Sort Colors

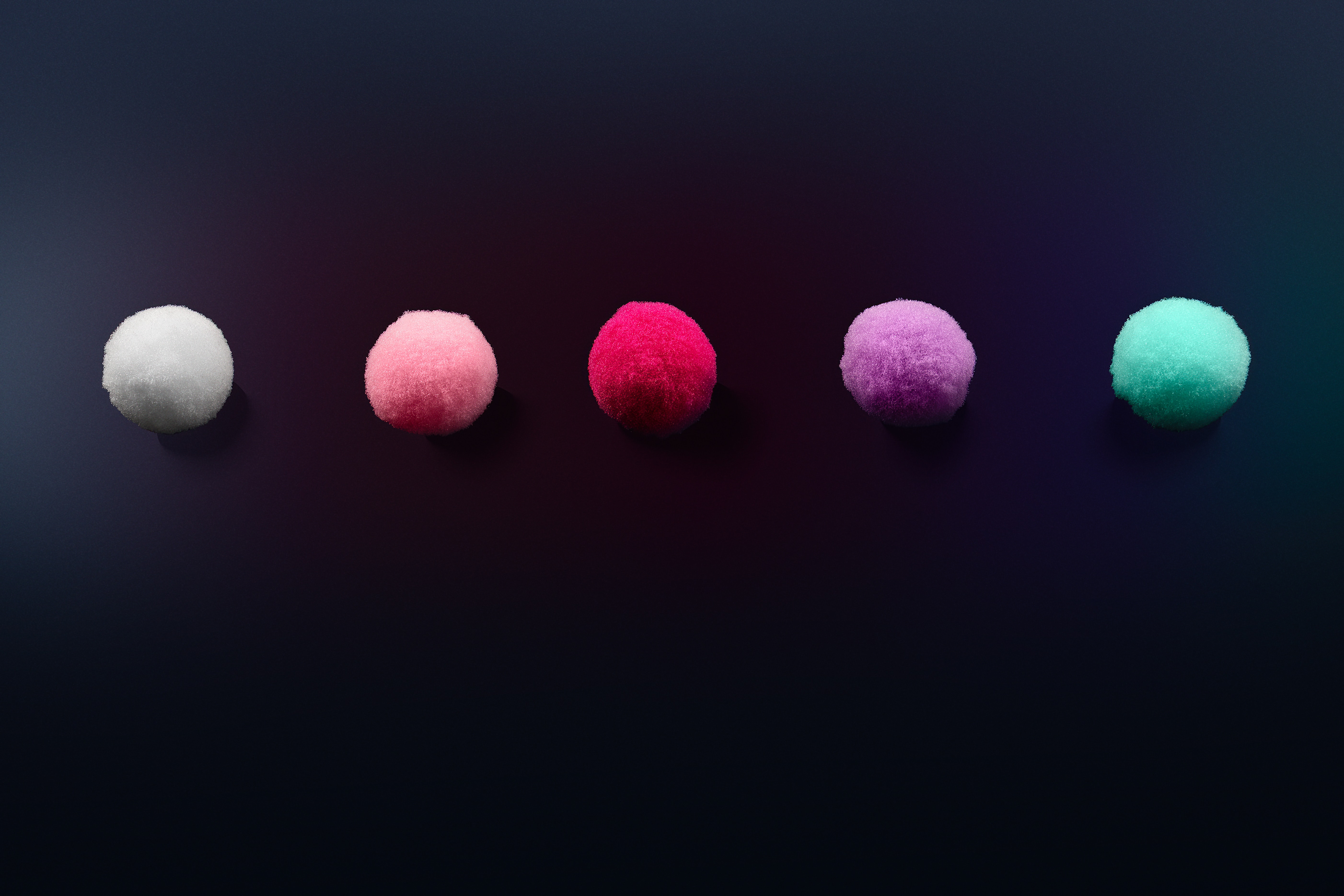
Problem statement
Sort the array containing red, white, and blue colors (0, 1, 2) in-place without using the library's sort function. Use the Dutch National Flag algorithm.
Constraints
n == nums.length
1 <= n <= 300
nums[i]
is either0
,1
, or2
Examples
nums = [1, 0, 2, 1, 2, 1, 0, 2]
output = [0, 0, 1, 1, 1, 2, 2, 2]
Sudo Code
Set three pointers: low, mid, and high to the start, start, and end of the array, respectively
Loop while mid is less than or equal to high.
If the element at mid is 0, swap with low, increment both low and mid
If the element at mid is 2, swap with high, decrement high.
If the element at mid is 1, increment mid
Dry Run
Solution
public void sortColors(int[] nums) {
int n = nums.length;
// Step 1. Set three pointers: low, mid, and high to the start, start, and end of the array, respectively.
int low = 0;
int mid = 0;
int high = n-1;
// Step 2. Loop while mid is less than or equal to high.
while(mid <= high){
if(nums[mid] == 0){
// Step 3. If the element at mid is 0, swap with low, increment both low and mid
int temp = nums[mid];
nums[mid] = nums[low];
nums[low] = temp;
mid++;
low++;
} else if(nums[mid] == 2){
// Step 4. If the element at mid is 2, swap with high, decrement high.
int temp = nums[mid];
nums[mid] = nums[high];
nums[high] = temp;
high--;
}else{
// Step 5. If the element at mid is 1, increment mid.
mid++;
}
}
}
Time complexity Analysis
Time complexity - O(N)
Space Complexity - O(1)
Topics Covered
Two pointers
Companies
Amazon, Google, Microsoft, Apple, Adobe, Linkedln, Uber, Qualcomm
Subscribe to my newsletter
Read articles from Shreyash Chavhan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
