Securing Web Applications with Laravel Authentication and Authorization Features
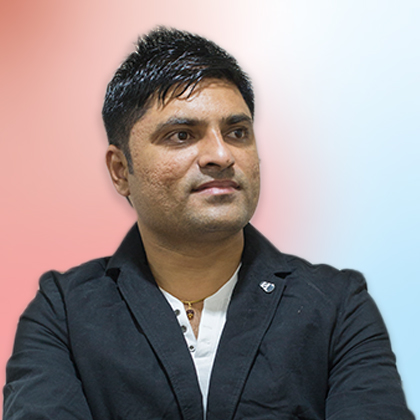
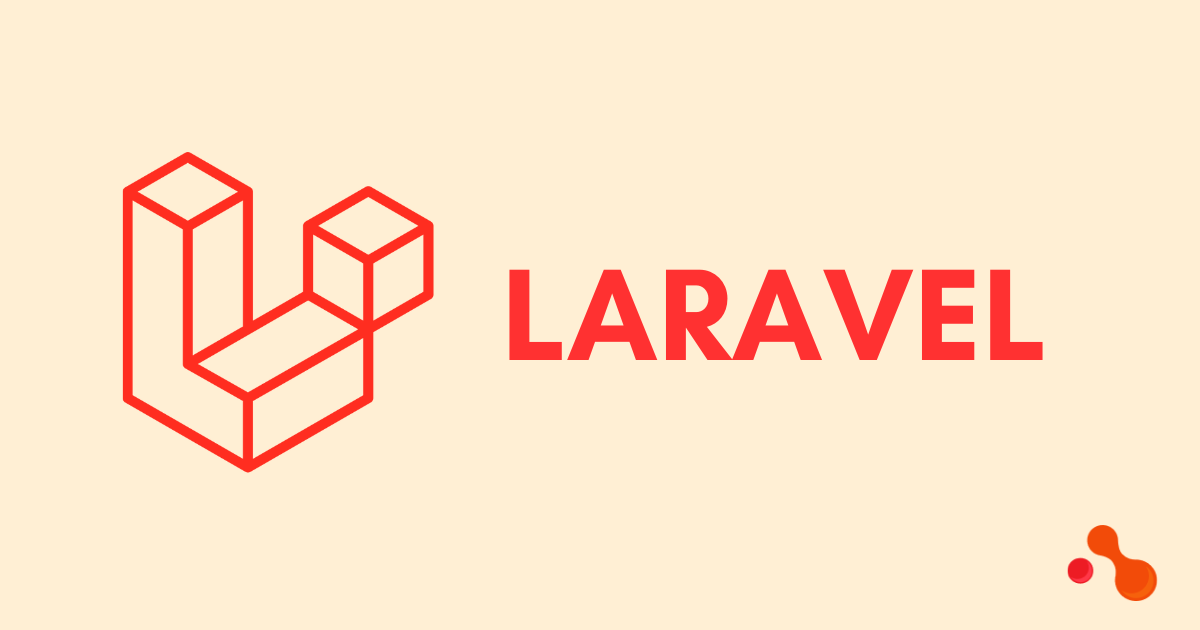
I. Introduction
In today's digital world, web application security is crucial to safeguard our personal information and prevent unauthorized access to sensitive data. Securing web applications is a top priority for developers and businesses alike. One powerful tool that helps achieve this is Laravel, a popular PHP framework. Laravel comes with built-in authentication and authorization features that make it easier to protect our applications. In this blog, we'll explore the significance of web application security, and how Laravel's authentication and authorization features work together to provide a safer online experience for everyone.
II. Understanding Laravel Authentication
Authentication is the process of verifying the identity of users accessing a web application. Laravel, a popular PHP framework, offers robust built-in authentication features to make this process secure and straightforward for developers. Here's a breakdown of how Laravel handles authentication:
A. What is Authentication? Authentication is the process of confirming whether a user is who they claim to be before granting access to certain features or resources within a web application.
B. How Laravel Handles Authentication
User Registration and Login
Laravel provides pre-built methods for user registration and login, making it easy to create signup and login forms.
Passwords are hashed, ensuring that sensitive information remains secure in the database.
Password Reset Functionality
Laravel includes a password reset feature out of the box, allowing users to reset their passwords if forgotten.
Secure reset tokens are generated and verified to prevent unauthorized access.
Remember Me Functionality
- Laravel allows users to opt for a "Remember Me" option during login, enabling automatic authentication on subsequent visits.
C. Customizing Authentication in Laravel
Custom User Providers
- Developers can create custom user providers to integrate Laravel with various authentication systems or user repositories.
Multi-Authentication
- Laravel supports multiple authentication guard configurations, enabling different authentication methods for various user types (e.g., users and admins).
Social Media Authentication
- Laravel supports seamless authentication via social media platforms like Facebook, Twitter, or Google, allowing users to log in using their social accounts.
In summary, Laravel's authentication features simplify the process of securing web applications, making it accessible for developers to build secure login systems without reinventing the wheel.
III. Laravel's Authorization Fundamentals
A. What is Authorization?
Authorization is the process of determining whether a user is allowed to perform specific actions within a web application.
It ensures that users can only access resources and perform actions they are authorized to do.
B. Role-Based Access Control (RBAC) in Laravel
RBAC is a popular authorization model where permissions are assigned based on user roles.
Laravel provides built-in support for RBAC, making it easier to manage access control.
C. Policies and Gates
Creating Policies
Policies are classes that define authorization rules for specific resources, like models or controllers.
They centralize the authorization logic for better maintainability.
Defining Authorization Logic
Inside policies, you can define methods corresponding to actions (e.g., view, create) and check user permissions.
The logic determines whether a user with a specific role can perform the action on a given resource.
Using Gates for Fine-Grained Authorization
Gates are more dynamic and can handle complex authorization logic for various actions and resources.
They offer a more granular level of control over access to application features.
Understanding Laravel's authorization fundamentals helps ensure your web application remains secure and only grants access to authorized users, protecting sensitive data and preventing unauthorized actions.
IV. Implementing Authentication in Laravel
Laravel development allows you to build secure web applications, and one crucial aspect is implementing authentication to protect user data. Let's explore the steps involved in setting up authentication in Laravel:
A. Setting Up a New Laravel Project:
Install Laravel using Composer, a PHP package manager.
Create a new project with the 'laravel new project-name' command.
B. Configuring Authentication Middleware:
Laravel provides pre-built authentication middleware to protect routes.
Add the 'auth' middleware to specific routes or groups in the 'routes/web.php' file.
C. Creating User Registration and Login Functionality:
Use Laravel's built-in 'make:auth' command to generate registration and login views and controllers.
Customize the views and logic to match your application's requirements.
D. Implementing Password Reset Functionality:
Laravel offers an easy way to add password reset functionality.
Use 'php artisan make:auth' command to generate password reset views and controllers.
Configure the mailing settings in the '.env' file for password reset emails.
E. Enabling "Remember Me" Functionality:
Laravel provides a 'remember me' feature to keep users logged in.
Enable it by adding the 'remember_token' column to the users' database table.
By following these steps, your Laravel web application will have a strong authentication system, providing enhanced security for your users' data. As Laravel development services can be outsourced to a reliable Laravel web development company, even non-experts can benefit from robust security in their applications.
V. Leveraging Laravel Authorization Features
In Laravel development, ensuring the security of your web application is of utmost importance. Laravel offers powerful authentication and authorization features to safeguard your application and control user access effectively. Let's explore how to leverage Laravel's authorization features in a straightforward manner:
A. Setting Up Roles and Permissions:
Define user roles (e.g., admin, user, moderator) and their corresponding permissions.
Assign roles to users based on their responsibilities and access requirements.
Use packages like "Spatie/permissions" to simplify role and permission management.
B. Creating and Applying Policies:
Policies allow fine-grained control over model authorization.
Define policies for resources like posts, comments, or any data you want to protect.
Implement policies with easy-to-understand rules to grant or deny access.
C. Implementing Authorization with Gates:
Gates are useful for handling complex authorization logic.
Set up gates to manage access based on user attributes or custom logic.
Apply gates in your controllers and views to control user actions.
D. Handling Unauthorized Access:
Customize error responses for unauthorized access.
Use middleware to redirect or display proper messages for unauthorized users.
Secure sensitive routes with middleware to prevent unauthorized entry.
By understanding and implementing these Laravel authorization features, you can enhance your web application's security and provide users with the right level of access, making your Laravel web development company's services even more reliable and trustworthy.
VI. Best Practices for Securing Laravel Applications
Laravel development is gaining popularity due to its robust features, but it's crucial to prioritize security when building web applications. Protecting user data and preventing unauthorized access should be top priorities. Here are essential best practices to secure your Laravel web development:
A. Securing Passwords and Sensitive Data
Hash passwords using bcrypt for strong encryption.
Never store sensitive data in plain text.
Use Laravel's encryption for additional security.
B. Handling User Sessions Securely
Implement secure session management to prevent session hijacking.
Set session expiration to reduce the risk of unauthorized access.
C. Protecting Against Cross-Site Request Forgery (CSRF) Attacks
Apply Laravel's built-in CSRF protection using tokens.
Verify requests with CSRF middleware.
D. Utilizing HTTPS and SSL Certificates
Enable HTTPS to encrypt data transmitted between the server and clients.
Install SSL certificates to ensure secure connections.
E. Regularly Updating Dependencies and Laravel Version
Keep dependencies up-to-date to patch security vulnerabilities.
Upgrade to the latest Laravel version for improved security features.
By adhering to these best practices, Laravel development services and companies can build secure web applications, instilling confidence in users and protecting sensitive data from potential threats.
VII. Advanced Security Techniques
In addition to basic authentication and authorization, implementing advanced security techniques is crucial for protecting your web applications from sophisticated threats. Here are five essential techniques explained in simple terms:
A. Two-Factor Authentication (2FA):
Requires users to provide two forms of identification before accessing their accounts.
Commonly involves a combination of a password and a one-time code sent to their mobile device.
Adds an extra layer of security, reducing the risk of unauthorized access.
B. OAuth and Social Media Integration:
OAuth allows users to log in using their social media accounts (e.g., Facebook, Google).
Enhances user experience by eliminating the need for separate login credentials.
Developers should implement OAuth securely to prevent data breaches.
C. API Token Authentication:
Grants access to specific parts of the application using unique tokens.
Useful for providing access to external services or allowing users to interact with your API securely.
D. Content Security Policy (CSP):
Mitigates cross-site scripting (XSS) attacks by defining which sources of content are allowed to be loaded.
Prevents malicious scripts from executing on your web pages.
E. Rate Limiting to Prevent Brute Force Attacks:
Limits the number of login attempts or API requests a user can make within a specific timeframe.
Protects against brute force attacks, where attackers try various combinations to gain unauthorized access.
By understanding and implementing these advanced security techniques, you can significantly enhance the safety of your web applications, safeguarding both your data and your users' information.
VIII. Dealing with Common Security Vulnerabilities
A. SQL Injection
SQL injection is a type of attack where attackers manipulate a web application's database by inserting malicious SQL code.
Prevention: Use parameterized queries or prepared statements in your code to validate and sanitize user inputs.
B. Cross-Site Scripting (XSS)
XSS occurs when attackers inject malicious scripts into web pages viewed by other users.
Prevention: Always sanitize and validate user inputs and use output encoding to prevent script execution.
C. Cross-Site Request Forgery (CSRF)
CSRF attacks trick authenticated users into unknowingly performing actions on a website they did not intend to.
Prevention: Implement CSRF tokens in your forms to ensure requests come from trusted sources.
D. Session Hijacking and Fixation
Session hijacking involves unauthorized users stealing valid session IDs to impersonate others.
Prevention: Use secure session handling mechanisms, enable secure cookies, and rotate session IDs regularly.
E. Mass Assignment Vulnerabilities
Mass assignment vulnerabilities allow attackers to modify data they shouldn't have access to through request parameters.
Prevention: Implement proper input validation and use Laravel's built-in protection against mass assignment vulnerabilities.
IX. Security Testing and Quality Assurance
A. Writing Security Tests
Develop automated tests specifically targeting security vulnerabilities in your application.
Use tools like PHPUnit or Laravel Dusk to create security test cases.
B. Conducting Vulnerability Assessments
Regularly perform vulnerability assessments to identify weaknesses in your application's security.
Use tools like OWASP ZAP or Burp Suite to scan for potential vulnerabilities.
C. Code Reviews and Peer Testing
Have experienced developers review your code for security flaws and best practices.
Encourage peer testing and constructive feedback to improve overall security.
D. Using Security Scanning Tools
Utilize automated security scanning tools to identify potential vulnerabilities in your codebase.
Regularly update and run these tools to keep your application secure.
Remember, securing web applications is an ongoing process, and no system can be entirely foolproof. However, by implementing best practices and staying vigilant about security, you can significantly reduce the risk of attacks and keep your users' data safe. Always prioritize security in your development process and stay informed about the latest security trends and updates in the Laravel community.
X. Conclusion
In conclusion, Laravel offers powerful tools for securing web applications through its authentication and authorization features. Authentication ensures only authorized users can access sensitive parts of a site, while authorization allows fine-tuning access permissions. Prioritizing web application security is crucial in safeguarding user data and maintaining trust. By following best practices, such as securing passwords, using HTTPS, and keeping dependencies up-to-date, developers can create safer web applications. Embracing Laravel's security offerings and implementing proper security measures will go a long way in protecting both users and the application itself. Let's build safer web experiences together with Laravel!
Subscribe to my newsletter
Read articles from Hire Remote Developers directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
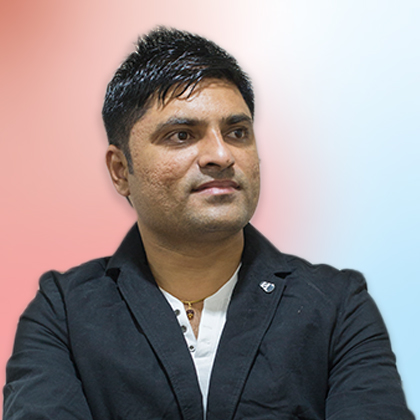
Hire Remote Developers
Hire Remote Developers
Hi, I am Mukesh Ram I founded Acquaint Softtech with a vision to make quality developers affordable to everyone. With my blood, sweat, and tears I haven’t just been able to sustain but thrive over the years. Today, I am proud to say that we have grown to a family of over 70 awesome people and expanding quickly. Every day I look forward to uniting with my professional family to provide them the best environment to grow their careers and propel us towards fulfilling the vision of Acquaint Softtech. And crack jokes in between! On a personal front, I am a foodie and a life enthusiast who loves to live each moment to the fullest.