Learn the Python language from 0
Table of contents
- Introduction
- Installing Python and choosing a development environment
- 2. Installing VSCode:
- 3. Installing Git Bash:
- 4. Configure Python in VSCode:
- 5. Configure Git Bash to use Python:
- The basics of Python programming
- Basic Python syntax
- Sample Code: Variables and Data Types python
- Variable declarations and display variable values
- Variable declarations
- Get user information with input()
- Store data in a list
- Sets (set):
- Dictionaries (dict):
- Tuples (tuple):
- Exploring possibilities with control structures
- Loops
- List Comprehensions
- Functions:
- Function with Parameters:
- Function with Default Parameters:
- Introduction to Libraries
- The power of Python libraries : Extend Python's capabilities with pi
- Going Further with Object-Oriented Programming (OOP):
- Going further in Python
- Using GitHub: Uploading Your Python Files
- Conclusion
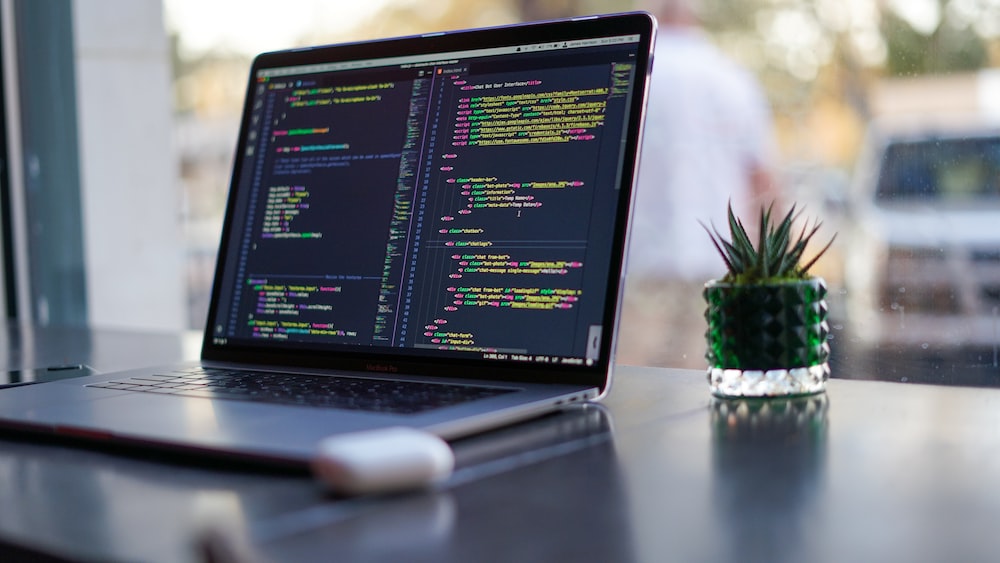
Introduction
It's not unusual these days to hear about languages like Python, javascript and Kotlin, and many people wonder how to learn them from scratch. Where do you start if you want to get good at any programming language ? Well, we're delighted to tell you about learning one of the most popular programming languages, Python. Indeed, Python is a very popular programming language in the scientific world, as it's used for both calculations of large masses of data and providing relevant results, flexible syntax and a widespread community. You'll probably find this language in the world of Artificial Intelligence, where its tools are widespread, in web development, where it's widely used with frameworks such as Flask and Django, and in other fields such as data science.
Installing Python and choosing a development environment
The development environment refers to a set of tools that enable you to work on, execute or modify Python code. That's why, before you start coding in Python, you'll need to install the Python interpreter on your system. We'll show you how to do this, and guide you in choosing a development environment suited to your needs.
To install Python language on different operating systems and configure development tools such as VSCode and Git Bash on Windows, follow the steps below:
1. Installing Python:
On Windows:
Go to the official Python website: https://www.python.org/downloads/
Download the installer for the version of Python you want to install (eg Python 3.9).
Run the installer and check the option "Add Python x.x to PATH" so that Python is added to the system PATH (important to use it from the terminal).
On macOS:
- macOS comes with a pre-installed version of Python. You can check the current version by opening the terminal and typing "python --version". To install a specific version or a newer version, you can use Homebrew or pyenv.
On Linux:
- Most Linux distributions come with Python pre-installed. You can check the current version by opening the terminal and typing "python --version". If Python is not already installed, you can install it from your distro's official repositories.
2. Installing VSCode:
Visual Studio Code, often abbreviated as VS Code, is a lightweight, free, and open-source code editor (an application for writing code) developed by Microsoft. It is designed for use by developers and supports many programming languages including Python, JavaScript, HTML, CSS, C++, and many more. It has many features like:
User-friendly interface: VS Code has a clean and intuitive user interface that makes it easy to navigate and edit code.
Rich extension: It supports a wide range of community-developed extensions, which allows users to add additional features as per their needs.
Git integration: VS Code has native Git integration that makes it easy to manage code versions right from the editor.
Integrated debugging: It allows to debug the code directly from the editor, which simplifies the debugging process.
To install Vscode go to Visual Studio Code official website: https://code.visualstudio.com/ and download the installation program corresponding to your operating system (Windows, macOS, or Linux).
Run the installer and follow the instructions to install VSCode on your computer.
3. Installing Git Bash:
Git Bash is a terminal emulator for Windows that provides a Linux-like command line interface (CLI) for running Git commands. Git is a widely used version control system for tracking changes to project source code files. This allows developers to collaborate efficiently, roll back to earlier versions of code, and manage code evolution over time.
It is widely used because of its many advantages:
Command-line interface: Git Bash provides a command-line interface similar to that of a Unix terminal, making it easy to execute Git commands.
Git support: Git Bash includes all Git commands, so developers can easily manage Git repositories on their local machine.
Unix-like environment: Git Bash provides a Unix-like environment, meaning that developers familiar with Unix/Linux commands can work with ease.
For installation, go to the official Git website: https://git-scm.com/downloads
Download the installation program corresponding to your operating system (Windows).
Run the installer and follow the instructions to install Git Bash on your computer.
4. Configure Python in VSCode:
Open VSCode.
Install the "Python" extension from the extension market (Ctrl+Shift+X, then search for "Python" and install the extension offered by Microsoft).
After installation, open a project folder containing your Python files.
Make sure the correct Python interpreter is selected in VSCode. You can do this at the bottom left of the VSCode window, by clicking on the current interpreter and choosing the Python interpreter you installed earlier.
5. Configure Git Bash to use Python:
Open Git Bash.
To check if Python is correctly configured in Git Bash, type "python --version" in the Git Bash terminal. This should display the version of Python you installed previously.
You now have Python installed on your OS and configured with VSCode and Git Bash (for Windows) development tools. You are ready to start developing in Python on your platform!
Creating, Saving, and Running a Python File on VSCode (Including the "Play" Option)
1. Creating the Python File on VSCode:
Launch Visual Studio Code (VSCode) on your operating system.
Open a new file by clicking on "File" in the menu bar, then "New File," or use the keyboard shortcut
Ctrl + N
(Windows/Linux) orCmd + N
(macOS).Name your file with the ".py" extension, for example, "my_script.py".
2. Editing and Saving the Code:
Write your Python code in the newly created file.
To save the file, use the keyboard shortcut
Ctrl + S
(Windows/Linux) orCmd + S
(macOS), or simply click on "File" in the menu bar, then "Save" (or "Save As" to save it to a specific location).Choose the directory where you want to save the Python file and click "Save."
3. Running the Python File on VSCode:
Option 1: Using the "Play" Button
- In the top right corner of the VSCode window, you will see a "Run" button (a green triangle). Click on it to run your Python file.
Option 2: Using the Terminal
Open a terminal in VSCode by clicking on "View" in the menu bar, then "Terminal," or use the keyboard shortcut
Ctrl + `` (Windows/Linux) or
Cmd + `` (macOS).In the terminal, make sure you are in the directory where you saved the Python file. You can use the
cd
command to navigate to the desired folder. For example:cd /path/to/your_folder
.To run the Python file, use the
python
command followed by the name of your file. For example:python my_script.py
.
Running the Python File in Command Line (on All Operating Systems):
Open a terminal or command prompt on your operating system.
Navigate to the directory where you saved the Python file using the
cd
command. For example:cd /path/to/your_folder
.To run the Python file, use the
python
command followed by the name of your file. For example:python my_script.py
.
The basics of Python programming
In this section, we'll explore the fundamental concepts of Python that serve as the basis for writing efficient programs. We'll cover variables and data types, control structures such as loops and conditions, and functions for organizing and reusing code.
Basic Python syntax
This section introduces you to the basic syntax of Python through concrete examples. You'll learn how to write your first "Hello, world!" program, manipulate variables, perform mathematical operations and use essential control structures. Sample code: "Hello, world!" program
Go to your code editor (Vscode in our case) write this piece of code.
print("Hello world")
The code snippet print("hello world")
is an instruction that displays the text "hello world" on the screen. In Python, print() is a built-in function (or native function) for displaying information to the console.
When this code is executed, the result will simply be the display of the text "hello world" in the standard output, usually the console or terminal where the program is running.
Sample Code: Variables and Data Types python
Variable declarations and display variable values
In Python, data types define the nature and representation of values that we can manipulate in a program. Here's a brief explanation of the main data types in Python:
Integers (int): Integers are whole numbers without decimals (positive, negative, or zero). For example: 1, -5, 100, 0.
Floating-Point Numbers (float): Floating-point numbers represent real numbers with a decimal part. For example: 3.14, -0.5, 2.0.
Strings (str): Strings are sequences of characters, enclosed in either single quotes ('') or double quotes (""). For example: "Hello, World!", 'Python'.
Booleans (bool): Booleans represent truth values, either True or False. They are used for logical and comparison operations.
Lists (list): Lists are ordered and mutable collections of elements of different types. They are defined using square brackets and can contain zero or multiple elements.
Tuples (tuple): Tuples are similar to lists, but they are immutable, meaning once created, their elements cannot be changed.
Sets (set): Sets are unordered collections of unique elements. They are useful for performing mathematical operations like union, intersection, etc.
Dictionaries (dict): Dictionaries are associative collections that allow storing key-value pairs. Each key must be unique and is used to access the associated value.
Python is a dynamically typed language, which means that variables can change types depending on the value assigned to them. For example, a variable can hold an integer at one point and then be changed to hold a string at another point. This flexibility in data types makes Python very adaptable and easy to use for a wide range of programming tasks.
Variable declarations
# Variable declarations
name = "John" # Declare a variable 'name' with the value "John"
age = 30 # Declare a variable 'age' with the value 30
height = 1.75 # Declare a variable 'height' with the value 1.75
is_adult = True # Declare a variable 'is_adult' with the value True
# Displaying the variable values
print("Name:", name) # Prints "Name: John"
print("Age:", age) # Prints "Age: 30"
print("Height:", height) # Prints "Height: 1.75"
print("Is adult:", is_adult) # Prints "Is adult: True"
Get user information with input()
Beyond the display, we may also want to retrieve information that is written by a user at the keyboard. this is where another function comes in: the '''input()" function that we will associate with a variable and integrating between pins the message that we want to display to the user so that he knows what he must enter
# Variable declarations
name = input("Enter your name: ") # Ask the user to input their name and store it in the 'name' variable
age = int(input("Enter your age: ")) # Ask the user to input their age and convert it to an integer before storing it in the 'age' variable
height = float(input("Enter your height in meters: ")) # Ask the user to input their height and convert it to a float before storing it in the 'height' variable
is_adult_input = input("Are you an adult? (yes/no): ") # Ask the user to input whether they are an adult and store it in the 'is_adult_input' variable
# Convert the 'is_adult_input' to a boolean value (True or False) based on the user's input
is_adult = is_adult_input.lower() == 'yes' # Convert the input to lowercase and check if it is 'yes', then store the result in the 'is_adult' variable
# Displaying the variable values
print("Name:", name) # Prints the name entered by the user
print("Age:", age) # Prints the age entered by the user
print("Height:", height) # Prints the height entered by the user
print("Is adult:", is_adult) # Prints whether the user is an adult (True or False)
Please note that the input() function captures user input as a string. If we wish to store the values as other data types, we need to perform an appropriate conversion. In the example, we use int() to convert the age input to an integer and float() to convert the size input to a decimal number.
Store data in a list
A list in Python is an ordered collection of elements, where each element is identified by an index (a number) that indicates its position in the list. Lists can contain elements of different data types, such as integers, strings, decimal numbers, Booleans and even other lists. Lists are defined using square brackets [] and elements are separated by commas.
Python
# List of integers
my_integer_list = [1, 2, 3, 4, 5]
# List of strings
my_string_list = ["apple", "banana", "orange", "kiwi"]
# Mixed list with different data types
my_mixed_list = [10, "Hello", 3.14, True]
# Accessing elements by index
first_element = my_integer_list[0]
last_element = my_string_list[-1]
# Modifying an element in the list
my_integer_list[2] = 10
# Adding an element to the end of the list
my_string_list.append("mango")
# Extending the list with more elements
my_integer_list.extend([6, 7, 8, 9, 10])
# Removing an element from the list
del my_mixed_list[1]
# Length of a list
list_length = len(my_integer_list)
# Checking if an element is in the list
is_present = "apple" in my_string_list
# Displaying the results
print("List of integers:", my_integer_list)
print("List of strings:", my_string_list)
print("Mixed list:", my_mixed_list)
print("First element:", first_element)
print("Last element:", last_element)
print("Modified integer list:", my_integer_list)
print("Modified string list:", my_string_list)
print("Extended integer list:", my_integer_list)
print("Length of integer list:", list_length)
print("Is 'apple' in the string list?", is_present)
Sets (set):
A set is an unordered collection of unique elements. Sets are defined using curly braces {}
or the built-in set()
function. Duplicate elements are automatically removed from sets.
# Example of a set
fruits_set = {"apple", "banana", "orange", "kiwi", "banana"}
# Output: {'kiwi', 'banana', 'orange', 'apple'}
print(fruits_set)
In this example, we define a set called fruits_set
containing four elements: "apple", "banana", "orange", and "kiwi". Note that we included "banana" twice, but sets automatically eliminate duplicates. When we print the set, it displays all unique elements in an unordered manner.
Dictionaries (dict):
A dictionary is an associative collection that stores key-value pairs. Dictionaries are defined using curly braces {}
with key-value pairs separated by colons :
.
# Example of a dictionary
student_scores = {"John": 85, "Alice": 92, "Bob": 78}
# Accessing values using keys
john_score = student_scores["John"] # Output: 85
alice_score = student_scores["Alice"] # Output: 92
In this example, we have a dictionary called student_scores
that associates the names of students with their corresponding scores. Each student's name is a key, and their score is the corresponding value. We can access the values by providing the keys within square brackets.
Tuples (tuple):
A tuple is a collection of ordered and immutable elements. Tuples are defined using parentheses ()
or just a comma-separated sequence of elements.
# Example of a tuple
person_info = ("John Doe", 30, "New York")
# Accessing elements in a tuple
name = person_info[0] # Output: "John Doe"
age = person_info[1] # Output: 30
location = person_info[2] # Output: "New York"
In this example, we have a tuple named person_info
that contains three elements: a person's name, age, and location. Tuples are immutable, meaning their elements cannot be changed after creation. We can access elements in a tuple using their index, just like in a list.
Tuples are often used to represent a fixed collection of related values, such as coordinates (x, y) or RGB color values (red, green, blue). Due to their immutability, tuples provide a level of data integrity and safety when working with constant data sets.
Exploring possibilities with control structures
The code in a programming language offers the possibility of choosing between several options, a bit like in the menu of a restaurant, we would surely want to choose a dish. These numerous possibilities also allow to check if certain values entered by the user are correct.
# Variable declarations
name = input("Enter your name: ")
age = int(input("Enter your age: "))
height = float(input("Enter your height in meters: "))
is_adult = age >= 18
# Control structure (if-else)
if is_adult:
print("Hello", name + "! You are an adult.")
else:
print("Hello", name + "! You are not yet an adult.")
# Control structure (if-elif-else)
if age == 18:
print("Congratulations on turning 18,", name + "!")
elif age < 18:
print("You are still a minor,", name + ".")
else:
print("You have passed 18 years of age,", name + ".")
# Control structure (nested if-else)
if is_adult:
if height > 1.80:
print("You are a tall adult!")
else:
print("You are an adult with an average height.")
else:
print("Since you are not an adult, we won't check your height.")
this example, we've used three different control structures:
The first control structure (if-else) checks if the user is an adult based on their age and prints an appropriate message.
The second control structure (if-elif-else) checks if the user has turned exactly 18 years old, and prints a special message for this case. If the user's age is below 18, it prints a message stating that they are still a minor, and if the user's age is above 18, it prints a message indicating that they have passed 18 years of age.
The third control structure (nested if-else) first checks if the user is an adult and then checks if their height is above 1.80 meters. Depending on the conditions, it prints different messages about the user's height.
Loops
In programming, loops are used to execute a block of code repeatedly until a certain condition is met. Python provides two main types of loops: for
loop and while
loop.
For Loop:
A for
loop is used to iterate over a sequence (such as a list, tuple, string, etc.) and execute a block of code for each element in the sequence.
# Example of a for loop
fruits = ["apple", "banana", "orange", "kiwi"]
for fruit in fruits:
print(fruit)
Explanation: In this example, we have a list of fruits, and we use a for
loop to iterate over each element in the list. The loop assigns each fruit to the variable fruit
, and then the code inside the loop (in this case, print(fruit)
) is executed for each fruit in the list. The loop will output each fruit on separate lines.
While Loop:
A while
loop is used to repeatedly execute a block of code as long as a certain condition is true.
# Example of a while loop
count = 0
while count < 5:
print("Count:", count)
count += 1
Explanation: In this example, we have a variable count
initialized to 0. The while
loop checks the condition count < 5
. As long as the condition is true, the code inside the loop (in this case, print("Count:", count)
) will be executed. The loop increments the count
variable by 1 in each iteration until count
becomes 5. The loop will print the count from 0 to 4.
Loop Control Statements:
Python also provides loop control statements to modify the behavior of loops:
- Break Statement: The
break
statement is used to exit the loop prematurely when a certain condition is met.
# Example of break statement
numbers = [1, 2, 3, 4, 5]
for num in numbers:
if num == 3:
break
print(num)
Output:
1
2
- Continue Statement: The
continue
statement is used to skip the rest of the loop's current iteration and continue with the next iteration.
# Example of continue statement
numbers = [1, 2, 3, 4, 5]
for num in numbers:
if num == 3:
continue
print(num)
Output:
1
2
4
5
List Comprehensions
List comprehensions are a concise and powerful way to create lists in Python. They provide a compact syntax to generate a new list by performing an operation on each element of an existing list (or other iterable) while applying a condition (optional).
The general syntax of a list comprehension is as follows:
new_list = [expression for item in iterable if condition]
Explanation:
expression
: Represents the operation to be performed on each element of the iterable. It could be a simple value, a calculation, or any transformation applied to the element.item
: Represents each element in the iterable that we are iterating over.iterable
: Represents the existing list, tuple, string, or any other iterable from which we are creating the new list.condition
(optional): Represents an optional filtering condition. If specified, only the elements that meet the condition will be included in the new list.
Example 1: Creating a list of squares using a for loop and a list comprehension.
# Using a for loop
numbers = [1, 2, 3, 4, 5]
squares = []
for num in numbers:
squares.append(num ** 2)
print(squares) # Output: [1, 4, 9, 16, 25]
# Using list comprehension
numbers = [1, 2, 3, 4, 5]
squares = [num ** 2 for num in numbers]
print(squares) # Output: [1, 4, 9, 16, 25]
Example 2: Creating a list of even numbers using a for loop and a list comprehension with a condition.
# Using a for loop
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
even_numbers = []
for num in numbers:
if num % 2 == 0:
even_numbers.append(num)
print(even_numbers) # Output: [2, 4, 6, 8, 10]
# Using list comprehension with a condition
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
even_numbers = [num for num in numbers if num % 2 == 0]
print(even_numbers) # Output: [2, 4, 6, 8, 10].
Functions:
In Python, a function is a reusable block of code that performs a specific task or set of tasks. Functions are defined using the def
keyword, followed by the function name, a set of parentheses ()
, and a colon :
. The code block that makes up the function is indented below the function definition.
# Example of a simple function
def greet():
print("Hello, welcome!")
# Calling the function
greet() # Output: Hello, welcome!
In this example, we define a function called greet()
that prints the message "Hello, welcome!". The function is created using the def
keyword, and it does not take any input parameters (indicated by empty parentheses ()
). When the function is called using greet()
, it executes the code inside the function and displays the output.
Function with Parameters:
Functions can also take input parameters (arguments) to perform tasks based on specific values provided during the function call.
# Example of a function with parameters
def add_numbers(a, b):
result = a + b
return result
# Calling the function
sum_result = add_numbers(5, 10)
print(sum_result) # Output: 15
Explanation: In this example, we define a function called add_numbers(a, b)
that takes two parameters a
and b
. The function calculates the sum of these two numbers and stores the result in the variable result
. The return
keyword is used to specify the value to be returned from the function.
When we call the function add_numbers(5, 10)
, it adds the values 5
and 10
, and the returned result (15
) is stored in the variable sum_result
. We then print the value of sum_result
, which displays 15
.
Function with Default Parameters:
Python allows you to set default values for function parameters. If a value is not provided for a default parameter during the function call, the default value will be used.
# Example of a function with default parameter
def greet_person(name="Guest"):
print("Hello,", name)
# Calling the function
greet_person() # Output: Hello, Guest
greet_person("John") # Output: Hello, John
Explanation: In this example, we define a function called greet_person(name="Guest")
that takes a parameter name
with a default value of "Guest". If no name is provided during the function call, it uses the default value "Guest". Otherwise, it greets the person by their name.
When we call the function with greet_person()
, it uses the default value "Guest" and prints "Hello, Guest". When we call the function with greet_person("John")
, it greets the person named "John" and prints "Hello, John".
Introduction to Libraries
In Python, libraries (also known as modules) are sets of pre-written functions, classes, and methods that extend the core functionalities of the language. They allow developers to access advanced features without having to reinvent the wheel. Libraries are essential in making Python so versatile and widely used in various domains such as web development, data science, artificial intelligence, and more.
To use a library in your Python code, you need to import it using the import
statement. Once imported, you can access its features and utilize its classes and methods in your program.
Built-in and Downloadable Libraries (or Modules):
Python libraries are classified into two main categories:
- Built-in Libraries (Built-in Libraries):
Built-in libraries are libraries that come pre-installed with Python, meaning you don't need to install them separately. These libraries are available in all Python environments and can be used without downloading them.
Some examples of built-in libraries are: math
, random
, datetime
, sys
, os
, json
, csv
, etc. These libraries provide basic functionalities for performing mathematical operations, managing dates and times, handling files and directories, working with data in JSON or CSV format, and more.
- Downloadable Libraries (or Modules):
Downloadable libraries are third-party libraries that are not included in the basic installation of Python. You need to install them separately using pip
before you can use them in your programs.
These libraries extend the capabilities of Python for specific tasks such as scientific computing (NumPy, SciPy), data processing (Pandas), machine learning (scikit-learn, TensorFlow, PyTorch), web development (Django, Flask), data visualization (Matplotlib, Seaborn), and more.
Using downloadable libraries allows developers to leverage a vast community of contributors and access powerful tools to efficiently and rapidly solve complex problems.
Example with the math library:
The math
library is a standard library in Python that provides advanced mathematical functions. It contains functions for performing common mathematical operations such as calculating squares, square roots, trigonometric functions, etc.
Here's an example of using the math
library:
# Example with the math library
import math
# Calculating the square of a number
number = 5
square = math.pow(number, 2) # Using the pow() function from the math library
print("The square of", number, "is", square)
# Calculating the square root
number = 25
square_root = math.sqrt(number) # Using the sqrt() function from the math library
print("The square root of", number, "is", square_root)
In this example, we import the math
library using the import
statement. Then, we use two functions from the math
library: pow()
to calculate the square of a number and sqrt()
to calculate the square root of a number.
Example with the NumPy library:
The NumPy (Numerical Python) library is a highly popular library in Python, primarily used for mathematical operations and manipulations of multidimensional arrays (arrays). NumPy offers powerful functionalities for scientific computations and data manipulation.
Here's a simple example of using the NumPy library:
port numpy as np
# Creating a NumPy array from a list
my_list = [1, 2, 3, 4, 5]
my_array = np.array(my_list)
print("NumPy array:", my_array)
# Operations on the array
square_array = np.square(my_array) # Calculating the squares of the elements in the array
print("Squares of elements:", square_array)
sum_array = np.sum(my_array) # Calculating the sum of the elements in the array
print("Sum of elements:", sum_array)
In this example, we import the NumPy library using import numpy as np
, where np
is a commonly used alias for NumPy. Next, we create a NumPy array from a list using the np.array()
function. Then, we perform operations on the NumPy array, such as calculating the squares of its elements using np.square()
and finding the sum of its elements using np.sum()
.
NumPy is widely used in scientific and data science domains for fast and efficient numerical computations, making it one of the essential libraries in Python for data processing and mathematical calculations.
The power of Python libraries : Extend Python's capabilities with pi
Python, as an open-source programming language, is highly extensible, offering developers the possibility of accessing a wide range of functionality beyond the language's basic features. This is made possible through the use of libraries or external modules that have been developed and made available by other programmers. An essential tool facilitating this process is pip (Package Installer for Python).
With the help of pip, Python developers can easily discover, install and manage external libraries, enabling them to exploit existing solutions and add powerful functionality to their projects. This access to a vast ecosystem of libraries enables developers to streamline their development process, save time and create more sophisticated applications without having to reinvent the wheel.
In this context, we'll explore the process of installing libraries with pip, distinguish between built-in and downloadable libraries, and see how Python's versatility is amplified thanks to this collaborative ecosystem of contributing libraries. Let's dive into the world of Python libraries and their impact on the Python development landscape.
How to Install a Library with pip:
pip
is a Python package manager that facilitates the process of installing, updating, and removing third-party libraries.
To install a library using pip
, follow these steps:
Open a command prompt (or terminal) on your operating system.
Use the
pip install
command followed by the name of the library you want to install. For example, to install thenumpy
library, use the command:pip install numpy
.
pip
will automatically download and install the library and its dependencies if needed
An example
Here's a complete example that uses the googletrans
library to translate text without the need for an internet connection. We will be using the "offline" mode of the library, which allows us to perform translations using a local translation database.
Installing the googletrans Library:
Before running the example, make sure to install the googletrans
library using pip
. You can do this by opening a command prompt (or terminal) on your operating system and typing the following command:
pip install googletrans==4.0.0-rc1
Make sure to use the specific version 4.0.0-rc1
of googletrans
to enable the "offline" mode.
Once the library is installed, you can use the code below to perform translations without an internet connection using the googletrans
library in "offline" mode.
from googletrans import Translator
def translate_text_offline(text, target_language):
# Initialize the Translator in "offline" mode
translator = Translator(service_urls=['translate.googleapis.com'])
# Perform the translation without an internet connection
translated_text = translator.translate(text, dest=target_language)
return translated_text.text
# Text to translate
text_to_translate = "Hello, how are you?"
# Target language for translation (e.g., 'fr' for French)
target_language = 'fr'
try:
# Translate the text offline
translated_text = translate_text_offline(text_to_translate, target_language)
# Display the translated text
print("Original text:", text_to_translate)
print("Translated text:", translated_text)
except Exception as e:
print("An error occurred:", e)
Please note that the "offline" mode of the googletrans
library may not provide as comprehensive translation coverage as the online mode that relies on the Google Translate API. However, it can be useful in scenarios where an internet connection is not available or when dealing with sensitive data that you prefer not to transmit over the internet.
Introduction to Object-Oriented Programming (OOP):
Object-Oriented Programming (OOP) is a programming paradigm that revolves around the concept of objects. It is a powerful programming approach that helps in organizing code, making it more modular, maintainable, and scalable. In OOP, code is structured into objects, each representing a real-world entity or concept.
Key Concepts of Object-Oriented Programming:
Classes and Objects: In OOP, a class is a blueprint or template for creating objects. It defines the properties (attributes) and behaviors (methods) that the objects of the class will possess. An object is an instance of a class, and it represents a specific entity in the program.
Encapsulation: Encapsulation is the concept of bundling data (attributes) and methods (behaviors) together within a class. The internal details of the class are hidden from the outside, and only the necessary interfaces are exposed to interact with the object.
Inheritance: Inheritance is a mechanism where a class (subclass or derived class) can inherit properties and behaviors from another class (superclass or base class). It promotes code reusability and enables hierarchical relationships between classes.
Polymorphism: Polymorphism allows objects of different classes to be treated as objects of a common superclass. It enables the same method or function to work with different types of objects, providing flexibility and extensibility to the code.
Example: Creating a Simple Class in Python
Let's create a simple class in Python to demonstrate the basic concepts of OOP:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def say_hello(self):
print(f"Hello, my name is {self.name} and I am {self.age} years old.")
# Create an object of the Person class
person1 = Person("John", 30)
# Access object attributes and methods
print(person1.name) # Output: "John"
print(person1.age) # Output: 30
person1.say_hello() # Output: "Hello, my name is John and I am 30 years old."
In this example, we defined a Person
class with attributes name
and age
, and a method say_hello()
. We then created an object person1
of the Person
class and accessed its attributes and methods.
Inheritance in Object-Oriented Programming (OOP):
Inheritance is a key concept in OOP that allows a class (subclass or derived class) to inherit properties and behaviors from another class (superclass or base class). This enables code reusability and promotes the creation of hierarchical relationships between classes.
In Python, we use the syntax class Subclass(BaseClass):
to create a subclass that inherits from a base class. The subclass can access the attributes and methods of the base class and can also override or extend them as needed.
Example: Using Inheritance in Python
Let's create a simple example to illustrate inheritance:
# Base class
class Animal:
def __init__(self, species):
self.species = species
def make_sound(self):
print("Unknown sound")
# Subclass inheriting from Animal
class Dog(Animal):
def __init__(self, breed):
# Call the constructor of the base class (Animal)
super().__init__("Dog")
self.breed = breed
def make_sound(self):
print("Bark")
# Create objects of the classes
animal = Animal("Unknown")
dog = Dog("Labrador")
# Access attributes and methods
print(animal.species) # Output: "Unknown"
print(dog.species) # Output: "Dog"
print(dog.breed) # Output: "Labrador"
animal.make_sound() # Output: "Unknown sound"
dog.make_sound() # Output: "Bark"
In this example, we have a base class Animal
with a constructor that sets the species
attribute and a method make_sound()
that prints an unknown sound. We then create a subclass Dog
that inherits from Animal
. The Dog
class extends the constructor to set the species
attribute to "Dog" and overrides the make_sound()
method to print "Bark" for a dog's sound.
Polymorphism in Object-Oriented Programming (OOP):
Polymorphism is another important concept in OOP that allows objects of different classes to be treated as objects of a common superclass. It enables a single function or method to work with different types of objects, providing flexibility and extensibility to the code.
Polymorphism is often achieved through method overriding, where a subclass provides a specific implementation of a method defined in its superclass. Python supports polymorphism naturally since it is a dynamically-typed language.
Example: Polymorphism in Python
# Base class
class Shape:
def area(self):
return 0
# Subclasses inheriting from Shape
class Square(Shape):
def __init__(self, side):
self.side = side
def area(self):
return self.side * self.side
class Circle(Shape):
def __init__(self, radius):
self.radius = radius
def area(self):
return 3.14 * self.radius * self.radius
# Create objects of the classes
square = Square(5)
circle = Circle(3)
# Call the area() method for different objects
print("Area of square:", square.area()) # Output: 25
print("Area of circle:", circle.area()) # Output: 28.26
In this example, we have a base class Shape
with a method area()
that returns 0. We then create two subclasses, Square
and Circle
, that inherit from Shape
and override the area()
method with specific implementations for calculating the area of a square and a circle.
By calling the area()
method for different objects (square
and circle
), we demonstrate polymorphism as the same method name is used for different objects, but each object's specific implementation of the method is executed accordingly.
Going Further with Object-Oriented Programming (OOP):
Object-Oriented Programming (OOP) is a vast and powerful paradigm that goes beyond the basic concepts of classes, objects, inheritance, encapsulation, and polymorphism. As you delve deeper into OOP, you'll encounter additional advanced concepts that can further enhance your code design and development capabilities.
1. Abstraction: Abstraction is the process of simplifying complex systems by focusing only on the essential features while hiding unnecessary details. In OOP, abstraction is achieved through abstract classes and interfaces. Abstract classes are classes that cannot be instantiated and may contain abstract methods that must be implemented by their subclasses. Interfaces define a contract that classes must adhere to, specifying the methods they need to implement.
2. Composition: Composition is a design principle that allows you to create more complex objects by combining simpler objects. It enables you to build complex classes by aggregating multiple objects as attributes. Composition promotes a "has-a" relationship, where one class contains another class as a component.
3. Encapsulation and Access Modifiers: Encapsulation not only involves bundling data and methods within a class but also controlling access to them. Access modifiers in OOP (e.g., public, private, protected) define the scope and visibility of class members. By using access modifiers, you can enforce data hiding and ensure that certain class members are only accessible within the class itself or its subclasses.
4. Static and Class Methods: Static methods and class methods are methods that are bound to the class rather than an instance of the class. Static methods do not require access to the instance and are usually used for utility functions. Class methods, on the other hand, take the class itself as the first parameter and are commonly used for alternative constructors or to modify class-level attributes.
5. Method Overloading and Operator Overloading: Method overloading allows a class to have multiple methods with the same name but different parameters. Python doesn't support traditional method overloading, but you can achieve similar functionality using default arguments or variable-length argument lists. Operator overloading allows you to define special behaviors for operators (+, -, *, /, etc.) when used with objects of your custom classes.
6. Design Patterns: Design patterns are reusable solutions to common software design problems. In OOP, design patterns help you organize your code and address specific challenges in a structured and efficient manner. Popular design patterns include Singleton, Factory, Observer, Decorator, and more.
Going further in Python
Official Documentation:
To dive even deeper into Python, you should explore the official documentation, which serves as a comprehensive and reliable resource. The Python official documentation provides detailed information about the language, its standard library, and additional modules. You can find the documentation at https://docs.python.org/.
Online Courses on Python:
Learning Python online has become extremely accessible through a multitude of interactive courses and learning platforms. Whether you are a beginner or already experienced in programming, online courses offer you a flexible and convenient opportunity to improve your Python skills. Here are some of the leading platforms offering Python courses:
Coursera: Coursera offers Python courses at different levels, taught by prestigious universities. You can learn the basics of the language as well as advanced topics like data science and machine learning.
Udemy: Udemy provides a vast selection of Python courses, ranging from beginner-level to advanced courses covering specific domains such as web development, data analysis, etc.
edX: edX offers university-level Python courses delivered by renowned institutions. You can take free courses or opt for a paid certification.
Codecademy: Codecademy offers interactive tutorials that allow you to code directly in the browser. Their Python courses are tailored to beginners and will teach you the fundamentals of the language.
Participation in Open-Source Projects:
An excellent way to enhance your Python skills is by contributing to open-source projects. By participating in collaborative projects, you can work with other experienced developers, learn new techniques, and develop meaningful projects. Here are some tips to get started:
Search for open-source projects on platforms like GitHub. Look for projects that interest you and match your skill level.
Explore open issues in the project and see if you can contribute by fixing bugs or adding new features.
Be active in the open-source community, ask questions, share your ideas, and collaborate with other developers.
Make sure to understand the rules and guidelines of the project before contributing.
Python Communities:
Being part of the Python community is an excellent way to learn, share knowledge, and connect with other Python enthusiasts. Here are some ways to join the Python community:
Forums and Discussion Groups: Forums like Stack Overflow and Reddit r/learnpython are excellent resources to ask questions and get answers from the Python community.
Local Meetups and Conferences: Look for Python User Groups in your area to participate in local meetups. Additionally, Python conferences like PyCon are events where you can meet Python developers from around the world and attend interesting presentations.
Books on Python:
Books are a valuable resource for in-depth learning of Python and gaining a comprehensive understanding of the language. Here are some recommended Python books:
Selection of Books for Beginners:
"Automate the Boring Stuff with Python" by Al Sweigart: This book is ideal for beginners who want to learn Python by automating common tasks.
"Python Crash Course" by Eric Matthes: This book covers the basics of Python and includes practical projects to reinforce your skills.
Books on Advanced Python:
"Fluent Python" by Luciano Ramalho: This book is aimed at intermediate and advanced Python programmers who want to deepen their understanding of the language and its advanced features.
"Python Cookbook" by David Beazley and Brian K. Jones: This book presents practical recipes to solve common problems in Python and explores advanced topics.
Using GitHub: Uploading Your Python Files
Once you have saved your Python files with the ".py" extension, you can upload them to a GitHub repository to maintain a version-controlled and accessible copy for other developers. Follow these simple steps:
Create a repository on GitHub:
Go to GitHub and log in to your account.
Click on the "New" button to create a new repository.
Give your repository a name, add a description (optional), and choose visibility and initialization options (with a README file, license, etc.).
Click on "Create repository" to set up your repository.
Upload via the terminal (Git Bash):
Open Git Bash on your computer.
Navigate to the root folder of your project using the
cd
(Change Directory) command. For example:cd /path/to/your_project
.Initialize a local Git repository using the
git init
command. This creates a ".git" subfolder containing versioning information for your project.Link your local repository to the remote (GitHub) repository using the
git remote add
command. For example:git remote add origin
https://github.com/your_user/your_repository.git
.Add your files to the staging area using the
git add
command. For example:git add
file1.py
file2.py
.Commit your files using the
git commit
command. For example:git commit -m "Add my Python files"
.Upload your files to GitHub using the
git push
command. For example:git push origin master
.You may be prompted to enter your GitHub credentials to authorize the upload.
Conclusion
Python, as an open-source programming language, captivates developers with its simplicity, readability, and extensive capabilities. Throughout this journey, we've explored the fundamentals of Python, starting from basic syntax, data types, and control structures. We've delved into more advanced concepts like functions, object-oriented programming, and the power of Python libraries and modules. Understanding how to work with lists, manipulate data, and harness the potential of external libraries like NumPy and Googletrans broadens our programming horizons.
Moreover, we've witnessed the importance of version control through GitHub, allowing developers to collaborate, share, and maintain their projects efficiently. Utilizing online courses, contributing to open-source projects, and diving into Python books equips developers with continuous growth and enhances their creativity in software development. Python's versatility and the supportive community foster an environment where developers of all levels can embark on an ever-evolving coding adventure. Armed with this knowledge, we wish you happy coding and an exciting journey exploring the endless possibilities of Python!
References
The official python documentation: https://docs.python.org/fr/3/
Apprenez à programmer en Python de Vincent Le Goff :https://www.eyrolles.com/Informatique/Livre/apprenez-a-nbsp-programmer-en-python-9782416006555/
Learn Python 3 the Hard Way :https://www.amazon.com/Learn-Python-Hard-Way-Introduction/dp/0134692888
Python tutorial : https://zestedesavoir.com/tutoriels/2514/un-zeste-de-python/
Apprenez les bases du langage Python - OpenClassrooms : https://openclassrooms.com/fr/courses/7168871-apprenez-les-bases-du-langage-python
La meilleure façon d’apprendre Python 2023 (tutoriels Python … - Kinsta : https://kinsta.com/fr/blog/facon-d-apprendre-python/
Python tutorial : https://www.guru99.com/python-tutorials.html
Subscribe to my newsletter
Read articles from Fabrice Sangwa directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Fabrice Sangwa
Fabrice Sangwa
Whatever you want to do, do it now but it may take you some time but don't wait until you are ready to do it, start first. I'm Python Developper.