Decoding API Communication: Unraveling the Differences between Axios and Fetch for JavaScript Applications
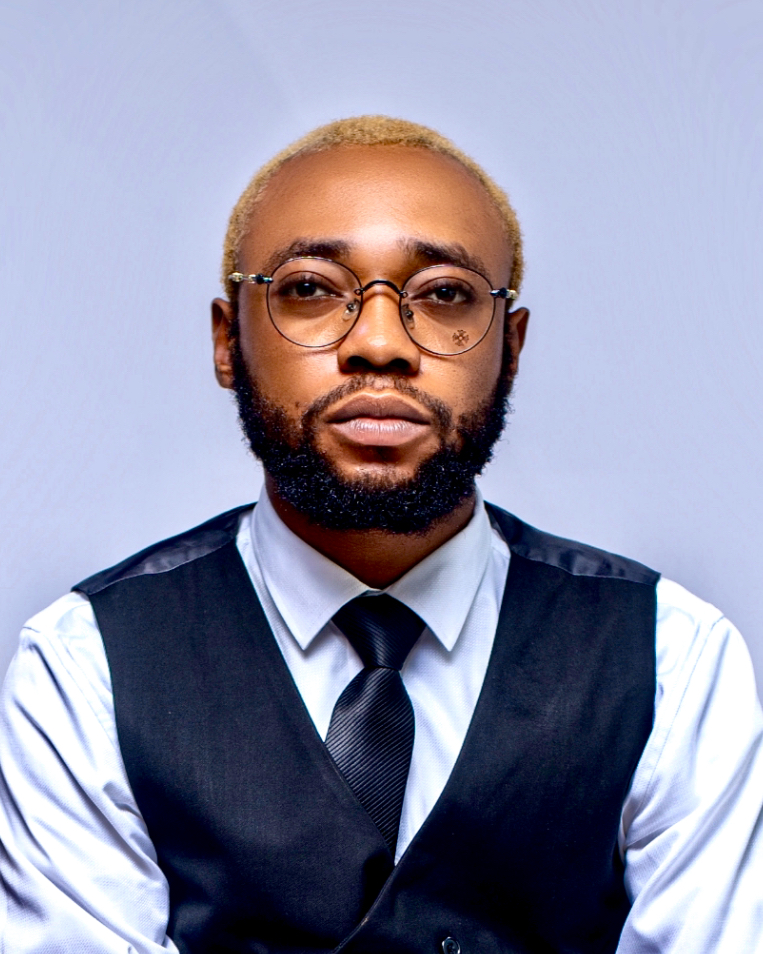
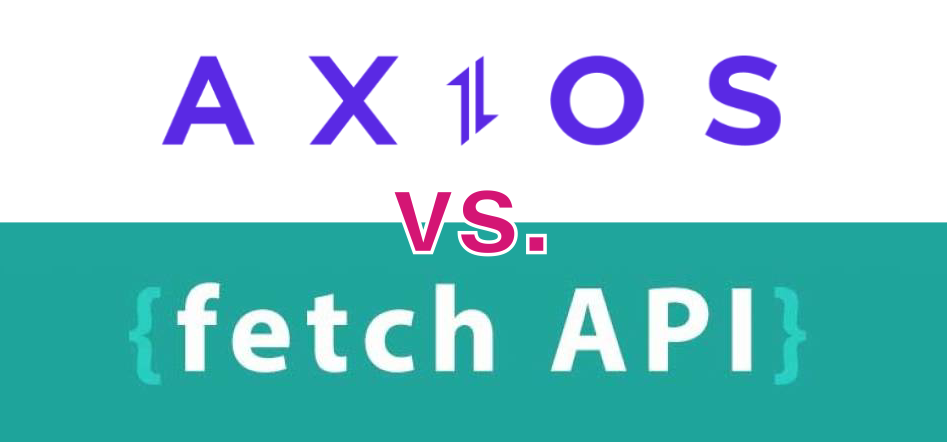
Introduction
In the fast-paced world of web development, efficient communication with APIs is vital for creating dynamic and interactive applications. When it comes to making API calls in JavaScript, developers often have two popular options: Axios and the native Fetch API. Both methods serve the same purpose - retrieving data from APIs - but they differ in implementation and features. In this article, we will embark on a journey to compare these two data fetchers and discover their strengths, weaknesses, and performance differences.
What is Axios?
Axios is a powerful JavaScript library widely used for making HTTP requests. It runs on both client and server-side environments, making it versatile for any project. It provides an easy-to-use interface to send asynchronous HTTP requests to RESTful APIs, and it can handle request and response transformations seamlessly. Axios also includes features like request/response interceptors, automatic JSON parsing, and CSRF protection, making it a developer-friendly choice for handling API calls.
What is Fetch API?
Fetch API is a modern addition to JavaScript that comes natively built into the language, offering a simple and concise way to perform network requests. It is part of the Web Fetch API standard, providing a global fetch()
method that returns a Promise. Fetch API is lightweight, adheres to the newer standards of ES6 Promises, and can handle basic GET and POST requests. However, compared to Axios, Fetch API lacks some advanced features, such as request/response interceptors and automatic request transformations.
Code Samples: Axios vs. Fetch
Let's dive into practical examples to understand how Axios and Fetch differ in implementation and syntax.
Axios Example:
Step 1: Using Axios for a GET request
axios.get('https://api.example.com/data')
.then(response => {
console.log('Axios Response:', response.data);
})
.catch(error => {
console.error('Axios Error:', error);
});
Step 2: Using Axios for a POST request
const data = { username: 'JohnDoe', email: 'johndoe@example.com' };
axios.post('https://api.example.com/users', data)
.then(response => {
console.log('User created:', response.data);
})
.catch(error => {
console.error('Axios Error:', error);
});
Fetch API Example:
Step 1: Using Fetch for a GET request
fetch('https://api.example.com/data')
.then(data => {
console.log('Fetch Response:', data);
})
.catch(error => {
console.error('Fetch Error:', error);
});
Step 2: Using Fetch for a POST request
const data = { username: 'JohnDoe', email: 'johndoe@example.com' };
fetch('https://api.example.com/users', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify(data),
})
.then(data => {
console.log('User created:', data);
})
.catch(error => {
console.error('Fetch Error:', error);
});
Advantages of Axios:
Simplicity and Consistency: Axios provides a consistent API and syntax for handling different types of requests, making it easy to use and maintain.
Advanced Features: Axios offers request/response interceptors, automatic JSON parsing, and CSRF protection out-of-the-box, reducing the need for additional code.
Browser Compatibility: Axios works seamlessly across different browsers and environments, ensuring consistent behavior.
Advantages of Fetch API:
Native Browser Support: Fetch API is built into modern JavaScript environments, reducing the need for external libraries and dependencies.
Lightweight: Fetch API is lightweight and focused on core functionality, making it a good choice for simple projects that don't require advanced features.
ES6 Promises: Fetch API returns Promises, making it easier to work with asynchronous code.
Limitations of Axios and Fetch API:
Size: Axios has a larger bundle size compared to Fetch API, which may be a concern for performance-critical applications.
CORS (Cross-Origin Resource Sharing) Handling: Both Axios and Fetch API are subject to browser CORS restrictions, which can be challenging when making requests to different domains.
Performance Considerations:
When it comes to performance, both Axios and Fetch API are reasonably fast for basic use cases. However, Axios might have a slight edge due to its advanced features that can lead to optimized code and better request/response handling. For most small to medium-sized applications, the performance difference might not be significant enough to be a deciding factor.
Conclusion:
In the end, choosing between Axios and Fetch API largely depends on your project requirements and preferences. If you prioritize simplicity, lightweight solutions, and native browser support, Fetch API could be a suitable option. On the other hand, if you need more advanced features, consistent syntax, and better handling of interceptors and responses, Axios might be the way to go. Always consider your project's scope, performance needs, and the development team's familiarity with the library before making a decision. Regardless of the choice, both Axios and Fetch API enable seamless data fetching, ensuring your web applications can communicate effortlessly with APIs.
Subscribe to my newsletter
Read articles from Obiuwevbi Gospel Jonathan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
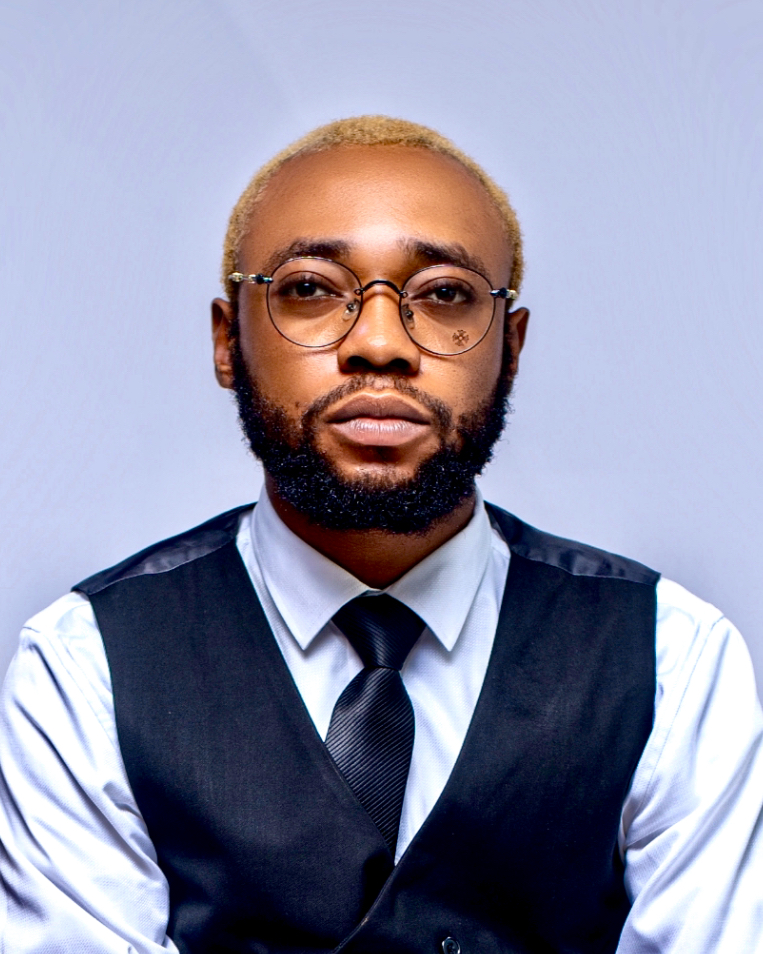
Obiuwevbi Gospel Jonathan
Obiuwevbi Gospel Jonathan
A passionate developer With a solid foundation in the MERN (MongoDB, Express, React, Node.js) stack, I love building exceptional web applications that deliver a seamless user experience. My expertise lies in leveraging the power of React, along with frameworks like Material UI and Tailwind CSS, to create stunning and responsive user interfaces. When it comes to the backend, I specialize in crafting APIs using Node.js. I enjoy architecting efficient and scalable solutions, ensuring smooth communication between the frontend and backend components of an application. As a tech enthusiast, I'm always eager to explore new challenges and push the boundaries of what's possible in the ever-evolving tech world. I thrive on innovative thinking and constantly strive to bring fresh perspectives to my projects.