Understanding JavaScript Debugging: Solving Common Challenges with Ease.

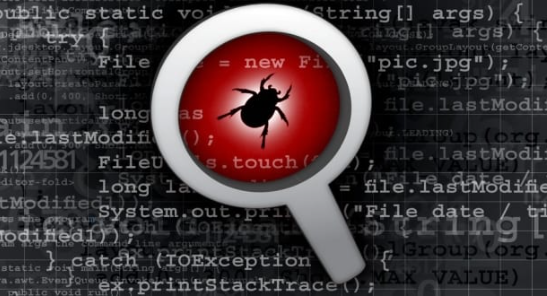
Introduction.
In development, we are often faced with perplexing challenges while debugging our JavaScript code. Sometimes I find myself needing that second pair of eyes or having to take a stroll and get some fresh air. We’ve all been there where we run into issues that seem to throw a wrench into the project development process. In this post, using some code examples that aim to demonstrate techniques that would reduce or prevent common issues from occurring, we will look at tips on demystifying and conquering some of these common JavaScript challenges that have been known to test even the most seasoned developers.
Handling Undefined Variables.
One of the most common stumbling blocks in JavaScript development or any other programming language for that matter is dealing with undefined variables. As much as this annoys anyone considering you’ll have gone as far as understanding your code concept but missed that small variable definition or assignment, the key to overcoming this challenge lies in understanding variable scoping and initialization.
- Scoping; JavaScript uses lexical scoping, which means that variables are scoped to their containing functions or blocks. Variables declared with
‘var’
are globally and/or function-scoped (note that the use of var is no longer recommended, especially after ES6) while variables declared with‘let’
and‘const’
are block-scoped. It is therefore important to understand the scope in which a variable is declared to avoid unexpected behavior or conflict,
// Example of Variable Scoping
function example() {
if (true) {
var x = 10; // Function-scoped
let y = 20; // Block-scoped
const z = 30; // Block-scoped
}
console.log(x); // Output: 10
console.log(y); // ReferenceError: y is not defined
console.log(z); // ReferenceError: z is not defined
}
example();
- Initialization; Always ensure that all variables are properly initialized before accessing them so as to avoid encountering undefined values. Consider using default values or implementing conditional checks to handle cases where variables may be undefined as shown in the below example..,
// Example of Handling undefined variables
let name;
if (name === undefined) {
name = 'John Doe';
}
console.log(name); // Output: John Doe
…or you can use the ‘typeof’
operator, this approach will return a string that represents the data type of the variable in which case, ‘undefined’
will show you that the variable in question hasn’t been created or doesn’t exist but if it does, it hasn’t been assigned a value.
if (typeof name === 'undefined') {
console.log(`The variable ${name} is undefined`);
}
Tackling Asynchronous Challenges.
JavaScript's asynchronous nature is a blessing for instance when it comes to handling DOM on user events, but at the same time, it can lead to tricky debugging scenarios, especially when dealing with promises, callbacks, or async/await functions. Here are a few tips to help get around the async challenge:
- Debugging Promises; When working with promises, use
console.log
or breakpoints strategically to trace the flow of promise chains. To avoid unintentional swallowing or error hiding, ensure that you handle errors and usecatch
to capture any unhandled rejections.
// Example of Debugging Promises
fetch('https://api.example.com/data') // Make a GET request to the specified URL
.then(response => {
console.log(response);
return response.json();
})
.then(data => {
console.log(data); // Log the parsed JSON data to the console
})
.catch(error => {
console.error('Error:', error); // Log any errors that occur during the promise chain
});
- Working with Callbacks; Break down callback functions into smaller, testable units. Utilize error-first callbacks, where the first parameter of the callback is reserved for an error object, to handle potential errors. Use the power of debugging tools (and gain the ability to closely examine the code's execution flow, track down issues, and gain insights into variables and data at runtime) to step through callback functions and identify any issues.
// Example of Debugging Callbacks
function fetchData(callback) {
if (typeof callback === 'function') {
// Simulating asynchronous operation
setTimeout(() => {
const data = { id: 1, name: 'John Doe' };
callback(null, data);
}, 2000);
}
}
fetchData((error, data) => {
if (error) {
console.error('Error:', error);
} else {
console.log(data);
}
});
Taming the DOM.
Working with the Document Object Model (DOM) can sometimes be challenging when it comes to debugging. To make this task more manageable, here are some best practices to keep in mind,
- Proper Element Selection: When interacting with the DOM, it's important to select the correct elements that you want to work with. Verifying and making the correct selection can prevent unnecessary debugging headaches caused by targeting the wrong elements. Use selectors such as
getElementById
,querySelector
, orquerySelectorAll
to target specific elements. Let’s understand how these selectors work in order to use them appropriately.
// Example of DOM Element Selection
const button = document.getElementById('myButton');
console.log(button);
const elements = document.querySelectorAll('.item');
console.log(elements);
getElementById
: This method allows you to select an element by its unique id attribute. Make sure that the id you provide matches the actual id attribute of the desired element.
// Example - getElementById
const myElement = document.getElementById('myElement');
querySelector
: This method lets you select elements using CSS selectors. You can use various CSS selectors, for instance, tag name, class, or attribute selectors, to target specific elements. Remember to use the correct syntax for the selector you are using.
// Example of querySelector
const myElement = document.querySelector('.myClass');
querySelectorAll
: is similar toquerySelector
, however, this method selects multiple elements that match a specific CSS selector. It returns a collection of elements, allowing you to iterate over them if necessary.
// Example - querySelectorAll
const myElements = document.querySelectorAll('.myClass');
myElements.forEach(element => {
// Do something with each selected element
});
- Event Listener Woes: Ensure event listeners are attached to the correct elements and that the associated functions are properly defined and invoked. Use descriptive function names to make your code more readable and easier to debug.
// Example of Event Listener
const button = document.getElementById('myButton');
button.addEventListener('click', handleClick);
function handleClick(event) {
event.preventDefault();
console.log('Button clicked!');
}
- Handling Asynchronous DOM Updates: When manipulating the DOM asynchronously, utilize callbacks or promises to ensure the DOM updates are complete before further operations are performed. This will help avoid issues such as accessing elements before they are rendered or updated.
Conclusion.
This is by no means a detailed guide to debugging, but I hope this overview of tips and strategies will help navigate through some of the most common JavaScript challenges that have plagued us all at some point. Remember to approach debugging with patience, utilize debugging tools like browser developer consoles and breakpoints, and always test assumptions along the way.
Feel free to share your own debugging stories and additional tips in the comments section and remember to subscribe to my FREE newsletter and get notified on future content. Until next time!
Subscribe to my newsletter
Read articles from Mandla directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Mandla
Mandla
Developer and Tech Enthusiast with a Drive to Transform Industries through Innovation.