Introduction of C# (part 2/2)
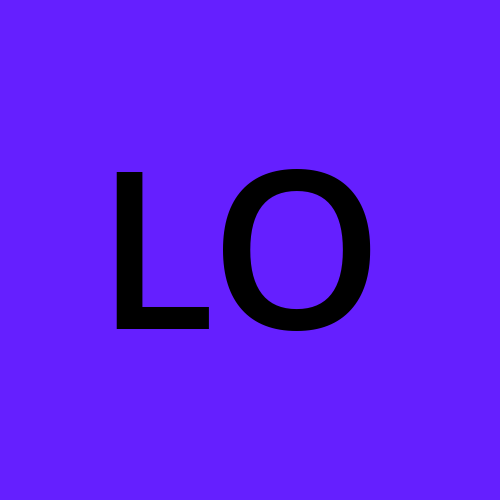

If/Else
Conditions and If Statements
C# supports the usual logical conditions from mathematics:
Less than: a < b
Less than or equal to: a <= b
Greater than: a > b
Greater than or equal to: a >= b
Equal to: a == b
Not Equal to: a != b
You can use these conditions to perform different decisions.
C# has the following conditional statements
Use if to specify a block of code to be executed, if a specified condition is true.
Use else to specify a block of code to be executed if the same condition is false.
Use else if to specify a new condition to test, if the first condition is false.
Use the switch to specify many alternative blocks of code to be executed.
In the example below, we test two values to find out if 20 is greater than 18. If the condition is True, print some text:
We can also test variables:
In the example above, we use two variables, x, and y to test whether x is greater than y (using the > operator). As x is 20 and y is 18 and we know that 20 is greater than 18, we print to the screen that "x is greater than y".
The else statement
Use the else statement to specify a block of code to be executed if the condition is False.
In the example above, time (20) is greater than 18, so the condition is False. Because of this, we move on to the else condition and print to the screen "Good evening.". If the time was less than 18, the program would print "Good day.".
Loops
Loops can execute a block of code as long as a specified condition is reached. Loops are handy because they save time, reduce errors, and they make code more readable.
While Loop
The while loop loops through a block of code as long as a specified condition is True:
In the example below, the code in the loop will run over and over again, as long as a variable (i) is less than 5:
The Do/While Loop
The do/while loop is a variant of the while loop. This loop will execute the code block once, before checking if the condition is True, and then it will repeat the loop as long as the condition is True.
For Loop
When you know exactly how many times you want to loop through a block of code, use the for loop instead of a while loop:
Statement 1 is executed (one time) before the execution of the code block.
Statement 2 defines the condition for executing the code block.
Statement 3 is executed (every time) after the code block has been executed.
Statement 1 sets a variable before the loop starts (int i = 0).
Statement 2 defines the condition for the loop to run (i must be less than 5). If the condition is true, the loop will start over again, if it is false, the loop will end.
Statement 3 increases a value (i++) each time the code block in the loop has been executed.
This example will only print even values between 0 and 10:
Nested Loops
It is also possible to place a loop inside another loop. This is called a nested loop. The "inner loop" will be executed one time for each iteration of the "outer loop":
Foreach Loop
There is also a foreach loop, which is used exclusively to loop through elements in an array:
The following example outputs all elements in the cars array, using a foreach loop:
Arrays
Arrays are used to store multiple values in a single variable, instead of declaring separate variables for each value.
To declare an array, define the variable type with square brackets:
We have now declared a variable that holds an array of strings.
To insert values to it, we can use an array literal - place the values in a comma-separated list, inside curly braces:
To create an array of integers, you could write:
Access Elements of an Array
You can access an array element by referring to the index number.
This statement accesses the value of the first element in cars:
Change an Array Element
To change the value of a specific element, refer to the index number:
Array Length
To find out how many elements an array has, use the Length property:
Other Ways to Create an Array
If you are familiar with C#, you might have seen arrays created with the new keyword and perhaps you have seen arrays with a specified size as well. In C#, there are different ways to create an array:
It is up to you which option you choose. In our tutorial, we will often use the last option, as it is faster and easier to read.
However, you should note that if you declare an array and initialize it later, you have to use the new keyword:
Loop Through an Array
You can loop through the array elements with the for loop, and use the Length property to specify how many times the loop should run.
The following example outputs all elements in the cars array:
You can loop through the array elements with the foreach loop.
The following example outputs all elements in the cars array:
Method Parameters
Parameters and Arguments
Information can be passed to methods as parameters. Parameters act as variables inside the method. They are specified after the method name, inside the parentheses. You can add as many parameters as you want, just separate them with a comma.
The following example has a method that takes a string called fname as a parameter. When the method is called, we pass along a first name, which is used inside the method to print the full name:
Multiple Parameters
You can have as many parameters as you like, just separate them with commas:
Default Parameter Value
You can also use a default parameter value, by using the equals sign (=).
If we call the method without an argument, it uses the default value ("Norway"):
Return Values
In the previous page, we used the void keyword in all examples, which indicates that the method should not return a value.
If you want the method to return a value, you can use a primitive data type(such as int or double) instead of void, and use the return keyword inside the method:
This example returns the sum of a method's two parameters:
You can also store the result in a variable (recommended, as it is easier to read and maintain):
Named Arguments
It is also possible to send arguments with the key: value syntax. That way, the order of the arguments does not matter:
C# OOP (Object-Oriented Programming)
OOP stands for Object-Oriented Programming.
Procedural programming is about writing procedures or methods that perform operations on the data, while object-oriented programming is about creating objects that contain both data and methods.
Object-oriented programming has several advantages over procedural programming:
OOP is faster and easier to execute.
OOP provides a clear structure for the programs.
OOP helps to keep the C# code DRY "Don't Repeat Yourself", and makes the code easier to maintain, modify and debug.
OOP makes it possible to create fully reusable applications with less code and shorter development time.
What are Classes and Objects?
Classes and objects are the two main aspects of object-oriented programming.
Classes and Objects
Everything in C# is associated with classes and objects, along with its attributes and methods. For example: in real life, a car is an object. The car has attributes, such as weight and color, and methods such as drive and brake.
A Class is like an object constructor or a "blueprint" for creating objects.
Create a Class and Object
To create a class, use the class keyword. To create an object of the Program, specify the class name, followed by the object name, and use the keyword new:
Multiple Objects
You can create multiple objects of one class:
Using Multiple Classes
You can also create an object of a class and access it in another class. This is often used for better organization of classes (one class has all the fields and methods, while the other class holds the Main() method (code to be executed)).
Conclusion
C# is a powerful and versatile programming language developed by Microsoft that runs on the .NET Framework. C# can be used to create various types of applications, such as web apps, desktop apps, mobile apps, games, and more. C# is easy to learn with the help of online tutorials, examples, and quizzes. C# has many features that make it a great choice for developers, such as classes, structs, records, interfaces, delegates, events, generics, iterators, and more. C# also supports expression-bodied members, which allows you to write concise and readable code. C# is a strongly typed language that supports equality comparisons and operators. C# also has a rich set of built-in types, such as strings, numbers, arrays, and collections. C# is a modern and innovative language that is constantly evolving with new features and enhancements.
Subscribe to my newsletter
Read articles from Leoneil Odrunia directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
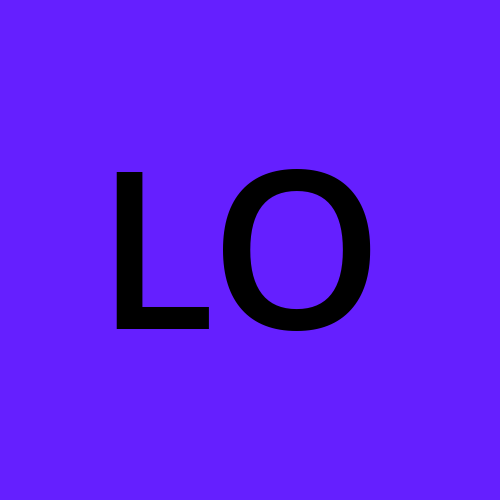