Event Bubbling in JavaScript
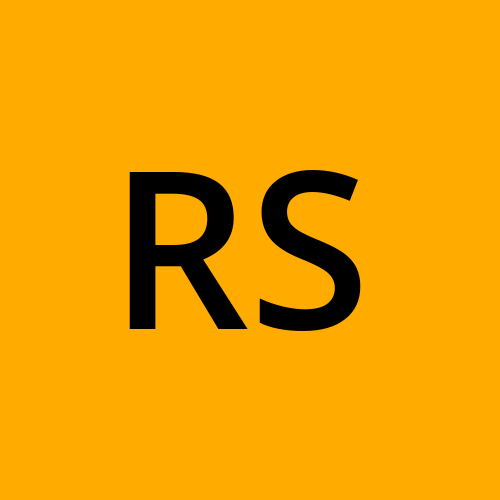
Event Bubbling is a concept of event propagation in the DOM. when an event occurs inside an HTML element. it gets propagated or bubbles up to its parent and ancestor element in the DOM tree until it gets to the root element.
Now let's see this behavior live in React Application. Here I've created three div
element with onClick
event handler so that we can console.log
if this event handler gets executed on this element.
import "./styles.css";
export default function App() {
return (
<div className="App">
<div
className="green"
onClick={(e) => {
console.log("green!");
}}
>
<div
className="yellow"
onClick={(e) => {
console.log("yellow!");
}}
>
<div
className="pink"
onClick={(e) => {
console.log("pink!");
}}
></div>
</div>
</div>
</div>
);
}
.App {
font-family: sans-serif;
text-align: center;
}
.green {
background-color: green;
width: 600px;
height: 600px;
position: absolute;
}
.yellow {
background-color: yellow;
width: 400px;
height: 400px;
position: absolute;
top: 100px;
left: 100px;
}
.pink {
background-color: pink;
width: 200px;
height: 200px;
position: absolute;
top: 100px;
left: 100px;
}
Now UI will look like this. So now when we inspect the console by clicking on these three different div.
If we click on the different color box. we get these outputs in consoles. which clearly shows how click
events get propagated till the root ancestor and onClick
event handler gets executed for each of these div.
We can stop this propagation at any div
by calling e.stopPropagation()
on any event handler so this event will not get propagated further.
Hope this helps! Let me know if you have any other questions.
Resources
Subscribe to my newsletter
Read articles from Rohit Saw directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
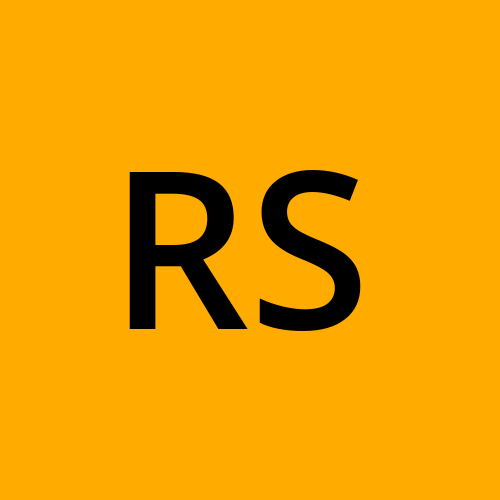
Rohit Saw
Rohit Saw
FullStack Developer