Advanced Linux shell scripting for DevOps engineers

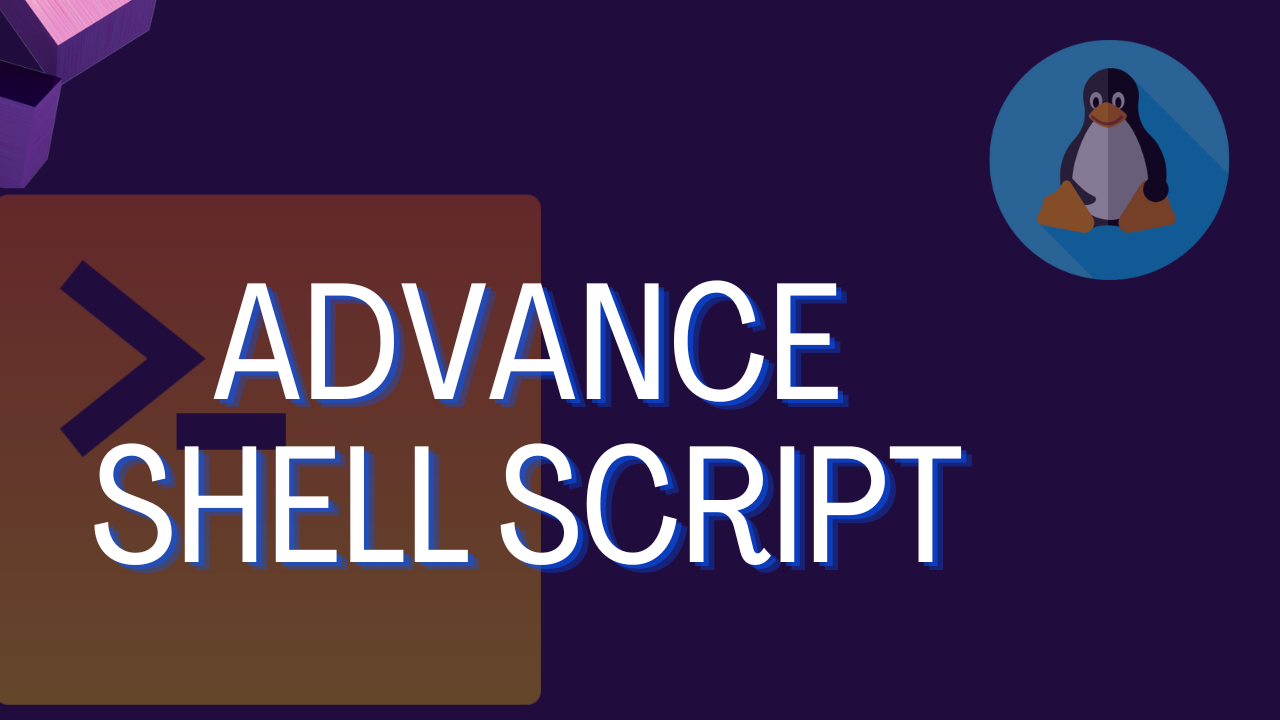
As a DevOps engineer, the ability to write efficient and powerful shell scripts is paramount, as it allows you to automate tasks, manage systems, and streamline processes, all while promoting collaboration between development and operations teams.
Table of Contents:
Understanding the Fundamentals ๐
What is a Shell Script?
Choosing the Right Shell: Bash, Zsh, or Others?
Executing Shell Scripts: Shebang, Permissions, and Running Scripts.
Working with Variables and Data ๐ป
Declaring and Assigning Variables
Special Variables: $0, $#, $*, $@, $?, and $$
Command Substitution and Arithmetic Operations
Conditional Statements and Control Structures ๐
If-Else Statements
Case Statements
Looping with For and While
File and Directory Handling ๐
Reading and Writing Files
Text Processing with Sed and Awk
Managing Directories: Creating, Listing, and Deleting
Advanced Text Processing ๐
Regular Expressions and Pattern Matching
Grepping, Cutting, Sorting, and Unique Filters
Combining Tools: Pipes and Redirections
Working with Functions ๐ ๏ธ
Declaring and Using Functions
Local and Global Variables
Recursion and Function Libraries
Process Management and Job Control ๐
Process IDs (PIDs) and Signals
Background and Foreground Processes
Managing Jobs with bg, fg, and Jobs Command
Handling Errors and Debugging ๐
Exit Status and Return Codes
Trapping Signals and Errors
Debugging Techniques: Echo, set -x, and set -e
Interacting with the System ๐ฅ๏ธ
Environment Variables
System Information: uname, df, free, and top
Working with Date and Time
Shell Scripting Best Practices ๐
Code Readability and Maintainability
Error Handling and Logging
Optimizing Shell Scripts: Performance Tips
Automating DevOps Tasks ๐๐ง
Building Deployment Scripts
Continuous Integration (CI) Pipelines
Configuration Management with Shell Scripts3
Example
**
Shell Script: Using Loops or Commands with Start Day and End Day Variables using Arguments** ๐๐
#!/bin/bash
if [ $# -ne 2 ]; then
echo "Usage: $0 <start_day> <end_day>"
exit 1
fi
for (( day = $1; day <= $2; day++ )); do
echo "Day $day"
done
Create a Script to backup all your work done till now.
#!/bin/bash
#Define the backup directory and filename
backup_dir="/path/to/backup" # Replace with your desired backup directory backup_filename="backup_$(date +%Y%m%d%H%M%S).tar.gz"
#Create the backup directory if it doesn't exist
mkdir -p "$backup_dir"
#Compress and backup the files
tar -czf "$backup_dir/$backup_filename" /path/to/work/done/till/now # Replace with the directory containing your work
echo "Backup created successfully: $backup_dir/$backup_filename"
Cron and Crontab, to automate the backup Script
backup_script.sh
#!/bin/bash
#Define the backup directory and filename
backup_dir="/path/to/backup" # Replace with your desired backup directory backup_filename="backup_$(date +%Y%m%d%H%M%S).tar.gz"
#Create the backup directory if it doesn't exist
mkdir -p "$backup_dir"
#Compress and backup the files
tar -czf "$backup_dir/$backup_filename" /path/to/work/done/till/now # Replace with the directory containing your work
echo "Backup created successfully: $backup_dir/$backup_filename"
Cron
#!/bin/bash
#Absolute path to the backup_script.sh
backup_script="/path/to/backup_script.sh" # Replace with the actual path to backup_script.sh
(crontab -l ; echo "0 2 * $backup_script") | crontab -
echo "Backup job has been scheduled to run daily at 2:00 AM."
Subscribe to my newsletter
Read articles from Saurabh Mathuria directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
