Named Parameters in JavaScript
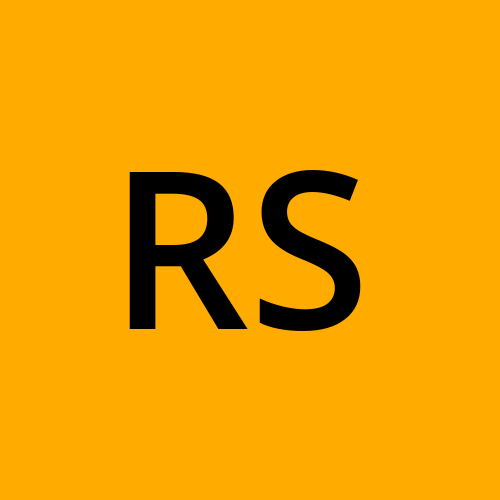
What is Named Parameters
Named means that when you call a function, you attach the argument to a label. Named arguments enable you to specify an argument for a parameter by matching the argument with its name rather than with its position in the parameter list. So you can pass an argument to Function in any order irrespective of its position.
Named parameters are supported explicitly in many languages like Python, Dart, CSharp, Ruby, Scala, and Kotlin. JavaScript, by default, does not support Named Parameters. However, you can do something similar using object literals and destructuring.
Object Literals and Destructuring
The destructuring assignment is a cool feature that came along with JavaScript ES6. It makes it possible to unpack values from arrays, or properties from objects, into distinct variables. Here We are unpacking variables a & b from the object a.
let obj = { a: 1, b: 2, c: 3 }
let { a, b } = obj
console.log(a, b)
Named Parameter in Javascript
Using Object literals and destructuring we can implement Named Parameters in Javascript. You can avoid errors when calling the function without any arguments by assigning the object to the empty object, {}
, even if you have default values set up.
const placeOrder = ({
productId = 'defaultId',
productName = 'defaultProductName',
sellerName = 'defaultSellerName'
} = {}) => {
console.log("Order Placed ->", productId, productName, sellerName);
}
placeOrder({
productId: "1",
productName: "computer",
sellerName: "Amazon"
})
// Order Placed -> 1 computer Amazon
placeOrder({
productId: "2",
productName: "mobile"
})
// Order Placed -> 2 mobile defaultSellerName
placeOrder()
// Order Placed -> defaultId defaultProductName defaultSellerName
Named parameters, by default, are optional. But you can easily make them required by validating parameters before executing the function.
Hope this helps! Let me know if you have any other questions.
Resources
Subscribe to my newsletter
Read articles from Rohit Saw directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
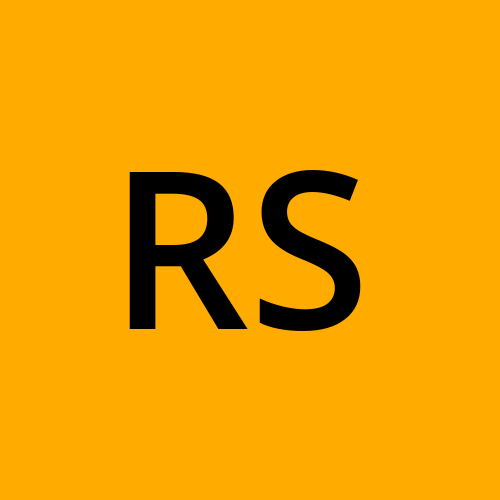
Rohit Saw
Rohit Saw
FullStack Developer