Top 30 React Native Interview Questions For All Levels
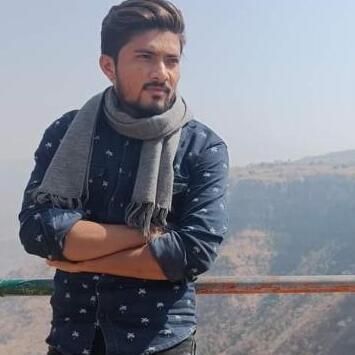
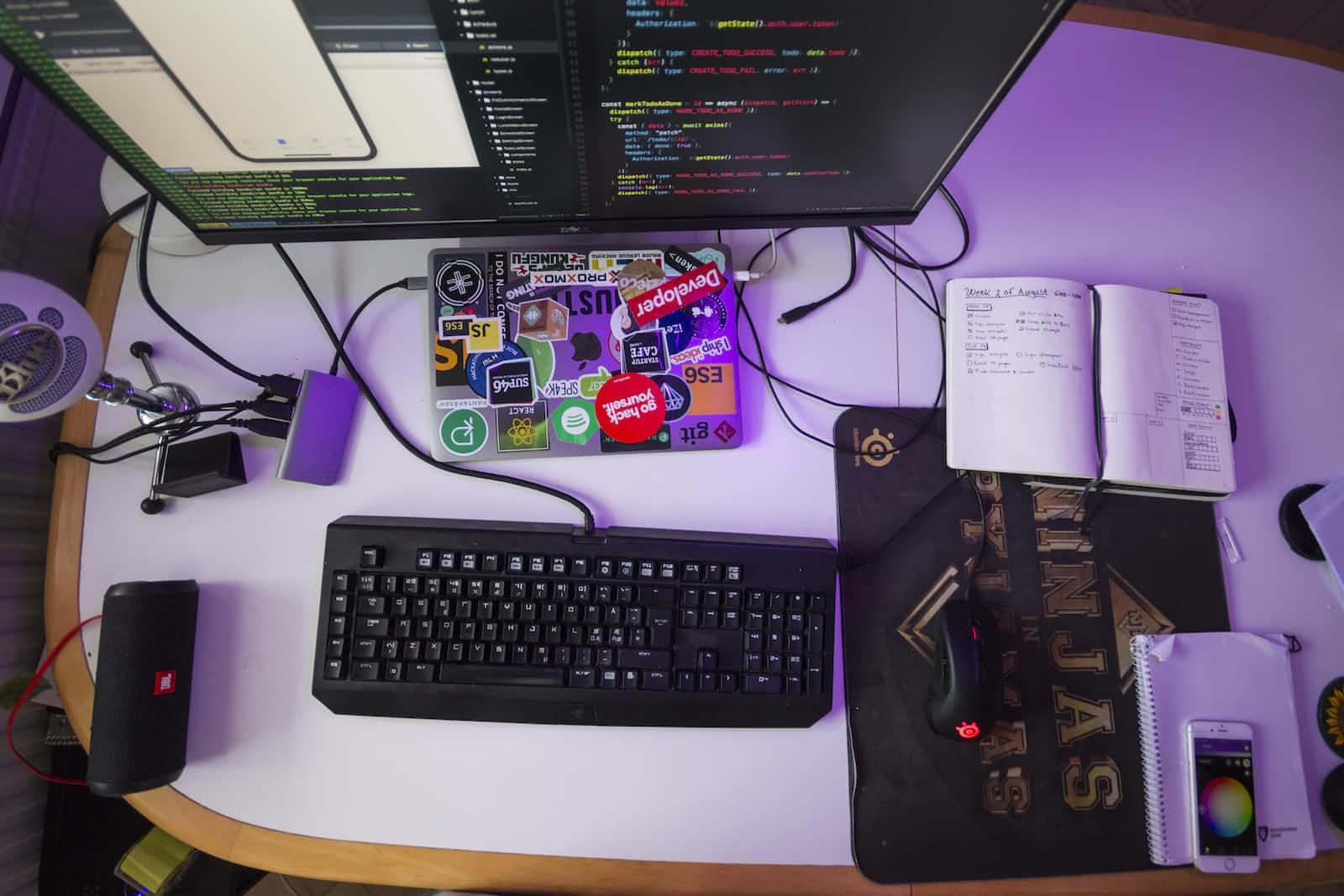
Junior Level:
What is React Native and how does it differ from React?
Explain the concept of JSX in React Native.
What are the core components in React Native?
How do you handle user input in React Native?
What is the purpose of state in React Native?
How do you style components in React Native?
What is the role of the
props
object in React Native?How do you handle navigation between screens in React Native?
Explain the concept of flexbox and how it is used in React Native.
What are the differences between
View
andText
components in React Native?
Mid-level:
Describe the component lifecycle methods in React Native and their purposes.
How can you optimize the performance of a React Native application?
What is Redux, and how is it used in React Native applications?
How do you handle asynchronous operations in React Native?
Explain the purpose of higher-order components (HOCs) in React Native.
What is the purpose of the
StyleSheet
API in React Native?How can you handle device permissions (e.g., camera, location) in React Native?
Describe the concept of animations in React Native and how to implement them.
What is the purpose of the
FlatList
component in React Native?How can you handle data persistence in a React Native application?
Expert Level:
Explain the difference between React Native and native development.
Describe the process of linking native modules in React Native.
How does React Native handle platform-specific code?
What are the limitations of React Native compared to native development?
Describe the bridging mechanism used in React Native for communication between JavaScript and native code.
How can you handle app localization in React Native?
Explain the concept of code push and its use in React Native.
How can you optimize the memory usage of a React Native application?
What are the strategies for testing React Native applications?
Describe the process of integrating third-party libraries in a React Native project.
Coding & Most Asked Questions in React Native
Junior Level:
How do you create a basic React Native component?
Explain the process of styling components in React Native.
How can you handle user input and form submissions in React Native?
Implement a button that changes its text when clicked in React Native.
Create a custom component that accepts a prop and displays it as a text element.
How can you navigate between screens in a React Native app using React Navigation?
Explain how to fetch data from an API in a React Native application.
Build a simple login form with validation using React Native components.
Implement a list view of items fetched from an API in a React Native app.
Create a basic animation in React Native using the Animated API.
Mid-level:
Implement a custom tab bar navigation using React Navigation in a React Native app.
How can you handle device permissions (e.g., camera, location) in React Native?
Build a screen that displays an image gallery with swipe functionality in React Native.
Explain the concept of state management in React Native and provide examples.
How can you handle offline data storage in a React Native application?
Implement a form validation library in React Native from scratch.
Build a reusable component that fetches data and displays a loading indicator while waiting for the response.
Explain how to handle screen orientation changes in a React Native app.
How can you optimize the performance of a React Native app that handles large amounts of data?
Create a custom hook for handling API requests in React Native.
Expert Level:
Explain the process of building a native module for React Native in iOS and Android.
Implement deep linking in a React Native app to handle URLs that open specific screens.
How can you implement internationalization (i18n) in a React Native application?
Build a custom component for handling complex animations and transitions in React Native.
Explain the process of integrating native third-party libraries in a React Native project.
How can you implement push notifications in a React Native app using Firebase Cloud Messaging (FCM)?
Implement gesture recognition and touch events handling in a React Native app.
Explain how to perform unit testing and UI testing in a React Native application.
Build a chat application with real-time messaging using React Native and a backend service like Firebase.
Describe the process of deploying a React Native app to the App Store and Google Play Store.
Subscribe to my newsletter
Read articles from Mayur Patil directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
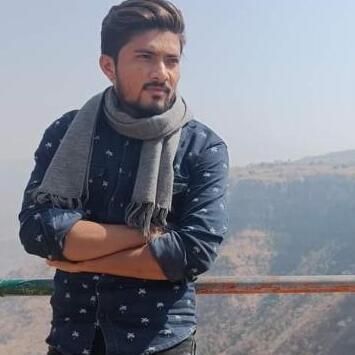
Mayur Patil
Mayur Patil
Frontend Developer || UI Developer || JavaScript || React