Writing an Encryption Script Using Fernet Cryptography in Python

Table of contents
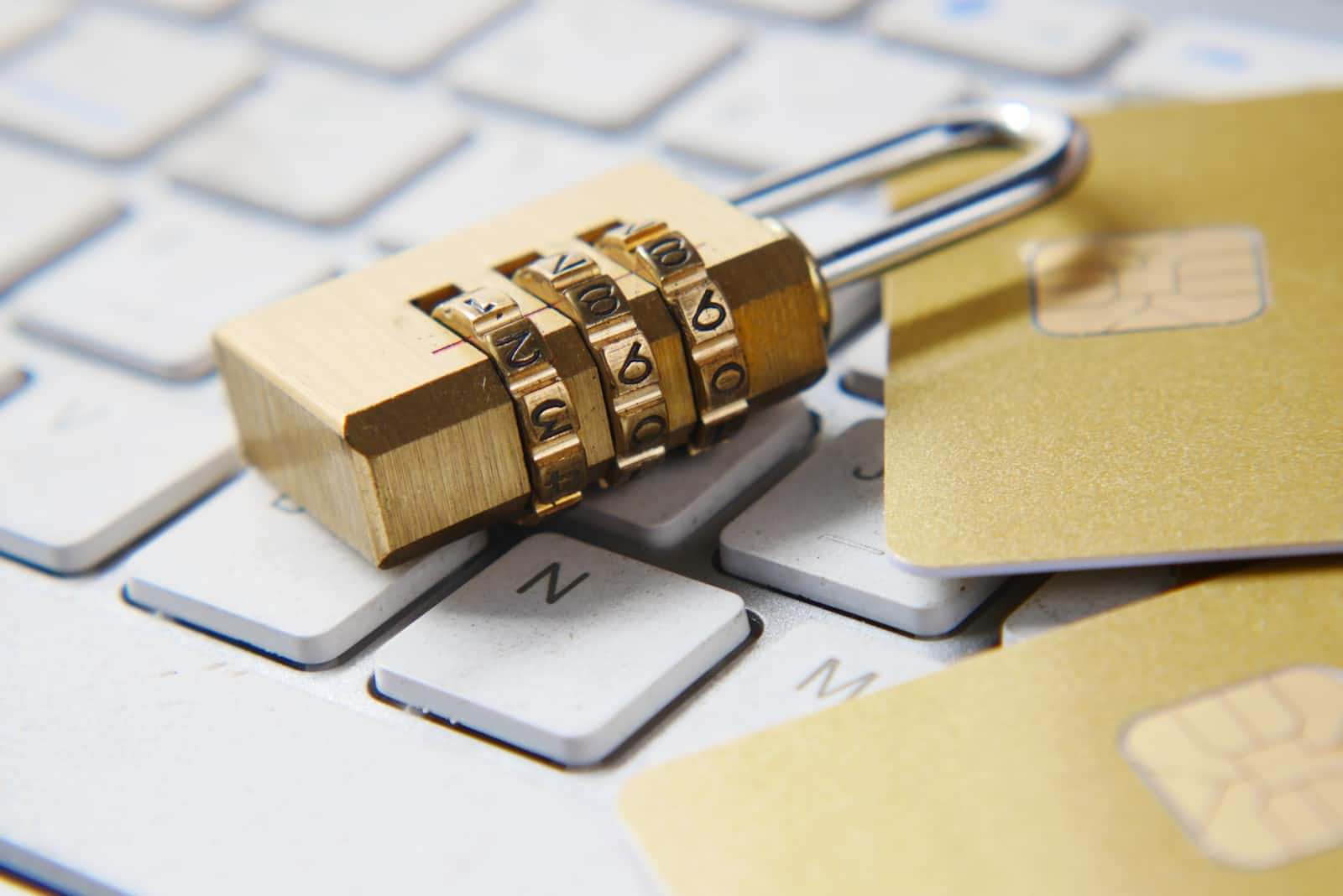
Introduction
With the increasing need for data security and privacy, encryption plays a crucial role in safeguarding sensitive information. Python, being a versatile programming language, offers several cryptographic libraries to implement encryption algorithms effectively. One such library is cryptography, which provides support for Fernet cryptography—a symmetric encryption algorithm that ensures both confidentiality and integrity of data. In this article, we will explore how to write an encryption script using Fernet cryptography in Python.
Getting Started
To get started with writing our encryption script, we need to install a Python module cryptography
which is required to work with Fernet cryptography in Python.
pip install cryptography
Next, we'll create our first script file key.py
to generate and save our encryption and decryption key.
#!/usr/bin/python3
from cryptography.fernet import Fernet
# generate key for encryption
encryption_key = Fernet.generate_key()
# save the encryption and decrytion key
with open('encrypt.key', 'wb') as key_file:
key_file.write(encryption_key)
From the above code, we import the Fernet object from the cryptography.fernet module, we then call the generate_key method on the Fernet object to generate our key and save it in the encrytion_key variable.
Then, we'll save the generated key to a file encrypt.key
in a binary format. But why binary format? well, that's because the Fernet key for encryption decryption must be in binary representation.
Let's run our key.py
script to generate the key
chmod 555 key.py
# lets check if permission as been updated
ll
-r-xr-xr-x 1 ayo ayo 431 Jul 16 11:20 key.py
# run script
./key.py
ll
-rwxrwxrwx 1 ayo ayo 431 Jul 16 11:20 key.py
-rw-rw-r-- 1 ayo ayo 44 Jul 16 11:00 encrypt.key
Now that we have our key generated, let's create our encrypt.py
script for our encryption and decryption.
In the encrypt.py script file, let's start by importing all the necessary modules for the script.
#!/usr/bin/python3
from cryptography.fernet import Fernet
from sys import argv
from dataclasses import dataclass
Next, let's read our key to the script in a binary representation
#!/usr/bin/python3
from cryptography.fernet import Fernet
from sys import argv
from dataclasses import dataclass
# open the key
with open('encrypt.key', 'rb') as key_file:
encryption_key = key_file.read()
Next, we'll create our Encryption
class for encryption and decryption
#!/usr/bin/python3
from cryptography.fernet import Fernet
from sys import argv
from dataclasses import dataclass
# open the key
with open('encrypt.key', 'rb') as key_file:
encryption_key = key_file.read()
@dataclass
class Encryption:
data: str
def encrypt_data(self) -> str:
f = Fernet(encryption_key)
encrypted_data = f.encrypt(self.data.encode()).decode()
print(encrypted_data)
def decrypt_data(self) -> str:
f = Fernet(encryption_key)
decrypted_data = f.decrypt(self.data.encode()).decode()
print(decrypted_data)
Okay, let's take a second to understand what's going on inside our Encryption class, we used the Python dataclass decorator to store instance data for our class, the data attribute is a string to encrypt or decrypt. Next, we have two instance methods encrypt_data and decrypt_data, in the two methods we instantiate the Fernet class with the loaded encryption key passed as a parameter, we then called the encrypt and decrypt method of the Fernet class on the f instance of the Fernet class with the object string data passed as an argument.
The encode() method is used the convert the string data to a binary representation for Fernet and decode() is used to convert the binary data back to a string for the user.
Now, let's add the logic to encrypt or decrypt from the command line base on user input.
#!/usr/bin/python3
from cryptography.fernet import Fernet
from sys import argv # for commandline argument
from dataclasses import dataclass
# open the key
with open('encrypt.key', 'rb') as key_file:
encryption_key = key_file.read()
@dataclass
class Encryption:
""" Encryption class
"""
data: str
def encrypt_data(self) -> str:
""" encrypt data method
"""
# create a Fernet object
f = Fernet(encryption_key)
encrypted_data = f.encrypt(self.data.encode()).decode()
print(encrypted_data)
def decrypt_data(self) -> str:
""" decrypt data method
"""
f = Fernet(encryption_key)
decrypted_data = f.decrypt(self.data.encode()).decode()
print(decrypted_data)
if argv[1] == 'encrypt':
# if the second argument passed from the command line is encrypt
encryption = Encryption(argv[2])
encryption.encrypt_data()
elif argv[1] == 'decrypt':
# if decrypt
encryption = Encryption(argv[2])
encryption.decrypt_data()
Let's run the encrypt script to encrypt some data.
➜ scripts ./encrypt.py encrypt ThisIsASecret
gAAAAABks9r2PZqMpgGnYHeAwagIQJPcfD_EPepLvp_byxskjXpbutAcHa3XKkup6W5_tfhUyGKwS62-EX2wpkKKW-_7wNJUzQ==
Now, we have our encrypted string, let's decrypt and see if we'll get back out string ThisIsASecret.
➜ scripts ./encrypt.py decrypt gAAAAABks9r2PZqMpgGnYHeAwagIQJPcfD_EPepLvp_byxskjXpbutAcHa3XKkup6W5_tfhUyGKwS62-EX2wpkKKW-_7wNJUzQ==
ThisIsASecret
Voila!!, we have it, our secret string
Wrap Up
We've just written a simple script that can be used to encrypt and decrypt a string using Fernet cryptography in Python.
Hope this was fun!
If you enjoy the tutorial, be sure to like and subscribe to my newsletter for more scripting and articles.
Thank you.
Subscribe to my newsletter
Read articles from Alaran Ayobami directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Alaran Ayobami
Alaran Ayobami
A nerd in a learning loop