finally() in javascript
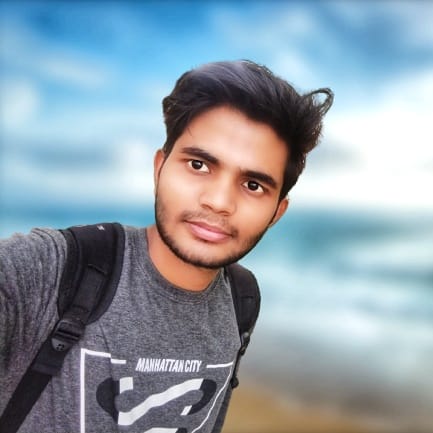
finally
is a key method used within the try-catch
structure in JavaScript. This method is triggered regardless of whether the preceding promise is fulfilled or rejected. Its primary function is to handle final tasks after promise resolution, which might include communicating to the user that their request has been processed. The processing is done regardless of whether the request was successful or unsuccessful.
Here's a basic rundown:
The
try
block contains the code that might throw an exception.The
catch
block contains the code to handle the exception, if one occurs in thetry
block.The
finally
block contains code that will execute after thetry
andcatch
blocks, regardless of whether an exception was thrown or caught.
The primary purpose of the finally
block is to include any cleanup code that must be executed irrespective of whether an error occurs. For example, if you open a file in the try
block, you would close it in the finally
block to ensure the file gets closed, whether an error occurs or not.
Here's an example:
try {
// Code that may throw an exception
throw new Error('This is an error');
} catch (error) {
// Code to handle the exception
console.error('An error occurred: ', error);
} finally {
// Code that gets executed regardless of an exception
console.log('This will always run, error or not.');
}
In this example, an error is thrown inside the try
block. The catch
block catches the error and logs it. Regardless of the outcome (whether an error was thrown or not), the finally
block runs and logs 'This will always run, error or not.' to the console.
It's important to note that finally
can be used without a catch
block. This is particularly useful when you want to run cleanup code, but you don't want to handle the error at that specific part of your code. The error will then propagate up the call stack to the next parent try-catch
, or cause the program to terminate if no other try-catch
is found.
Thank you for reading. I encourage you to follow me on Twitter where I regularly share content about JavaScript and React, as well as contribute to open-source projects. I am currently seeking a remote job or internship.
Twitter: https://twitter.com/Diwakar_766
GitHub: https://github.com/DIWAKARKASHYAP
Portfolio: https://diwakar-portfolio.vercel.app/
Subscribe to my newsletter
Read articles from Diwakar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
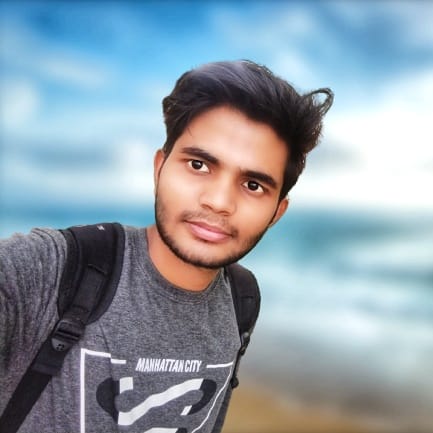
Diwakar
Diwakar
As a passionate developer, I thrive on acquiring new knowledge. My journey began with web development, and I am now actively engaged in open source contributions, aiding individuals and businesses.