Common mistakes to avoid in implementing printf
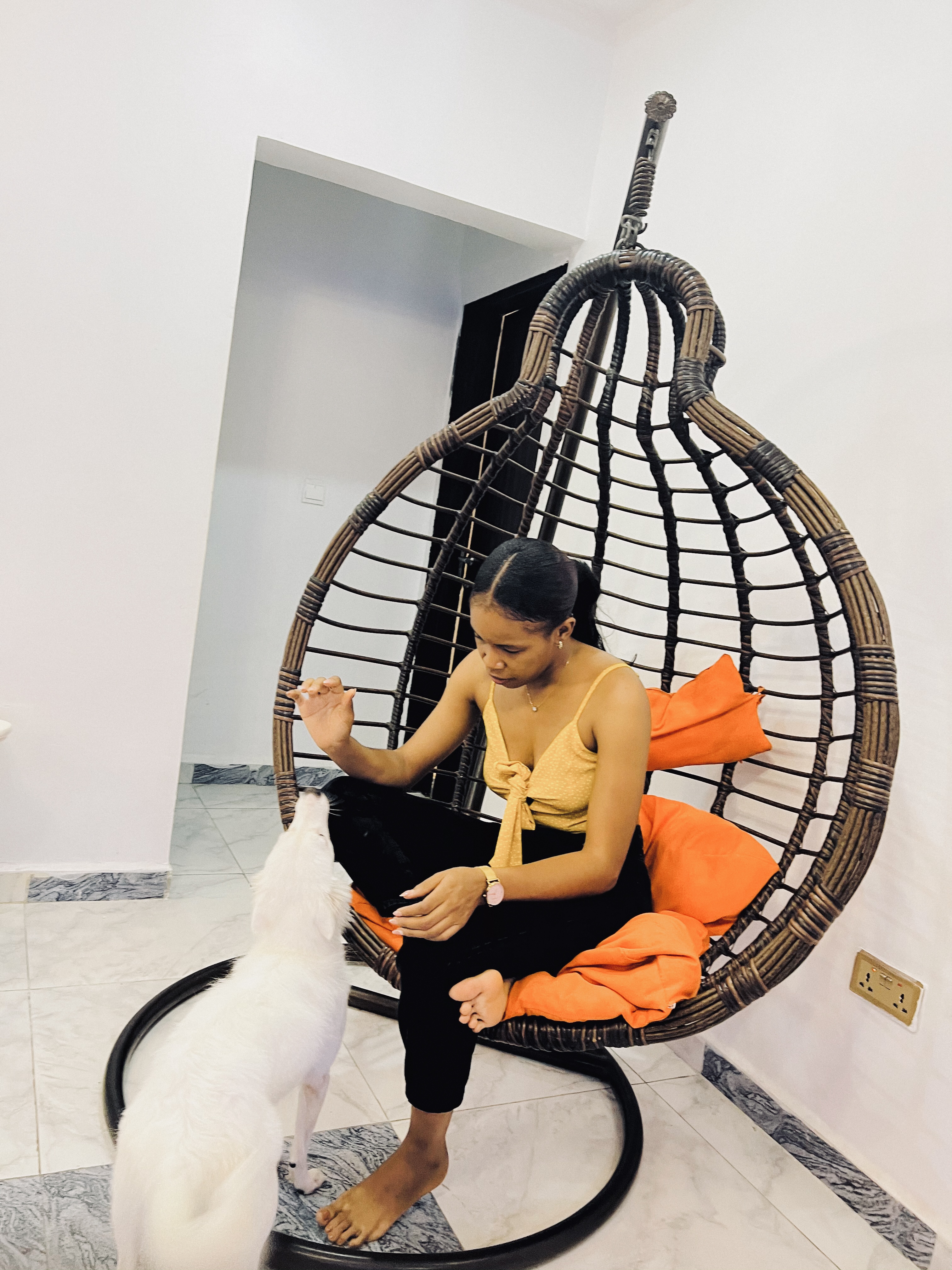
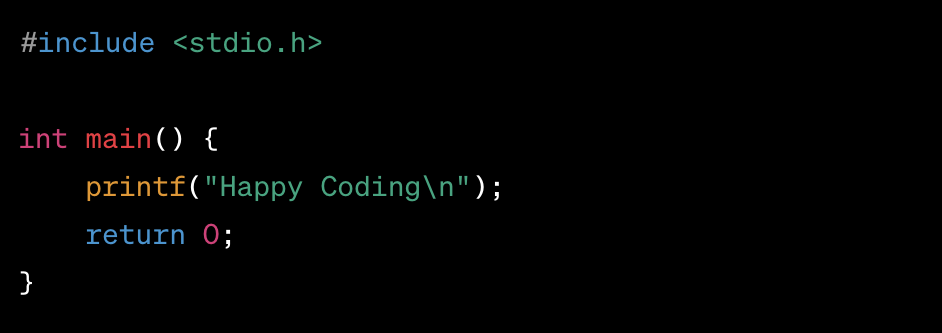
When implementing printf
there are several common mistakes we should avoid to ensure the correct behavior and prevent potential issues.
Incorrect format specifiers: Ensure that the format specifiers used in the
printf
function match the corresponding data types of the arguments. Mismatched specifiers can lead to undefined behavior or output errors.int num = 42; myPrintf("The answer is %f", num); // Incorrect format specifier for an integer // Output: The answer is -1222399568.000000
Missing or extra arguments: Always provide the required number of arguments for each format specifier in the
printf
function. Missing or extra arguments can cause memory corruption and unexpected results.int x = 10, y = 20, z = 30; myPrintf("The values are %d, %d, %d\n", x, y); // Missing the third argument 'z' // Output: The values are 10, 20, 0
Undefined behavior due to incorrect data types: Be mindful of the data types being passed as arguments. For example, passing an incompatible data type for a format specifier (e.g., passing an integer when
%f
expects a floating-point number) can result in undefined behavior.char ch = 'A'; myPrintf("The character is %d\n", ch); // Incorrect format specifier for a char // Output: The character is 65
Buffer overflows: Be cautious about using
%s
format specifiers with user-provided strings. Always ensure that the destination buffer has enough space to hold the entire string, including the null-terminator. Otherwise, it can lead to buffer overflows and security vulnerabilities.char buffer[10]; char* longString = "This is a very long string"; myPrintf("Short string: %s\n", longString); // Buffer overflow! // Output: Short string: This is a v // (Incomplete output due to buffer overflow)
Incorrect handling of variable argument lists: When implementing variadic functions like
printf
, pay special attention to how you extract and use the variable argument list (usingva_list
,va_start
,va_arg
, andva_end
). Mishandling the variable arguments can lead to undefined behavior.void myPrintfWithMistake(const char* format, ...) { va_list args; va_start(args, format); // Incorrect way to iterate over the variable arguments while (args != NULL) { int arg = va_arg(args, int); printf("%d ", arg); } va_end(args); } // This will lead to undefined behavior as there's no way to know when to stop the loop.
Lack of error handling: Consider adding appropriate error handling to handle exceptional cases, such as when the format string is invalid or when there is an issue with writing the output.
Platform-specific behavior: The behavior of
printf
can vary between different platforms and compilers. Avoid making assumptions about the behavior and ensure that your implementation is compatible with the target environment.Recursive
printf
calls: Avoid callingprintf
or any formatted output functions within the sameprintf
statement. This can lead to unexpected results, infinite recursion, or stack overflow.
Remember that overcoming challenges is a journey, and it's okay to encounter difficulties along the way. Stay patient, committed, and open to learning, and you'll find yourself better equipped to conquer any challenge that comes your way.
Happy Coding!
Resources
Subscribe to my newsletter
Read articles from Peace Chinagwam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
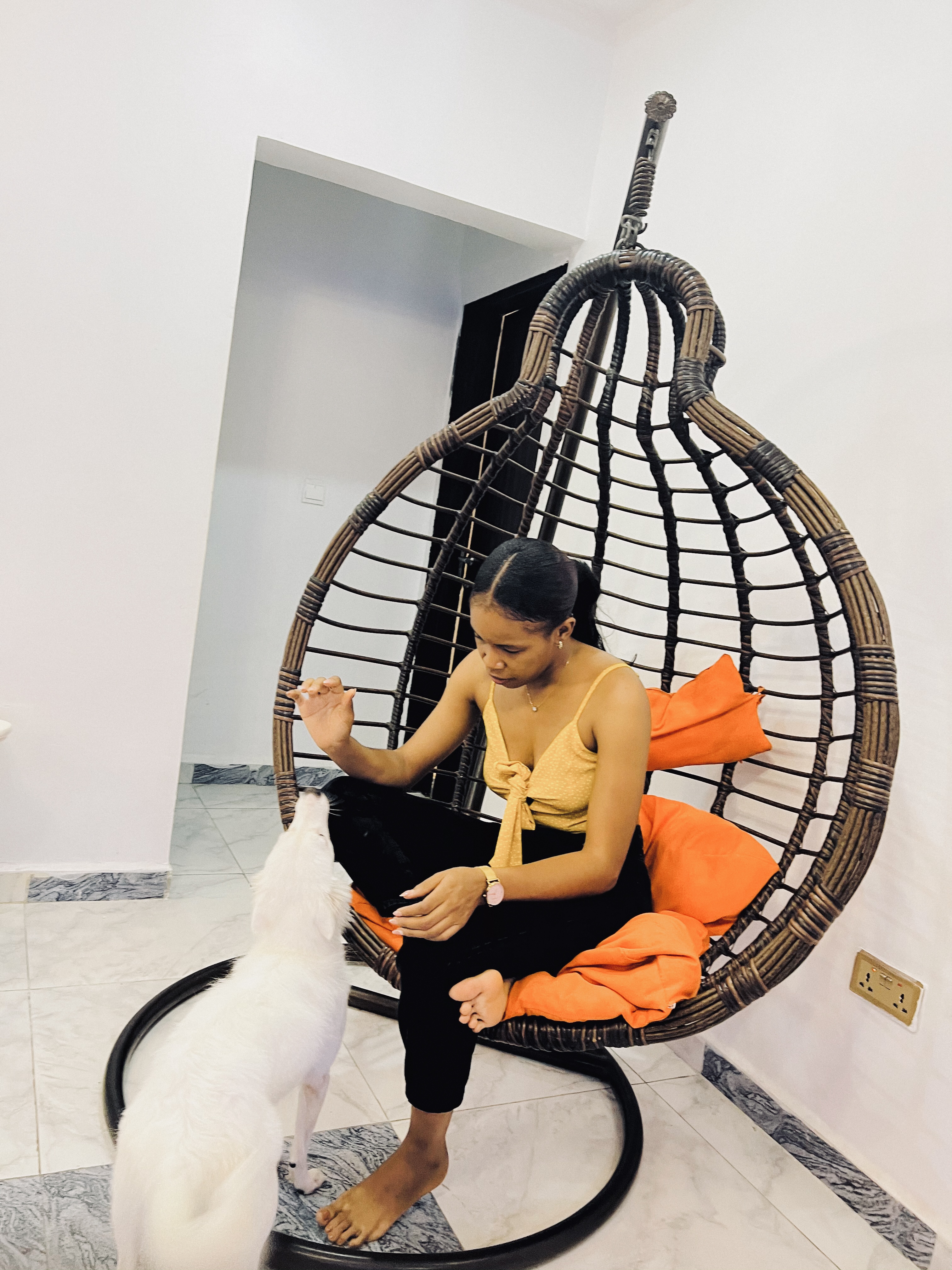
Peace Chinagwam
Peace Chinagwam
I am a front-end developer and technical writer who loves creating user-friendly designs and explaining tricky things in simple words.