Generator Function in JavaScript
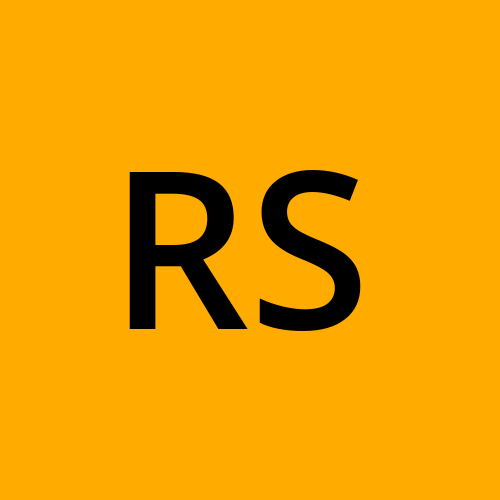
Generator Function is used to Pause and Resume the execution of the function with its context (variable bindings) saved across re-entrances. This allows you to generate values on-the-fly, without having to generate all of the values at once.
A generator is a special type of function that does not return a single value, instead, it returns an iterator object with a sequence of values. In a generator function, a yield statement is used rather than a return statement.
we can directly loop over returned iterator from the generator function or we can get the next value from the iterator object by calling next()
method of iterator which will return an object { value: any, done: boolean }
where value
is any value that is yield
by generator function and done
is a boolean flag to indicate if the execution of the generator function is complete.
A return
statement in a generator, when executed, will make the generator finish (i.e. the done
property of the object returned by it will be set to true
).
The implementation
we can create a generator function in javascript by using function*
and yield
syntax. here is an example of a Fibonacci generator that will generate the next Fibonacci number on the fly.
function* fibonacci(){
let a = 0;
let b = 1;
while (true){
yield a;
let tmp = a;
a = b;
b = tmp + b;
}
}
const fibb = fibonacci();
// Generating first 10 fibonacci number on fly
for(let i=0; i<10; i++){
console.log(fibb.next().value)
}
/* output
0
1
1
2
3
5
8
13
21
34
------------------------------ */
Advantage
Lazy Evaluation: Suppose there is a large or infinite stream of data. we cannot spend our whole life evaluating that data. Hence we can use Generator function to evaluate as and when required.
Memory Efficient: Generator functions are memory efficient because only those data and those computations that are necessary are used. eg: Suppose we want to read and process all files in a particular directory, we can always write a generator function to read and process files one by one as required.
Time-efficient when compared to overhead in managing intermediary lists as shown below image.
Hope this helps! Let me know if you have any other questions.
Resources
Subscribe to my newsletter
Read articles from Rohit Saw directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
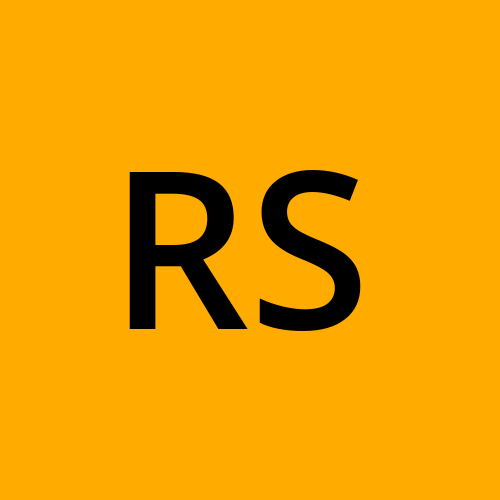
Rohit Saw
Rohit Saw
FullStack Developer