How to setup react ?
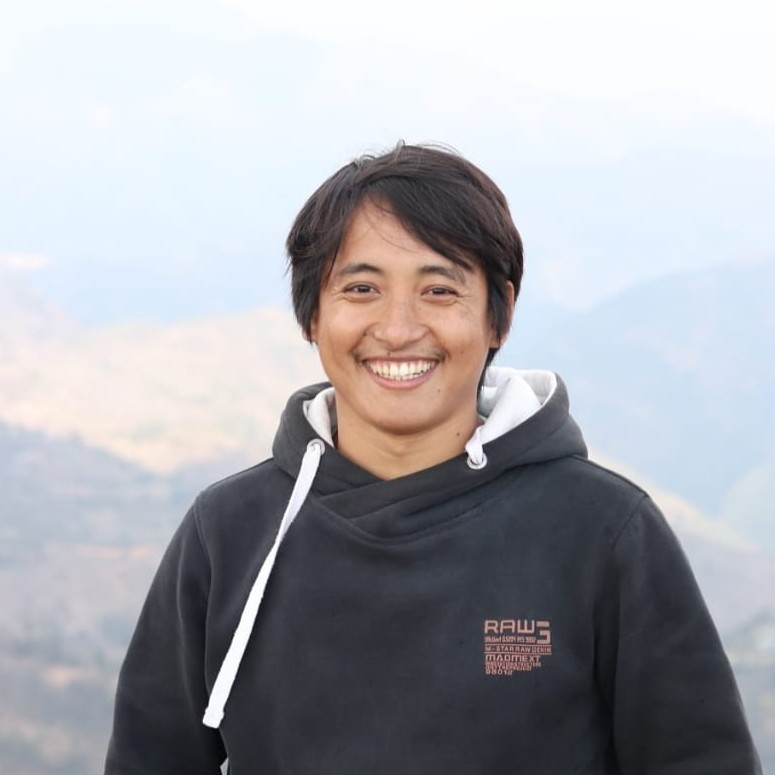
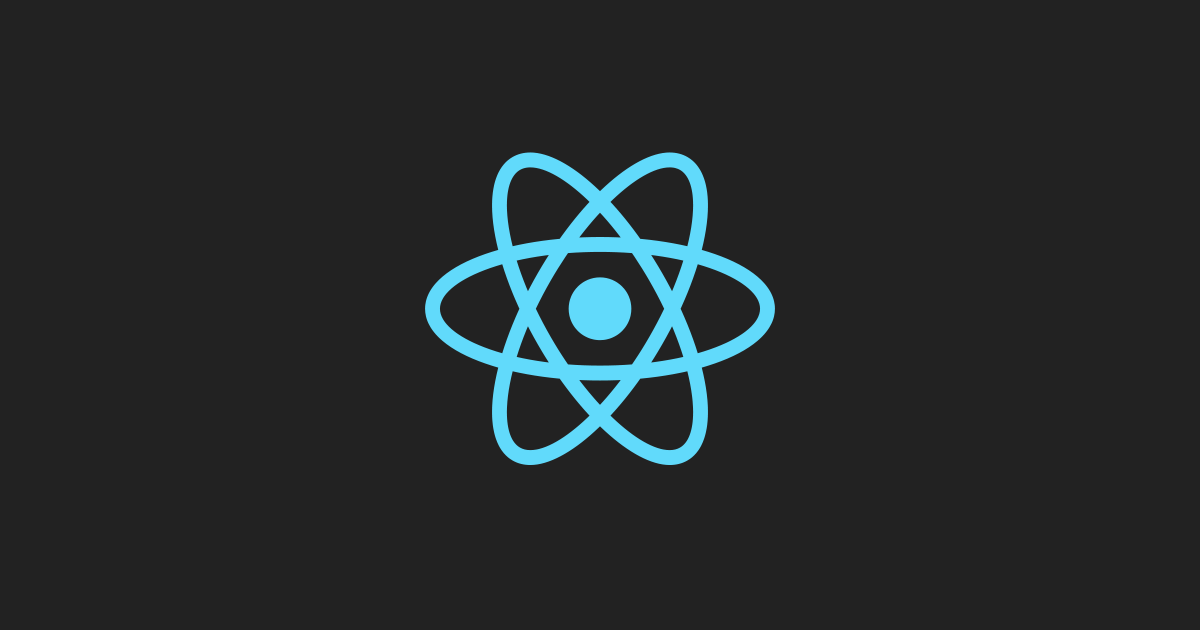
To create react app.
npx create-react-app my-app
cd my-app
npm start
To create a project called my-app, we need to run this command
npx create-react-app my-app
and then change the directory to the my-app folder and run this command
cd my-app
to run the app
npm start
we can see lots of files after the app is executed.
- package.json -> All dependencies, scripts and versions are added to the package.json file.
2. src -> In this folder app.js, index.js and index.css are located.
- index.js -> Whenever the page is loaded this is the first file that will be
executed. this is the entry point of reaction.
let's see what is inside the index.js, and understand how the code is working
as we can see in the above code snippets some of the files, packages and components are imported.
on line 7, 'root
' element is fetched by using getElementById and stored in the root variable and this root element is stored inside the public folder -> index.html
const root = ReactDOM.createRoot(document.getElementById('root'));
Here's a breakdown of what the code does:
ReactDOM
: This is the entry point to the React DOM library, which allows you to interact with the DOM and render React components into the actual HTML page.createRoot(element: DOMContainer, options?: RootOptions)
: This function is used to create a root for your React application. A root is the entry point of a React tree. It takes two arguments:element
: The DOM container where you want to render your React application. In this case, it is the element with the ID "root". This means that the React application will be rendered inside the HTML element with the ID "root."options
(optional): An object that allows you to pass additional options to the root. These options are not required for basic usage.
After calling ReactDOM.createRoot()
, you can use the returned root to render your React components into the specified DOM container.
const root = ReactDOM.createRoot(document.getElementById('root'));
// You can then render a React component into the root
root.render(<App />);
In the above code, we are renderering App component
This App component is nothing but a function and we are returning 'Hello World'.
and we need to run this command called npm start
to execute the react app.
now, we have successfully created our first react app .
In this tutorial, I have shown you how to set up react project and to build the first React app step by step. I hope you like this.
Subscribe to my newsletter
Read articles from Santosh Thapa directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
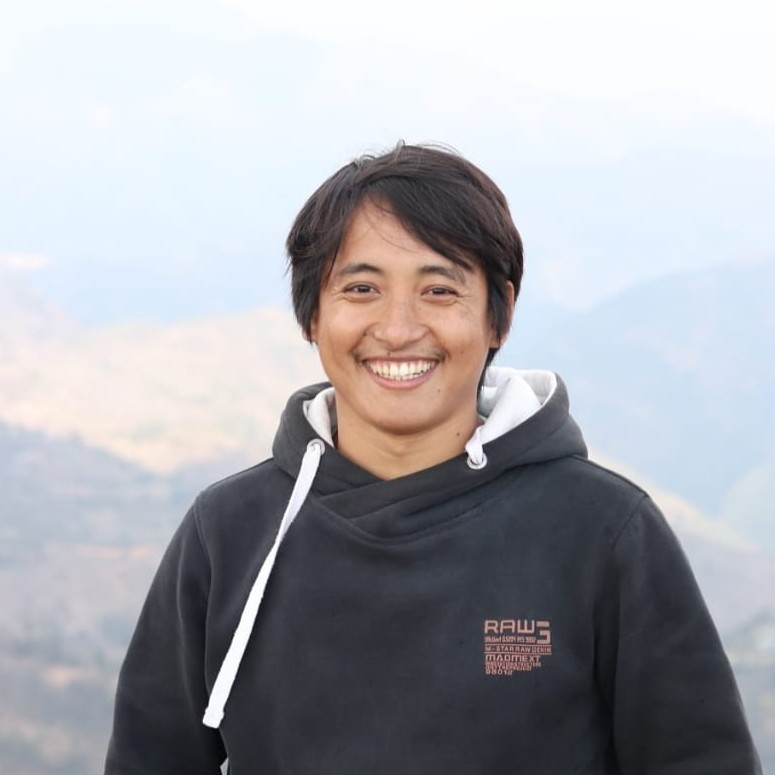
Santosh Thapa
Santosh Thapa
I am a Full Stack developer!