray = array1.concat(emptyArray);
console.log(newArray); // [1, 2, 3]
Explanation: In this example, array1.concat(emptyArray)
concatenates array1
with an empty array, resulting in the original array array1
being returned.
Example 6: Concatenating Arrays with Nested Arrays:
const array1 = [1, 2];
const array2 = [[3, 4], [5, 6]];
const newArray = array1.concat(array2);
console.log(newArray); // [1, 2, [3, 4], [5, 6]]
Explanation: In this example, array1.concat(array2)
concatenates array1
with array2
, which contains nested arrays. The resulting newArray
contains all the elements from array1
, as well as the nested arrays from array2
.
Additional Notes:
The concat()
method is a flexible tool for array manipulation, and it offers several advantages:
It can concatenate arrays of any length, making it suitable for merging arrays of varying sizes.
The original arrays remain unchanged, ensuring data integrity and preserving the original data structures.
The
concat()
method can be chained with other array methods to perform complex array operations.
Conclusion:
Array concatenation is a powerful feature in JavaScript, enabling you to merge arrays effortlessly. The concat()
method offers a simple and efficient way to create a new array by combining elements from multiple arrays. Whether you need to merge two arrays, concatenate multiple arrays, or even work with nested arrays, concat()
provides a flexible solution.
By harnessing the capabilities of array concatenation, you can streamline your code and manipulate arrays more effectively. Whether you're working with homogeneous or heterogeneous data, or even complex data structures, array concatenation allows you to create cohesive arrays tailored to your specific needs. Embrace the power of array concatenation in JavaScript and unlock new possibilities in array manipulation.
Subscribe to my newsletter
Read articles from Kapil Chaudhary directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
