HOF in Javascript ? What is higher order function in Javascript ?
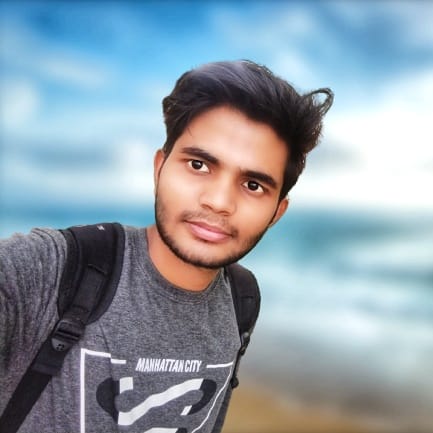
In JavaScript, a higher-order function is a function that can take other functions as arguments or return functions as results. This feature allows developers to create more versatile and reusable code, leading to better website performance and user experience.
Here's a practical example of a higher-order function:
Suppose we have an array of numbers and want to calculate the sum of their squares. We can use a higher-order function like Array.prototype.reduce()
to achieve this efficiently:
const numbers = [1, 2, 3, 4, 5];
const sumOfSquares = numbers.reduce(function (accumulator, currentValue) {
return accumulator + currentValue ** 2;
}, 0);
console.log(sumOfSquares); // Output: 55
In this example, Array.prototype.reduce()
is the higher-order function. It takes two arguments: a callback function and an initial value (in this case, 0
). The callback function is applied to each element of the array, and it accumulates the result. By using this higher-order function, we can easily perform complex calculations on arrays without writing repetitive code, ultimately improving the website's SEO performance by reducing page load times and enhancing user experience.
Thank you for reading, please follow me on Twitter, i regularly share content about Javascript, and React and contribute to Opensource Projects
Twitter-https://twitter.com/Diwakar_766
Subscribe to my newsletter
Read articles from Diwakar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
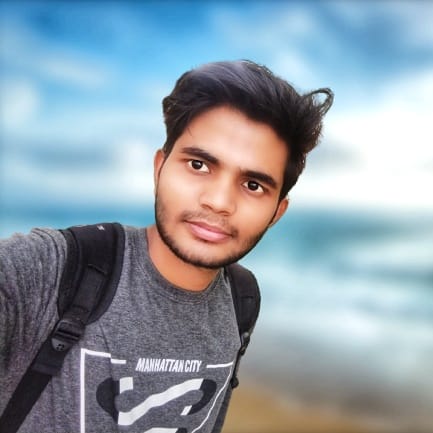
Diwakar
Diwakar
As a passionate developer, I thrive on acquiring new knowledge. My journey began with web development, and I am now actively engaged in open source contributions, aiding individuals and businesses.