Embracing Perfection: A Journey into Rust Programming
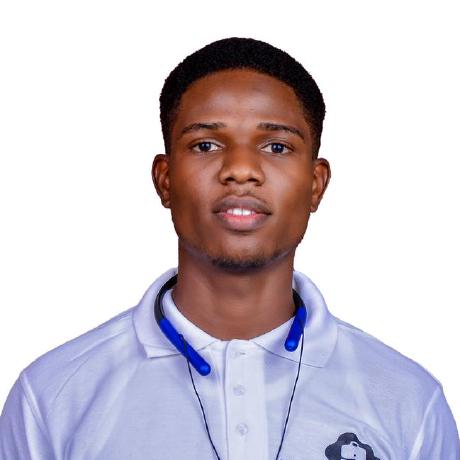
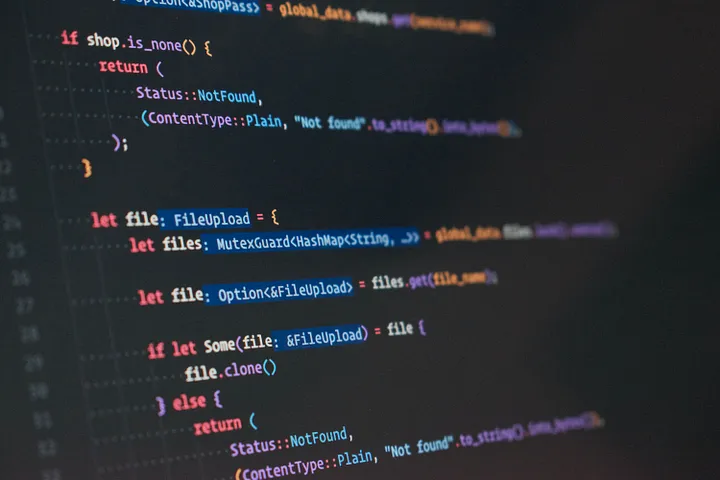
Embarking on the journey of learning Rust is like stepping into a world that demands perfection. While it may not be the easiest language to master, Rust offers a unique set of features and concepts that push developers to consider every aspect of their code. In this article, we will explore some of the core features and concepts that make Rust a powerful and compelling language for developers. Whether you’re a beginner or an experienced programmer, these insights will help you understand and appreciate the value of Rust.
1. Embracing Functional and Object-Oriented Concepts in Rust
Rust is a versatile language that combines elements of functional programming and object-oriented programming (OOP) paradigms. While it is not a strictly functional or purely OOP language, Rust provides powerful features and concepts that allow developers to embrace functional and OOP principles in their code. Let’s explore how Rust handles these concepts and empowers developers to write expressive and modular code.
Functional Programming in Rust
Functional programming emphasizes immutability, pure functions, and higher-order functions. Although Rust is not a pure functional language, it offers several features that align with functional programming principles.
Immutability and Functional Concepts:
Rust encourages immutability by default. Variables are immutable by default, and you need to explicitly mark them as mutable when necessary. This approach aligns with functional programming’s emphasis on immutability to avoid unintended side effects and improve code clarity.
Example: Immutability in Rust
fn main() {
let x = 5; // Immutable binding
let y = {
let x = 2; // Shadowing with new binding
x + 1
};
println!("x: {}", x); // Prints 5
println!("y: {}", y); // Prints 3
}
In this example, the variable x
is initially bound to the value 5. Inside the block, we create a new binding, also named x
, which shadows the outer binding. This demonstrates Rust’s support for functional-style immutability through shadowing.
Higher-Order Functions:
Rust supports higher-order functions, allowing you to pass functions as arguments or return them from other functions. This enables powerful abstractions and functional composition.
Example: Higher-Order Functions in Rust
fn apply_twice<F>(f: F, x: i32) -> i32
where
F: Fn(i32) -> i32,
{
f(f(x))
}
fn add_one(x: i32) -> i32 {
x + 1
}
fn main() {
let result = apply_twice(add_one, 2);
println!("Result: {}", result); // Prints 4
}
In this example, the apply_twice
function takes a closure f
and applies it twice to the argument x
. The closure F
is a higher-order function that takes an i32
argument and returns an i32
. We pass the add_one
function as an argument to apply_twice
, demonstrating the ability to pass functions as arguments and apply them within Rust.
Object-Oriented Programming in Rust
While Rust does not have traditional class-based OOP like languages such as Java or C++, it provides features that allow developers to achieve object-oriented designs and encapsulation.
Structs and Methods:
Rust uses structs to define custom data types and associated methods. Methods are functions defined within the context of a struct, enabling data encapsulation and object-like behavior.
Example: Structs and Methods in Rust
struct Rectangle {
width: u32,
height: u32,
}
impl Rectangle {
fn area(&self) -> u32 {
self.width * self.height
}
}
fn main() {
let rect = Rectangle {
width: 10,
height: 5,
};
println!("Area: {}", rect.area()); // Prints 50
}
In this example, we define a Rectangle
struct with width
and height
fields. We implement an associated method area
for the struct, which calculates and returns the area of the rectangle. By using the impl
block,
we define the implementation of the methods associated with the Rectangle
struct.
Traits and Polymorphism:
Rust’s trait system provides a way to achieve polymorphism and code reuse, similar to interfaces in OOP languages. Traits define behavior that types can implement, allowing for dynamic dispatch and runtime polymorphism.
Example: Traits and Polymorphism in Rust
trait Animal {
fn sound(&self);
}
struct Dog;
struct Cat;
impl Animal for Dog {
fn sound(&self) {
println!("Woof!");
}
}
impl Animal for Cat {
fn sound(&self) {
println!("Meow!");
}
}
fn main() {
let dog: Dog = Dog;
let cat: Cat = Cat;
let animals: Vec<Box<dyn Animal>> = vec![Box::new(dog), Box::new(cat)];
for animal in animals {
animal.sound();
}
}
In this example, we define the Animal
trait with a method sound
. We implement the Animal
trait for the Dog
and Cat
structs, each providing their own implementation of the sound
method. We create a vector of boxed dyn Animal
trait objects and iterate over them, calling the sound
method dynamically at runtime.
2. Ownership and Borrowing:
Rust’s ownership and borrowing system can be likened to a set of buckets representing different scopes. Each bucket represents a local scope, and the entities within the bucket are dropped or deallocated when the bucket or local scope goes out of scope. Understanding this analogy can help grasp the concepts of ownership and borrowing in Rust.
Imagine we have a bucket representing the main function scope. Inside this bucket, we can place entities, which can be either moved or borrowed when a new bucket (such as a function) is created. Let’s explore these concepts further:
Example 1: Ownership (Moving)
fn main() {
let string = String::from("Hello");
let new_string = take_ownership(string);
// The string is moved to the `take_ownership` function and no longer accessible here.
println!("New string: {}", new_string);
}
fn take_ownership(s: String) -> String {
// The ownership of the `s` string is transferred to this function.
// We can perform operations on `s` without affecting the original string.
s + " World!"
}
In this example, the take_ownership
function takes ownership of the string
and returns a new string. The original string is moved to the function, and it cannot be accessed in the main function afterward.
Example 2: Borrowing (Referencing)
fn main() {
let string = String::from("Hello");
let length = calculate_length(&string);
// The `string` is still accessible in the main function after borrowing.
println!("Length of {}: {}", string, length);
}
fn calculate_length(s: &String) -> usize {
// The `s` parameter is a reference to the original string.
// We can perform operations on the borrowed string without taking ownership.
s.len()
}
In this example, the calculate_length
function borrows the string
by accepting a reference (`&string`). The reference allows the function to access the string’s data without taking ownership. Therefore, the original string remains accessible in the main function even after borrowing.
By distinguishing between ownership (moving) and borrowing (referencing), Rust ensures memory safety and eliminates common bugs caused by multiple owners or invalid access to data. This system encourages developers to think carefully about how data is passed between scopes and promotes efficient and safe code.
3. Pattern Matching:
Rust’s pattern matching is a versatile tool that allows developers to handle different scenarios and deconstruct complex data structures. It provides concise and expressive syntax, enabling effective case handling and value extraction. Let’s delve into the concept of pattern matching in Rust:
Example 1: Matching Enums
enum Message {
Quit,
Move { x: i32, y: i32 },
Write(String),
}
fn process_message(msg: Message) {
match msg {
Message::Quit => {
println!("Quit message received");
}
Message::Move { x, y } => {
println!("Move to ({}, {})", x, y);
}
Message::Write(text) => {
println!("Write message: {}", text);
}
}
}
In this example, we define an enum called Message
with different variants. The match
keyword allows us to pattern match against each variant and execute the corresponding code block. Pattern matching simplifies the handling of different types of messages and ensures comprehensive coverage.
Example 2: Destructuring Tuples
fn print_coordinates(&(x, y): &(i32, i32)) {
println!("Coordinates: ({}, {})", x, y);
}
fn main() {
let coordinates = (10, 20);
print_coordinates(&coordinates);
}
Here, we have a function called print_coordinates
that accepts a reference to a tuple (i32, i32)
as a parameter. By using pattern matching, we can easily destructure the tuple into its individual components (x, y)
. This enables us to access and utilize the coordinates within the function.
Pattern matching allows developers to handle complex scenarios and extract values from data structures in a concise and expressive manner. By embracing pattern matching, Rust programmers can write clean and structured code that effectively handles diverse cases.
4. Lifetimes in Rust: Preventing Dangling References and Fixing Errors
Rust’s lifetime system ensures that references are always valid and prevents dangling references, which are references to memory that no longer exists. Lifetimes track the duration in which a reference is valid and enforce strict rules to guarantee memory safety. Understanding lifetimes, avoiding dangling references, and fixing related errors is crucial for writing reliable and efficient code in Rust.
Example 1: Dangling Reference
fn get_string() -> &String {
let string = String::from("Hello");
&string
}
fn main() {
let dangling_ref = get_string();
println!("Dangling reference: {}", dangling_ref);
// Error: The `string` in `get_string` is dropped, leaving `dangling_ref` pointing to invalid memory.
}
In this example, the get_string
function creates a String
and returns a reference to it. However, since the reference’s lifetime is tied to the local scope of the get_string
function, it becomes a dangling reference once the function ends. When the dangling_ref
is used in the main
function, it points to invalid memory, leading to undefined behavior and a potential crash. Rust’s lifetime system prevents this scenario by disallowing dangling references at compile time.
Example 2: Proper Lifetime Annotation
fn get_longest<'a>(x: &'a str, y: &'a str) -> &'a str {
if x.len() > y.len() {
x
} else {
y
}
}
fn main() {
let string1 = String::from("Hello");
let result;
{
let string2 = String::from("World");
result = get_longest(&string1, &string2);
println!("Longest string: {}", result);
}
// Both `string1` and `string2` are still valid until this point, so the reference `result` is not dangling.
}
In this updated example, the get_longest
function has a lifetime annotation ’a
that indicates the references x
and y
have the same lifetime. The main function creates two String
instances, string1
and string2
, and passes their references to get_longest
. Since the lifetime of the references is properly managed, the returned reference result
remains valid and does not become a dangling reference.
Example 3: Fixing the Dangling Reference Error
fn get_string() -> String {
let string = String::from("Hello");
string
}
fn main() {
let valid_string = get_string();
let valid_ref = &valid_string;
println!("Valid reference: {}", valid_ref);
// The `valid_string` is still valid, and the reference `valid_ref` can be safely used.
}
In this example, we modify the get_string
function to return the actual String
instead of a reference to it. By doing so, the ownership of the String
is transferred to the caller, ensuring that the string remains valid outside the function scope. In the main
function, we assign the returned String
to the valid_string
variable and create a valid reference, valid_ref
, to it. This approach avoids the issue of dangling references altogether, as the data remains accessible and valid throughout its lifetime.
By returning the actual owned value rather than a reference in situations where it makes sense, developers can avoid dangling references and leverage Rust’s ownership model to ensure memory safety. It’s important to consider
5. Single Mutable Reference: Ensuring Mutability and Memory Safety
In Rust, the concept of ownership extends to mutable references, ensuring that there is only a single mutable reference to a variable within a particular scope. This design choice enforces strict rules to prevent data races and guarantee memory safety, making Rust code highly reliable. Understanding why Rust allows only one mutable reference and how it enhances the safety and predictability of your programs is essential.
The restriction of having a single mutable reference to a variable prevents potential issues such as data races, where multiple threads concurrently access and modify the same data, leading to unpredictable and erroneous behavior. By allowing only one mutable reference, Rust avoids scenarios where multiple references could simultaneously modify a value and cause race conditions.
Example 1: Single Mutable Reference in Action
fn main() {
let mut number = 5;
let reference = &mut number; // Mutable reference
*reference += 1; // Modifying the value through the reference
println!("Modified number: {}", number); // Output: Modified number: 6
}
In this example, we define a mutable variable number
and then create a mutable reference reference
to it using the &mut
syntax. By dereferencing the reference with *reference
, we can modify the value of number
. In this case, we increment number
by 1. As a result, the value of number
is changed to 6, and we can observe the modified value when we print it.
By allowing only one mutable reference to a variable, Rust ensures that modifications are well-controlled, preventing potential conflicts and race conditions. This restriction guarantees that concurrent access to mutable data is properly synchronized, making Rust programs inherently thread-safe and avoiding subtle bugs caused by data races.
While the limitation of a single mutable reference might require some adjustments in how you structure your code, it contributes to the overall safety and reliability of your Rust programs. It encourages explicit and intentional control over mutable access, reducing the chances of programming errors and making it easier to reason about code behavior.
In situations where you need to modify data from different parts of your code simultaneously, Rust provides mechanisms like interior mutability, which allows for controlled mutability through specific types such as Cell
, RefCell
, or Mutex
. These constructs ensure that mutations are synchronized and safe, even in multi-threaded scenarios.
6. Simultaneous Access with Synchronization Primitives
While Rust’s ownership and borrowing model restricts simultaneous mutable access to a variable, there are cases where you genuinely need multiple references for concurrent read or write operations. Rust provides synchronization primitives that allow controlled and safe simultaneous access to shared data, ensuring thread safety and preventing data races. Let’s explore one such synchronization primitive, the mutex, and how it enables concurrent access without compromising safety.
Mutex: Synchronizing Exclusive Access
One of the most commonly used synchronization primitives in Rust is the mutex (short for mutual exclusion). A mutex allows multiple threads to access a shared resource, but only one thread can have exclusive access to it at a time. Other threads must wait until the owning thread releases the lock.
Example: Simultaneous Access with Mutex
use std::sync::{Mutex, Arc};
use std::thread;
fn main() {
let counter = Arc::new(Mutex::new(0));
let mut handles = vec![];
for _ in 0..10 {
let counter = Arc::clone(&counter);
let handle = thread::spawn(move || {
let mut data = counter.lock().unwrap();
*data += 1;
});
handles.push(handle);
}
for handle in handles {
handle.join().expect("Thread panicked");
}
println!("Counter value: {:?}", *counter.lock().unwrap());
}
In this example, we use an additional synchronization primitive, Arc
(short for atomic reference counting), to share ownership of the mutex counter
among multiple threads. Arc
enables multiple ownership of a value across threads, ensuring its reference count is properly tracked.
We create an Arc
around the mutex Mutex::new(0)
, which initializes the shared counter to zero. Each thread receives a cloned Arc
reference to the counter, incrementing it by acquiring a lock on the mutex using the lock
method. Once the increment is complete, the lock is automatically released as the data
variable goes out of scope.
By using Arc
to share ownership of the mutex, we ensure that each thread can safely access and modify the counter concurrently. The mutex enforces exclusive access, preventing data races and guaranteeing consistent results.
Using synchronization primitives like mutexes and smart pointers like Arc
enables Rust programmers to handle cases where simultaneous access to shared data is necessary while ensuring thread safety and preventing data races. By combining these primitives with Rust’s ownership and borrowing model, you can build concurrent applications that are both safe and efficient.
Conclusion:
In summary, Rust is a programming language that offers a unique set of features and concepts to developers. Its ownership and borrowing system ensures memory safety and eliminates common bugs, while pattern matching provides a concise way to handle complex data structures. Lifetimes enforce reference management and prevent dangling references.
Rust’s synchronization primitives enable safe concurrent access to shared data, and its support for functional programming and object-oriented programming allows for expressive and modular code. Immutability by default, higher-order functions, structs, methods, traits, and polymorphism contribute to Rust’s versatility.
By mastering these core features, developers can write robust, efficient, and safe code in Rust. The language’s growing ecosystem and active community make it an exciting choice for a wide range of applications. Embrace Rust’s philosophy of safe systems programming and unlock the potential of this powerful language.
Subscribe to my newsletter
Read articles from Oyinbo David Bayode directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
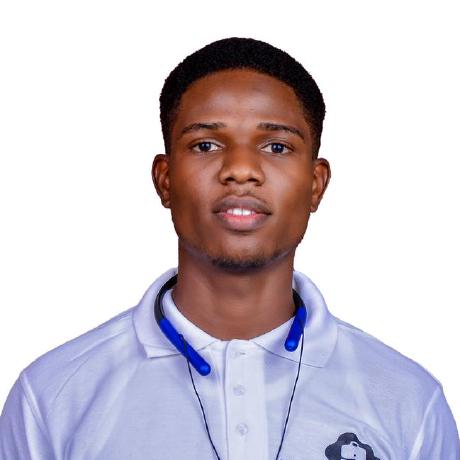