iOS Interview QnA: Result Type in Swift
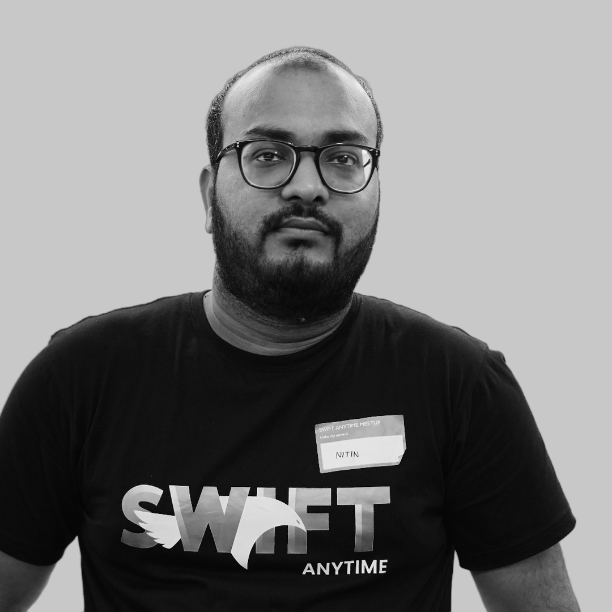
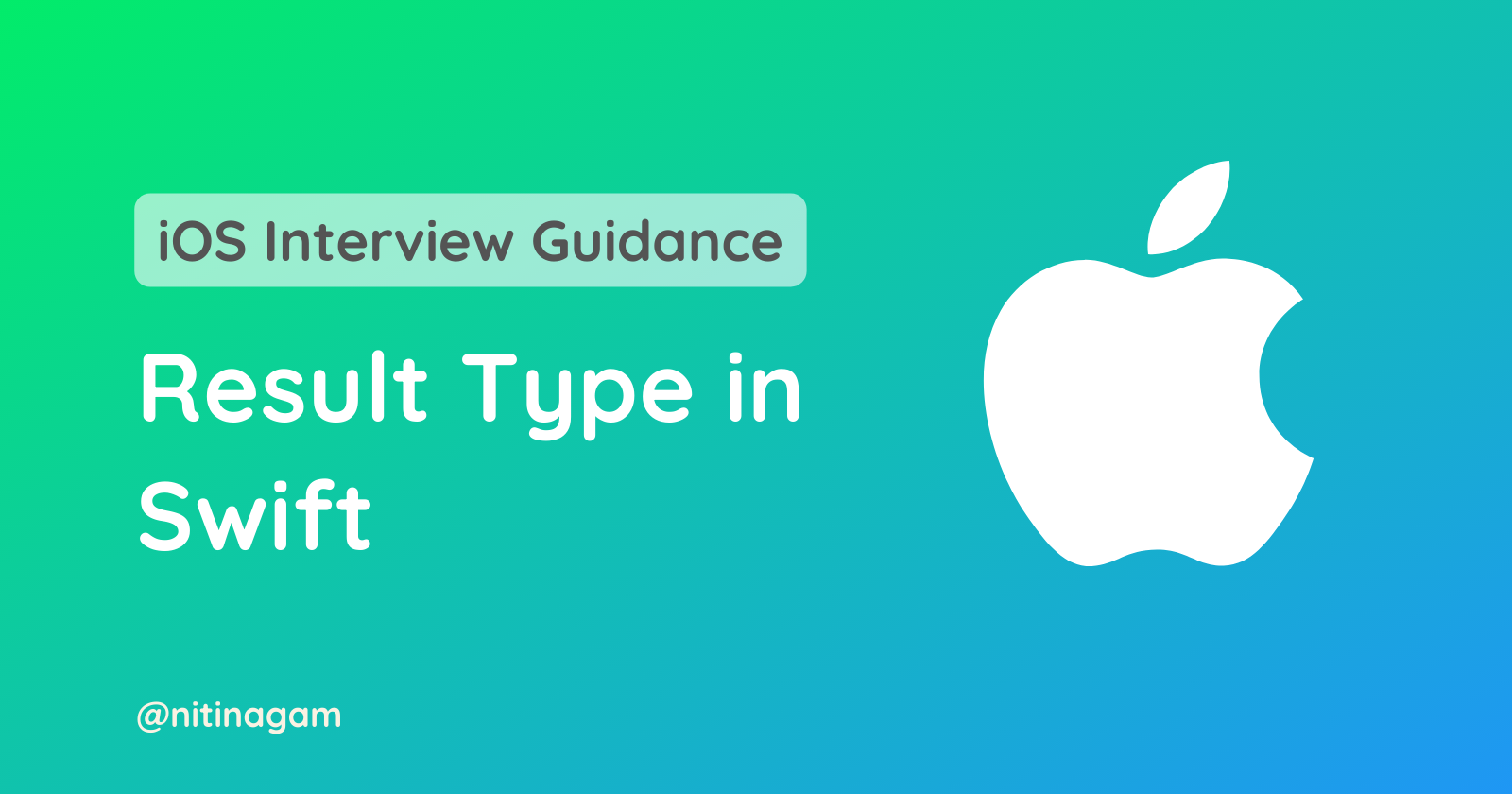
Level: Easy
How would you use the Result type to manage network requests? Why is the Result type effective for implementation?
The Result type is a powerful addition introduced in Swift 5 to handle asynchronous results. It can succeed with a value or fail with an error. It is particularly useful in scenarios where you want to handle success and failure cases explicitly without throwing errors or using optional types.
When performing asynchronous tasks, such as network requests or database queries, the Result type can be used to represent the success or failure of the operation.
Example of a Result type in a network request:
enum NetworkError: Error {
case noInternet
case serverError
}
func fetchData(completion: (Result<String, NetworkError>) -> Void) {
// Create an asynchronous network request here
let hasInternet = true // use it accordingly
if hasInternet {
completion(.success("send received data here..."))
} else {
completion(.failure(.noInternet))
}
// send your network request here...
}
How to use the above function?
fetchData { result in
switch result {
case .success(let data):
print(data)
case .failure(let error):
print("Error: \(error)")
}
}
In the example above, the fetchData
function uses the Result
type to represent the success or failure of a network request.
Using Result
enhances the readability, safety, and maintainability of your Swift code, especially in situations where multiple outcomes need to be considered.
Many operations can fail for various reasons and Result
can handle multiple error cases effectively. By using an enum to define different error types, developers can handle each failure case separately, making error management more robust and maintainable.
Subscribe to my newsletter
Read articles from Nitin Aggarwal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
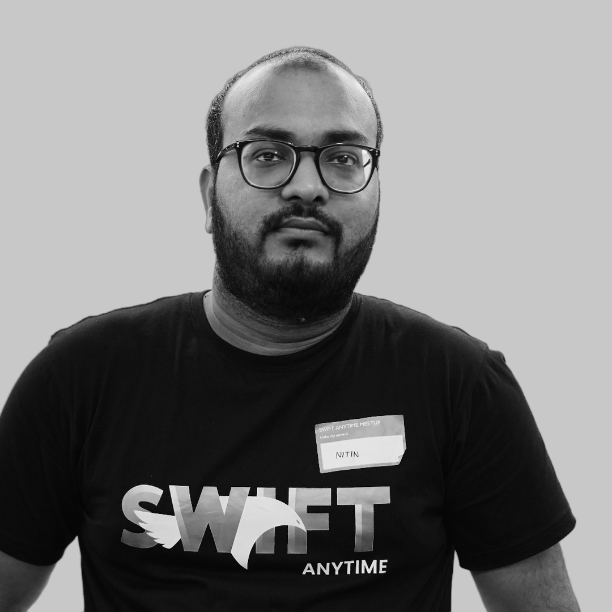
Nitin Aggarwal
Nitin Aggarwal
I’m a Lead iOS Engineer at Dainik Bhaskar. I believe in trying something new rather than sticking with what I already know since innovation is the key here. I’m passionate about developing readable, clean, maintainable source code and delivering quality iOS apps. In order to build a strong iOS community, I enjoy writing technical stuff.