Demystifying Buffers and Flags in the printf Function.
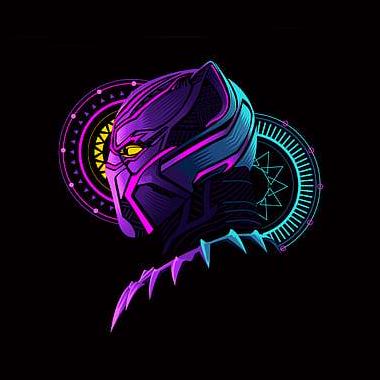
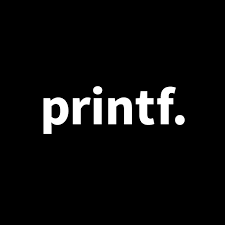
Introduction
The printf
function is a powerful tool in C and C++ programming for formatting and printing output to the console or file stream. While using printf
is a common practice among developers, understanding the concepts of buffers and flags is crucial to utilize this function effectively and securely. In this blog, we will take a deep dive into buffers and flags in the context of printf
, unraveling their significance, practical applications, and best practices for safe coding.
1. Buffers - Understanding the Basics
A buffer is a temporary storage area in memory used to hold data before it is processed or output. In the case of the printf
function, the data to be displayed is first assembled and stored in a buffer. This approach reduces the overhead of multiple smaller write operations to the output device, enhancing the efficiency of the printing process. Buffers play a vital role in managing the flow of data between the application and the output device.
2. Buffer Overflow - The Potential Danger
Buffer overflows occur when data exceeds the capacity of the allocated buffer, resulting in data being written beyond the intended memory area. This can lead to erratic behaviour, program crashes, or even opening the door to security vulnerabilities. As developers, it is imperative to ensure that the buffer size is sufficient to accommodate the largest possible formatted output. Employing functions like snprintf
specifying the buffer size can help prevent buffer overflows and keep your code secure.
3. printf Function - A Brief Overview
The printf
function's signature is as follows:
int printf(const char *format, ...);
format
: A string containing format specifiers for the subsequent arguments....
: Variable number of arguments corresponding to the format specifiers.
The printf
function scans the format
string for format specifiers, replacing them with the corresponding argument values and outputting the formatted result. Understanding how to use format specifiers effectively is essential for displaying data of different types and controlling precision and width.
4. Format Specifiers - Unleashing the Power
Format specifiers are special placeholders in the format
string that defines the type of data to be displayed and how it should be presented. They allow you to print data of different types and control various aspects of formatting. Common format specifiers include:
%d
,%i
: Integers (decimal)%u
: Unsigned integers (decimal)%o
: Unsigned integers (octal)%x
,%X
: Unsigned integers (hexadecimal)%f
: Floating-point numbers (standard notation)%e
,%E
: Floating-point numbers (scientific notation)%g
,%G
: Floating-point numbers (shortest representation)%c
: Characters%s
: Strings
5. Flags - The Art of Formatting
Flags are optional components added to format specifiers to modify the output's behaviour. They allow you to control the alignment, sign representation, padding, and other formatting aspects. Some commonly used flags include:
-
: Left-align output within the field width.+
: Display the sign for both positive and negative numbers.0
: Pad numeric output with leading zeros.#
: Use an alternate form (e.g., add "0x" prefix for hexadecimal values).
By utilizing flags strategically, you can achieve the desired presentation of data in your printf
statements.
6. Security - Keeping Your Code Safe
Improper use of printf
can lead to security vulnerabilities, particularly format string vulnerabilities. These occur when the format
string is user-controlled, allowing attackers to insert malicious format specifiers and access sensitive data from the program's stack or memory. To mitigate these risks, always validate user input and avoid using user-controlled data as the format
string. Use constants or predefined format strings instead of dynamic or user-controlled ones whenever possible.
7. Flushing Buffers - Understanding the Mechanism
In C, buffers are typically flushed automatically when a newline character is printed or when the buffer is full. However, you can manually flush the buffer using fflush(stdout)
. Proper buffer flushing ensures timely and correct output display, especially in scenarios where immediate output is required.
Conclusion
Understanding buffers and flags in the printf
function is essential for writing efficient and secure code. Buffers play a crucial role in assembling formatted output, while flags enable fine-tuning the presentation. By being mindful of buffer size, employing buffer overflow prevention techniques, and understanding security concerns related to user-controlled data, developers can harness the full potential of printf
while safeguarding their code from vulnerabilities. So, the next time you use printf
, remember to embrace the power of buffers and flags to create more polished, efficient, and secure applications.
Subscribe to my newsletter
Read articles from iVGeek directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
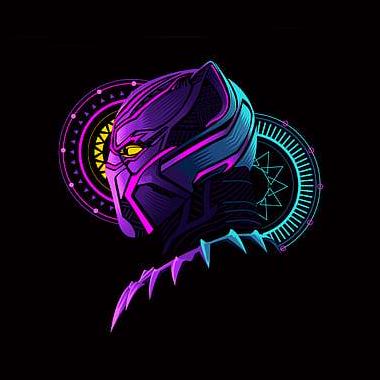
iVGeek
iVGeek
Full-stack developer by day, coffee consumer by night, and code magician in between. I turn caffeine into code and bugs into features. On a mission to make the world a better place—one line of code and one cup of coffee at a time. Lover of memes, dogs, and clean code. Let's code, laugh, and caffeinate together! ☕️✨