Java Double: Parse String with Comma

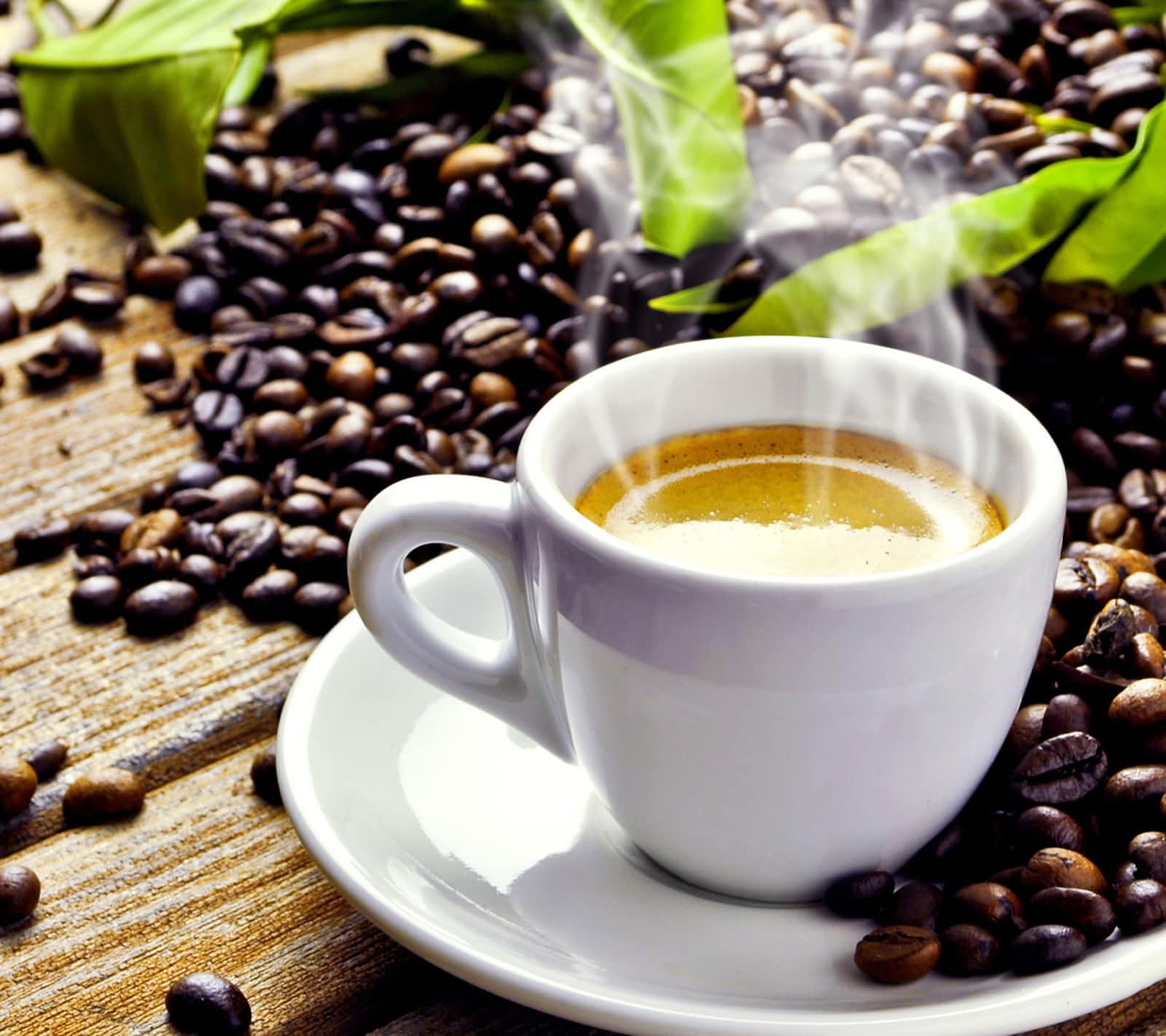
Converting numbers expressed as strings in Java Double object values (or the double primitive counterpart) could be tricky in specific situations. This is due to the number of formats available, which can also be different in different countries. In this post, we describe how to deal with strings containing a comma as a decimal separator, instead of a point.
You can find the code fragments used in this post in the following GitHub project: java miscellaneous tips.
Parse String with Comma without Group Separators
In the simplest scenario, we have a number represented as a string in which the only peculiarity is the use of a comma instead of a point as a decimal separator. In this case, we can simply replace the occurrence of the comma with a point, using the String replaceAll method, as in the following example.
String numStr = "121,234";
Double doubleNum = Double.valueOf(numStr.replaceAll(",", "."));
System.out.println(doubleNum);
In the example above we use the Double’s valueOf method to parse the string. As an alternative, we can use the parseDouble method, which returns the primitive type counterpart of Double, as in the following:
double doubleNum = Double.parseDouble(numStr.replaceAll(",", "."));
Parse String with Comma with Group Separators
A more complex situation is when inside our string we have also a grouping of the thousands in the integer part of the number. In some countries, they use a point for separating the thousands in the integer part and a comma to separate the fractional part. This is the case in Italy, for instance.
To deal with this scenario, we can use the NumberFormat class and get an instance from it specifying the Locale, as in the following example:
String numStr = "123.111,234";
NumberFormat format = NumberFormat.getInstance(Locale.ITALY);
Number number = format.parse(numStr);
double doubleNum = number.doubleValue();
System.out.println(doubleNum);
The example above will print the value “123111.234”. Instead of specifying the Locale we can also instantiate a DecimalFormat and set it with a DecimalFormatSymbols object with the proper decimal and grouping separators:
String numStr = "123.111,234";
DecimalFormat decimalFormat = new DecimalFormat();
DecimalFormatSymbols symbols = new DecimalFormatSymbols();
symbols.setDecimalSeparator(',');
symbols.setGroupingSeparator('.');
decimalFormat.setDecimalFormatSymbols(symbols);
Number num = decimalFormat.parse(numStr);
System.out.println(num.toString());
Parse String with Comma with Group Separators: an Alternative
An alternative to the approach described above is to combine the DecimalFormatSymbols with the specific Locale and pass it to a DecimalFormat object in combination with a pattern, as in the following:
String numStr = "123.111,234";
DecimalFormatSymbols symbols = new DecimalFormatSymbols(Locale.ITALY);
DecimalFormat decimalFormat = new DecimalFormat("#,###.##", symbols);
Number num = decimalFormat.parse(numStr);
System.out.println(num.toString());
The particularity of the example above is that the pattern “#,###.##” follows a standard in which the comma is used as a grouping separator and the point as a decimal separator. It is the DecimalFormatSymbols set with the Locale that forces the proper Italian representation with those characters inverted.
Subscribe to my newsletter
Read articles from Mario Casari directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
