10 Essential JavaScript Concepts to Master Before Diving into React
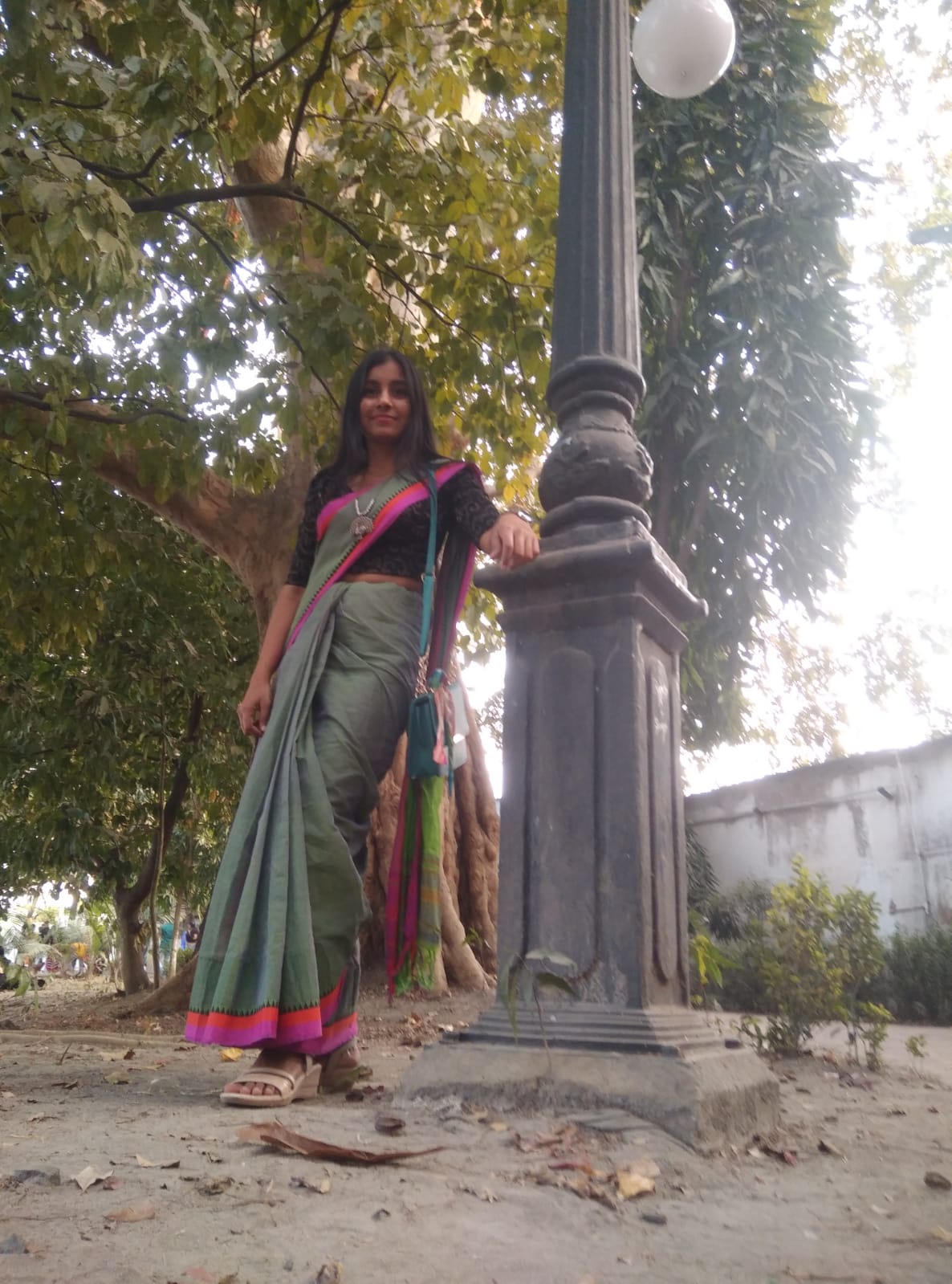
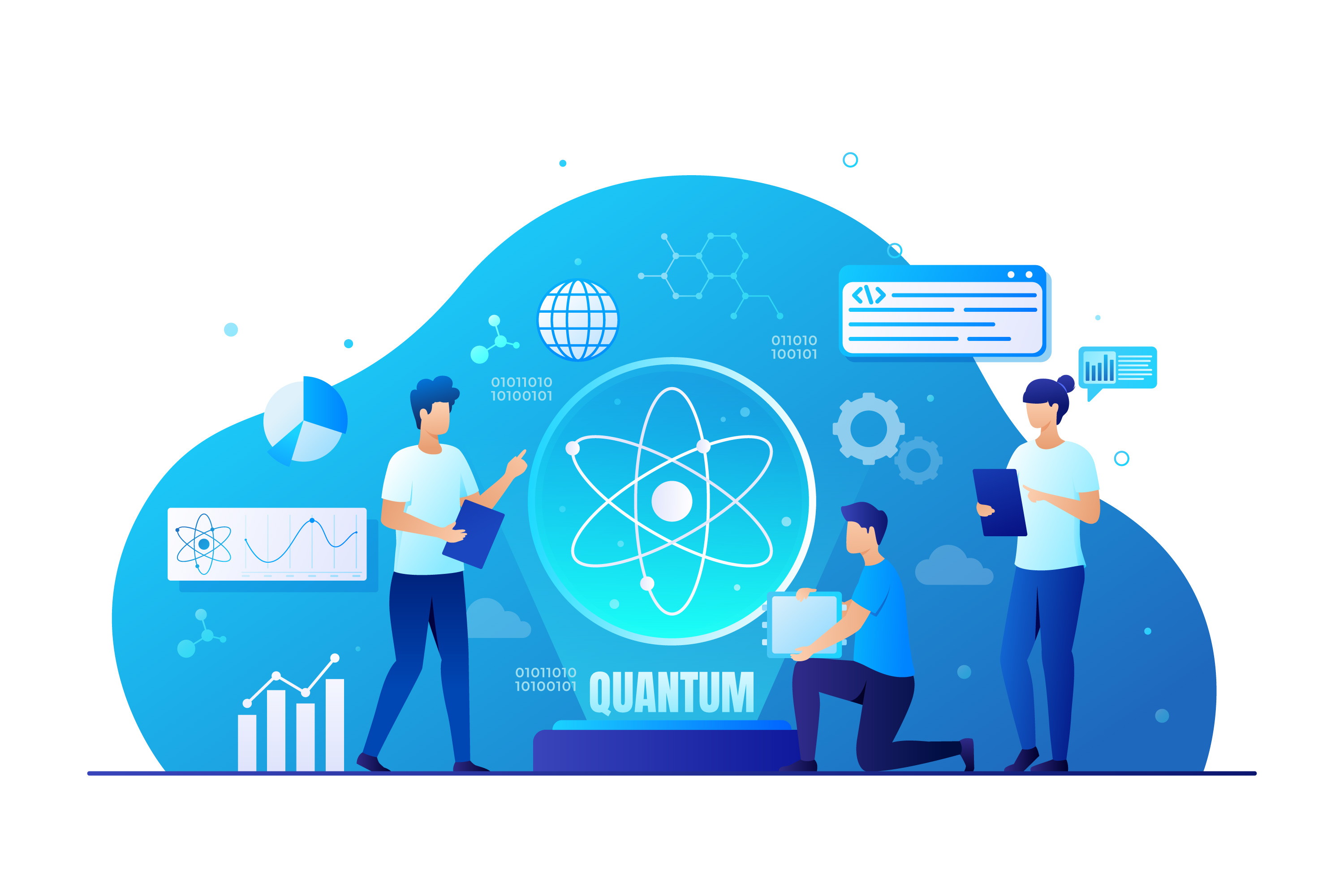
Hey there! Are you excited to dive into the exciting world of React, the popular JavaScript library for building user interfaces, that offers a powerful and efficient way to create Dynamic web applications
. But wait, are you wondering what a dynamic website is? Well, a dynamic web application is a type of website that can change and update its content in real-time based on user interactions and data changes, unlike static websites that display fixed contents.
However, before diving into React, make sure you have a solid grasp of JavaScript basics. Without a solid foundation, you may struggle a lot to write or understand others' code efficiently. So, take your time to learn the fundamentals before you begin your React journey. To help you out, here are Ten must-know JavaScript concepts
that you should be aware of:
1. Modules
React is all about building components. Each component is defined in a separate file, and to access code defined in one file from another, we use import and export operations. However, for beginners, understanding how to connect these separate files can be a great hurdle. This is where JavaScript modules come to help.
JavaScript modules allow us to organize code into separate files and control their accessibility. With the "import"
statement, we can bring code from one file into another, making it available for use. The "export"
statement lets us expose specific functions, objects, or components from a file, enabling other files to use them.
here's an example-
// math.js
// Export the function for other files to use
export const addNumbers = (num1, num2) => {
console.log("Hello from math.js");
return `Sum of ${num1} and ${num2} is: ` + (num1 + num2);
};
// result.js
// Import the addNumbers function from the "math.js" module to use it
import { addNumbers } from "./math.js";
// Call the addNumbers function with arguments 4 and 6, and print the result
console.log(addNumbers(4, 6));
/*Output
[
Hello from math.js //called from rusult.js
10
] *
2. Arrow Functions and Anonymous function
Both Arrow functions and Anonymous functions are extensively used in React. Arrow functions allow us to write normal/regular JavaScript functions with shorter syntax.
On the other hand, Anonymous functions are functions without a specific name. They are widely used in React to handle events, as they allow for inline function definitions.
For example-
// Normal function
// Takes a parameter 'message' and returns it
function normalFunction(message) {
return message;
}
// Calling the normal function with a message and logging the result to the console
console.log(normalFunction("Hey, I'm a normal function"));
// Arrow function
// Takes a parameter 'message' and returns it
const arrowFunction = message => message;
// Calling the arrow function with a message and logging the result to the console
console.log(arrowFunction("Hey, I'm an arrow function"));
//Anonymous function
<button onclick="(() => { alert('I am an anonymous function'); })()">click me</button>
//when you click the button, it will display the alert message "I am an anonymous function"
3. Ternary operator (Conditional operator)
While you all already be familiar with using if-else conditions but many of you might not be aware of the ternary operator, which you'll find yourself using extensively in React. It's nothing but a simple shorthand version of an if-else statement that you should be aware of. This handy ternary operator will become your best friend when dealing with conditional logic in your React applications.
here's an example-
//using normal if else statement
const isLogged = true;
if(isLogged == true){
console.log("Welcome");
}
else{
console.log("Please log in");
}
//Output: Welcome
//using ternary operation
const isLogged = true;
isLogged ? document.write("Welcome") : alert("Please log in");
//Output: Welcome
4. Scoping
Scoping defines how expressions and values are visible and accessible in different parts of the code. JavaScript has three main types of scoping: Global scope, Functional scope, and Block scope.
1. Global scope
allows variables declared outside any function or block to be accessible from anywhere in the code.
// Global scope
var globalVar = "You can access me anywhere as I'm a global variable";
const func = () => {
console.log(globalVar); //Accessible from inside the function
};
console.log(globalVar) //Assessible from outside the function
2. Functional Scope
confines variables declared within a function to be only accessible within that function and not outside of it.
//Functional scope
const func = () => {
const functionalVar = "You can access me only inside this function" ; //'functionalVar' is accessible only within this function
console.log(functionalVar);
}
console.log(functionalVar) //This would result in an error as 'functionalVar' is not accessible here
3. Block scope
restricts the visibility of variables to the block where they are declared, ensuring they cannot be accessed outside that block.
//Block scope
const message = "I'm a global variable";
const func = ()=>{
if(message)
{
let newMessage = "I'm a block variable you can access me only inside this if satement";
console.log(newMessage) // Accessible as it is define in local scope
console.log(message) //Accessible as defined globally
}
console.log(message)// Accessible as defined globally
console.log(newMessage) // This would result in an error because 'newMessage' is defined in the local scope of the if statement
}
func()
5. Destructuring
Destructuring is another important concept in JavaScript that makes working with arrays and objects easier. Think destructuring like unwrapping a gift!
It helps us easily take out multiple values from arrays or objects and put them into separate variables. Cool, right?
Let's see some examples-
// Array Destructuring
const studentDetails = ["Kajal", 21, "B.Tech"];
//Not a good way - accessing each elements using indexes
const studentName = studentDetails[0];
console.log(studentName); //Output: Kajal
//A better way - destructuring the array elements into separate variables at once
const [name, age, course] = studentDetails;
console.log(name, age, course);
//Output: Kajal 21 B.Tech
//object Destructuring
const studentDetails = {
name: "Kajal",
age: 20,
course: "B.Tech"
};
//Not the best way - accessing each property using '.'
console.log(studentDetails.name); //Output: Kajal
//A better way- using object destructuring to extract properties at once
const {name, age, course} = studentDetails;
console.log(name, age, course);
//Output: Kajal 20 B.Tech
Note - These are just a few examples that are not enough, visit the official sites to learn these concepts in detail.
6. Rest and Spread operator
In React, the spread and rest operators (...) are frequently used. Spread allows us to easily copy, compose and merge elements from an array or properties from an object to another array or object, making duplication and merging of data simple. On the other hand, the rest operator is used to gather multiple function arguments and bundle them together into an array.
here are examples-
//rest operator
const func = (...numbers)=>{
//When the function is called all the arguments passed to the function are collected into the 'number' array
console.log(numbers);
};
func(2,4,5,6,7)
//Output: [2, 4, 5, 6, 7]
// spread operator
const num = [2,3,4,5,6];
const num2 =[4,6,7,8,0];
//Merge the array using the spread operator
const mergenum=[...num , ...num2];
console.log(mergenum); //Output: [2,3,4,5,6,4,6,7,8,0]
//object
const person = {name:"John", age:30};
//It copies the properties from 'person' an adds a new property 'job'
const updatedPerson = {...person, job: "programmer"};
console.log(updatedPerson);
//Output: {name: "John", age: 30, job: "programmer"}
7. Array methods
Array methods in JavaScript are pre-built functions designed to work with Iterables like arrays and objects, enabling easy data manipulation. Understanding all array methods like map, filter, find, reduce, split, and more is crucial, especially when working with React applications. Among them, .map() is one of the most frequently used methods, commonly used to render lists of data to the DOM.
Example -
//.Map()
const persons = [
{ name: "John", age: 30 },
{ name: "Liza", age: 25 },
{ name: "Bob", age: 18 }
];
const IsAdult = persons.map(person => {
return {
...person,
status: person.age >= 20 ? "Adult" : "Young" //check each person's age and set the status accordingly
}
})
console.log(IsAdult);
/*Output
[
{ name: 'John', age: 30, status: 'Adult' },
{ name: 'Liza', age: 25, status: 'Adult' },
{ name: 'Bob', age: 18, status: 'Young' }
] */
8. Synchronous and Asynchronous JavaScript
In synchronous (sync) JavaScript
, each line of code is sequentially executed one after the other so, the next line of code waits for the current line to finish executing before proceeding to the next one. If any task takes a long time to complete, it will hold up the execution of the following code until it's done.
const names=["John","Liza","Bob"]
const syncFunc=(...name)=>{
// This line will be printed before the loop starts executing
console.log("Executuion start");
// Then the forEach loop will execute
name.forEach(val=>console.log(val));
// This line will be printed after the loop is done executing
console.log("Execution done");
};
syncFunc(...names);
/*output:-
Executuion start
John
Liza
Bob
Execution done
*/
In asynchronous (async) JavaScript
, certain tasks that may take some time to complete are handled differently. Instead of waiting for the tasks to finish like synchronous JS, the code continues executing other tasks while waiting for a time-consuming operation to complete. When the task is done, It returns and executes the result. This keeps the program responsive, without getting stuck. Asynchronous code is often used for tasks like fetching data from the database, reading files, or waiting for user input.
const names=["John","Liza","Bob"]
const AsyncFunc=(...name)=>{
// This line will be printed first
console.log("Executuion start")
//setTimeout is async, so it will stop the execution for 5000 milliseconds (5 seconds)
setTimeout(() => {
setTimeout(()=>{
name.forEach(val=>console.log(val))
},5000)
// This line will print just after the first line, it won't wait for the setTimeout to finish
console.log("Execution done")
}
AsyncFunc(...names)
/* Output:-
Executuion start
Execution done
John
Liza //after 5s john liza and Bob will execute//
Bob
*/
9. Callback
A callback is a function passed as an argument to another function in JavaScript. It specifies what should happen after a particular task is completed. Callbacks are commonly used for managing asynchronous tasks, providing flexibility in handling the order of execution.
//Fetch data from the server
function fetchData(callback) {
setTimeout(() => {
const data = { message: "Data received from server!" };
callback(data);
}, 5000);
}
//Read file
function readData(callback) {
setTimeout(() => {
const content = "This is the file content.";
callback(content);
}, 1000);
}
// Callback function for handling fetched data
fetchData((data) => {
console.log(data.message);
// Callback function to read the file content after data fetched,
readData((content) => {
console.log(content);
});
});
/* Output:-
Data received from server!
This is the file content.
*/
10. Promises
When you request data, there are two possible outcomes: it might be fulfilled (successful) or not. In this situation, we use a Promise, which can be in one of three states: "Pending," "Fulfilled," or "Rejected."
Suppose you are requesting data from the server. The Promise starts in the "Pending" state, indicating that the operation is ongoing, and the Promise is waiting for the server to respond. If the server successfully fulfills the request and provides the data, the Promise enters the "fulfilled" state. It then invokes the .then()
method, allowing you to handle the data.
However, if there is an error or the request is not successful, the Promise goes into the "Rejected" state. In such situations, it invokes the .catch()
method, which allows you to catch and handle the error.
// URL for fetching user data from the server
const UserData = "https://random-data-api.com/api/v2/users";
// fetch() function allows us to fetch data from the server , which returns a Promise
const data = fetch(UserData);
// if the promise resolved it will handel by .then() method
data.then((fetchUserData) => {
// Parse the response data as JSON using .json() method, which also returns a Promise
return fetchUserData.json().then((finalData) => console.log(finalData));
}).catch(() => {
// If there is an error during the fetch, then it will handel by .catch() method
console.log("Opps! there are some error while fetchig the data.");
});
/* Output:-
{
id: 5499,
uid: '18f5c4e2-42f3-40b2-a6b3-1163c14732aa',
password: 'f0y4HvTj62',
first_name: 'Nanci',
last_name: 'Quitzon',
username: 'nanci.quitzon',
}
*/
Last but not least - Handling Events
Events are actions that happen on a web page, such as clicking a button, submitting a form, or typing in a text box. It allows to write event handlers, which are special functions that respond to these actions. When an event occurs, the corresponding event handler is called, and you can perform specific tasks or actions based on that event.
for example -
//Click event
<button onclick="(() => { alert('This is a click event'); })()">click me</button>
//when you click the button, it will display the alert message "I am an click event"
//output- This is a click event
Thank you for taking the time to read my blog! I hope you enjoyed the explanations and found them helpful. Please feel free to leave your feedback. Have a fantastic day! ๐๐
Subscribe to my newsletter
Read articles from Rinki Shaw directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
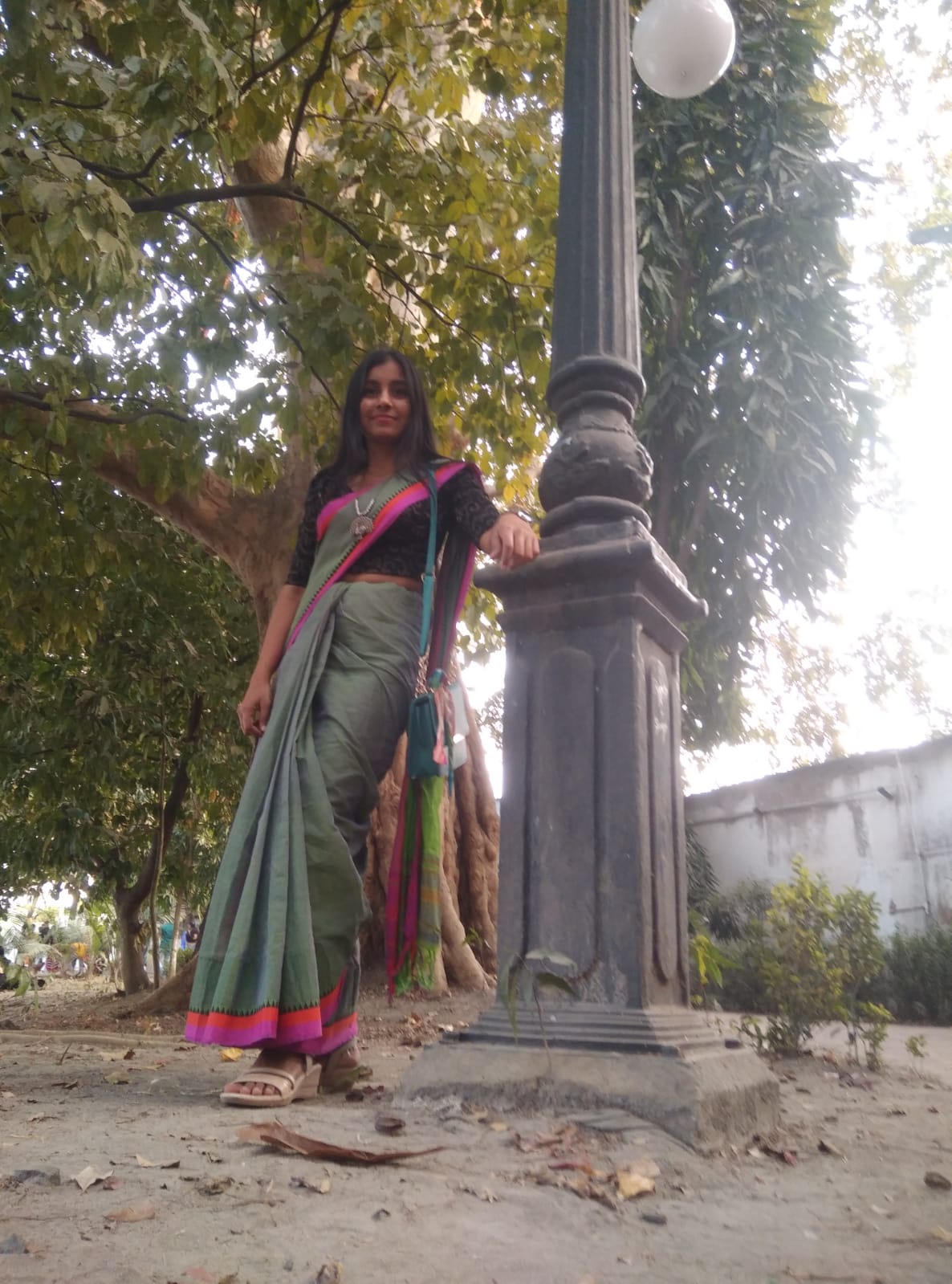
Rinki Shaw
Rinki Shaw
Hi there! I'm a passionate frontend developer exploring backend work too. I enjoy challenges and love learning new technologies. I'm eager to showcase my skills and learn from industry experts.