How to Extend the Default Django User Model

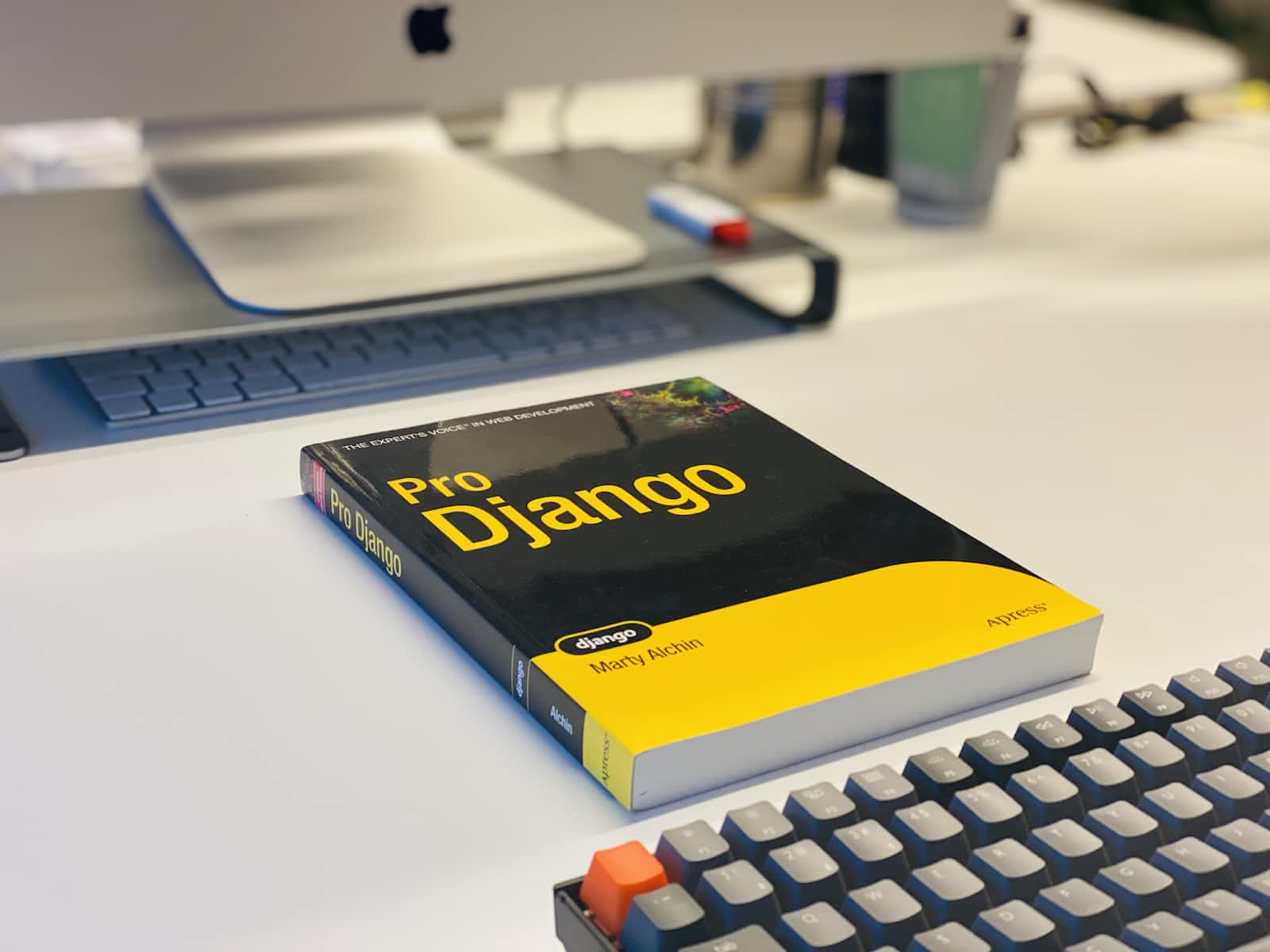
Introduction:
The Django web framework provides a powerful authentication system out-of-the-box with its default User model. However, there are often cases where we need to add additional fields or functionality to the User model to suit our application's specific requirements. In this blog post, we will explore how to extend the default Django User model to accommodate these customizations.
Why Extend the Default User Model?
Extending the default User model allows us to store additional information about our users without modifying the core Django code. This approach ensures compatibility with future Django updates and simplifies database migrations. By extending the User model, we can easily add fields such as a user's profile picture, contact information, or any other relevant data.
Creating a Custom User Model
To extend the default User model, we need to create a custom model that inherits from AbstractUser or AbstractBaseUser. The choice between the two depends on the level of customization required.
AbstractUser: If we only need to add extra fields to the existing User model, AbstractUser is the recommended base class. To create a custom User model using AbstractUser, follow these steps:
a. Create a new Django app (if not already created) to house our custom User model.
b. In the app's models.py file, import AbstractBaseUser and BaseUserManager from django.contrib.auth.models.
c. Create a new class that inherits from AbstractUser.
d. Add any additional fields or methods required for your application.
from django.contrib.auth.models import AbstractUser class CustomUser(AbstractUser): # Add custom fields or methods here pass
e. Update the AUTH_USER_MODEL setting in settings.py to point to our custom User model.
# settings.py AUTH_USER_MODEL = 'your_app_name.CustomUser'
AbstractBaseUser: If we require more extensive customization, such as using email instead of username for authentication, we should use AbstractBaseUser. This approach allows us to define our own authentication fields and methods. The steps for creating a custom User model using AbstractBaseUser are as follows:
a. Create a new Django app (if not already created) to house our custom User model.
b. In the app's models.py file, import AbstractBaseUser and BaseUserManager from django.contrib.auth.models.
c. Create a new class that inherits from AbstractBaseUser.
d. Define the required fields and methods for authentication.
e. Implement a custom UserManager that inherits from BaseUserManager.
from django.contrib.auth.models import AbstractBaseUser, BaseUserManager class CustomUserManager(BaseUserManager): # Implement custom methods for user management pass class CustomUser(AbstractBaseUser): # Define custom fields here pass def get_full_name(self): # Custom method implementation pass def get_short_name(self): # Custom method implementation pass # ... other required methods for authentication objects = CustomUserManager()
f. Update the AUTH_USER_MODEL setting in settings.py to point to our custom User model and UserManager.
# settings.py
AUTH_USER_MODEL = 'your_app_name.CustomUser'
Migrating Existing Data:
After creating the custom User model, we need to migrate the existing data to the new model. Django provides a built-in command called makemigrations that generates the necessary migration files. Run the following commands in your terminal:
python manage.py makemigrations
python manage.py migrate
Conclusion
Extending the default Django User model allows us to add custom fields and functionality without modifying the core Django code. By following the steps outlined in this blog post, you can create a custom User model tailored to your application's specific requirements. Remember to plan your customizations carefully and consider any potential impacts on existing code and database relationships.
Subscribe to my newsletter
Read articles from Johanan Oppong Amoateng directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Johanan Oppong Amoateng
Johanan Oppong Amoateng
I am a self taught backend and mobile developer. I use Django,Flask or Node for my backends and for mobile i use either Jetpack Compose or Flutter. I love watching anime