Introduction of C# (part 1/2)
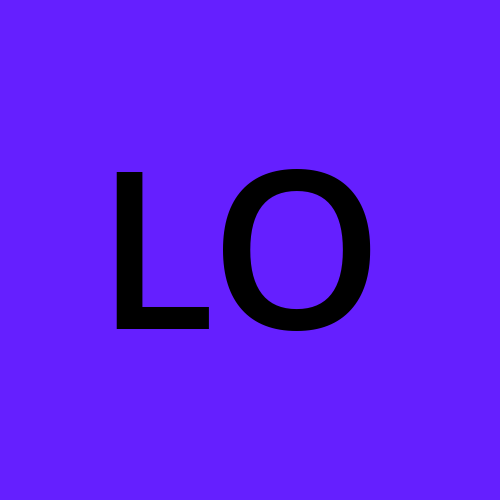
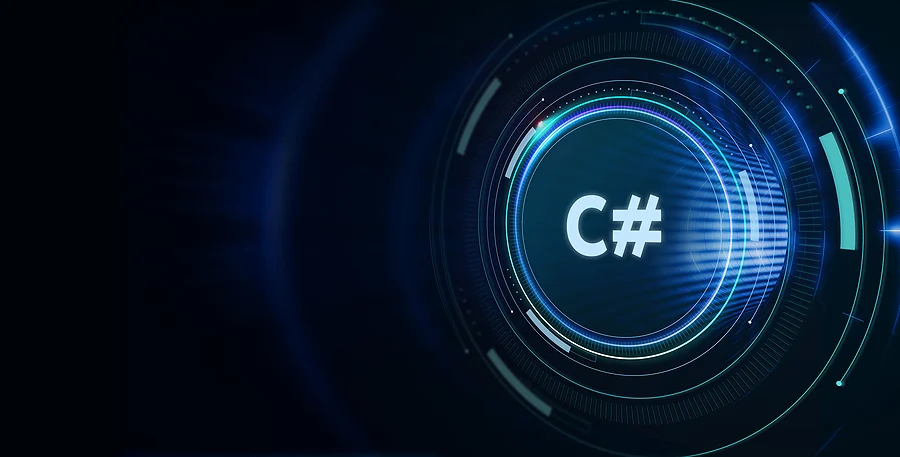
Table of Contents
What is C#?
C# is used for
IDE (Integrated Development)
Syntax
Variables
- Data Types
User Input
Operators
Arithmetic Operators
Assignment Operators
Comparison Operators
Logical Operators
What is C#?
C# is pronounced, "C-Sharp". It is an object-oriented programming language created by Microsoft that runs on the .NET Framework. C# has roots in the C family and is close to other popular languages like C++ and Java. The first version was released in the year 2002. The latest version, C# 11, was released in November 2022.
C# is used for:
Mobile applications
Desktop applications
Web applications
Web services
Websites
Games
VR
Database applications
And much, much more!
Why use C#?
It is one of the most popular programming languages in the world.
It is easy to learn and simple to use.
It has huge community support.
C# is an object-oriented language that gives a clear structure to programs and allows code to be reused, lowering development costs.
As C# is close to C, C++, and Java, it makes it easy for programmers to switch to C# or vice versa.
IDE (Integrated Development Environment)
Using an IDE is the easiest way to get started with C#. An IDE (Integrated Development Environment) is used to edit and compile code. You can use Visual Studio Community, which is free to download from https://visualstudio.microsoft.com/vs/community/. Applications written in C# use the .NET Framework, so it makes sense to use Visual Studio, as Microsoft created the program, framework, and language.
Syntax
Line 1: using System means that we can use classes from the System.namespace.
Line 2: A blank line. C# innores white space. However, multiple lines make the code readable.
Line 3: namespace is used to organize your code, and it is a container for classes and other namespaces.
Line 4: The curly braces {} mark the beginning and the end of a block of code.
Line 5: class is a container for data and methods, which brings functionality to your program. Every line of code that runs in C# must be inside a class. In the example above, it is named class Program.
Line 7: Another thing that always appears in a C# program, is the Main method. Any code inside its curly brackets {} will be executed. You don't have to understand the keywords before and after Main. You will get to know them bit by bit while reading this blog.
Line 9: Console is a class of the System namespace, which has a WriteLine() method that is used to output/print text. In the example above, it will output "Hello World!".
If you omit the using System line, you would have to write System.Console.WriteLine() to print/output text.
Note: Every C# statement ends with a semicolon ;
Note: C# is case-sensitive meaning "MyClass" and "myclass" has a different meaning.
Variables
Variables are containers for storing data values. In C#, there are different types of variables (defined with different keywords) for example:
int - stores integers (whole numbers), without decimals such as 123 or -123.
double - stores floating point numbers with decimals such as 19.99 or -19.99.
char - stores single characters such as 'a' or 'B'. Char values are surrounded by single quotes.
string - stores text such as "Hello World". String values are surrounded by double quotes.
bool - stores values with two states: true or false.
Example
Data Types
User Input
You have already learned that Console.WriteLine() is used to output (print) values. Now we will use Console.ReadLine() to get user input. In the following example, the user can input his or her username, which is stored in the variable userName. Then we print the value of userName.
The Console.ReadLine() method returns a string. Therefore, you cannot get information from another data type, such as int. The following program will cause an error:
Convert string into an int:
Operators
Operators are used to performing operations on variables and values. In the example below, we use the + (plus) operator to add together two values:
Although the + operator is often used to add together two values, like in the example above, it can also be used to add together a variable and a value, or a variable and another variable:
Arithmetic Operators
Arithmetic operators are used to perform common mathematical operations:
Assignment Operators
Assignment operators are used to assign values to variables. In the example below, we use the assignment operator (=) to assign the value 10 to a variable called x:
The addition assignment operator (+=) adds a value to a variable:
List of all assignment operators:
Comparison Operators
Comparison operators are used to compare two values (or variables). This is important in programming because it helps us to find and make decisions.
The return value of a comparison is either True or False. These values are known as Boolean values, and you will learn more about them in the Booleans and If/Else chapter.
In the following example, we use the greater than operator (>) to find out if 5 is greater than 3:
List of all comparison operators:
Logical Operators
As with the comparison operator, you can also test for True or False values with logical operators. Logical operators are used to determine the logic between variables or values:
Subscribe to my newsletter
Read articles from Leoneil Odrunia directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
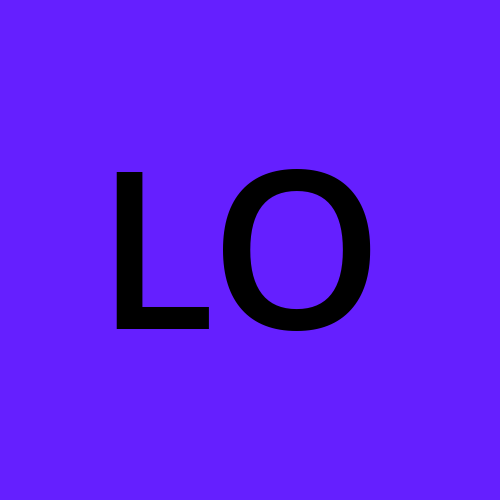