Python Decorators Explained: Dive into the Power of Function and Class Modification
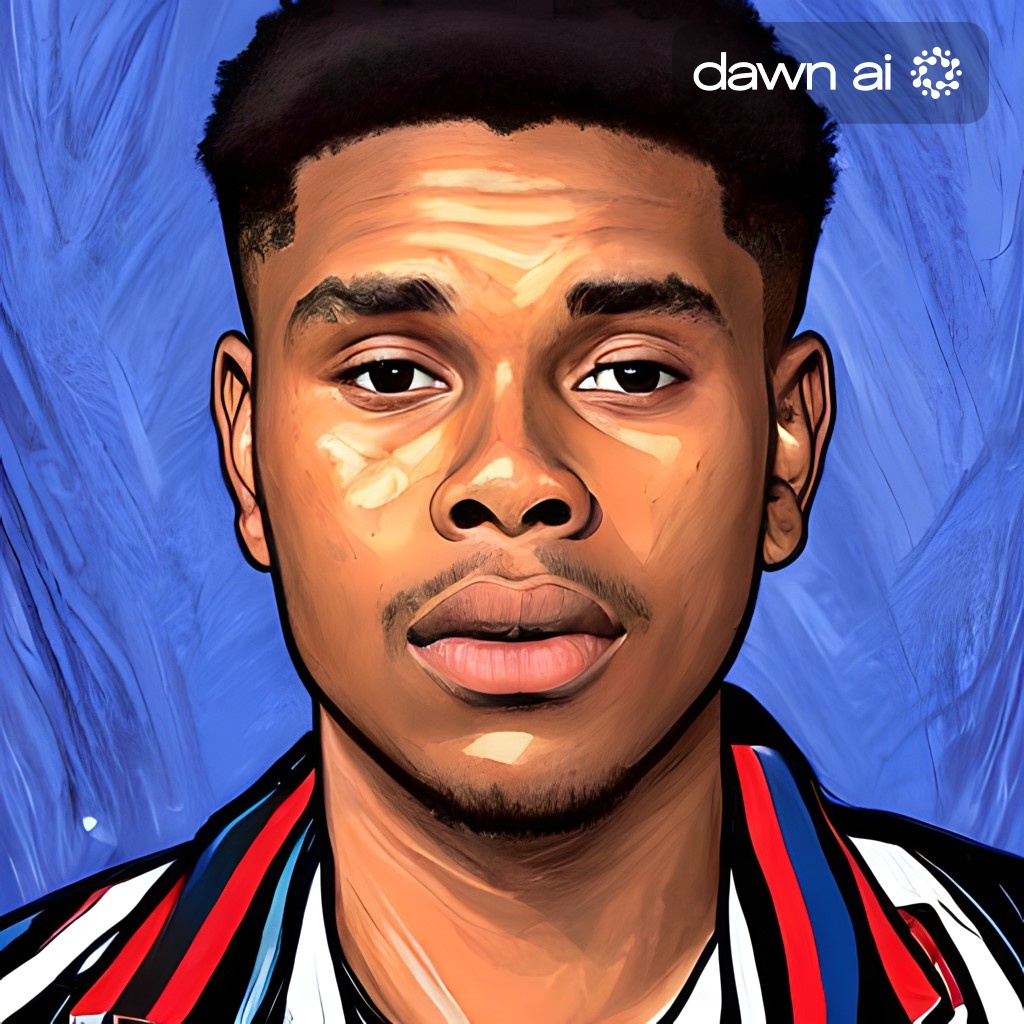
Table of contents
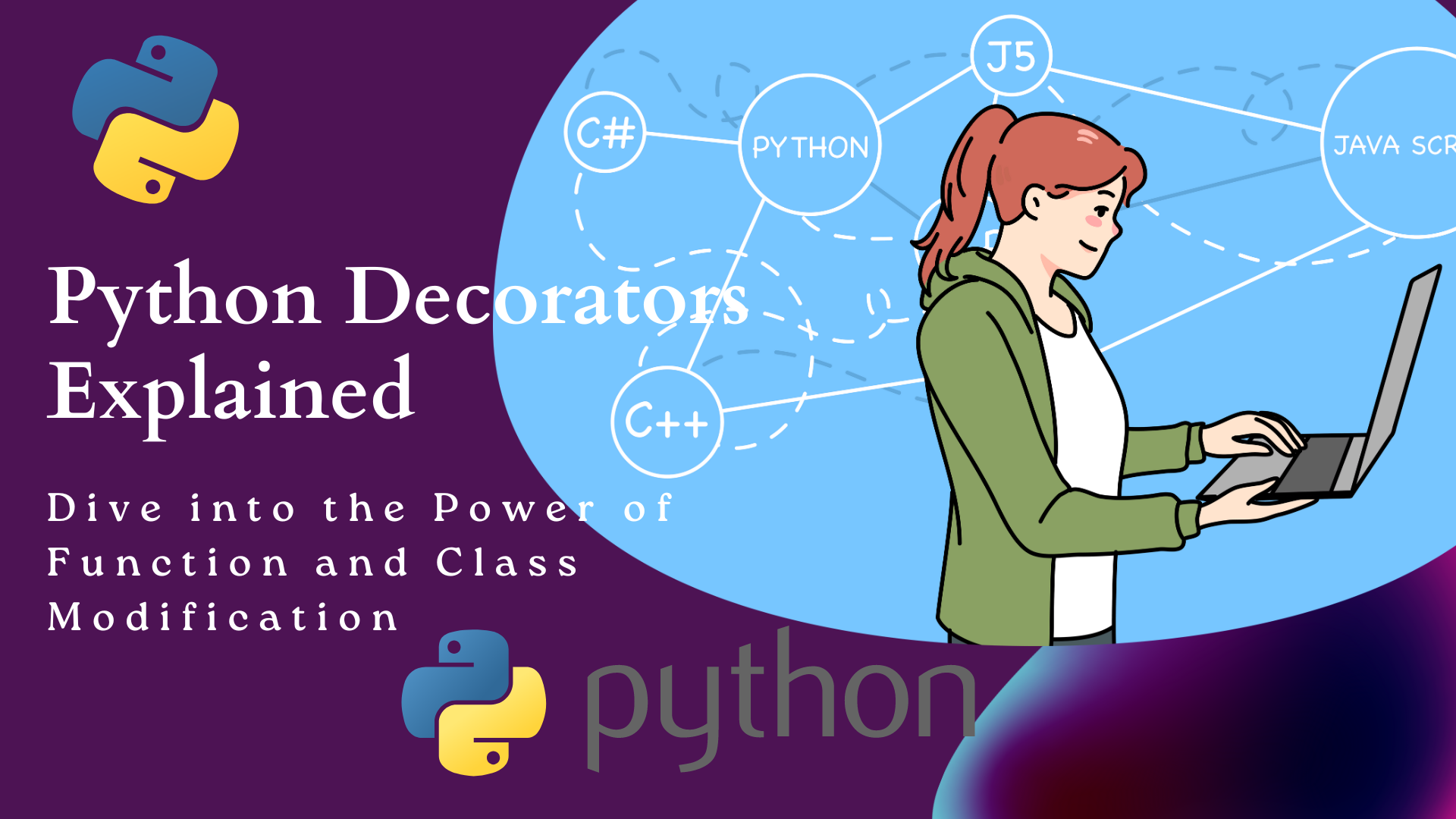
Python is known for its simplicity and readability, but it also provides powerful features that can enhance code functionality. One such feature is decorators, a concept that may seem mysterious to beginners but is widely used by experienced developers. In this article, we will unravel the magic of decorators and show you how they can be used to modify functions and classes. By the end of this journey, you'll have a solid understanding of decorators and how to leverage them in your Python projects.
Prerequisites: Before diving into decorators, it's essential to have a basic understanding of Python programming. Familiarity with the following concepts will be helpful:
Functions and Function Calls: Understand how to define and call functions in Python.
Closures: Familiarity with the concept of nested functions and their scope.
Decorator Syntax: Knowledge of the
@decorator
syntax for applying decorators to functions and classes.Classes: Basic understanding of Python classes and their methods.
What are Decorators? At its core, a decorator is a Python function that enhances or modifies another function or class. Think of decorators like toppings on your favorite pizza. They don't change the fundamental essence of the pizza, but they add extra flavor and functionality to it. Decorators help us keep our code DRY (Don't Repeat Yourself) by enabling us to wrap additional behavior around functions and classes without altering their original code.
Creating a Decorator: To create a decorator, we define a higher-order function - a function that takes another function as an argument and returns a new function. Let's start with a simple example:
def my_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
@my_decorator
def say_hello():
print("Hello, world!")
say_hello()
In this example, we've defined a decorator my_decorator
that takes a function func
as an argument and returns a new function wrapper
. The wrapper
function adds some extra behavior before and after calling the original function, in this case, say_hello
.
Output:
Something is happening before the function is called.
Hello, world!
Something is happening after the function is called.
Imagine you own a restaurant, and the chef prepares the dish (function). The chef brings the dish to the waiter (decorator), who adds a garnish (extra functionality) before serving it to the customer (calling the function).
Using Decorators for Logging: One practical use of decorators is logging. We can create a decorator that logs function calls along with their arguments and returns values. Let's see how:
import functools
def log_function_call(func):
@functools.wraps(func)
def wrapper(*args, **kwargs):
result = func(*args, **kwargs)
print(f"Function '{func.__name__}' called with args: {args}, kwargs: {kwargs}.")
print(f"Returned: {result}")
return result
return wrapper
@log_function_call
def add(a, b):
return a + b
result = add(3, 5)
Output:
Function 'add' called with args: (3, 5), kwargs: {}.
Returned: 8
In this example, we've created the log_function_call
decorator to log information about the function calls and their return values.
Conclusion: Decorators are a powerful tool in Python that allows us to modify functions and classes easily. By creating higher-order functions that wrap around our original code, we can add new features, logging, authentication, and more, all while keeping our code clean and maintainable.
In this article, we've only scratched the surface of what decorators can do. As you continue your Python journey, you'll encounter more complex use cases and scenarios where decorators shine. Embrace the power of decorators, and you'll find yourself writing more elegant and efficient Python code.
Now, it's your turn to sprinkle the magic of decorators into your Python projects and take your code to new heights!
Remember, like any powerful feature, decorators should be used judiciously. Don't overuse them, and make sure your code remains understandable, readable, and maintainable for you and your team.
Happy coding!
Subscribe to my newsletter
Read articles from Jude Nwabueze Echezona directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
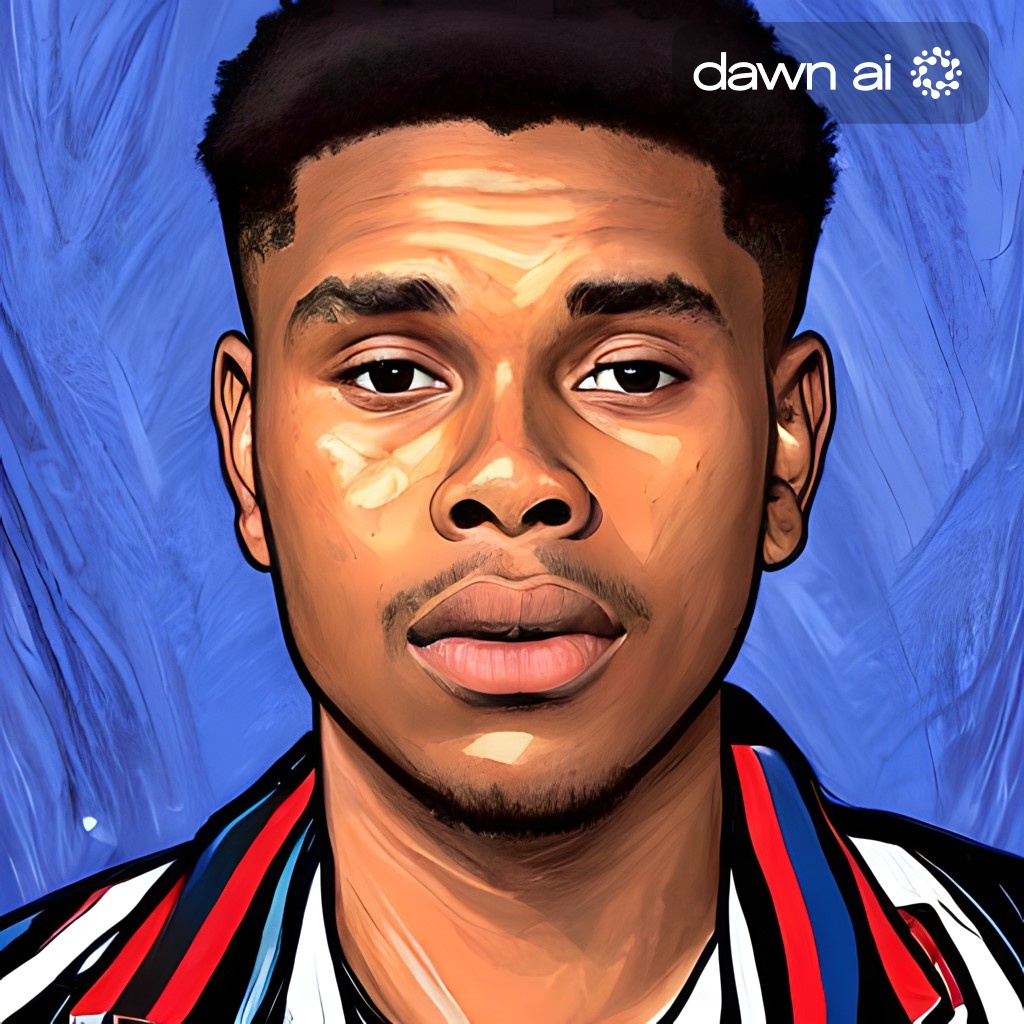
Jude Nwabueze Echezona
Jude Nwabueze Echezona
I'm a technical writer, I excel at translating complex technical concepts into simplified, easy-to-digest content that resonates with developers and non-technical target audiences alike. My strength lies in my ability to create engaging, informative, and well-articulated articles, tutorials, whitepapers, and docs.