Unveiling Hidden Gems: Lesser-Known JavaScript Features

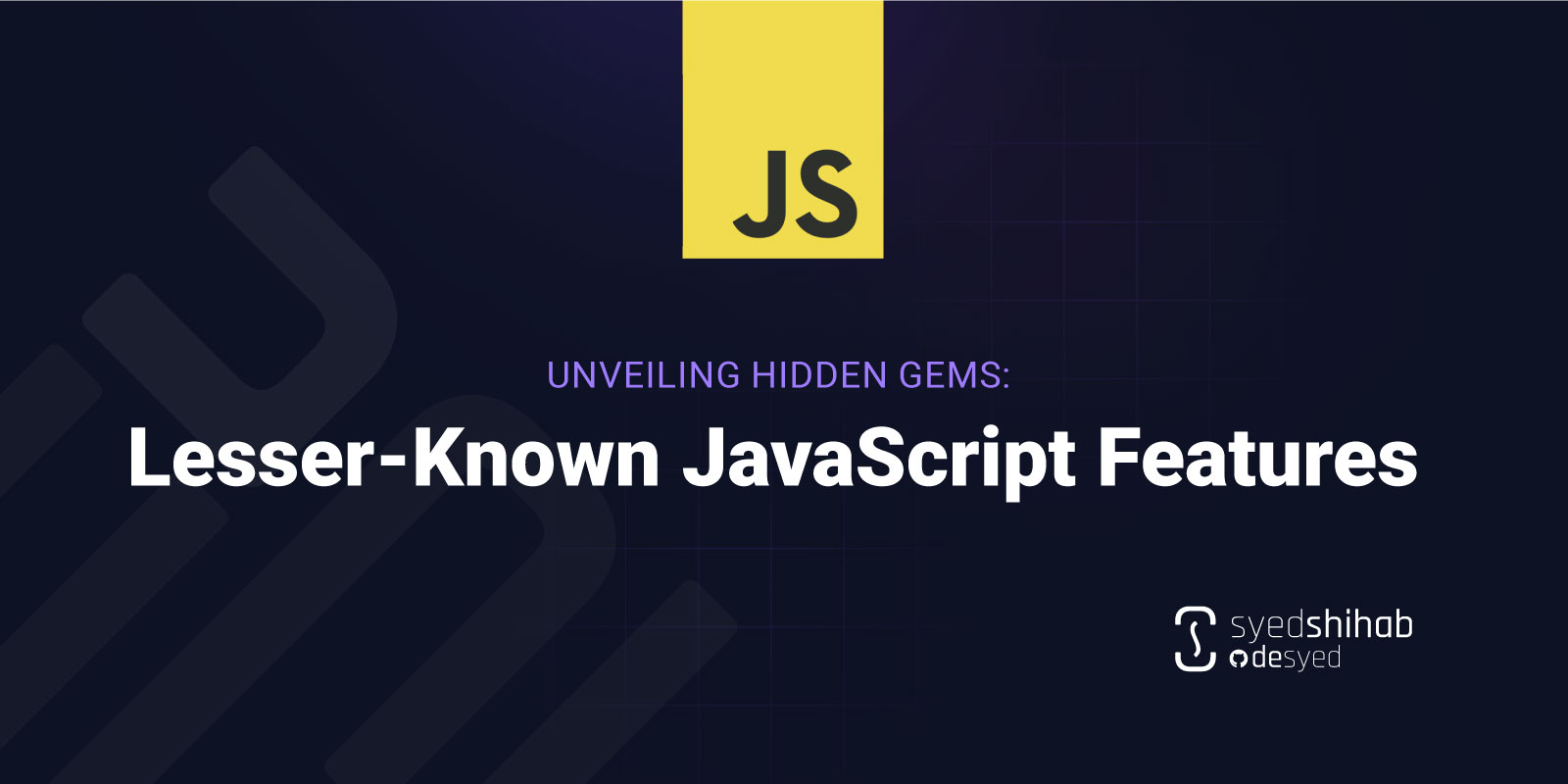
JavaScript, the ubiquitous programming language powering the interactive web, has grown exponentially over the years, evolving from a simple scripting language to a versatile and powerful tool for developers worldwide. While most of us are familiar with the language's essential and widely-used features, there exists a treasure trove of lesser-known, often overlooked, and underutilized JavaScript functionalities that can unlock new possibilities and boost our coding efficiency.
In this article, we embark on a journey of discovery, delving deep into the realms of JavaScript to unearth these hidden gems. From uncommon methods to rarely-explored syntax, we'll shine a light on the obscure corners of JavaScript, revealing their potential to elevate our coding practices to new heights.
The arguments Object
Every function in JavaScript has access to an arguments
object which contains all the arguments passed to the function. This object is array-like, meaning it has a length
property but you cannot use array methods on it directly.
You can use the arguments
object when you want a function that can accept a variable number of arguments.
function sum() {
let total = 0;
for (let i = 0; i < arguments.length; i++) {
total += arguments[i];
}
return total;
}
sum(1, 2, 3); // 6
The with Statement
The with
statement is used to provide an object as a scope for finding properties. However, it is generally not recommended to use with
as it can cause performance issues and bugs.
const animal = {
name: 'bird',
age: '5 days'
}
with (animal) {
// code here has access to obj's properties
console.log(`This is ${name} and it is ${age} old`)
}
// This is bird and it is 5 days old
The in
Operator
The in
operator checks if a property exists in an object or its prototype chain.
const person = {
name: 'John',
age: 30,
city: 'New York'
};
console.log('name' in person); // true
console.log('gender' in person); // false
Keep in mind that the in
operator does not distinguish between properties with a value of undefined
and properties that do not exist in the object. It only checks for the existence of the property name. To distinguish between these cases, you can use the hasOwnProperty()
method:
Functions as Properties
Functions are a special type of object, which means they can have properties just like any other object. Since functions are objects, you can assign properties to them directly.
Here's an example of adding properties to a function:
function greet() {
console.log('Hello!');
}
// Adding properties to the greet function
greet.language = 'English';
greet.year = 2023;
// Accessing the properties of the greet function
console.log(greet.language); // "English"
console.log(greet.year); // 2023
In this example, we have a function named greet
, which we define with the function
keyword. After defining the function, we can assign properties to it directly, as shown by greet.language
and greet.year
.
Keep in mind that adding properties to functions is not a common practice, as functions are usually used to encapsulate behavior rather than data. However, in some cases, it can be helpful to attach metadata or additional information to functions. For example, some libraries or frameworks might add properties to functions to store configuration options or other relevant data.
Void Operator
The void
operator in JavaScript is a unary operator that discards the return value of an expression and always evaluates to undefined
. It is typically used when you want to perform an action without affecting the current page or navigating to a new URL.
The syntax of the void
operator is as follows:
void expression
The expression can be any valid JavaScript expression, and the void
operator will return undefined
regardless of the value of the expression.
Example:
let result = void 42; // Evaluates the expression 42, but the result is always undefined
console.log(result); // undefined
Constructor Brackets are optional
Constructor brackets (parentheses) are optional when calling a constructor function using the new
keyword. If a constructor function doesn't require any arguments, you can omit the parentheses when instantiating an object.
Here's an example of a constructor function with no arguments:
// constructor with brackets
const date = new Date();
// constructor without brackets
const date = new Date;
+ Plus Operator
The plus operator (+
) in JavaScript has various additional functionalities beyond addition and string concatenation. Here are some additional features and use cases of the plus operator:
+'5.06' // returns 5.06
+'-5' // returns -5
+'0xFF' // returns 255
+true // returns 1
+false // returns 0
+null // returns 0
+'abc' // returns NaN
+dateObj // returns (timestamp)
+momentObj // returns (timestamp)
!! Bang Bang Operator
The "Bang Bang" operator, also known as the "Double NOT" operator, is a JavaScript idiom used to explicitly convert a value into its corresponding boolean representation. It involves using two consecutive exclamation marks (!!
) before an expression.
The primary purpose of the "Bang Bang" operator is to quickly and explicitly convert a truthy or falsy value to its actual boolean value (true
or false
). It essentially coerces a value to a boolean without changing its truthiness or falsiness.
const value1 = 'Hello';
const value2 = 0;
console.log(!!value1); // Output: true (Truthy value 'Hello' converted to boolean true)
console.log(!!value2); // Output: false (Falsy value 0 converted to boolean false)
The Comma Operator
The comma operator evaluates each expression from left to right and returns the value of the last expression.
example:
let a = 1, b = 2;
// Using the comma operator in variable assignments
let result = (a += 5, b *= 3, a + b); // equivalent to (a += 5), (b *= 3), (a + b)
console.log(result); // 12 (1 + 5 + 2 * 3)
The debugger Statement
The debugger
statement allows you to debug your JavaScript code by setting a breakpoint.
function doSomething() {
debugger; // breakpoint
// ...
}
I have a passion for JavaScript, and I find joy in crafting articles about this language. However, I must admit that writing these articles requires a significant amount of time and effort. If you've found this article enjoyable, I kindly request you to share and recommend it.
Wishing you happiness in your coding journey!
Subscribe to my newsletter
Read articles from Syed Shihab directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Syed Shihab
Syed Shihab
A Javascript Developer.