Variadic Functions in C: Unleashing the Full Potential of Your Code
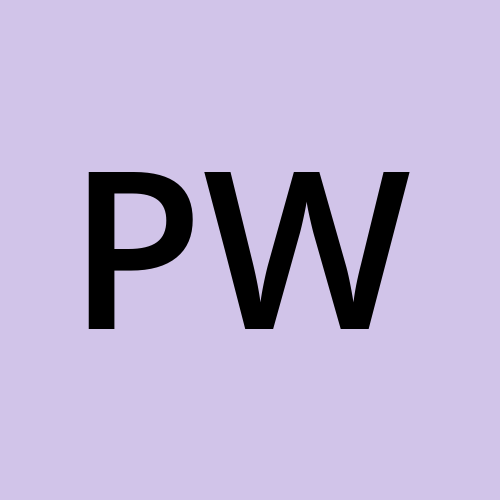
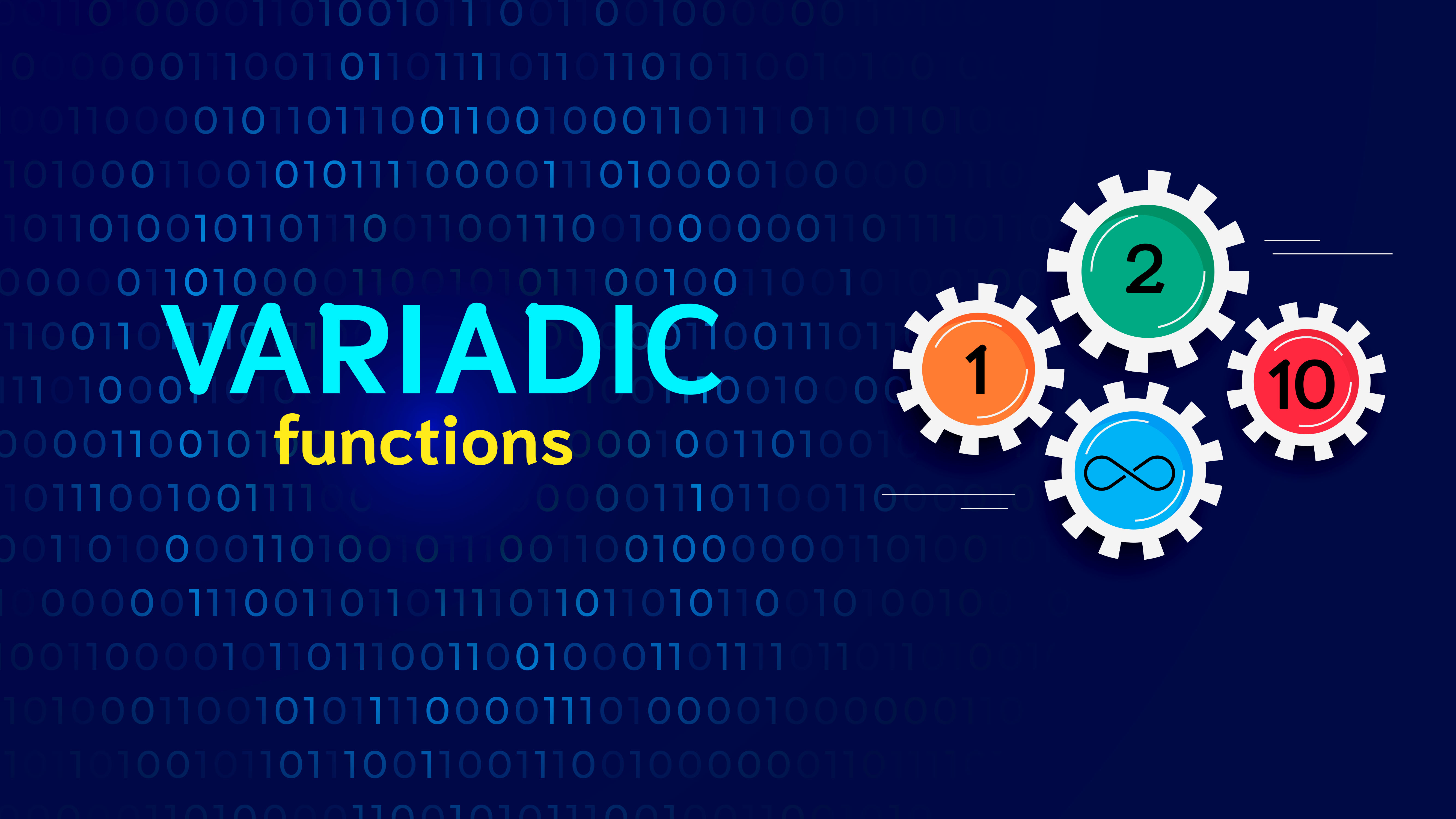
For those starting out in C, you will have discovered that most functions have a fixed number of arguments, limiting their flexibility. For instance, consider this simple example:
int sum(int a, int b)
{
return (a + b);
}
This function takes exactly two arguments, making it inflexible for scenarios where the number of arguments may vary. If we need to sum up the marks of students in a class, the fixed argument approach becomes impractical when the number of students changes frequently.
Enter variadic functions, a powerful feature in C that allows functions to accept an indefinite number of arguments, providing more flexibility and versatility. Today, we'll explore what variadic functions are and how to work with them.
A variadic function is a function that can take an arbitrary number of arguments. It proves useful in situations where the number of arguments is unknown, or even when the argument types vary. An iconic example of a variadic function is the printf
function, which outputs formatted text.
Before delving further, we must familiarize ourselves with the <stdarg.h>
header file. This header allows a function to become variadic by facilitating the handling of an indefinite number of arguments. It introduces the special data type va_list
and several macros that assist in handling the argument list received by the function.
Here is the general syntax for defining a variadic function with at least one known argument, followed by an ellipsis (3 dots ...
which represents the variable arguments):
int function_name(data_type variable_name, ...);
The va_list
data type, is defined in the stdarg.h
header and is used to declare a variable that holds information about the variable argument list used in variadic functions. Think of this variable as a pointer to the different arguments supplied to the variadic function. It needs to be declared before working with the argument list.
This section outlines the essential macros for handling variadic functions, and we'll follow up with an illustrative example:
va_start
: It takes two arguments: ava_list
object and a reference to the last known argument (the argument just before the ellipsis). This macro ensures that theva_list
object points to the first argument in the list of arguments.va_arg
: This macro helps access the next variable/argument in the list. It takes two arguments: theva_list
object and a data type descriptor.va_end
: This macro performs clean-up operations. It takes one argument: theva_list
object.
Now, consider a function that creates arrays. It would be ideal for the function to be flexible enough to take different numbers of variables at different times and therefore a situation that calls for a variadic function.
#include <stdio.h>
#include <stdlib.h>
#include <stdarg.h>
// Function to allocate an array and store variable arguments in it
void allocateArray(int numValues, ...)
{
va_list ap; // Declare a variable of type va_list to handle variable arguments
va_start(ap, numValues); // Initialize the va_list variable using va_start
int* array = (int*)malloc(numValues * sizeof(int)); // Dynamically allocate memory for the array
if (array == NULL)
{
printf("Memory allocation failed.\n");
return;
}
// Loop through the variable arguments and store them in the allocated array
for (int i = 0; i < numValues; i++)
{
int value = va_arg(ap, int); // Retrieve the next argument from the va_list using va_arg
array[i] = value;
}
va_end(ap); // Clean up the va_list after using it with va_end
// Print the allocated array
printf("Allocated Array: ");
for (int i = 0; i < numValues; i++)
{
printf("%d ", array[i]);
}
printf("\n");
free(array); // Remember to free the allocated memory to avoid memory leaks
}
int main()
{
// Call the allocateArray function with different numbers of arguments
allocateArray(4, 1, 2, 3, 4); // Allocating an array of size 4 with values 1, 2, 3, 4
allocateArray(3, 10, 20, 30); // Allocating an array of size 3 with values 10, 20, 30
allocateArray(3, 1, 2, 11, 8, 6); // Allocating an array of size 5 with values 1, 2, 11, 8, 6
return 0;
}
The allocateArray
function above demonstrates how to create a flexible function that accepts different numbers of arguments using variadic functions.
Now that you have grasped the concept of variadic functions and their essential components, you can add this powerful tool to your C programming arsenal. It enables you to write more adaptable and versatile functions, promoting cleaner and more readable code. Happy coding!
Subscribe to my newsletter
Read articles from Peter Wisdom directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
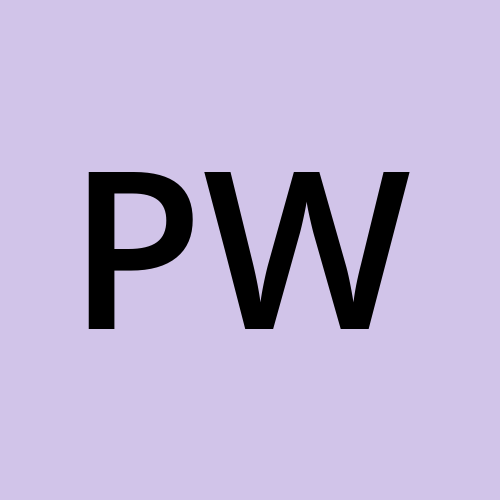
Peter Wisdom
Peter Wisdom
Software Engineering Student at ALX... Love building the imagination, making it tangible! Love learning and sharing the knowledge