What is the ternary operator in JavaScript?
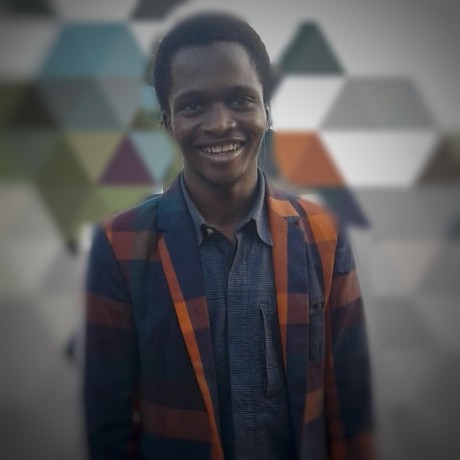
The Conditional (ternary) is a shorthand way of writing simple if-else statements in JavaScript. It's denoted by the ? :
syntax and takes the following form:
condition ? expressionIfTrue : expressionIfFalse;
Here's how it works:
The
condition
is an expression that evaluates to a boolean value (true
orfalse
).If the
condition
is true, theexpressionIfTrue
will be evaluated and returned as the result of the whole expression.If the
condition
is false, theexpressionIfFalse
will be evaluated and returned as the result.
Here's an example of using the conditional operator:
const age = 20;
// Using the conditional operator to check if age is greater than or equal to 18
const message = age >= 18 ? "You are an adult" : "You are a minor";
console.log(message); // Output: "You are an adult"
In this example, if age
is greater than or equal to 18, the message "You are an adult" will be assigned to the message
variable. Otherwise, the message "You are a minor" will be assigned.
The conditional operator is a concise and handy way to handle simple conditional expressions in JavaScript. However, for more complex logic or multiple conditions, using regular if-else statements might be more readable and maintainable.
Subscribe to my newsletter
Read articles from Agbeniga Agboola directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
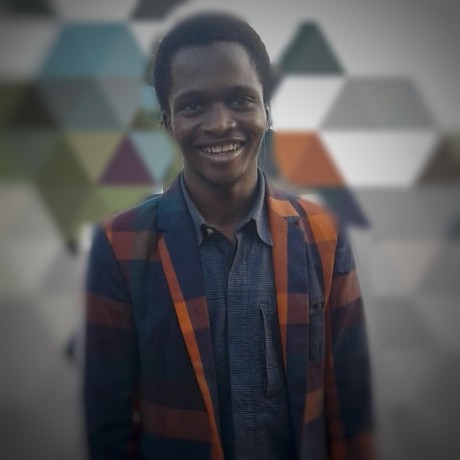