Multiple Inheritance in Object-Oriented Programming
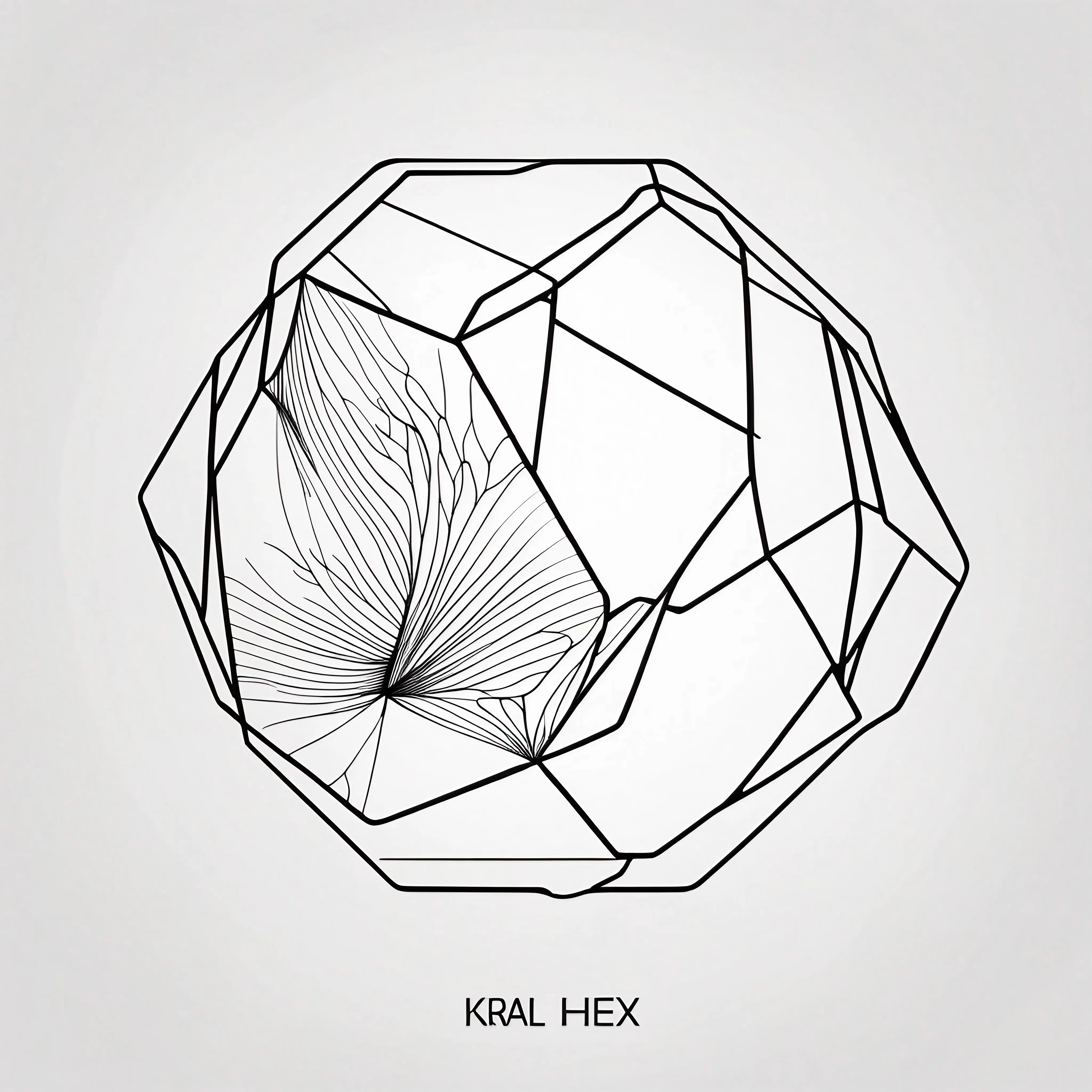
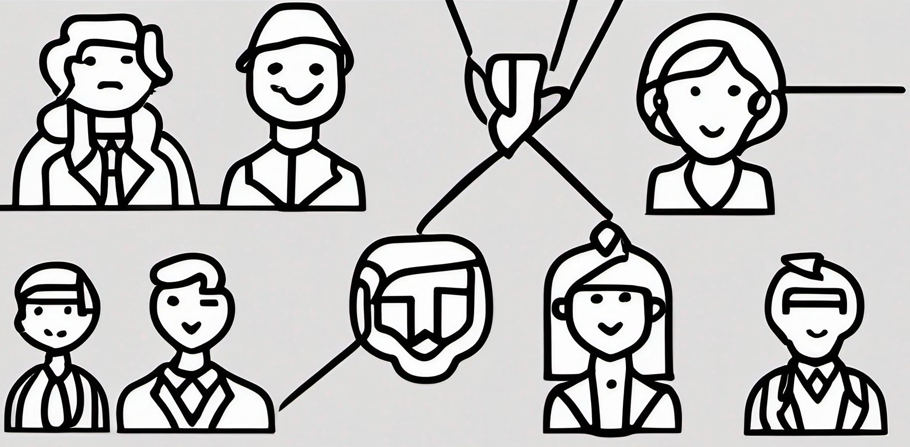
Introduction
Object-Oriented Programming (OOP) is a powerful paradigm that allows developers to model real-world entities as objects with attributes and behaviors. One of the fundamental concepts in OOP is inheritance, where a class can acquire the properties and behaviors of another class. In most programming languages, a class can only inherit from a single parent class, known as single inheritance. However, there are scenarios where a class needs to inherit from multiple parent classes, giving rise to Multiple Inheritance. In this blog post, we'll explore multiple inheritance in OOP, understand its implications, and provide some practical use cases.
The Basics of Inheritance
Before diving into multiple inheritance, let's briefly review single inheritance. In single inheritance, a class (subclass) can inherit from a single-parent class (superclass). This establishes an "is-a" relationship, where the subclass is a specialized version of the superclass.
class Animal:
def speak(self):
pass
class Dog(Animal):
def speak(self):
return "Woof!"
class Cat(Animal):
def speak(self):
return "Meow!"
In this example, both Dog
and Cat
inherit from the Animal
class, allowing them to access the speak()
method, which they override with their specific implementation.
Introducing Multiple Inheritance
Multiple inheritance allows a class to inherit from more than one parent class. This creates a more complex hierarchy, and the subclass will have access to attributes and methods from all its parent classes.
class Flyable:
def fly(self):
return "I can fly!"
class Swimmable:
def swim(self):
return "I can swim!"
class FlyingFish(Flyable, Swimmable):
pass
In this example, we have defined two parent classes, Flyable
and Swimmable
, each with a single method. The FlyingFish
class inherits from both of these classes, making it capable of both flying and swimming.
fish = FlyingFish()
print(fish.fly()) # Output: "I can fly!"
print(fish.swim()) # Output: "I can swim!"
Method Resolution Order (MRO)
When a class inherits from multiple parent classes, the order in which the parent classes are mentioned in the inheritance list matters. This order determines the Method Resolution Order (MRO) when searching for attributes or methods in the subclass.
Python uses the C3 Linearization algorithm to calculate MRO. The __mro__
attribute or the mro()
method can be used to view the MRO of a class.
class A:
def method(self):
print("A's method")
class B(A):
def method(self):
print("B's method")
class C(A):
def method(self):
print("C's method")
class D(B, C):
pass
d = D()
d.method()
print(D.__mro__) # Output: (<class '__main__.D'>, <class '__main__.B'>, <class '__main__.C'>, <class '__main__.A'>, <class 'object'>)
In this example, the D
class inherits from both B
and C
. As per the MRO, Python will first look for the method
in class B
, then in class C
, followed by class A
.
Use Cases of Multiple Inheritance
Graphical User Interfaces (GUIs): In GUI frameworks, a widget can inherit from multiple classes to combine functionalities. For instance, a button widget may inherit from classes that provide button behavior and classes that give it visual styling.
Mixins and Traits: Mixins are classes that are not meant to be instantiated but are used to provide additional functionalities to other classes. Multiple inheritance allows for creating reusable and modular mixins.
class Loggable:
def log(self, message):
print(f"Log: {message}")
class WithTimer:
def timer(self):
import time
start_time = time.time()
yield
print(f"Execution time: {time.time() - start_time}s")
class MyClass(Loggable, WithTimer):
def process(self):
with self.timer():
# Some time-consuming operation
self.log("Processing completed.")
Game Development: In games, multiple inheritance can be used to model game objects with various behaviors, such as a character that is both a "player" and an "enemy."
Plugins and Extensions: Multiple inheritance can facilitate the creation of plugins or extensions that add specific features to existing classes.
class Plugin:
def execute(self):
pass
class MyPlugin(Plugin):
def execute(self):
print("My plugin is running.")
class MyCustomClass(MyPlugin):
pass
obj = MyCustomClass()
obj.execute() # Output: "My plugin is running."
Conclusion
Multiple inheritance in OOP allows for creating more complex class hierarchies and enables classes to inherit features from multiple parent classes. While it brings versatility to your design, it's essential to use it judiciously and consider the method resolution order to prevent any confusion. Understanding multiple inheritance allows you to unlock new possibilities and develop more flexible and feature-rich object-oriented applications. Whether building GUIs, designing mixins, developing games, or creating plugins, multiple inheritance can be a valuable tool in your OOP toolkit.
Happy coding!
Subscribe to my newsletter
Read articles from Kralhex directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
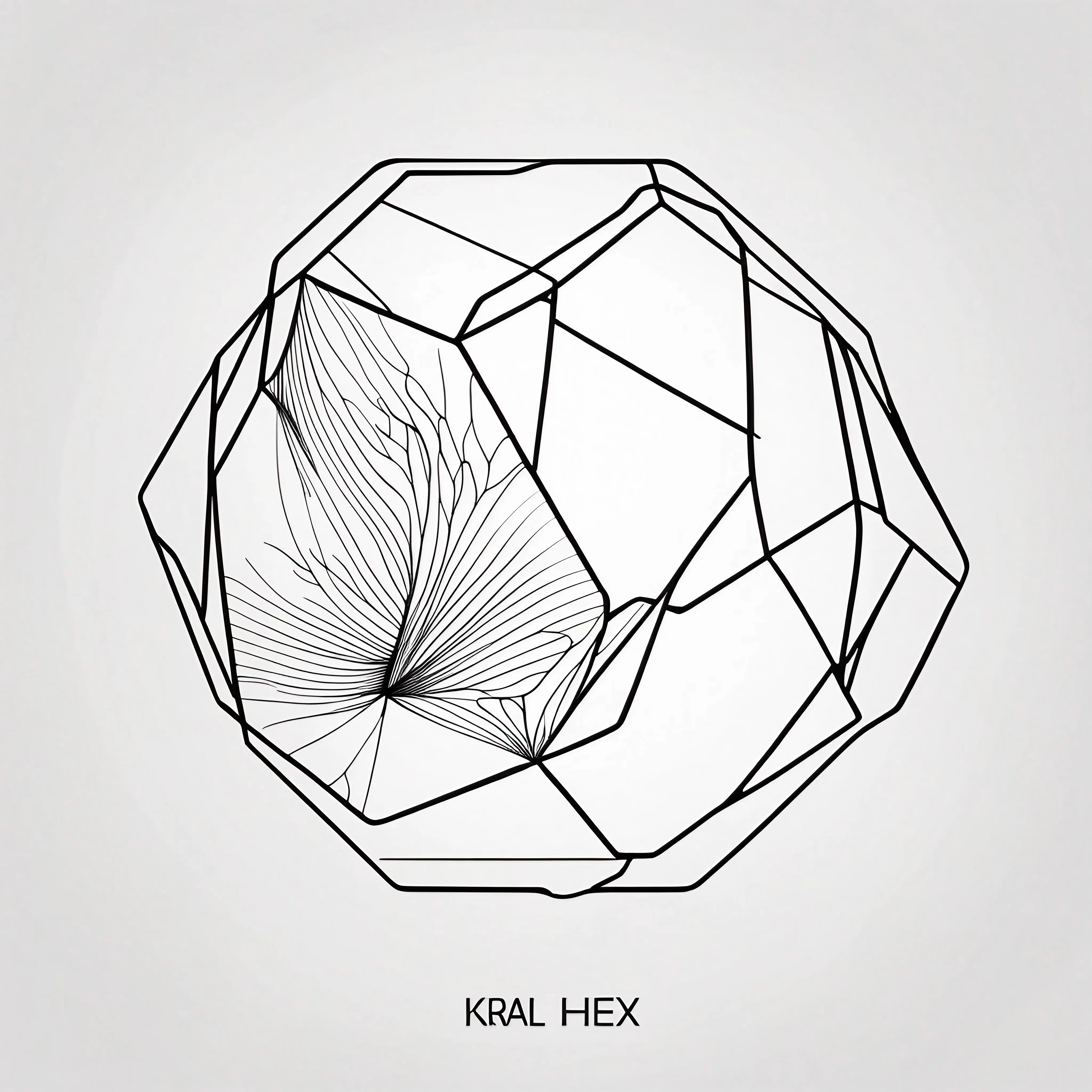
Kralhex
Kralhex
Over 6 years of total software development experience as a freelancer and a company employee. Everything I know, and will learn, is here.