Abstract class vs Interface

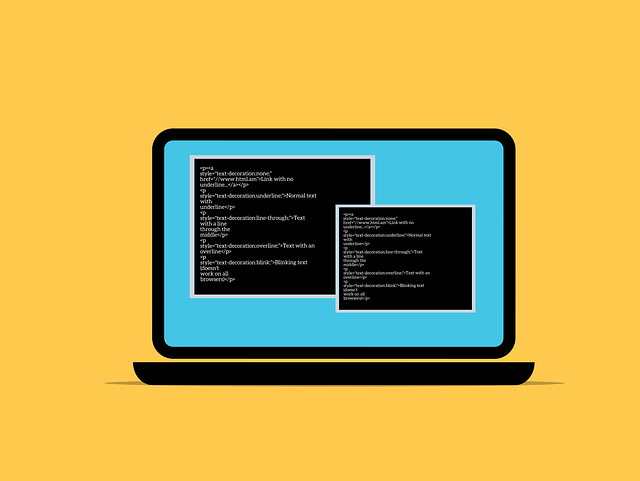
In C#, an abstract class and an interface are both used to define a set of methods, properties, and events that can be implemented by other classes. However, there are some key differences between them:
Implementation: An interface only defines a contract for what methods, properties, and events a class must have, but it does not provide any implementation. On the other hand, an abstract class can provide some default implementation for its members, but the derived classes can override them.
Multiple inheritance: A class can implement multiple interfaces, but it can only inherit from one abstract class. This is because C# does not support multiple inheritance of classes.
Access modifiers: Members of an interface are always public, while members of an abstract class can have any access modifier.
Constructor: An interface cannot have a constructor, while an abstract class can have one.
Fields: An interface cannot have fields, while an abstract class can have fields and other non-abstract members.
Partial implementation: An abstract class can have some members that are not abstract, while an interface cannot have any members with implementation.
Purpose: An abstract class is typically used to provide a base class with some common functionality that derived classes can use, while an interface is used to define a common set of methods and properties that classes should implement, regardless of their implementation details.
Abstract Class in Detail
An abstract class is a class that cannot be instantiated directly but serves as a base class for other classes. It is a class that provides a standard interface and some common functionality for its derived classes, but cannot be used on its own.
The abstract keyword is used to declare an abstract class, and it is typically used when you have a set of classes that share some common functionality, but they each have their unique implementation details. By declaring an abstract class, you can define the common functionality in the base class, and leave the specific details to the derived classes.
Some key features of C# abstract classes include:
Cannot be instantiated: As mentioned earlier, you cannot create an instance of an abstract class. It can only be used as a base class for other classes.
Can contain abstract members: An abstract class can contain abstract methods, properties, and events that have no implementation. These members must be implemented by the derived classes.
Can contain non-abstract members: An abstract class can also contain non-abstract members, such as fields, properties, methods, and events, that have an implementation. The derived classes can use these members, but they can also be overridden if necessary.
Provides inheritance: An abstract class provides inheritance to its derived classes, allowing them to inherit its properties, methods, and events.
It must be inherited from a derived class and implement all the abstract members of its base abstract class, or else it must also be declared abstract.
Overall, abstract classes in C# are a useful tool for designing and organizing class hierarchies, allowing you to provide a common interface and some common functionality for a set of related classes.
Interfaces in Detail
An interface is a type that defines a set of methods, properties, and events that a class must implement. It specifies a contract that a class must adhere to, without providing any implementation details. In other words, an interface defines what a class must do, but not how it should do it.
To declare an interface in C#, you use the interface keyword followed by the interface name, and then list the members that the interface defines. Here’s an example:
interface IMyInterface
{
void DoSomething();
int SomeProperty { get; set; }
event EventHandler SomeEvent;
}
In this example, the interface name IMyInterface
defines three members: a method called DoSomething()
, a property called SomeProperty
, and an event called SomeEvent
.
A class can implement one or more interfaces by declaring that it implements the interface and then implementing all the members of the interface. Here’s an example:
class MyClass : IMyInterface
{
public void DoSomething()
{
// Implementation goes here
}
public int SomeProperty { get; set; }
public event EventHandler SomeEvent;
}
In this example, the class named MyClass
implements the IMyInterface
interface by providing implementations for all the members of the interface.
Some key features of C# interfaces include:
Defines a contract: An interface defines a contract that a class must adhere to, specifying what members it must implement.
Provides polymorphism: Because a class can implement multiple interfaces, interfaces provide a way to achieve polymorphism in C#.
No implementation details: An interface does not provide any implementation details for its members, leaving that up to the implementing class.
Can inherit from other interfaces: An interface can inherit from one or more other interfaces, allowing you to build up a hierarchy of interfaces.
Can be used to achieve loose coupling: By programming to an interface rather than a specific class, you can achieve loose coupling and make your code more flexible and extensible.
Overall, interfaces in C# are a powerful tool for designing and implementing flexible, extensible software. They provide a way to define contracts between classes, achieve polymorphism, and build flexible, loosely coupled systems.
Subscribe to my newsletter
Read articles from Guillermo Valenzuela directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
