Best Toast Library in React

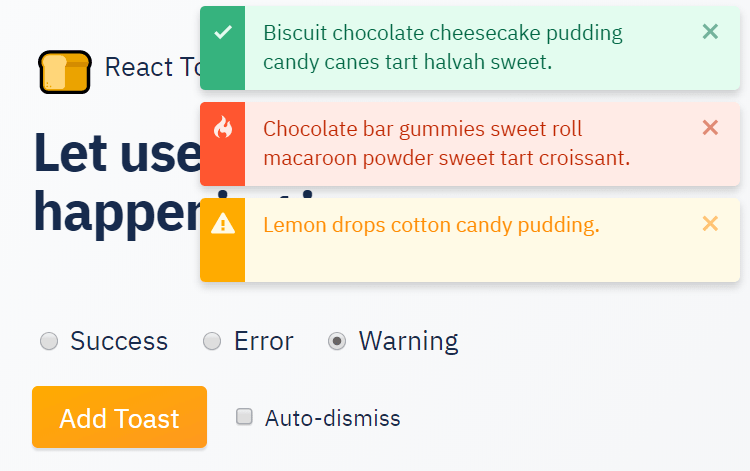
what is toast
A toast is a non-modal dialog that appears and disappears in the span of a few seconds. ... Typically, toast messages display one or two-line non-critical messages that do not require user interaction.
1. react-toastify
react-toastify is one of the most useful toast libraries out there. It has tons of cool features like;
Swipe to close
Display a React element inside the toast
Can remove a toast programmatically
much more.
Example for react-toastify:
First, you need to install the package. You can do this by running the following command in your project's root directory:
npm install react-toastify
Once the package is installed, you can start using react-toastify
in your components. Here's an example of a simple notification:
import React from 'react';
import { ToastContainer, toast } from 'react-toastify';
import 'react-toastify/dist/ReactToastify.css';
const MyComponent = () => {
const handleButtonClick = () => {
toast.success('Hello, world!', {
position: toast.POSITION.TOP_RIGHT
});
};
return (
<div>
<button onClick={handleButtonClick}>Show Notification</button>
<ToastContainer />
</div>
);
};
export default MyComponent;
In the above example, we import the necessary components from react-toastify
and the associated CSS file. We define a button with an onClick
event handler, which triggers the toast.success
function when clicked. The toast.success
function displays a success notification with the message "Hello, world!" in the top-right position.
The ToastContainer
component is used to render the notification container. It should be placed at the root level of your application or at a suitable location where the notifications should appear.
Remember to import the CSS file and include the ToastContainer
component in your root component or a suitable parent component where you want the notifications to be displayed.
This is a basic example, but react-toastify
provides many customization options for notifications, such as different types, positions, timeouts, and more. You can explore the documentation of react-toastify
for more details on how to use these options to fit your specific needs.
Please note that the example provided assumes you are using a bundler like Webpack or Parcel to compile your React application.
2. react-hot-toast
react-hot-toast is a small, accessible and easily customizable toast library that has;
Promise API
Headless Hooks
Lightweight (less than 5kb - including the styles)
Example for react-hot-toast:
First, you need to install the package. You can do this by running the following command in your project's root directory:
npm install react-hot-toast
Once the package is installed, you can start using react-hot-toast
in your components. Here's an example:
import React from 'react';
import { Toaster, toast } from 'react-hot-toast';
const MyComponent = () => {
const handleButtonClick = () => {
toast.success('Hello, world!');
};
return (
<div>
<button onClick={handleButtonClick}>Show Notification</button>
<Toaster />
</div>
);
};
export default MyComponent;
In the above example, we import the necessary components from react-hot-toast
. We define a button with an onClick
event handler, which triggers the toast.success
function when clicked. The toast.success
function displays a success notification with the message "Hello, world!".
The Toaster
component is used to render the toast container. It should be placed at the root level of your application or at a suitable location where the notifications should appear.
Remember to include the Toaster
component in your root component or a suitable parent component where you want the notifications to be displayed.
react-hot-toast
provides various options and customization features to control the appearance and behavior of the toasts. You can customize the position, duration, styling, and more. You can refer to the documentation of react-hot-toast
for a detailed overview of all the available options and customization possibilities.
Please note that the example provided assumes you are using a bundler like Webpack or Parcel to compile your React application.
3. react-toast-notifications
react-toast-notifications is also a cool library to get work with but unfortunately, it is no longer maintained.
Example for react-toast-notification
First, you need to install the package. You can do this by running the following command in your project's root directory:
npm install react-toast-notifications
Once the package is installed, you can start using react-toast-notifications
in your components. Here's an example:
import React from 'react';
import { useToasts } from 'react-toast-notifications';
const MyComponent = () => {
const { addToast } = useToasts();
const handleButtonClick = () => {
addToast('Hello, world!', {
appearance: 'success',
autoDismiss: true,
});
};
return (
<div>
<button onClick={handleButtonClick}>Show Notification</button>
</div>
);
};
export default MyComponent;
In the above example, we import the necessary components and hook from react-toast-notifications
. We use the useToasts
hook to access the addToast
function, which is used to show a toast notification.
The addToast
function accepts two parameters: the content of the notification (in this case, the message "Hello, world!") and an options object. In the example, we provide appearance: 'success'
to display a success notification, and autoDismiss: true
to automatically dismiss the notification after a certain duration.
You can customize the appearance, duration, position, and other properties of the toast notifications according to your needs. The react-toast-notifications
package provides various options for customization.
Please note that the example provided assumes you are using a bundler like Webpack or Parcel to compile your React application.
Conclusion:
In conclusion, there are several excellent libraries available for implementing toast notifications in React applications. Whether you choose react-toastify
, react-hot-toast
, or react-toast-notifications
, each library provides a convenient way to display user-friendly notifications.
react-toastify
offers a robust and highly customizable solution with support for different notification types, positions, and animation options. It provides a simple API for displaying notifications and is widely used and well-documented.
On the other hand, react-hot-toast
focuses on simplicity and ease of use. It offers a minimalistic API while still allowing for customization options such as position and duration. It is lightweight, easy to set up, and provides a clean and intuitive interface.
Lastly, react-toast-notifications
provides a comprehensive solution with extensive customization options, including appearance, auto-dismissal, and transition animations. It offers a hook-based approach, allowing for flexible integration into React components.
Ultimately, the choice of the best library for your project depends on your specific requirements and preferences. All three libraries mentioned above are actively maintained, have good community support, and are suitable for most use cases.
When implementing toast notifications, consider factors such as ease of use, customization options, community support, and integration with your existing codebase. Review the documentation and examples for each library to determine which one aligns best with your project's needs.
Regardless of the library you choose, implementing toast notifications can greatly enhance the user experience of your React application by providing informative and non-intrusive feedback to your users.
Subscribe to my newsletter
Read articles from Kunal Pakhale directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
