Terraform Functions with Examples

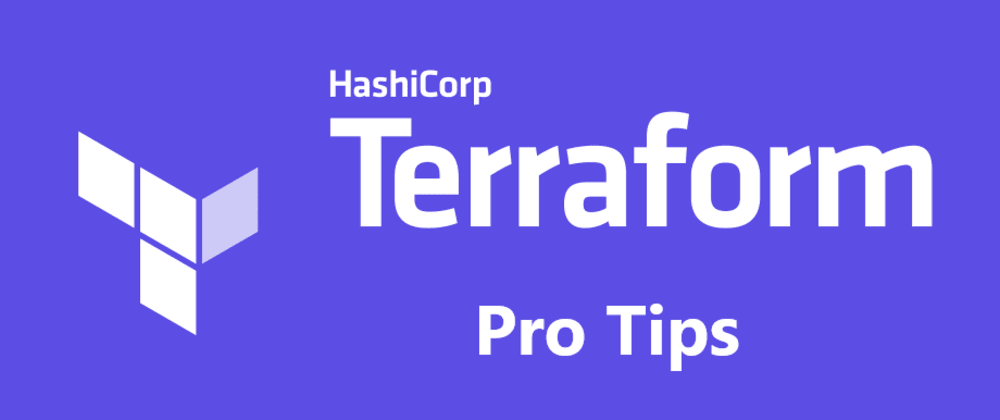
Terraform: Functions
To perform local tests and validate Terraform functions you can run the below command
terraform console
Terraform provides various functions that enable you to manipulate data, perform calculations, and work with files, dates, and networks. These functions prove useful for configuring infrastructure using Terraform.
Numeric Functions:
abs(number)
: Returns the absolute value of the given number. Example:abs(-19.86)
returns19.86
ceil(number)
: Returns the smallest integer greater than or equal to the given value. Example:ceil(7.1)
returns8
floor(number)
: Returns the largest integer less than or equal to the given value. Example:floor(7.1)
returns7
log(number, base)
: Calculates the logarithm of a given number in a specified base. Example:log(16, 2)
returns4
max(N1, N2, N3, Nn)
: Takes one or more numbers and returns the greatest number from the set. Example:max(3, 2, 6, 8.8, 7)
returns8.8
min(N1, N2,..Nn)
: Takes one or more numbers and returns the smallest number from the set. Example:min(3, 2, 6, 8.8, 7)
returns2
pow(number, power)
: Calculates an exponent by raising the first argument to the power of the second argument. Example:pow(8, 2)
returns64
signum(number)
: Determines the sign of a number, returning -1, 0, or 1 to represent the sign. Example:signum(-4)
returns-1
, andsignum(4)
returns1
String Functions:
chomp("string")
: Removes newline characters at the end of a string. Example:chomp("cloudaffaire\n")
returns"cloudaffaire"
(removing\n
from the end)format(spec, values...)
: Produces a formatted string by combining multiple values according to a specification string. Example:format("Welcome, to %s", "CloudAffaire")
returns"Welcome, to CloudAffaire"
, andformat("%4.4f+", 3.86)
returns"3.8600+"
formatlist(spec, values...)
: Produces a list of formatted strings by combining multiple values based on a specification string. Example:formatlist("www.%s.com", list("azure", "aws", "google"))
returns["
www.azure.com
", "
www.aws.com
", "
www.google.com
"]
indent(num_spaces, string)
: Adds a given number of spaces to the beginning of all but the first line in a multi-line string. Example:indent(8, "hi, \n welcome \n to \n cloudaffaire")
returns"hi, \n welcome \n to \n cloudaffaire"
join(separator, list)
: Concatenates elements of a list into a single string with the specified separator. Example:join(".", list("www", "google", "com"))
returns"
www.google.com
"
lower(string)
: Converts all letters in the given string to lowercase. Example:lower("CLOUDAFFAIRE")
returns"cloudaffaire"
upper(string)
: Converts all letters in the given string to uppercase. Example:upper("cloudaffaire")
returns"CLOUDAFFAIRE"
replace(string, substring, replacement)
: Searches a given string for another given substring and replaces each occurrence with a given replacement string. Example:replace("
www.google.com
", "google", "cloudaffaire")
returns"
www.cloudaffaire.com
"
split(separator, string)
: Divides a given string into a list based on a separator. Example:split(".", "
www.google.com
")
returns["www", "google", "com"]
strrev(string)
: Reverses the characters in a string. Note that the characters are treated as Unicode characters. Example:strrev("google")
returns"elgoog"
(supported in Terraform version 0.12 or later)substr(string, offset, length)
: Extracts a substring from a given string based on an offset and length. Example:substr("
www.google.com
", 4, 6)
returns"google"
title(string)
: Capitalizes the first letter of each word in the given string. Example:title("welcome to cloudaffaire")
returns"Welcome To Cloudaffaire"
trimspace(string)
: Removes any leading and trailing space characters from the given string. Example:trimspace(" hello, all ")
returns"hello, all"
Collection Functions
chunklist(list, chunk_size)
: Splits a list into fixed-size chunks, returning a list of lists. Example:chunklist(list("a", "b", "c", "d", "e", "f"), 3)
returns[["a", "b", "c"], ["d", "e", "f"]]
coalesce(strings, numbers)
: Takes any number of arguments and returns the first one that isn't null or an empty string. Example:coalesce("", 1, "a")
returns1
coalescelist(list1, list2, ..., listn)
: Takes any number of list arguments and returns the first one that isn't empty. Example:coalescelist(list(), list("a", "b", "c"), list("d", "e"))
returns["a", "b", "c"]
compact(list(string))
: Takes a list of strings and returns a new list with any empty string elements removed. Example:compact(list("a", "", "c", "", "d"))
returns["a", "c", "d"]
concat(list1, list2, ..., listn)
: Takes two or more lists and combines them into a single list. Example:concat(list("a", "b"), list("c", "d"), list("e", "f"))
returns["a", "b", "c", "d", "e", "f"]
contains(list, value)
: Determines whether a given list or set contains a specific value. Example:contains(list("a", "b", "c"), "a")
returnstrue
, andcontains(list("a", "b", "c"), "d")
returnsfalse
distinct(list)
: Takes a list and returns a new list with any duplicate elements removed. Example:distinct(list("a", "b", "b", "c"))
returns["a", "b", "c"]
element(list, index)
: Retrieves a single element from a list based on its index. Example:element(list("a", "b", "c"), 2)
returns"c"
(index starts from 0)index(list, value)
: Finds the index of a value in a list. Example:index(list("a", "b", "c"), "b")
returns1
flatten(list(list1, list2, ..., listn))
: Takes a list and replaces any nested lists with a flattened sequence of their contents. Example:flatten(list(list("a", "b"), list("c"), list(), list("d", "e")))
returns["a", "b", "c", "d", "e"]
keys(map)
: Takes a map and returns a list containing the keys from that map. Example:keys(map("name", "debjeet", "sex", "male"))
returns["name", "sex"]
length(list\map\string)
: Determines the length of a given list, map, or string. Example:length(list("a", "b"))
returns2
,length("debjeet")
returns7
, andlength(map("name", "debjeet", "sex", "male"))
returns2
list()
: Takes an arbitrary number of arguments and returns a list containing those values in the same order. Example:list("a", "b", "c")
returns["a", "b", "c"]
lookup(map, key, default)
: Retrieves the value of an element from a map given its key. If the key does not exist, the default value is returned. Example:lookup(map("name", "debjeet", "sex", "male"), "sex", "not found!")
returns"male"
, andlookup(map("name", "debjeet", "", "male"), "gender", "not found!")
returns"not found!"
merge(map1, map2, ..., mapn)
: Takes an arbitrary number of maps and returns a single map that contains a merged set of elements from all the maps. Example:merge(map("a", "one"), map("b", "two"), map("c", "three"))
returns{"a"="one", "b"="two", "c"="three"}
reverse(list)
: Takes a sequence and produces a new sequence of the same length with all the same elements but in reverse order. Example:reverse(list("a", "b", "c"))
returns["c", "b", "a"]
(supported in Terraform version 0.12 or later)setintersection(sets...)
: Takes multiple sets and produces a single set containing only the elements that all the given sets have in common. Example:setintersection(list("a", "b"), list("b", "c"), list("b", "d"))
returns["b"]
(supported in Terraform version 0.12 or later)setproduct(sets...)
: Finds all the possible combinations of elements from all the given sets by computing the Cartesian product. Example:setproduct(list("a", "b"), list("c", "d"))
returns[["a", "c"], ["a", "d"], ["b", "c"], ["b", "d"]]
(supported in Terraform version 0.12 or later)setunion(sets...)
: Takes multiple sets and produces a single set containing the elements from all the given sets. Example:setunion(list("a", "b"), list("c", "d"))
returns["a", "b", "c", "d"]
(supported in Terraform version 0.12 or later)slice(list, startindex, endindex)
: Extracts some consecutive elements from a list based on the start and end index. Example:slice(list("zero", "one", "two", "three"), 1, 3)
returns["one", "two"]
sort(list)
: Takes a list of strings and returns a new list with those strings sorted lexicographically. Example:sort(list("d", "c", "a", "b"))
returns["a", "b", "c", "d"]
transpose()
: Takes a map of lists of strings and swaps the keys and values to produce a new map of lists of strings. Example:transpose(map("a", list("one", "two"), "b", list("three", "four")))
returns{"four"=["b"], "one"=["a"], "three"=["b"], "two"=["a"]}
values(map)
: Takes a map and returns a list containing the values of the elements in that map. Example:values(map("name", "debjeet", "sex", "male"))
returns["debjeet", "male"]
zipmap(keyslist, valueslist)
: Constructs a map from a list of keys and a corresponding list of values. Example:zipmap(list("name", "sex"), list("debjeet", "male"))
returns{"name"="debjeet", "sex"="male"}
Encoding Functions
base64encode(string)
: Applies Base64 encoding to a given string. Example:base64encode("cloudaffaire")
returns"Y2xvdWRhZmZhaXJl"
base64gzip(string)
: Compresses a string with gzip and then encodes the result in Base64 encoding. Example:base64gzip("cloudaffaire")
returns"H4sIAAAAAAAA/0rOyS9NSUxLS8wsSgUAAAD//wEAAP//38z9sQwAAAA="
base64decode(string)
: Takes a string containing a Base64 character sequence and returns the original string. Example:base64decode("Y2xvdWRhZmZhaXJl")
returns"cloudaffaire"
csvdecode(string)
: Decodes a string containing CSV-formatted data and produces a list of maps representing that data. Example:csvdecode("a,b,c\n1,2,3\n")
returns[{"a"="1", "b"="2", "c"="3"}]
(supported in Terraform version 0.12 or later)jsonencode()
: Encodes a given value to a string using JSON syntax. Example:jsonencode(map("name", "debjeet"))
returns{"name":"debjeet"}
jsondecode()
: Interprets a given string as JSON, returning a representation of the result of decoding that string. Example:jsondecode("{\"name\":\"debjeet\"}")
returns{"name"="debjeet"}
(supported in Terraform version 0.12 or later)urlencode()
: Applies URL encoding to a given string. Example:urlencode("
https://cloudaffaire.com/?s=terraform
")
returns"https%3A%2F%2Fcloudaffaire.com%2F%3Fs%3Dterraform"
Filesystem Functions
dirname(string)
: Takes a string containing a filesystem path and removes the last portion from it. Example:dirname("/home/ec2-user/terraform/main.tf")
returns"/home/ec2-user/terraform"
pathexpand()
: Takes a filesystem path that might begin with a ~ segment and replaces that segment with the current user's home directory path. Example:pathexpand("~/.ssh/id_rsa")
returns"/home/ec2-user/.ssh/id_rsa"
basename(string)
: Takes a string containing a filesystem path and removes all except the last portion from it. Example:basename("/home/ec2-user/terraform/main.tf")
returns"
main.tf
"
file(path)
: Reads the contents of a file at the given path and returns them as a string. Example:file("/home/ec2-user/terraform/main.tf")
returns the content of the file "main.tf"fileexists(path)
: Determines whether a file exists at a given path. Example:fileexists("/home/ec2-user/terraform/main.tf")
returnstrue
if the file "main.tf" exists (supported in Terraform version 0.12 or later)filebase64(path)
: Reads the contents of a file at the given path and returns them as a base64-encoded string. Example:filebase64("/home/ec2-user/terraform/main.tf")
returns the content of the file "main.tf" as base64 data (supported in Terraform version 0.12 or later)templatefile(path, vars)
: Reads the file at the given path and renders its content as a template using a supplied set of template variables.
Date & Time Functions
formatdate(spec, timestamp)
: Converts a timestamp into a different time format. Example:formatdate("MMM DD, YYYY", "2018-01-02T23:12:01Z")
returns"Jan 02, 2018"
(supported in Terraform version 0.12 or later)timeadd(timestamp, duration)
: Adds a duration to a timestamp, returning a new timestamp. Duration is a string representation of a time difference, consisting of sequences of number and unit pairs, like "1.5h" or "1h30m". The accepted units are "ns", "us" (or "µs"), "ms", "s", "m", and "h". The first number may be negative to indicate a negative duration, like "-2h5m". Example:timeadd("2019-05-10T00:00:00Z", "10m")
returns"2019-05-10T00:10:00Z"
timestamp()
: Returns the current date and time. Example:timestamp()
returns the current date and time.
IP & Network Functions
cidrhost(prefix, hostnum)
: Calculates a full host IP address for a given host number within a given IP network address prefix. Example:cidrhost("10.0.0.0/16", 4)
returns"10.0.0.4"
cidrnetmask(prefix)
: Converts an IPv4 address prefix given in CIDR notation into a subnet mask address. Example:cidrnetmask("10.0.0.0/16")
returns"255.255.0.0"
cidrsubnet(prefix, newbits, netnum)
: Calculates a subnet address within a given IP network address prefix. The prefix must be given in CIDR notation, newbits is the number of additional bits with which to extend the prefix, and netnum is a whole number that can be represented as a binary integer with no more than newbits binary digits. Example:cidrsubnet("10.0.0.0/16", 8, 2)
returns"10.0.2.0/24"
Subscribe to my newsletter
Read articles from Nimesh Panchal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Nimesh Panchal
Nimesh Panchal
I am Nimesh Panchal, a highly skilled and experienced professional with expertise in multiple cloud platforms, including AWS, Azure, and GCP. I am also certified in various cloud and virtualisation technologies, such as CKA, AWS, Azure, GPC, VMware, and Nutanix Throughout my career, I have demonstrated a strong passion for cloud computing and have actively contributed to the success of cloud adoption in diverse industries. My hands-on experience in designing, implementing, and managing cloud-based solutions has allowed me to drive operational efficiency, cost optimization, and scalability for businesses. As a cloud enthusiast, I stay updated with the latest advancements and best practices in the cloud domain. My commitment to continuous learning has enabled me to effectively leverage cloud technologies and deliver impactful solutions to complex challenges. Apart from my cloud expertise, I also possess a solid foundation in networking, virtualization, and data center technologies, making me a well-rounded IT professional. I take pride in collaborating with cross-functional teams and providing leadership in cloud migration, infrastructure design, and cloud security initiatives. My problem-solving skills, coupled with my ability to communicate technical concepts to non-technical stakeholders, have been instrumental in fostering seamless collaboration and driving successful cloud projects.