๐Todo Form Component in React
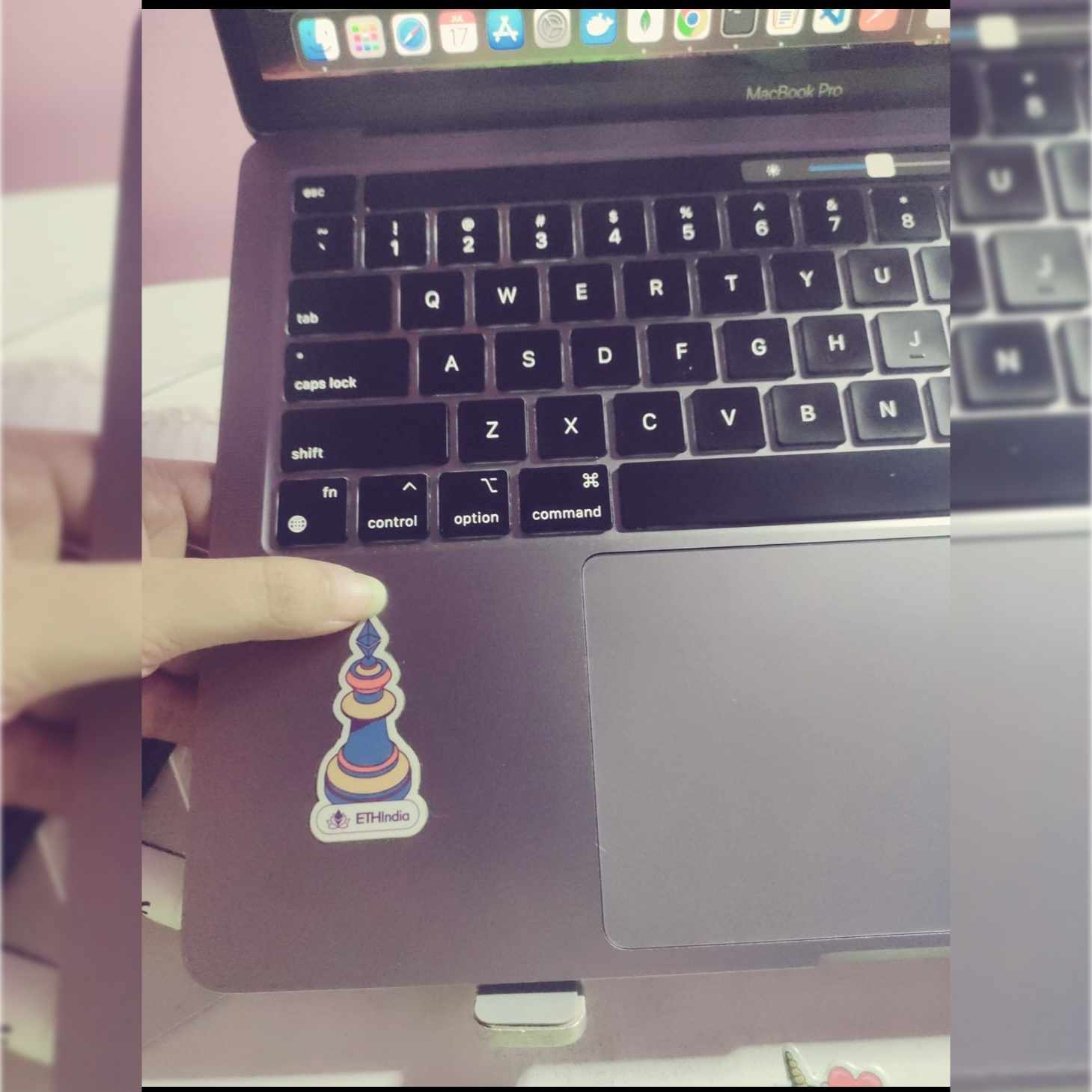
2 min read
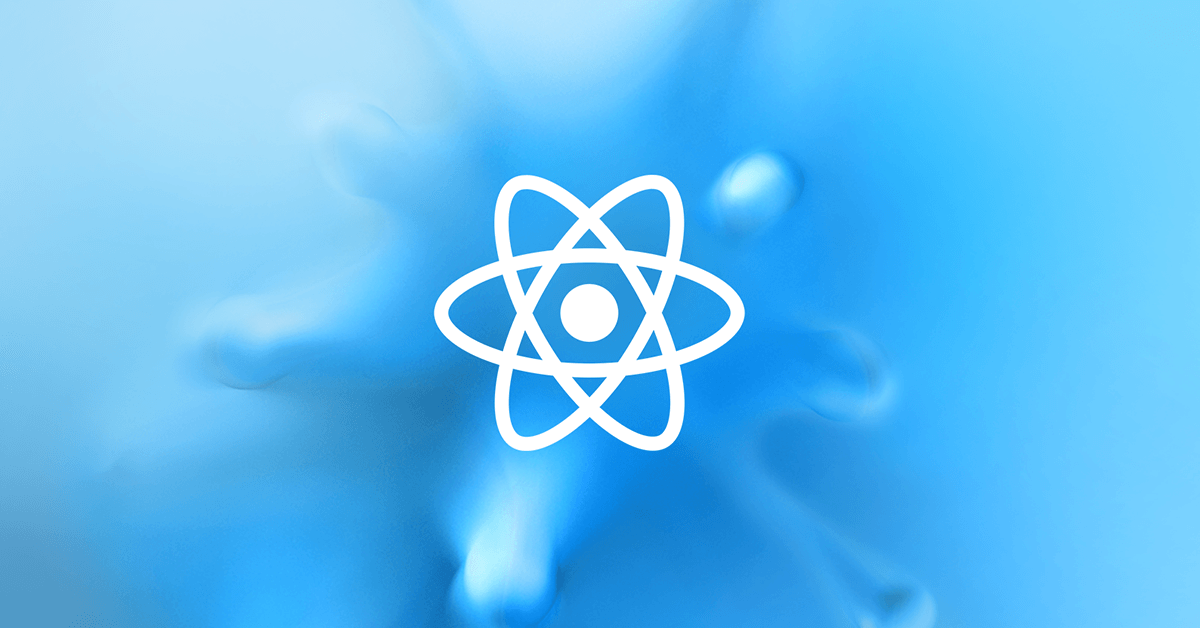
In this tutorial, we'll create a Todo Form component in React that allows users to input and submit their todo tasks along with date, start time, end time, and category. We'll also fetch the list of available categories from the server using an API call. Let's get started!
import React, { Component } from "react";
import { getData } from "../helper/apicalls";
class FormClass extends Component {
constructor(props) {
super(props);
this.state = {
category: "",
name: "",
date: "",
startTime: "",
endTime: "",
categories: []
}
}
componentDidMount() {
this.fetchData();
}
fetchData = async () => {
try {
const response = await getData("/category");
this.setState({ categories: response });
} catch (error) {
console.log(error);
}
}
handleSubmit = () => {
const { name, date, startTime, endTime, category } = this.state;
const formData = {
name,
date,
startTime,
endTime,
category
}
this.props.onSubmit(formData);
}
render() {
const { name, date, startTime, endTime, category, categories } = this.state;
return (
<div className="row g-3 my-5">
<div className="col-12">
<label htmlFor="inputAddress" className="form-label">
Enter Todo
</label>
<input
type="text"
className="form-control"
id="inputAddress"
placeholder="Express..."
value={name}
onChange={(e) => this.setState({ name: e.target.value })}
/>
</div>
<div className="col-12">
<label htmlFor="inputAddress2" className="form-label">
Enter Date
</label>
<input
type="date"
className="form-control"
id="inputAddress2"
placeholder="Apartment, studio, or floor"
value={date}
onChange={(e) => this.setState({ date: e.target.value })}
/>
</div>
<div className="col-md-6">
<label htmlFor="inputCity" className="form-label">
Start Time
</label>
<input
type="time"
className="form-control"
id="inputCity"
value={startTime}
onChange={(e) => this.setState({ startTime: e.target.value })}
/>
</div>
<div className="col-md-6">
<label htmlFor="inputCity" className="form-label">
End Time
</label>
<input
type="time"
className="form-control"
id="inputCity"
value={endTime}
onChange={(e) => this.setState({ endTime: e.target.value })}
/>
</div>
<div className="col-12">
<label htmlFor="inputState" className="form-label">
Category
</label>
<select
id="inputState"
className="form-select"
value={category}
onChange={(e) => this.setState({ category: e.target.value })}
>
<option value="">Choose...</option>
{categories &&
categories.map((cat) => (
<option value={cat.name} key={cat.id}>
{cat.name}
</option>
))}
</select>
</div>
<div className="col-12 d-grid">
<button
type="submit"
className="btn btn-info"
onClick={this.handleSubmit}
>
Add Todo
</button>
</div>
</div>
);
}
}
export default FormClass;
It creates a Todo Form component using React, fetches categories from the server, and handles form submissions. Feel free to add your own styles or modify the component to suit your specific needs. Happy coding! ๐
0
Subscribe to my newsletter
Read articles from Sumana directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
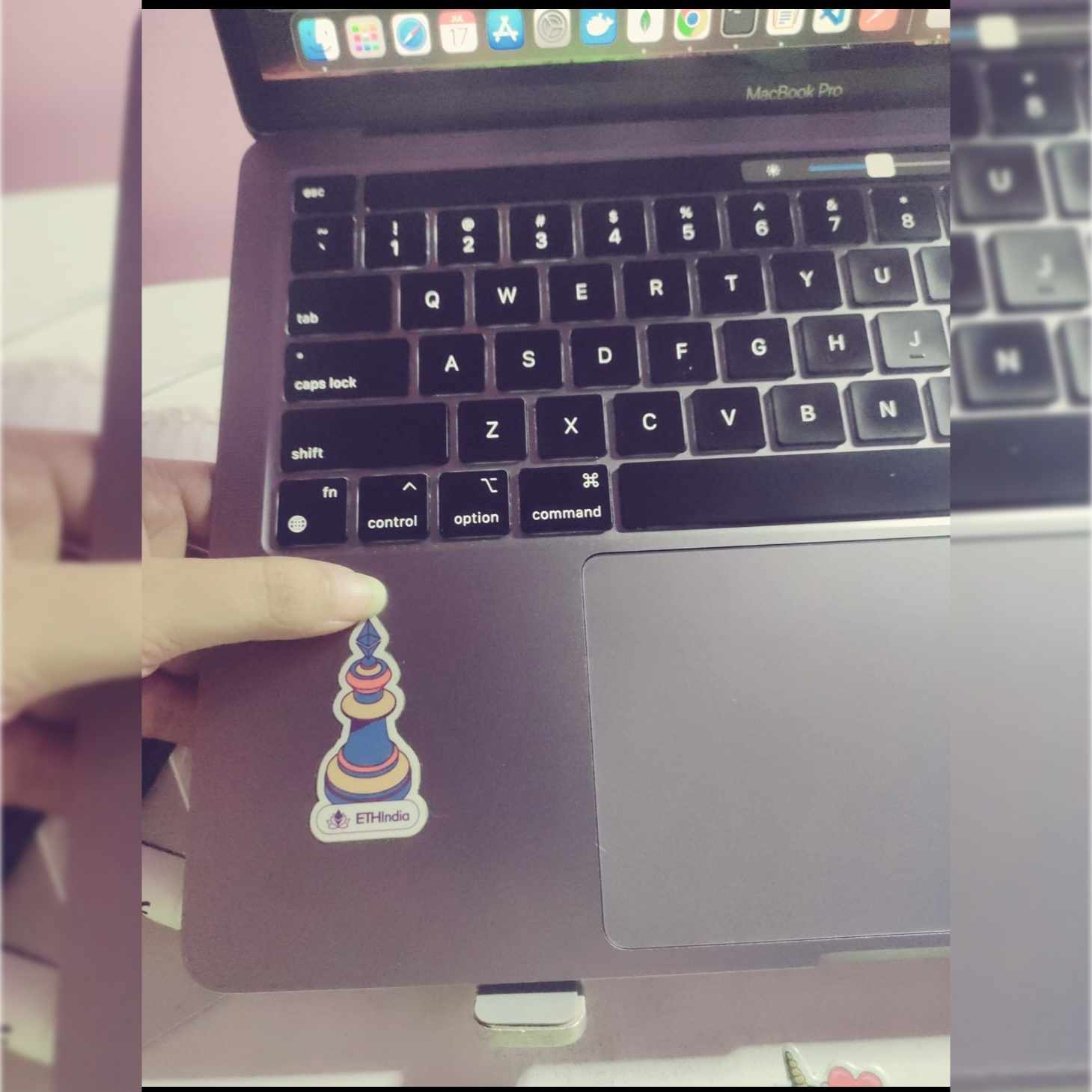