Spingboot Annotations

Table of contents
- Getting Started
- Spring Annotations
- @RequestHeader Annotation
- @RequestAttribute Annotation
- @Component Annotation
- @Autowired Annotation
- @Qualifier Annotation
- @Primary Annotation
- @Bean Annotation
- @Lazy Annotation
- @Scope Annotation
- @Value Annotation
- @PropertySource Annotation
- @Controller Annotation
- @ResponseBody Annotation
- @RestController Annotation
- @RequestMapping Annotation
- @RequestBody Annotation
- @ResponseBody Annotation
- @RequestParam Annotation
- @GetMapping Annotation
- @PostMapping Annotation
- @PutMapping Annotation
- @DeleteMapping Annotation
- @PatchMapping Annotation
- @PathVariable Annotation
- @ResponseStatus Annotation
- @Service Annotation
- @Repository Annotation
- @Controller Annotation
- @SpringBootApplication Annotation
- @EnableAutoConfiguration Annotation
- @Async Annotation
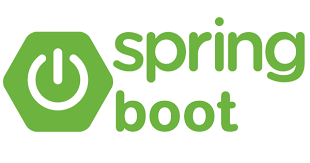
Getting Started
Let's kick off in creating our spring boot Rest API, if you have not yet configured your project please head on to this guide SpringBoot setup for beginners, otherwise let's continue learning.
In The spring boot world, we heavily use a lot of annotations so it will be better to familiarize ourselves with the major annotations before writing the code. The annotations are usually written starting with @ symbol followed by the annotation name for instance @GetMapping, Don't worry we are going to learn all these annotations.
Spring Annotations
@RequestHeader Annotation
It is used to get the details about the HTTP request headers. We use this annotation as a method parameter.
@RequestAttribute Annotation
It binds a method parameter to the request attribute. It provides convenient access to the request attributes from a controller method. With the help of @RequestAttribute annotation, we can access objects that are populated on the server side.
@Component Annotation
The @Component annotation indicates that an annotated class is a “spring bean/component”. It tells the Spring container to automatically create Spring beans.
@Autowired Annotation
Used to inject the bean automatically. The @Autowired annotation is used in constructor injection, setter injection, and field injection
@Qualifier Annotation
Used in conjunction with Autowired to avoid confusion when we have two or more beans configured for the same type.
@Primary Annotation
We use This annotation to give higher preference to a bean when there are multiple beans of the same type.
@Bean Annotation
The annotation indicates that a method produces a bean to be managed by the Spring container. It is usually declared in the Configuration class to create Spring Bean definitions.
@Lazy Annotation
By default, Spring creates all singleton beans eagerly at the startup/bootstrapping of the application context. You can load the Spring beans lazily (on-demand) using @Lazy annotation.
@Scope Annotation
Used to define the scope of the bean. We use @Scope to define the scope of a @Component class or a @Bean definition.
@Value Annotation
Used to assign default values to variables and method arguments. @Value annotation is mostly used to get value for specific property keys from the properties file. We can read spring environment variables as well as system variables using @Value annotation.
@PropertySource Annotation
Spring @PropertySource annotation is used to provide properties files to Spring Environment. Spring PropertySource annotation is repeatable, which means you can have multiple PropertySource on a Configuration class.
@Controller Annotation
This annotation is used to make a class as a web controller, which can handle client requests and send a response back to the client. This is a class-level annotation, which is put on top of your controller class.
@ResponseBody Annotation
The @ResponseBody annotation tells a controller that the object returned is automatically serialized into JSON and passed back into the HttpResponse object.
@RestController Annotation
RestController controller annotation is used for making restful web services. In simple terms, this annotation is used at the class level and allows the class to handle the requests made by the client.
@RequestMapping Annotation
The most common and widely used annotation in Spring MVC. It is used to map web requests onto specific handler classes and/or handler methods.
@RequestBody Annotation
It is used to bind HTTP requests with an object in a method parameter. Internally it uses HTTP MessageConverters to convert the body of the request. in simpler terms, it maps the body of an HTTP request to a defined object.
@ResponseBody Annotation
It binds the method return value to the response body. It tells the Spring Boot Framework to serialize a return an object into JSON and XML format.
@RequestParam Annotation
It is used to extract the query parameters from the URL. It is also known as a query parameter. It is most suitable for web applications.
@GetMapping Annotation
The GET HTTP request is used to get single or multiple resources and @GetMapping annotation for mapping HTTP GET requests onto specific handler methods.
@PostMapping Annotation
The POST HTTP method is used to create a resource and @PostMapping annotation for mapping HTTP POST requests onto specific handler methods.
@PutMapping Annotation
The PUT HTTP method is used to update the resource and @PutMapping annotation for mapping HTTP PUT requests onto specific handler methods.
@DeleteMapping Annotation
The DELETE HTTP method is used to delete the resource and @DeleteMapping annotation for mapping HTTP DELETE requests onto specific handler methods.
@PatchMapping Annotation
The PATCH HTTP method is used to update the resource partially and the @PatchMapping annotation is for mapping HTTP PATCH requests onto specific handler methods.
@PathVariable Annotation
It is used to extract the values from the URI. It is most suitable for the RESTful web service, where the URL contains a path variable.
@ResponseStatus Annotation
Spring Framework annotation that is used to customize the HTTP response status code returned by a controller method in a Spring MVC or Spring Boot application.
@Service Annotation
It is used at the class level. It tells the Spring that the class contains business logic.
@Repository Annotation
It is a class-level annotation. The repository is a DAOs (Data Access Object) that access the database directly. The repository does all the operations related to the database
@Controller Annotation
Used to create Spring beans at the controller layer.
@SpringBootApplication Annotation
Is a core annotation in the Spring Boot framework. It is used to mark the main class of a Spring Boot application. This annotation is a combination of three other annotations: @Configuration, @EnableAutoConfiguration, and @ComponentScan.
@EnableAutoConfiguration Annotation
Enables Spring Boot's auto-configuration feature, which automatically configures the application based on the classpath dependencies and the environment.
@Async Annotation
Can be provided on a method so that invocation of that method will occur asynchronously.
Subscribe to my newsletter
Read articles from Peter Kironji directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Peter Kironji
Peter Kironji
Hello I am Peter Kironji , a software developer passionate about Mobile developer with flutter java and kotlin also do backend development with spring boot on a daily basis. When I am not coding I love reading books, playing games and exploring the world.