Understanding the React Lifecycle Methods
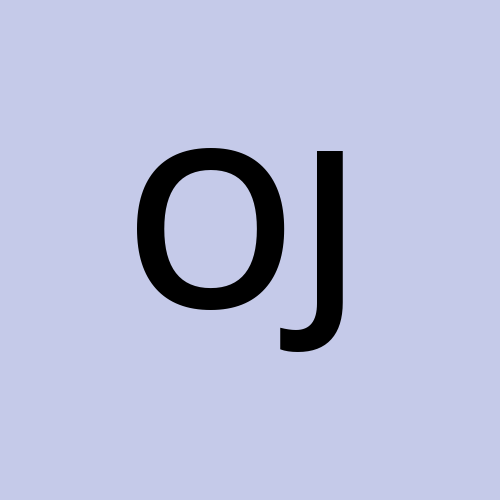
Introduction: React is a popular JavaScript library used for building user interfaces. One of the core features of React is its lifecycle methods, which allow developers to perform certain actions at specific stages during the component's lifecycle. In this technical writing, we will explore the React lifecycle methods and their purposes, providing you with a comprehensive understanding of how they work and how to leverage them effectively in your React applications.
Component Lifecycle Overview: React components go through a series of lifecycle phases from initialization to unmounting. Understanding these phases is crucial for managing component state, handling side effects, and optimizing performance.
Mounting Phase: During the mounting phase, a component is created and inserted into the DOM. The following lifecycle methods are invoked in this order:
constructor(): This method is called when the component is first created. It is used to initialize state and bind event handlers.
static getDerivedStateFromProps(): This method is invoked before rendering and allows you to update the state based on changes in props. It returns an object to update the state or null to indicate no change is necessary.
render(): This method is responsible for rendering the component's UI based on the current state and props. It returns a React element, which describes what should be rendered.
componentDidMount(): This method is called after the component is mounted and rendered to the DOM. It is commonly used to initiate API calls, set up event listeners, or perform other actions that require access to the DOM.
Updating Phase: During the updating phase, a component is re-rendered due to changes in state or props. The following lifecycle methods are invoked in this order:
static getDerivedStateFromProps(): This method is also called during the updating phase, similar to the mounting phase. It allows you to update the state based on new props.
shouldComponentUpdate(): This method is called before rendering when new props or states are received. It determines whether the component should re-render or not, based on the returned boolean value. Implementing this method can optimize performance by preventing unnecessary re-renders.
render(): Same as in the mounting phase, this method is responsible for rendering the component's updated UI.
componentDidUpdate(): This method is invoked after the component's update is applied to the DOM. It is often used for performing side effects like updating the DOM, fetching data based on prop changes, or interacting with external libraries.
Unmounting Phase: The unmounting phase occurs when a component is removed from the DOM. The following lifecycle method is invoked:
the component will unmount (): This method is called just before the component is unmounted and destroyed. It allows for cleanup tasks like removing event listeners, canceling API requests, or releasing resources.
Conclusion: React's lifecycle methods offer developers a powerful way to manage the behavior and state of their components. By understanding the different phases and when each method is invoked, you can effectively control the rendering process, handle data updates, and optimize performance. However, it's worth noting that with the introduction of React Hooks, some of the lifecycle methods have been replaced or modified. React Hooks provide an alternative way to achieve similar functionality while promoting a more functional component structure. As a developer, it's essential to stay updated with the latest React documentation to leverage the most appropriate techniques for your project.

Write an article on react life cycle
ChatGPT
Title: A Comprehensive Guide to React Lifecycle Methods
Introduction: React, a widely-used JavaScript library for building user interfaces, provides developers with a powerful feature called "lifecycle methods." These methods allow you to perform specific actions at different stages of a component's lifecycle. In this article, we will explore the React lifecycle methods in detail, understand their purpose, and learn how to leverage them effectively in your React applications.
Understanding the React Component Lifecycle: React components go through various lifecycle stages, from initialization to unmounting. By understanding these stages, you can control the behavior and state of your components and implement the necessary logic for rendering, data updates, and performance optimizations.
Mounting Phase: The mounting phase occurs when a component is created and inserted into the DOM. The following lifecycle methods are invoked during this phase:
a. constructor(): The constructor method is called when the component is first created. It is used for initializing state and binding event handlers. It's important to call super(props) inside the constructor to ensure that the component's parent class is properly initialized.
b. static getDerivedStateFromProps(): This method is invoked before rendering, both during the initial mounting and subsequent updates. It allows you to update the component's state based on changes in props. However, it's recommended to use this method sparingly and favor controlled components or React Hooks for managing state.
c. render(): The render method is responsible for returning the JSX that represents the component's UI based on its current state and props. It should be a pure function that does not modify the component state or interact with the browser's DOM.
d. componentDidMount(): This method is called after the component has been mounted and rendered to the DOM. It is commonly used for performing side effects, such as initiating API calls, setting up event listeners, or interacting with external libraries.
Updating Phase: The updating phase occurs when a component is re-rendered due to changes in its state or props. The following lifecycle methods are invoked during this phase:
a. static getDerivedStateFromProps(): This method is also called during the updating phase, similar to the mounting phase. It allows you to update the component's state based on new props. However, as mentioned earlier, it's recommended to use other state management solutions like React Hooks or Redux for more predictable state updates.
b. shouldComponentUpdate(): This method is called before rendering when new props or states are received. It provides an opportunity to optimize performance by determining whether the component should re-render or not. By default, React re-renders the component whenever it's props or state change, but you can implement this method to prevent unnecessary re-renders.
c. render(): Same as in the mounting phase, the render method is responsible for returning the updated JSX based on the component's new state and props.
d. componentDidUpdate(): This method is invoked after the component's update is applied to the DOM. It's commonly used for performing side effects, such as updating the DOM directly or fetching data based on prop changes. However, be cautious not to cause infinite update loops by updating the component's state inside this method without proper conditions.
Unmounting Phase: The unmounting phase occurs when a component is removed from the DOM. The following lifecycle method is invoked:
a. component will unmount (): This method is called just before the component is unmounted and destroyed. It allows for cleanup tasks, such as removing event listeners, canceling API requests, or releasing resources. It's crucial to perform necessary cleanup operations in this method to prevent memory leaks and avoid unwanted behavior.
Conclusion: React lifecycle methods provide developers with fine-grained control over component behavior and state at different stages of their lifecycle. By understanding these methods, you can effectively manage data updates, optimize performance, and handle necessary cleanup tasks. However, with the introduction of React Hooks, many lifecycle methods have become less relevant in modern React applications. React Hooks offer a more concise and flexible approach to managing component state and side effects. Therefore, it's recommended to explore and leverage React Hooks as a preferred alternative to lifecycle methods in your React projects.
Subscribe to my newsletter
Read articles from Oluwafemi Josephine directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
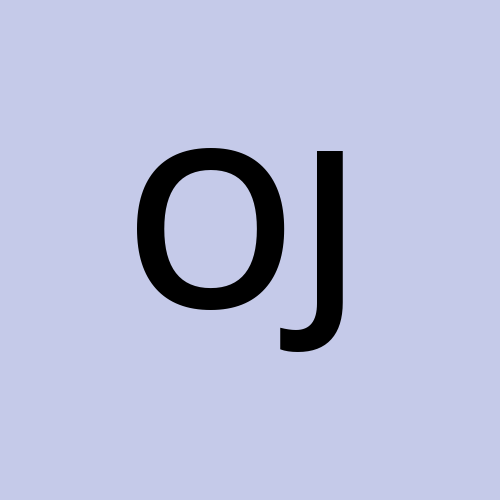
Oluwafemi Josephine
Oluwafemi Josephine
Hi, hi! I'm Josephine Oluwafemi, a passionate and dedicated MERN Stack Software Engineer with a strong background in full-stack web development. I specialize in leveraging the MERN (MongoDB, Express.js, React.js, Node.js) stack to create robust, scalable, and user-centric applications. My expertise lies in crafting efficient and intuitive user interfaces using React.js and Redux, building RESTful APIs with Node.js and Express.js, and designing scalable databases using MongoDB. Throughout my career, I have been involved in the entire software development lifecycle, from project conceptualization and architecture design to implementation, testing, and deployment. I thrive in dynamic and collaborative environments, working closely with cross-functional teams to deliver high-quality solutions that meet client requirements and exceed expectations. My skills extend beyond the MERN stack to encompass a wide range of front-end and back-end technologies, including HTML5, CSS3, JavaScript ES6+, Git, and SQL. I am constantly exploring new technologies and frameworks to stay up-to-date with the ever-evolving landscape of web development, ensuring that I can leverage the best tools to solve complex problems efficiently. If you're looking for a skilled MERN Stack Software Engineer who is passionate about building cutting-edge web applications and contributing to the success of your team, I would love to connect. Let's collaborate on creating exceptional digital experiences that drive user engagement and business growth. Feel free to reach out to me on LinkedIn or via email at Oluwafemijosephine4@gmail.com. I look forward to connecting with you and discussing how I can contribute to your organization's success. Key Skills: MERN Stack Development React.js, Redux Node.js, Express.js MongoDB, SQL HTML5, CSS3, JavaScript ES6+ Git, UI/UX Design Agile Methodologies Problem-solving and Analytical Thinking Excellent Communication and Collaboration Education: BSC, Lagos State University, 2023. Proficient in English Language.