Prop Drilling in React and How Redux Solves the Problem!
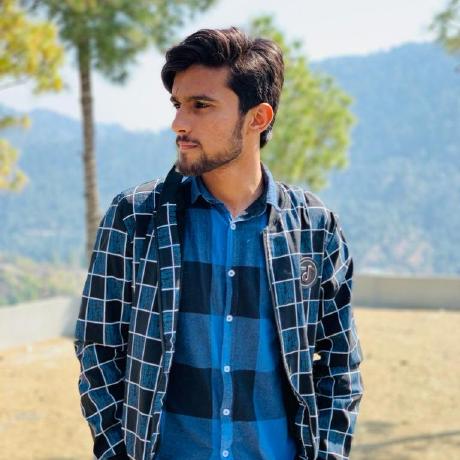
Table of contents
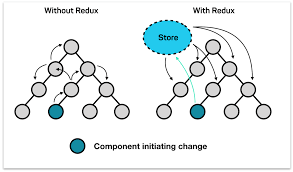
Title: Prop Drilling in React and How Redux Solves the Problem
Introduction In React application development, passing data through multiple nested components can become a cumbersome and error-prone process. This issue, known as "prop drilling," occurs when data needs to be passed down from a top-level component to a deeply nested child component through intermediate components. In this blog, we'll delve into the concept of prop drilling, explore its drawbacks, and demonstrate how Redux, a state management library, provides an elegant solution to this problem.
Understanding Prop Drilling Prop drilling is a natural consequence of React's unidirectional data flow. When a parent component needs to pass data to a child component, it does so by passing it down as props. However, if the data is required by several levels of nested components, each intermediate component must also receive the data as props and pass it down further. As the application grows and more components are added, this can lead to an overwhelming number of props being passed, resulting in less maintainable and harder-to-read code.
The Drawbacks of Prop Drilling Prop drilling can have several negative consequences on code quality and development efficiency:
Code Clutter: As props are passed through multiple components, the code becomes cluttered with props that are not directly relevant to the intermediate components.
Reduced Readability: The excessive number of props makes it challenging to understand the flow of data and the role of each component.
Brittleness: Changes in the data flow or the addition/removal of components can lead to the need to refactor a large portion of the codebase.
Performance Impact: Unnecessary passing of props can negatively impact application performance, leading to re-rendering of components even when their data remains unchanged.
Redux: A Solution to Prop Drilling Redux is a state management library that allows developers to maintain a global state accessible by any component in the application. This global state eliminates the need for prop drilling, as components can directly access the required data from the Redux store. Let's explore how Redux solves the prop drilling problem with a simple example.
Example: Prop Drilling without Redux Consider a React application with the following component hierarchy:
App
├── Parent
├── Child
Suppose the Child
component needs access to some data provided by the App
component. In the absence of Redux, we would pass the data through all the intermediate components:
// App.js
import React, { useState } from 'react';
import Parent from './Parent';
const App = () => {
const [data, setData] = useState('Some data');
return (
<div>
<Parent data={data} />
</div>
);
};
export default App;
// Parent.js
import React from 'react';
import Child from './Child';
const Parent = ({ data }) => {
return (
<div>
<Child data={data} />
</div>
);
};
export default Parent;
// Child.js
import React from 'react';
const Child = ({ data }) => {
return (
<div>
<p>Data received: {data}</p>
</div>
);
};
export default Child;
Example: Solving Prop Drilling with Redux With Redux, we can eliminate prop drilling by managing the shared data in the Redux store:
// store.js
import { configureStore, createSlice } from '@reduxjs/toolkit';
const dataSlice = createSlice({
name: 'data',
initialState: 'Some data',
reducers: {},
});
export default configureStore({
reducer: { data: dataSlice.reducer },
});
// App.js
import React from 'react';
import { Provider } from 'react-redux';
import Parent from './Parent';
import store from './store';
const App = () => {
return (
<Provider store={store}>
<div>
<Parent />
</div>
</Provider>
);
};
export default App;
// Parent.js
import React from 'react';
import { useSelector } from 'react-redux';
import Child from './Child';
const Parent = () => {
const data = useSelector((state) => state.data);
return (
<div>
<Child />
</div>
);
};
export default Parent;
// Child.js
import React from 'react';
import { useSelector } from 'react-redux';
const Child = () => {
const data = useSelector((state) => state.data);
return (
<div>
<p>Data received: {data}</p>
</div>
);
};
export default Child;
Conclusion Prop drilling can lead to messy and hard-to-maintain code, making it a common pain point for React developers. However, Redux offers a simple and elegant solution to this problem by providing a centralized store for state management. By using Redux, developers can eliminate prop drilling, resulting in cleaner, more maintainable, and efficient code.
Subscribe to my newsletter
Read articles from Abdullah rafiq directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
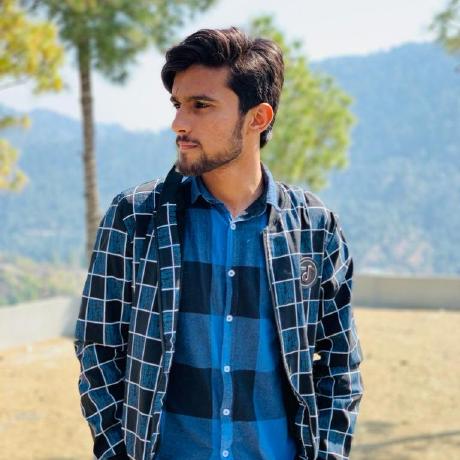
Abdullah rafiq
Abdullah rafiq
👋 Hi there! I am Full Stack Developer specializing in MERN stack, Next.js, Docker, and CI/CD. Passionate about building dynamic, scalable web applications.