Floating-Point Follies
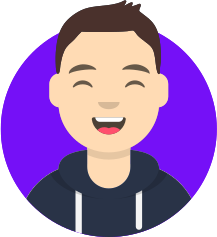
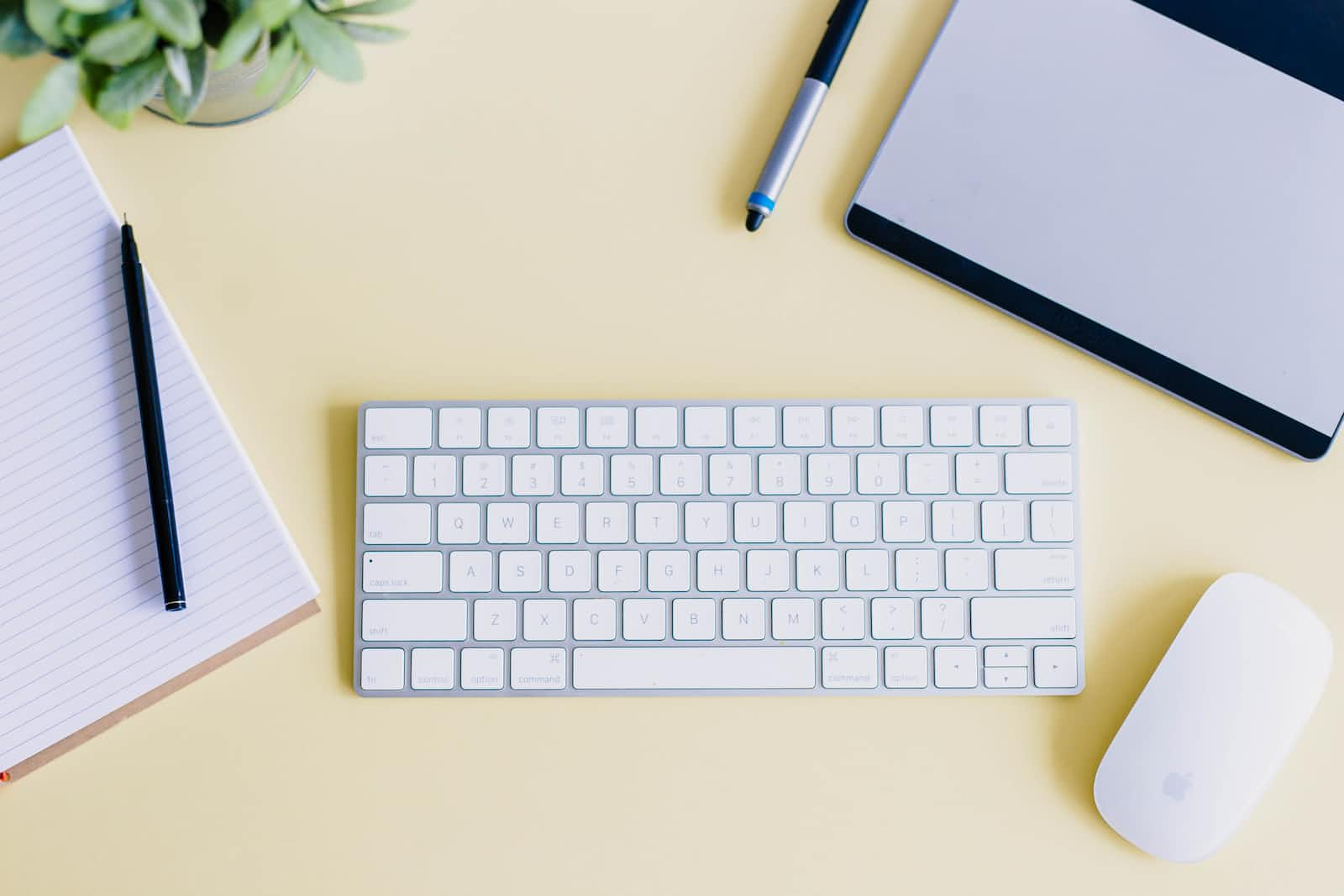
Greetings, code wranglers and binary whisperers! Today, we're diving headfirst into the wacky world of floating-point numbers. You know, those pesky decimals that make your computer seem like it flunked out of kindergarten math. Buckle up, because things are about to get hilariously precise!
The Basics of Floating-Point Numbers
Let's start with a simple question: What's 2.3 times 100? If you said 230, congratulations! You're smarter than a computer. Don't believe me? Let's ask our silicon-brained friend:
public static void main(String[] args) {
double a = 2.3;
double b = a * 100;
System.out.println("Result: " + b);
}
And the computer says... Result: 229.99999999999997
. Wait, what? Did our computer just fail basic arithmetic?
The IEEE 754 Standard: A Comedy of Errors
The culprit behind this numerical nonsense is the IEEE 754 standard. It's like the rulebook for how computers handle floating-point numbers. The problem? It's written in binary. And as anyone who's tried to read a rulebook in a foreign language can tell you, things can get lost in translation.
In our example, the decimal number 2.3 gets a binary makeover. But like a bad haircut, it doesn't quite come out as expected. When we multiply this binary imposter by 100, the result is a number that's close to 230, but not quite there.
Computers use binary (base 2) number system, while we humans use decimal (base 10). Some numbers that are simple and finite in decimal have infinite representations in binary. It's like trying to express one-third as a decimal. No matter how many decimal places you use, you can't express it exactly.
When we write 2.3
in our code, the computer stores an approximation of this number in binary. When we multiply this approximation by 100, the small error in the approximation gets multiplied too, leading to our unexpected result.
The BigDecimal Hero
So, how do we fix this? For calculations where precision is crucial, such as financial calculations, we can use the BigDecimal
class in Java. It's like the superhero of precision, swooping in to save our calculations from the evil clutches of approximation. Here's how we can use BigDecimal
to get the right answer:
import java.math.BigDecimal;
public static void main(String[] args) {
BigDecimal a = new BigDecimal("2.3");
BigDecimal b = a.multiply(new BigDecimal("100"));
System.out.println("Result: " + b);
}
And voila! The output is Result: 230
, just as we'd expect. BigDecimal
to the rescue!
The Plot Twist: Performance Trade-Off
But every hero has a weakness. For BigDecimal
, it's performance. While it's great at precision, it's a bit of a slowpoke. BigDecimal
operations can be slower than double
or float
operations because it takes more computational power to maintain that high precision. It's like choosing between a race car and a snail carrying a diamond. Sure, the snail is slow, but that diamond is worth the wait!
Wrapping Up
In the wild and wacky world of programming, even simple arithmetic can lead to a comedy of errors. But by understanding these quirks, we can write better, more accurate code. And remember, the next time your computer can't do basic math, it's not a bug, it's a feature!
So, here's to the floating-point follies, the decimal dramas, and the hilarious precision of it all. Stay tuned for more tales from the coding trenches. Until next time, happy coding!
Want to explore this topic further? I suggest reading the Handbook of Floating-Point Arithmetic by Jean-Michel Muller.
Subscribe to my newsletter
Read articles from Abou Zuhayr directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
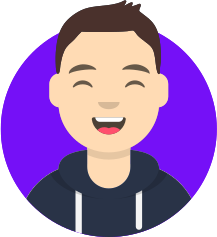
Abou Zuhayr
Abou Zuhayr
Hey, I’m Abou Zuhayr, Android developer by day, wannabe rockstar by night. With 6 years of Android wizardry under my belt and currently working at Gather.ai, I've successfully convinced multiple devices to do my bidding while pretending I'm not secretly just turning them off and on again. I’m also deeply embedded (pun intended) in systems that make you question if they’re alive. When I'm not fixing bugs that aren’t my fault (I swear!), I’m serenading my code with guitar riffs or drumming away the frustration of yet another NullPointerException. Oh, and I write sometimes – mostly about how Android development feels like extreme sport mixed with jazz improvisation. Looking for new challenges in tech that don’t involve my devices plotting against me!