Event Bubbling in JS(javascript)
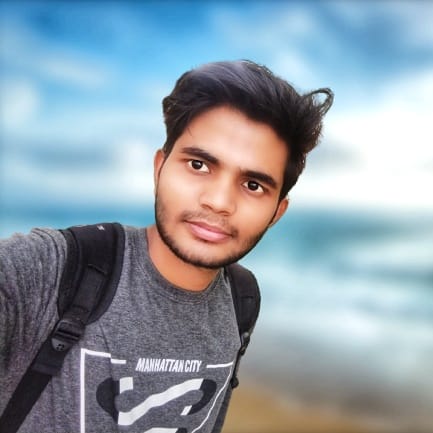
Event bubbling in JavaScript is a concept that explains how events propagate through the hierarchy of the DOM (Document Object Model). In simple terms, when an event like a click or a keypress happens on an element, it doesn't only affect that particular element. It also triggers the same event on its parent elements, continuing all the way up to the root of the document. This mechanism allows you to handle events at different levels of the DOM hierarchy, making event handling more efficient and flexible.
The process of event propagation occurs in two phases:
Event Capturing (Trickling): In this phase, the event starts at the root of the document and travels down the DOM tree through each parent element until it reaches the target element. However, this phase is rarely used and often ignored, as most developers work with event bubbling.
Event Bubbling: After the event reaches the target element, it starts to "bubble" up the DOM hierarchy, triggering the same event on each parent element, one level at a time, until it reaches the document's root.
Here's an example to illustrate event bubbling:
<!DOCTYPE html>
<html>
<head>
<title>Event Bubbling Example</title>
</head>
<body>
<div id="outer">
<div id="inner">
<button id="button">Click me!</button>
</div>
</div>
<script>
const buttonElement = document.getElementById('button');
buttonElement.addEventListener('click', function(event) {
console.log('Button clicked!');
console.log('Event Target:', event.target);
console.log('Current Target:', event.currentTarget);
});
const innerElement = document.getElementById('inner');
innerElement.addEventListener('click', function(event) {
console.log('Inner div clicked!');
});
const outerElement = document.getElementById('outer');
outerElement.addEventListener('click', function(event) {
console.log('Outer div clicked!');
});
</script>
</body>
</html>
Event bubbling is a concept in JavaScript that describes the way events propagate through the DOM (Document Object Model) hierarchy. When an event occurs on an element, such as a click or a keypress, the event doesn't just trigger on that specific element. Instead, it also triggers on its parent elements, all the way up to the root of the document.
The process of event propagation occurs in two phases:
Event Capturing (Trickling): In this phase, the event starts at the root of the document and travels down the DOM tree through each parent element until it reaches the target element. However, this phase is rarely used and often ignored, as most developers work with event bubbling.
Event Bubbling: After the event reaches the target element, it starts to "bubble" up the DOM hierarchy, triggering the same event on each parent element, one level at a time, until it reaches the document's root.
Here's an example to illustrate event bubbling:
<!DOCTYPE html>
<html>
<head>
<title>Event Bubbling Example</title>
</head>
<body>
<div id="outer">
<div id="inner">
<button id="button">Click me!</button>
</div>
</div>
<script>
const buttonElement = document.getElementById('button');
buttonElement.addEventListener('click', function(event) {
console.log('Button clicked!');
console.log('Event Target:', event.target);
console.log('Current Target:', event.currentTarget);
});
const innerElement = document.getElementById('inner');
innerElement.addEventListener('click', function(event) {
console.log('Inner div clicked!');
});
const outerElement = document.getElementById('outer');
outerElement.addEventListener('click', function(event) {
console.log('Outer div clicked!');
});
</script>
</body>
</html>
In this example, when you click the "Click me!" button, you will notice that all three event listeners fire in the following order:
Button clicked!
Event Target: [button element]
Current Target: [button element]
Inner div clicked!
Outer div clicked!
As you can see, the event starts from the button element and bubbles up through the inner div and the outer div, triggering the corresponding event handlers for each element.
Event bubbling is a powerful mechanism that simplifies event handling, as you can attach a single event listener to a parent element rather than attaching multiple listeners to each child element. However, in certain cases, you might need to stop event propagation to prevent undesired side effects. This can be achieved using the event.stopPropagation()
method inside your event handler.
Thank you for reading this blog, follow me on Twitter, I regularly share blogs and post on Javascript, React, Web development and opensource contribution
Twitter- https://twitter.com/Diwakar_766
Subscribe to my newsletter
Read articles from Diwakar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
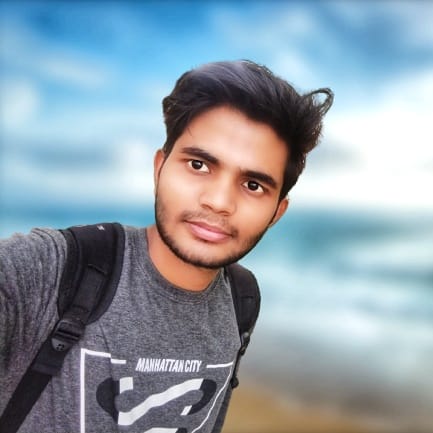
Diwakar
Diwakar
As a passionate developer, I thrive on acquiring new knowledge. My journey began with web development, and I am now actively engaged in open source contributions, aiding individuals and businesses.