Javascript Multiple Choice Questions
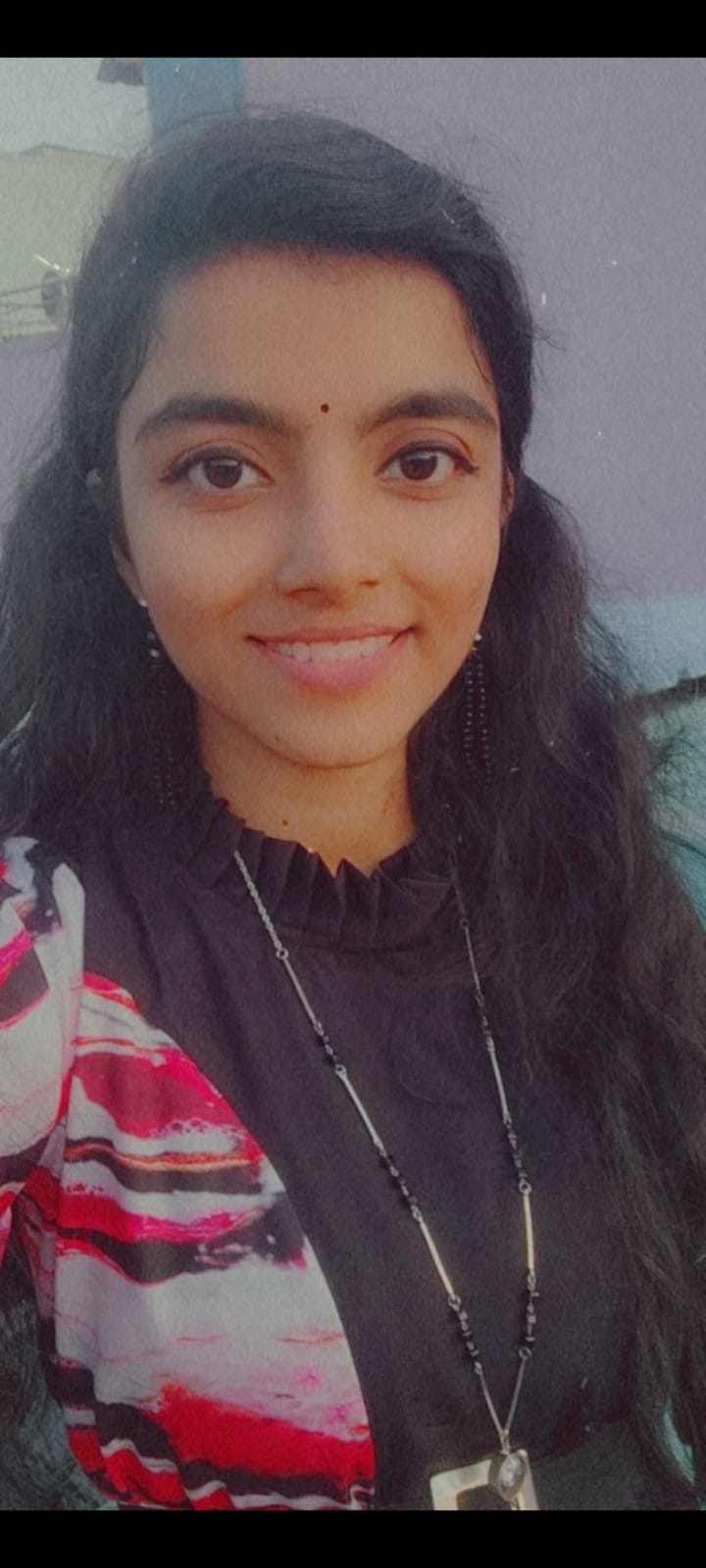
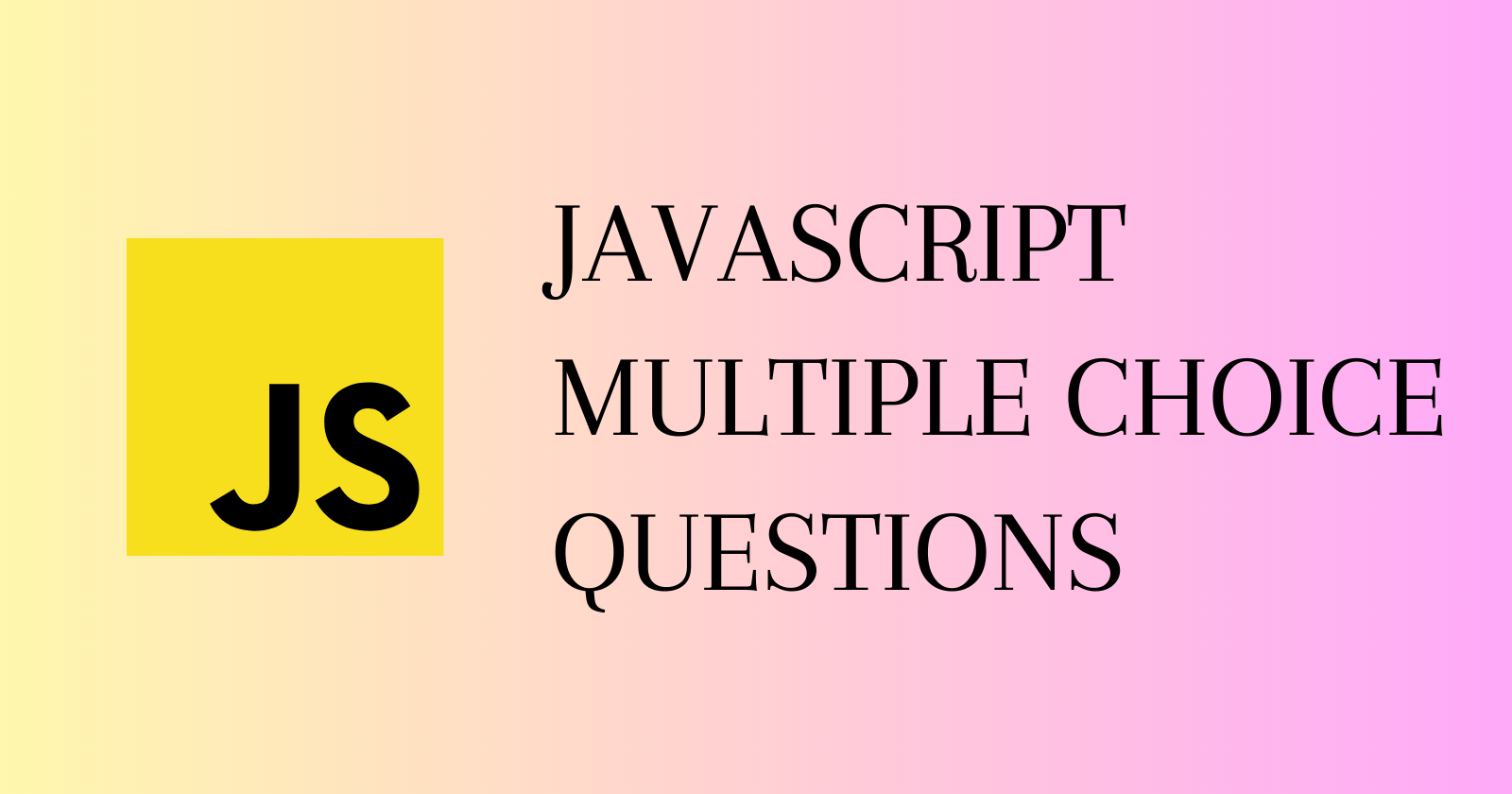
Which of the following is NOT a valid data type in JavaScript? a) Number b) String c) Boolean d) Character
Answer: d) Character
What will be the output of the following code?
var x = 5; console.log(x++);
a) 5 b) 6 c) 4 d) Error Answer: a) 5
What is the purpose of the
use strict
directive in JavaScript? a) It enables strict mode for the script. b) It allows using deprecated features. c) It is used to define global variables. d) It is a syntax error in JavaScript. Answer: a) It enables strict mode for the script.What is the difference between
==
and===
in JavaScript? a) Both are the same. b)==
checks value and type,===
checks only value. c)==
checks only value,===
checks value and type. d)==
is for strings,===
is for numbers. Answer: c)==
checks only value,===
checks value and type.What is the output of the following code?
var x = 10 + "5"; console.log(x);
a) 15 b) "105" c) 105 d) Error Answer: b) "105"
Which method is used to add elements to the end of an array in JavaScript? a) push() b) add() c) append() d) insert() Answer: a) push()
What does the
NaN
value represent in JavaScript? a) Not a Number b) Null and Not Defined c) Not Applicable Now d) None of the above Answer: a) Not a NumberWhat is the purpose of the
bind()
method in JavaScript? a) To concatenate two strings b) To create a new array c) To attach an event handler function d) To set the value ofthis
in a function Answer: d) To set the value ofthis
in a functionWhat will be the result of the following code?
var x = 5; var y = "10"; console.log(x + y);
a) 15 b) 510 c) "510" d) Error Answer: c) "510"
How do you check if a variable is an array in JavaScript? a) Using
typeof
b) UsingisArray()
c) Usingtype()
d) UsingisTypeOf()
Answer: b) UsingisArray()
What is the output of the following code?
var x = 10; function foo() { console.log(x); var x = 20; } foo();
a) 10 b) 20 c) undefined d) Error Answer: c) undefined
Which of the following is a correct way to create an object in JavaScript? a)
var obj = object();
b)var obj = {}
c)var obj = new Object();
d) All of the above Answer: d) All of the aboveWhat does the
this
keyword refer to in JavaScript? a) It refers to the current function's scope. b) It refers to the parent object. c) It refers to the global object. d) It depends on the context of its use. Answer: d) It depends on the context of its use.What is the output of the following code?
console.log(2 + "2" - 2);
a) 2 b) 22 c) 4 d) Error Answer: c) 4
Which of the following is NOT a valid way to create a function in JavaScript? a)
function myFunction() {}
b)var myFunction = function() {};
c)var myFunction = () => {};
d)var myFunction = => {}
Answer: d)var myFunction = => {}
What is the purpose of the
eval()
function in JavaScript? a) To evaluate regular expressions b) To execute code in a string c) To evaluate mathematical expressions d) To evaluate object properties Answer: b) To execute code in a stringWhat will be the result of the following code?
var x = 0; function foo() { console.log(x); var x = 1; } foo();
a) 0 b) 1 c) undefined d) Error Answer: c) undefined
How do you remove the last element from an array in JavaScript? a)
array.removeLast()
b)array.pop()
c)array.deleteLast()
d)array.splice(-1)
Answer: b)array.pop()
What does the
Array.prototype.map
()
method do in JavaScript? a) Adds an element to the end of the array b) Creates a new array by transforming each element of the original array c) Filters the array based on a condition d) Reverses the order of elements in the array Answer: b) Creates a new array by transforming each element of the original arrayWhat is the purpose of the
setTimeout()
function in JavaScript? a) To delay the execution of a function b) To execute a function repeatedly c) To set an interval for a function d) To stop the execution of a function Answer: a) To delay the execution of a functionWhat is the result of the following code?
var a = [1, 2, 3]; var b = a; b.push(4); console.log(a);
a) [1, 2, 3] b) [1, 2, 3, 4] c) [4, 1, 2, 3] d) Error Answer: b) [1, 2, 3, 4]
What does the
Array.prototype.filter()
method do in JavaScript? a) Adds an element to the end of the array b) Creates a new array by transforming each element of the original array c) Filters the array based on a condition d) Reverses the order of elements in the array Answer: c) Filters the array based on a conditionHow do you check if an object has a specific property in JavaScript? a) Using
obj.hasProperty(prop)
b) Usingobj.prop
c) Usingobj.hasOwnProperty(prop)
d) Usingobj.contains(prop)
Answer: c) Usingobj.hasOwnProperty(prop)
What is the output of the following code?
var x = 0; function foo() { x = 1; } foo(); console.log(x);
a) 0 b) 1 c) undefined d) Error Answer: b) 1
What is the purpose of the
Array.prototype.reduce()
method in JavaScript? a) To add elements to the beginning of an array b) To create a new array by transforming each element of the original array c) To combine all elements of an array into a single value d) To sort the elements of an array Answer: c) To combine all elements of an array into a single valueWhat is the result of the following code?
console.log(typeof null);
a) "null" b) "undefined" c) "object" d) "string" Answer: c) "object"
What is the purpose of the
Array.prototype.forEach()
method in JavaScript? a) To create a new array by transforming each element of the original array b) To loop over each element of an array and execute a callback function c) To sort the elements of an array d) To filter the array based on a condition Answer: b) To loop over each element of an array and execute a callback functionWhat will be the output of the following code?
var x = 1; if (function foo() {}) { x += typeof foo; } console.log(x);
a) 1undefined b) 1function c) 1string d) Error Answer: a) 1undefined
How do you convert a string to a number in JavaScript? a)
parseInt()
b)Number()
c)toNumber()
d)convertNumber()
Answer: b)Number()
What does the
Array.prototype.slice()
method do in JavaScript? a) Removes elements from the beginning of an array b) Creates a shallow copy of an array from the specified start to end c) Adds elements to the beginning of an array d) Reverses the order of elements in the array Answer: b) Creates a shallow copy of an array from the specified start to endWhat is the output of the following code?
var x = 10; var y = "5"; console.log(x - y);
a) 5 b) 10 c) 15 d) "55" Answer: a) 5
What will be the result of the following code?
console.log(typeof typeof 1);
a) "number" b) "string" c) "object" d) "undefined" Answer: b) "string"
What is the purpose of the
Array.prototype.unshift()
method in JavaScript? a) To remove elements from the beginning of an array b) To create a new array by transforming each element of the original array c) To add elements to the beginning of an array d) To filter the array based on a condition Answer: c) To add elements to the beginning of an arrayWhat is the output of the following code?
var x = 1; function foo() { x = 10; return; function x() {} } foo(); console.log(x);
a) 1 b) 10 c) undefined d) Error Answer: a) 1
What does the
Array.prototype.join()
method do in JavaScript? a) Adds elements to the end of an array b) Creates a new array by transforming each element of the original array c) Joins all elements of an array into a string using a specified separator d) Reverses the order of elements in the array Answer: c) Joins all elements of an array into a string using a specified separatorHow do you convert a number to a string in JavaScript? a)
number.toString()
b)String(number)
c)number.toText()
d)convertToString(number)
Answer: b)String(number)
What is the output of the following code?
var x = 0; function foo() { x = 1; return x; } console.log(foo());
a) 0 b) 1 c) undefined d) Error Answer: b) 1
What is the purpose of the
Array.prototype.some()
method in JavaScript? a) To add elements to the end of an array b) To check if at least one element in the array satisfies a condition c) To create a new array by transforming each element of the original array d) To sort the elements of an array Answer: b) To check if at least one element in the array satisfies a conditionWhat will be the result of the following code?
var x = [1, 2, 3]; x[10] = 10; console.log(x.length);
a) 3 b) 4 c) 10 d) 11 Answer: d) 11
What is the purpose of the
Array.prototype.reverse()
method in JavaScript? a) Adds elements to the end of an array b) Creates a new array by transforming each element of the original array c) Reverses the order of elements in the array d) Joins all elements of an array into a string using a specified separator Answer: c) Reverses the order of elements in the arrayWhat is the output of the following code?
var x = 10; var y = 5; if (x > y) { var result = "x is greater than y"; } console.log(result);
a) "x is greater than y" b) 10 c) undefined d) Error Answer: a) "x is greater than y"
What will be the result of the following code?
var x = 5; console.log(x--);
a) 5 b) 4 c) 6 d) Error Answer: a) 5
What is the purpose of the `Array.prototype.find()` method in JavaScript? a) To check if at least one element in the array satisfies a condition b) To create a new array by transforming each element of the original array c) To find the index of a specific element in the array d) To find the first element in the array that satisfies a condition Answer: d) To find the first element in the array that satisfies a condition
What does the
Array.prototype.concat()
method do in JavaScript? a) Adds elements to the end of an array b) Creates a new array by transforming each element of the original array c) Joins all elements of an array into a string using a specified separator d) Combines two or more arrays Answer: d) Combines two or more arraysWhat is the output of the following code?
var x = [1, 2, 3]; x.length = 0; console.log(x);
a) [1, 2, 3] b) [] c) [null, null, null] d) Error Answer: b) []
What will be the result of the following code?
console.log("Hello" instanceof String);
a) true b) false c) Error d) undefined Answer: b) false
What is the purpose of the
Array.prototype.splice()
method in JavaScript? a) To create a new array by transforming each element of the original array b) To add elements to the end of an array c) To remove elements from an array and replace them with new elements d) To sort the elements of an array Answer: c) To remove elements from an array and replace them with new elementsWhat is the output of the following code?
var x = "5"; var y = 2; console.log(x - y);
a) 3 b) 7 c) "3" d) Error Answer: a) 3
What does the
Array.prototype.push()
method do in JavaScript? a) Adds elements to the beginning of an array b) Creates a new array by transforming each element of the original array c) Adds elements to the end of an array d) Filters the array based on a condition Answer: c) Adds elements to the end of an arrayWhat is the purpose of the
Array.prototype.every()
method in JavaScript? a) To check if at least one element in the array satisfies a condition b) To create a new array by transforming each element of the original array c) To check if all elements in the array satisfy a condition d) To join all elements of an array into a string using a specified separator Answer: c) To check if all elements in the array satisfy a conditionWhat will be the output of the following code?
function foo() { return bar(); function bar() { return "Hello"; } } console.log(foo());
a) "Hello" b) undefined c) Error d) "foo" Answer: a) "Hello"
What is the result of the following code?
var x = 10; var y = "10"; console.log(x === y);
a) true b) false c) "true" d) Error Answer: b) false
What is the purpose of the
Array.prototype.flat()
method in JavaScript? a) To create a new array by transforming each element of the original array b) To flatten nested arrays into a single array c) To sort the elements of an array d) To join all elements of an array into a string using a specified separator Answer: b) To flatten nested arrays into a single arrayWhat is the output of the following code?
console.log(typeof [1, 2]);
a) "array" b) "object" c) "array,object" d) Error Answer: b) "object"
How do you check if a function is a constructor in JavaScript? a) Using
function.constructor
b) Usingfunction() instanceof Constructor
c) Usingfunction() instanceof Function
d) Usingfunction.prototype.constructor
Answer: c) Usingfunction() instanceof Function
What is the purpose of the
Object.prototype.hasOwnProperty()
method in JavaScript? a) To check if an object has a specific property b) To add a property to an object c) To create a new object by transforming the original object d) To check if an object is a prototype of another object Answer: a) To check if an object has a specific propertyWhat will be the result of the following code?
var x = 1; var y = 2; function foo() { console.log(x); var x = 10; console.log(y); } foo();
a) undefined, undefined b) 1, 2 c) undefined, 2 d) Error Answer: a) undefined, undefined
What is the purpose of the
Object.prototype.toString()
method in JavaScript? a) To convert an object to a string b) To check the constructor of an object c) To display the object's properties and values d) To get the string representation of an object Answer: d) To get the string representation of an objectWhat does the
Array.prototype.reduceRight()
method do in JavaScript? a) Adds elements to the end of an array b) Creates a new array by transforming each element of the original array c) Combines all elements of an array into a single value, starting from the right d) Reverses the order of elements in the array Answer: c) Combines all elements of an array into a single value, starting from the rightWhat is the output of the following code?
function foo() { return this; } console.log(foo());
a) null b) undefined c) Window object (in a browser environment) d) Error Answer: c) Window object (in a browser environment)
How do you declare a private variable in JavaScript? a)
this.privateVar = 10;
b)var privateVar = 10;
c)const privateVar = 10;
d)let privateVar = 10;
Answer: b)var privateVar = 10;
What is the output of the following code?
var x = 1; function foo() { console.log(x); var x = 10; console.log(x); } foo();
a) undefined, 10 b) 1, 10 c) 1, undefined d) Error Answer: a) undefined, 10
What is the purpose of the
Array.prototype.indexOf()
method in JavaScript? a) To check if an element exists in an array b) To find the index of the first occurrence of a specified element in an array c) To filter the array based on a condition d) To sort the elements of an array Answer: b) To find the index of the first occurrence of a specified element in an arrayWhat will be the result of the following code?
var x = 10; function foo() { x = 20; return; function x() {} } foo(); console.log(x);
a) 10 b) 20 c) undefined d) Error Answer: a) 10
What is the purpose of the
Object.prototype.isPrototypeOf()
method in JavaScript? a) To check if an object is a prototype of another object b) To convert an object to a string c) To display the object's properties and values d) To get the string representation of an object Answer: a) To check if an object is a prototype of another objectWhat is the output of the following code?
var x = 1; if (true) { var x = 2; } console.log(x);
a) 1 b) 2 c) undefined d) Error Answer: b) 2
What is the purpose of the
Array.prototype.every()
method in JavaScript? a) To add elements to the beginning of an array b) To check if at least one element in the array satisfies a condition c) To join all elements of an array into a string using a specified separator d) To check if all elements in the array satisfy a condition Answer: d) To check if all elements in the array satisfy a conditionWhat will be the result of the following code?
var x = [1, 2, 3]; x[5] = 5; console.log(x.length);
a) 3 b) 4 c) 6 d) 5 Answer: c) 6
What is the output of the following code?
var x = [1, 2, 3]; console.log(x.toString());
a) "1,2,3" b) [1, 2, 3] c) "1 2 3" d) Error Answer: a) "1,2,3"
How do you create a copy of an object in JavaScript? a)
var newObj = Object.create(obj);
b)var newObj = obj;
c)var newObj = Object.assign({}, obj);
d)var newObj = obj.clone();
Answer: c)var newObj = Object.assign({}, obj);
What is the output of the following code?
var x = 1; var y = 2; if (x < y) { var result = function() { return x; } } console.log(result());
a) 1 b) 2 c) undefined d) Error Answer: a) 1
What is the purpose of the
Array.prototype.slice()
method in JavaScript? a) To remove elements from the beginning of an array b) To create a new array by transforming each element of the original array c) To add elements to the beginning of an array d) To create a shallow copy of an array from the specified start to end Answer: d) To create a shallow copy of an array from the specified start to endWhat does the
Function.prototype.bind()
method do in JavaScript? a) To concatenate two strings b) To create a new array by transforming each element of the original array c) To attach an event handler function d) To set the value ofthis
in a function permanently Answer: d) To set the value ofthis
in a function permanentlyWhat will be the result of the following code?
var x = 1; function foo() { var x = 2; return x; } console.log(foo());
a) 1 b) 2 c) undefined d) Error Answer: b) 2
What is the output of the following code?
var x = [1, 2, 3]; console.log(x[6]);
a) undefined b) null c) Error d) 6 Answer: a) undefined
How do you check if an object is empty in JavaScript? a) Using
Object.empty(obj)
b) UsingObject.keys(obj).length === 0
c) UsingObject.isEmpty(obj)
d) Usingobj.isEmpty()
Answer: b) UsingObject.keys(obj).length === 0
What is the output of the following code?
console.log(2 + 2 - "2");
a) 2 b) 0 c) 4 d) Error Answer: c) 4
What is the purpose of the
Array.prototype.flatMap()
method in JavaScript? a) To create a new array by transforming each element of the original array b) To flatten nested arrays into a single array c) To sort the elements of an array d) To combine all elements of an array into a single value Answer: a) To create a new array by transforming each element of the original arrayWhat will be the result of the following code?
var x = 5; var y = "5"; console.log(x + y);
a) 10 b) "55" c) "5" d) Error Answer: b) "55"
What is the output of the following code?
function foo() { console.log(this); } foo.call(null);
a) null b) undefined c) Window object (in a browser environment) d) Error Answer: c) Window object (in a browser environment)
What does the
Array.prototype.copyWithin()
method do in JavaScript? a) Adds elements to the end of an array b) Creates a new array by transforming each element of the original array c) Copies elements within an array to a specified position d) Reverses the order of elements in the array Answer: c) Copies elements within an array to a specified positionWhat is the output of the following code?
var x = 1; var y = 2; function foo() { console.log(y); var y = 3; console.log(y); } foo();
a) undefined, 3 b) 2, 3 c) 2, undefined d) Error Answer: a) undefined, 3
What is the purpose of the
Array.prototype.lastIndexOf()
method in JavaScript? a) To check if an element exists in an array b) To find the index of the last occurrence of a specified element in an array c) To filter the array based on a condition d) To sort the elements of an array Answer: b) To find the index of the last occurrence of a specified element in an arrayWhat will be the result of the following code?
var x = [1, 2, 3]; var y = x.map(function(a) { return a + 1; }); console.log(y);
a) [2, 3, 4] b) [1, 2, 3] c) [1, 2, 3, 4] d) Error Answer: a) [2, 3, 4]
What is the output of the following code?
var x = [1, 2, 3]; x.length = 0; console.log(x);
a) [1, 2, 3] b) [] c) [null, null, null] d) Error Answer: b) []
What does the
Array.prototype.forEach()
method do in JavaScript? a) Adds elements to the end of an array b) Creates a new array by transforming each element of the original array c) Loops over each element of an array and executes a callback function d) Filters the array based on a condition Answer: c) Loops over each element of an array and executes a callback functionWhat will be the result of the following code?
var x = [1, 2, 3]; console.log(x instanceof Array);
a) true b) false c) Error d) undefined Answer: a) true
What is the purpose of the
Array.prototype.reverse()
method in JavaScript? a) Adds elements to the end of an array b) Creates a new array by transforming each element of the original array c) Reverses the order of elements in the array d) Joins all elements of an array into a string using a specified separator Answer: c) Reverses the order of elements in the arrayWhat is the output of the following code?
console.log(10 + 20 + "30");
a) "3030" b) "6030" c) "103020" d) Error Answer: a) "3030"
How do you check if an object is an instance of a specific class in JavaScript? a) Using
object instanceof Class
b) Usingobject.isInstance(Class)
c) Usingobject.prototype.instanceOf(Class)
d) Usingobject.instance(Class)
Answer: a) Usingobject instanceof Class
What is the purpose of the
Array.prototype.some()
method in JavaScript? a) To add elements to the beginning of an array b) To check if at least one element in the array satisfies a condition c) To join all elements of an array into a string using a specified separator d) To check if some elements in the array satisfy a condition Answer: d) To check if some elements in the array satisfy a conditionWhat will be the result of the following code?
var x = [1, 2, 3]; x.shift(); console.log(x);
a) [1, 2, 3] b) [2, 3] c) [2] d) Error Answer: b) [2, 3]
What is the purpose of the
Array.prototype.sort()
method in JavaScript? a) To create a new array by transforming each element of the original array b) To sort the elements of an array c) To add elements to the end of an array d) To filter the array based on a condition Answer: b) To sort the elements of an arrayWhat is the output of the following code?
var x = [1, 2, 3]; x.push(4); console.log(x);
a) [1, 2, 3] b) [1, 2, 3, 4] c) [4, 1, 2, 3] d) Error Answer: b) [1, 2, 3, 4]
How do you check if an object is an array in JavaScript? a) Using
typeof
b) UsingisArray()
c) Usingtype()
d) UsingisTypeOf()
Answer: b) UsingisArray()
What is the output of the following code?
var x = 10; var y = "10"; console.log(x === y);
a) true b) false c) "true" d) Error Answer: b) false
What is the purpose of the
Object.prototype.hasOwnProperty()
method in JavaScript? a) To check if an object has a specific property b) To add a property to an object c) To create a new object by transforming the original object d) To check if an object is a prototype of another object Answer: a) To check if an object has a specific propertyWhat will be the result of the following code?
var x = 1; function foo() { console.log(x); var x = 10; console.log(x); } foo();
a) undefined, 10 b) 1, 10 c) 1, undefined d) Error Answer: a) undefined, 10
What is the purpose of the
Object.prototype.toString()
method in JavaScript? a) To convert an object to a string b) To check the constructor of an object c) To display the object's properties and values d) To get the string representation of an object Answer: d) To get the string representation of an objectWhat does the
Array.prototype.flatMap()
method do in JavaScript? a) To create a new array by transforming each element of the original array b) To flatten nested arrays into a single array c) To sort the elements of an array d) To combine all elements of an array into a single value Answer: a) To create a new array by transforming each element of the original array
Subscribe to my newsletter
Read articles from Anusree Anilkumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
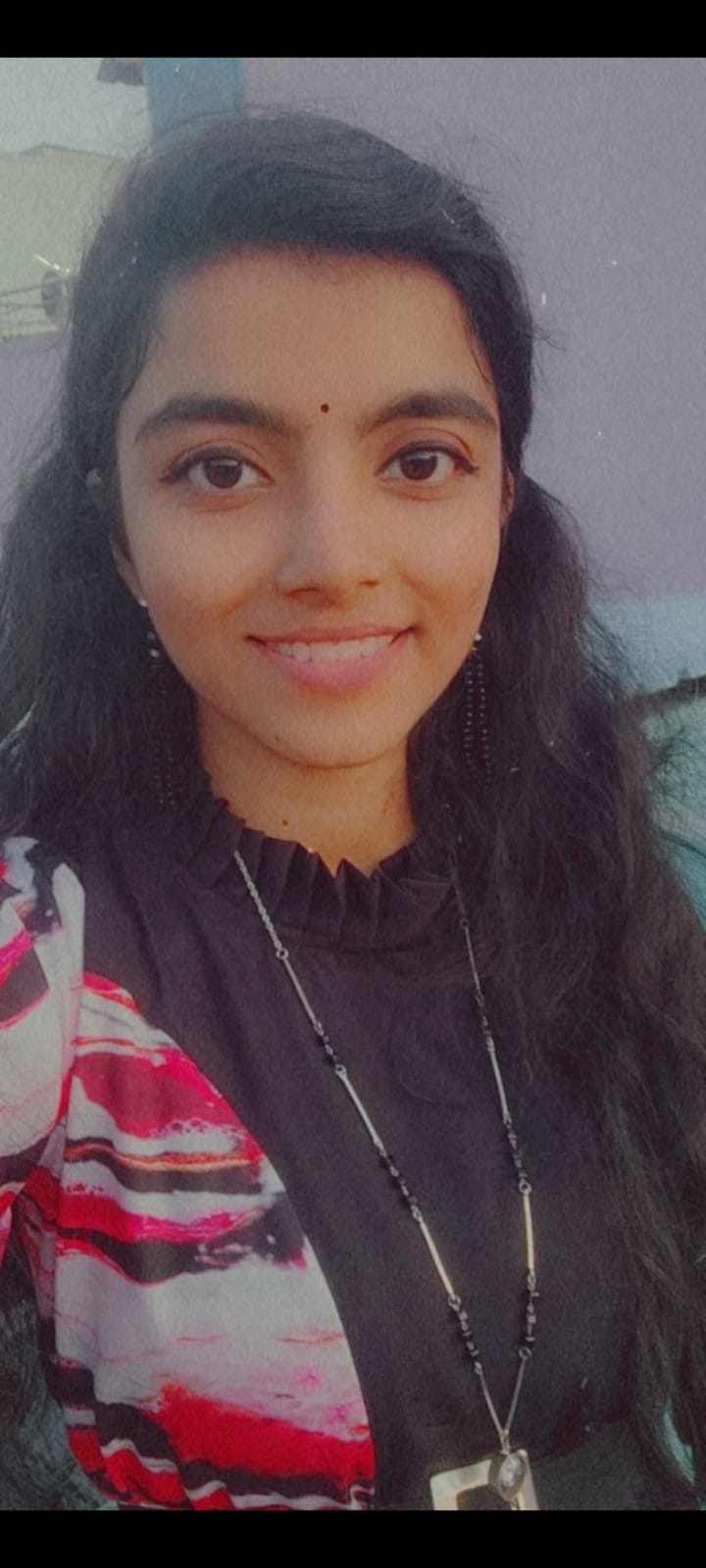