How to Handle 3rd party(public) CORS issue
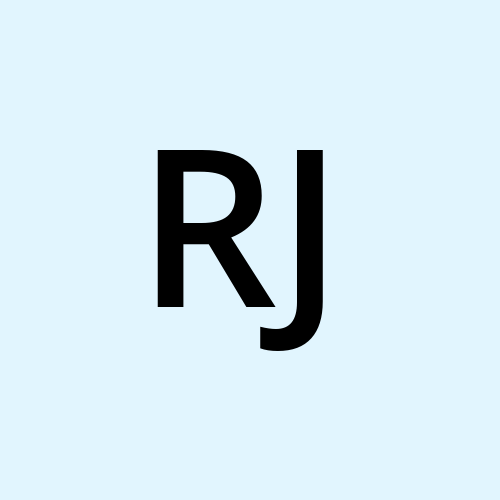
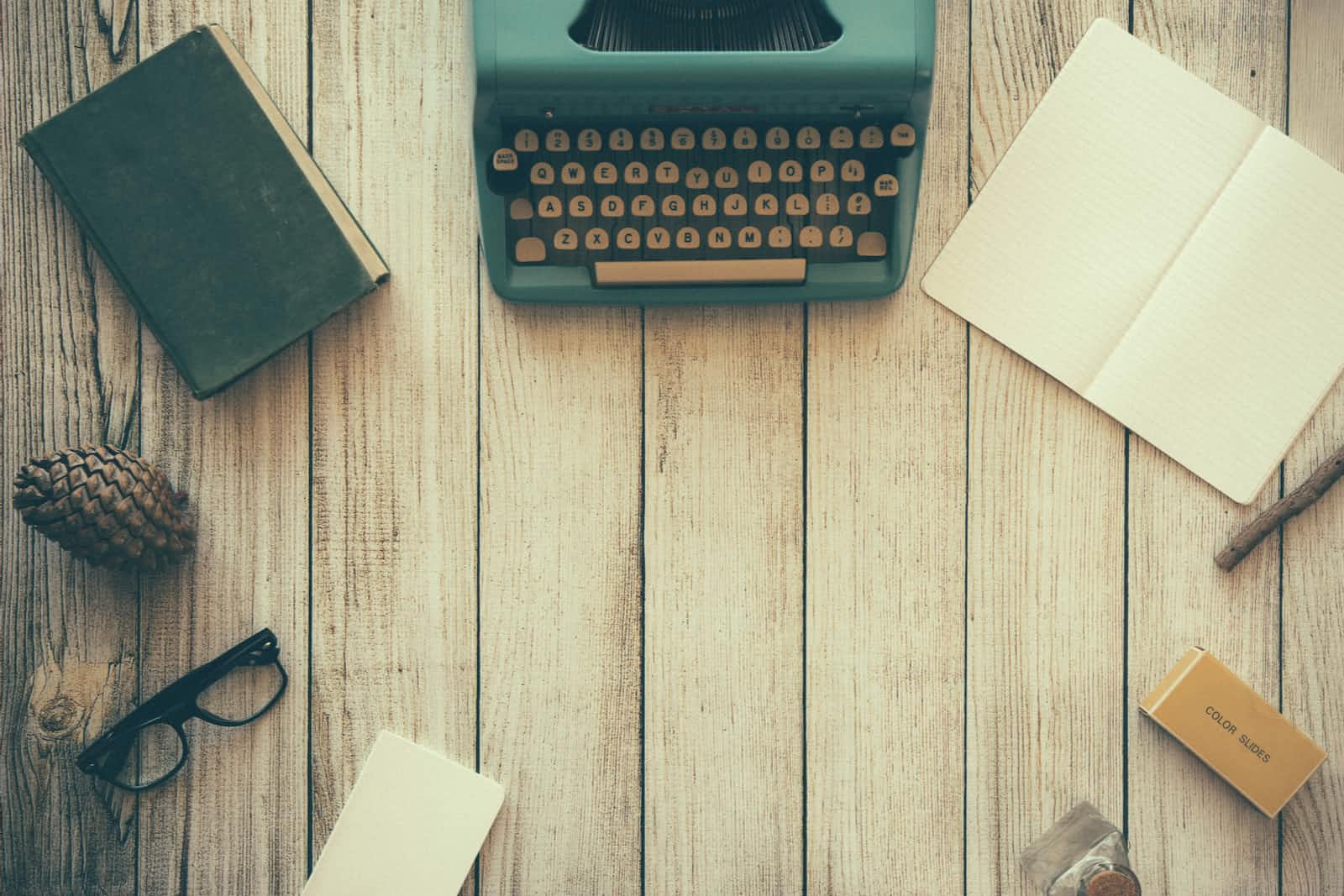
Sometimes we have a task to get the data from 3rd party APIs in UI, but UI is unable to handle those APIs and shows a Cors issue as the browser is blocking if the response is coming from other sources.
example, If UI is trying to get the data sales forces API or any other publicly hosted API, it will show a Cors issue because the server side they are not allowing request from other sources.
but they have exposed the api that users can get the data. If we run those apps in Postman then we can get the response.
To handle those kind of issues we need to implement one-mode middleware between UI and 3rd party API.
Node js is more suitable for this kind of scenario, as the UI guy knows about the javascript and Node is also on javascript.
EXAMPLE
Client Side
...
import axios from axios
useEffect(() => {
axios.get("3rd party api").then((response) => {
console.log(response);
}).catch(err)
}
// OR
useEffect(() => {
const getResponse = async() => {
const data = await axios.get("3rd party api");
}
getResponse()
}
// OR
useEffect(() => {
const getResponse = async() => {
const data = await fetch("3rd party api");
}
getResponse()
}
Not able to reach out the response and get a CORS error.
To handle it we need Node.js basic server that will run in between and handle to cors issue.
Initialize a new Node.js application
To initialize your app, run the following command in your terminal:
$> npm init
Install Express and other dependencies
From here on, you can run all your commands in your editor’s terminal.
Run the following command to install the Express framework:
npm install express
Above commands Initialize a node project from basic, but you need to install other dependencies.
npm install request-promist body-parser cors
you need to tell to the server to handle all the request using cors and response should allow all the type of request
const express = require("express")
const request = require("requesst-promise");
const cors = require("cors");
const bodyPaarser = require("body-parser");
let server = express()
server.use(cors());
let jsonServer = bodyParser.json();
server.get("/getResponse", (req, res => {
const option= {
method: 'GET',
uri: "https://3rd party api",
json: true,
headers: {
"Content-Type" : "application/json"
}
}
request(option).then((response) => {
res.status(200).json(response)
});
.catch((err) => {
console.log(err)
});
})
Call Node server exposed API in UI.
Client Side
...
import axios from axios
useEffect(() => {
axios.get("/getResponse").then((response) => {
console.log(response);
}).catch(err)
}
// OR
useEffect(() => {
const getResponse = async() => {
const data = await axios.get("/getResponse");
}
getResponse()
}
// OR
useEffect(() => {
const getResponse = async() => {
const data = await fetch("/getResponse");
}
getResponse()
}
Help me to post queries to get the response on any kind of problem-related to UI and Node technologies.
Subscribe to my newsletter
Read articles from Rohit Jangid directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
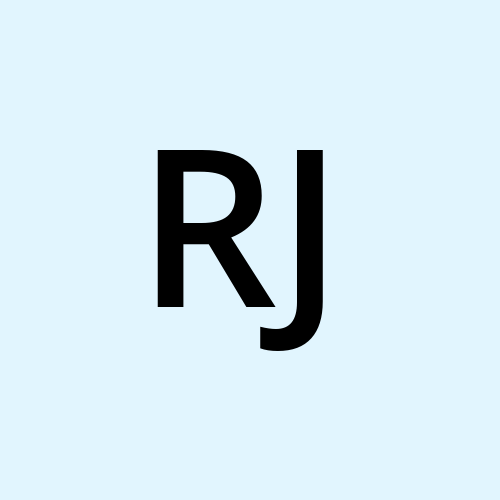
Rohit Jangid
Rohit Jangid
I am a full stack and webrtc developer on javascript technologies.