Smooth UX with Flutter Debounce
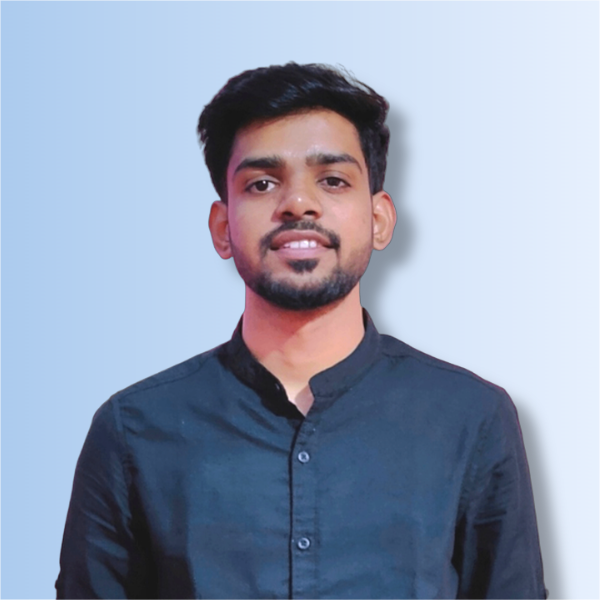
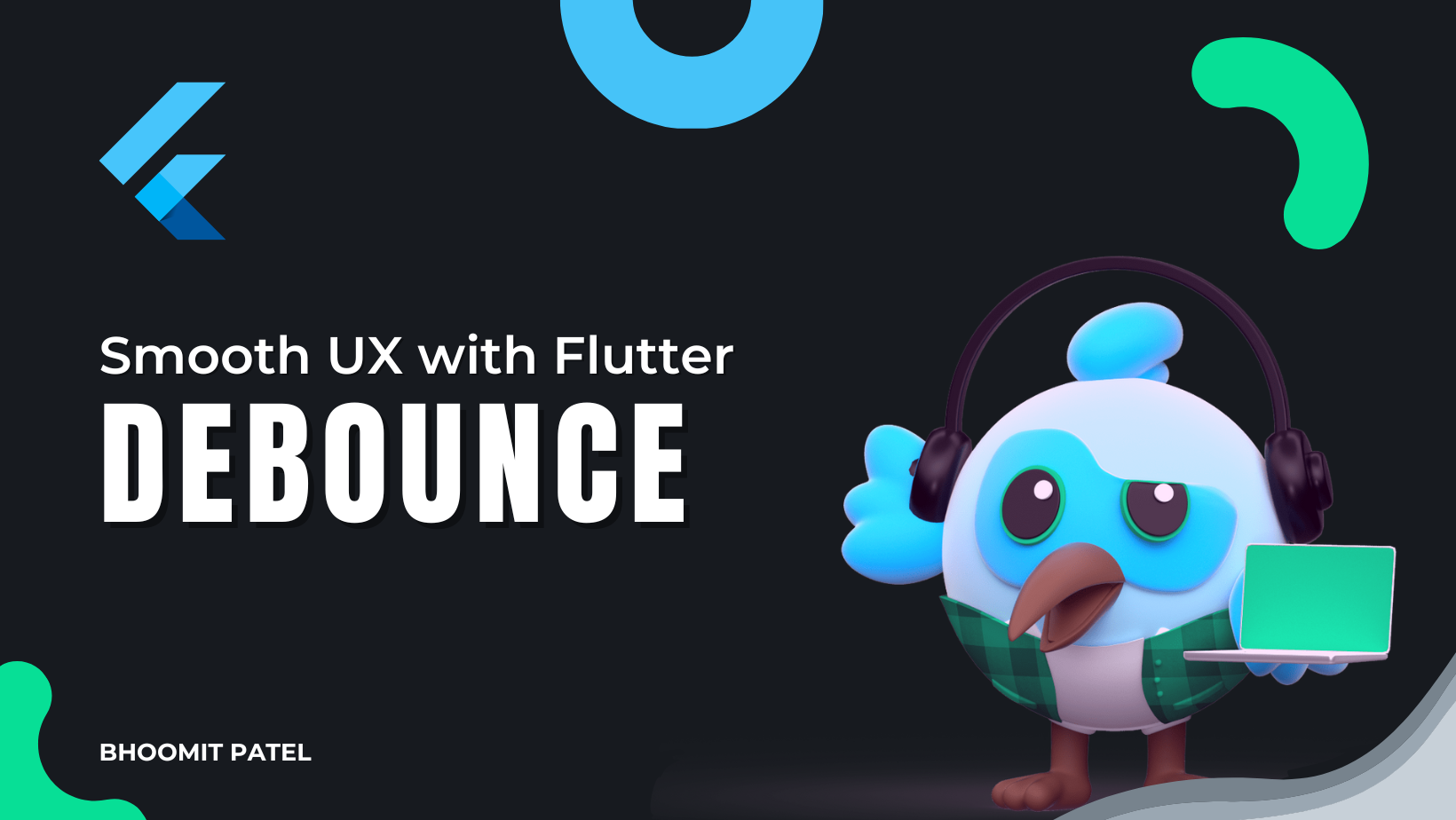
Introduction
In the world of mobile app development, providing a smooth and responsive user experience is paramount. One common challenge is handling rapid, repeated user inputs without overwhelming the app with unnecessary processing. This is where debouncing comes in. In this blog post, we will explore how to implement a debouncer in Flutter to enhance the performance and user experience of your app, particularly in scenarios like search functionalities.
What is Debouncing?
Debouncing is a programming practice used to ensure that time-consuming tasks do not fire so often. For example, when a user is typing into a search field, debouncing will help to wait until the user has stopped typing for a certain period before performing the search query. This can significantly reduce the number of requests sent and improve the overall performance of your app.
Implementing a Debouncer in Flutter
Let's dive into the implementation. We will create a Debouncer
class in Flutter and use it in a simple search functionality example.
Step 1: Create the Debouncer Class
First, we define the Debouncer
class which will handle the timing logic.
import 'dart:async';
import 'package:flutter/material.dart';
class Debouncer {
Debouncer({required this.interval});
final Duration interval;
VoidCallback? _action;
Timer? _timer;
void run(VoidCallback action) {
_action = action;
_timer?.cancel();
_timer = Timer(interval, _executeAction);
}
void _executeAction() {
_action?.call();
_timer = null;
}
void cancel() {
_action = null;
_timer?.cancel();
_timer = null;
}
}
Step 2: Using the Debouncer in a Search Screen
Next, we'll integrate this debouncer into a Flutter widget to handle search inputs.
class SearchWidget extends StatelessWidget {
final Debouncer _debouncer = Debouncer(interval: Duration(milliseconds: 500));
final TextEditingController _controller = TextEditingController();
@override
Widget build(BuildContext context) {
return TextField(
controller: _controller,
onChanged: (query) {
_debouncer.run(() {
_performSearch(query);
});
},
decoration: InputDecoration(
hintText: 'Search...',
border: OutlineInputBorder(),
),
);
}
void _performSearch(String query) {
print('Searching for: $query');
// Implement your search logic here
}
}
Explanation
Debouncer Class: This class manages the debouncing logic. When
run
is called, it resets the timer with the new action.SearchWidget: The
Debouncer
is used in theonChanged
callback of theTextField
. Every time the text changes, the debouncer resets the timer and only performs the search when the user stops typing for 500 milliseconds.
By implementing debouncing, you can significantly reduce the load on your app, leading to a smoother and more responsive user experience. This is particularly useful in search functionalities where rapid input changes are common.
Conclusion
Implementing a debouncer in your Flutter app is a straightforward yet powerful way to improve performance and user experience. By following the steps outlined above, you can ensure that your app handles rapid user inputs gracefully, providing a smooth and enjoyable experience for your users.
Stay tuned for more tips and tricks on enhancing your Flutter applications!
Happy Fluttering! ๐๐
#Flutter #SearchWithDebounce #OptimizePerformance #HappySearching ๐
Subscribe to my newsletter
Read articles from Bhoomit Patel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
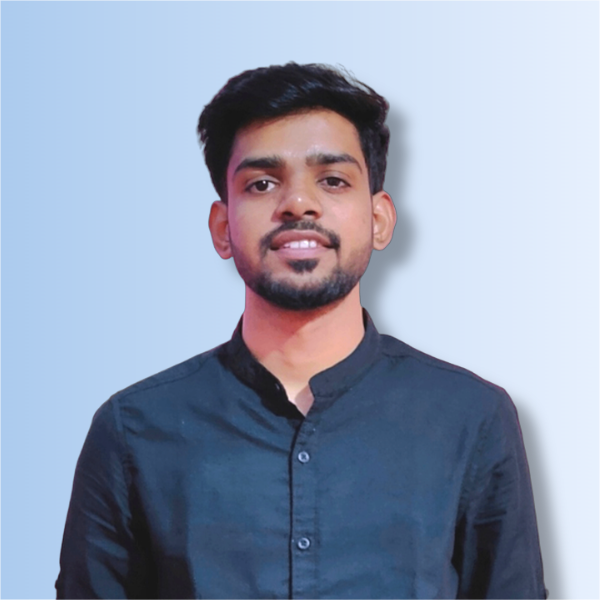
Bhoomit Patel
Bhoomit Patel
Flutter Developer with 4 years of experience creating user-friendly apps. Passionate about transforming ideas into efficient, beautiful solutions using Flutter. Skilled in UI/UX design and backend integration, with a creative approach to problem-solving and innovation.