What is First class function in JavaScript ?
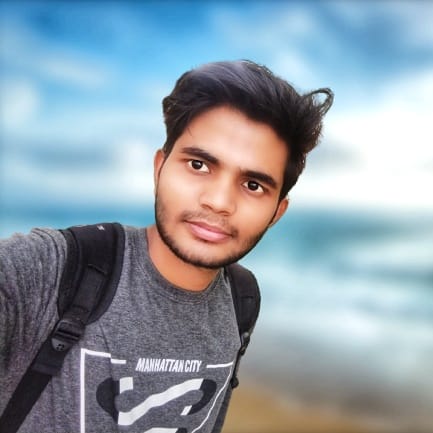
In JavaScript, functions are first-class citizens. This means that functions in JavaScript are treated like any other variable. A first-class function can:
Be stored in a variable:
let myFunction = function() { console.log('Hello World'); } myFunction(); // Outputs: Hello World
Be passed as an argument to another function:
function sayHello() { return 'Hello, '; } function greet(helloFn, name) { console.log(helloFn() + name); } greet(sayHello, 'John'); // Outputs: Hello, John
Be returned from another function:
function outerFunction() { return function innerFunction() { return 'Hello World'; } } let myFunction = outerFunction(); console.log(myFunction()); // Outputs: Hello World
Be stored in data structures such as an array, an object, or a map:
let funcArray = [function(){ return 'Hello '}, function(){ return 'World!'}]; console.log(funcArray[0]() + funcArray[1]()); // Outputs: Hello World! let funcObject = {sayHello: function(){ return 'Hello '}, sayWorld: function(){ return 'World!'}}; console.log(funcObject.sayHello() + funcObject.sayWorld()); // Outputs: Hello World!
These characteristics are what make JavaScript a functional language, since it supports the concept of treating functions as first-class citizens.
Thank you for reading, please follow me on Twitter, i regularly share content about Javascript, and React and contribute to Opensource Projects
Twitter-https://twitter.com/Diwakar_766
Subscribe to my newsletter
Read articles from Diwakar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
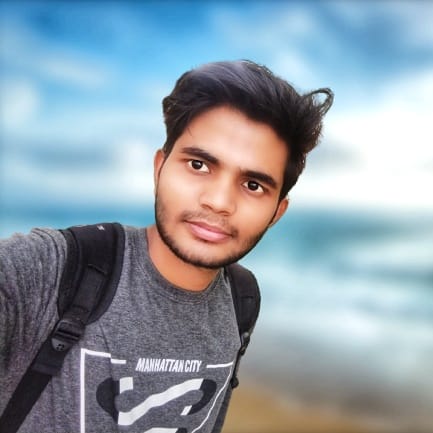
Diwakar
Diwakar
As a passionate developer, I thrive on acquiring new knowledge. My journey began with web development, and I am now actively engaged in open source contributions, aiding individuals and businesses.