Create a simple fileserver in Golang

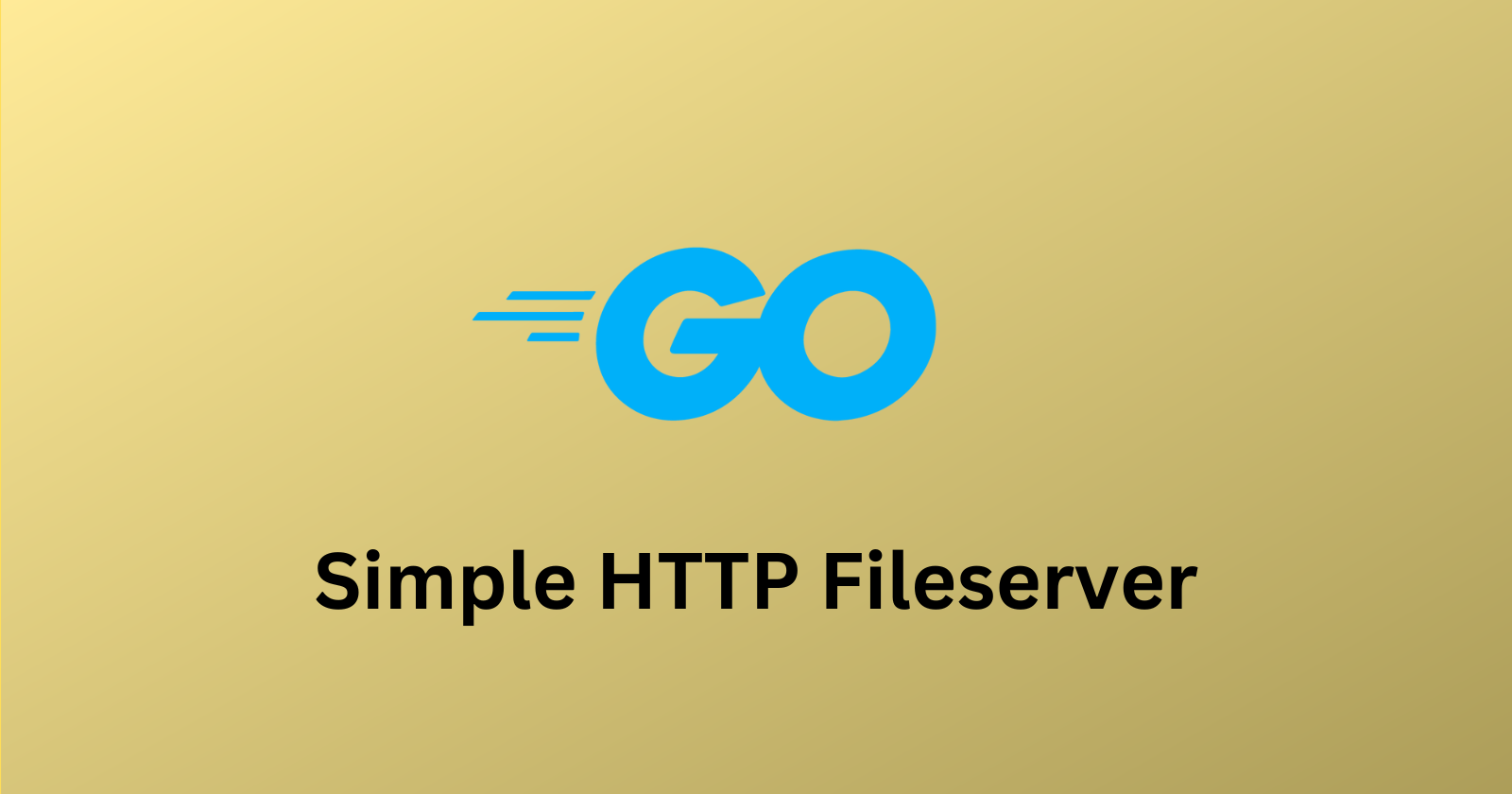
In modern software development, there are often scenarios where you need to share files or directories over the internet quickly and securely. Whether it's for collaborating with team members, sharing assets, or providing a simple file-sharing solution, having a quick way to expose a local directory through an HTTP server can be incredibly useful. In this blog post, we'll explore a concise and straightforward Go code snippet that allows you to achieve just that.
package main
import (
"fmt"
"net/http"
"os"
)
func main() {
// Replace "." with the actual path of the directory you want to expose.
directoryPath := "."
// Check if the directory exists
_, err := os.Stat(directoryPath)
if os.IsNotExist(err) {
fmt.Printf("Directory '%s' not found.\n", directoryPath)
return
}
// Create a file server handler to serve the directory's contents
fileServer := http.FileServer(http.Dir(directoryPath))
// Create a new HTTP server and handle requests
http.Handle("/", fileServer)
// Start the server on port 8080
port := 8080
fmt.Printf("Server started at http://localhost:%d\n", port)
err = http.ListenAndServe(fmt.Sprintf(":%d", port), nil)
if err != nil {
fmt.Printf("Error starting server: %s\n", err)
}
}
Understanding the code
Package and Imports: The code begins with the standard Go package declaration (
package main
) and the necessary imports. It utilizes the"fmt"
,"net/http"
, and"os"
packages.Setting the Directory Path: The variable
directoryPath
stores the path of the local directory that you want to expose. In this example, it is set to"."
, which represents the current working directory. You can change this to the desired directory path on your system.Checking Directory Existence: Before proceeding, the code checks if the specified directory exists. It does this by using
os.Stat()
which returns an error if the directory does not exist. The functionos.IsNotExist()
is then used to handle this specific error condition. If the directory doesn't exist, the code will print an error message and terminate.Creating the File Server: Assuming the directory exists, the code creates an HTTP file server handler using
http.FileServer(http.Dir(directoryPath))
. This file server will serve the contents of the specified directory.Handling Requests: The code then sets up a new HTTP server using
http.Handle("/", fileServer)
. This means that any incoming HTTP requests to the server will be handled by the file server created in the previous step.Starting the Server: The server is started on port 8080 by calling
http.ListenAndServe(fmt.Sprintf(":%d", port), nil)
. If the server encounters any errors during startup, they will be printed out.
Running the code
To run this code on your machine, follow these steps:
Save the code to a file with a ".go" extension (e.g.,
main.go
).Open your terminal or command prompt and navigate to the directory containing the file.
Compile and run the code by entering the command:
go run main.go
.
Once the server is up and running, you can access the contents of the specified directory by visiting http://localhost:8080
in your web browser.
Conclusion
In this blog post, we explored a simple yet powerful Go code snippet that allows you to expose a local directory through an HTTP server. This can be immensely useful for sharing files, collaborating with team members, or even setting up a basic file-sharing solution. By understanding this code, you've taken a step towards harnessing the potential of Go for handling web-related tasks efficiently. Remember to ensure the directory you want to expose exists and to keep security considerations in mind when making files accessible over the internet.
Subscribe to my newsletter
Read articles from Sidharthan Chandrasekaran Kamaraj directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Sidharthan Chandrasekaran Kamaraj
Sidharthan Chandrasekaran Kamaraj
Yet another developer, learning new things everyday :)