An introduction to javascript DOM

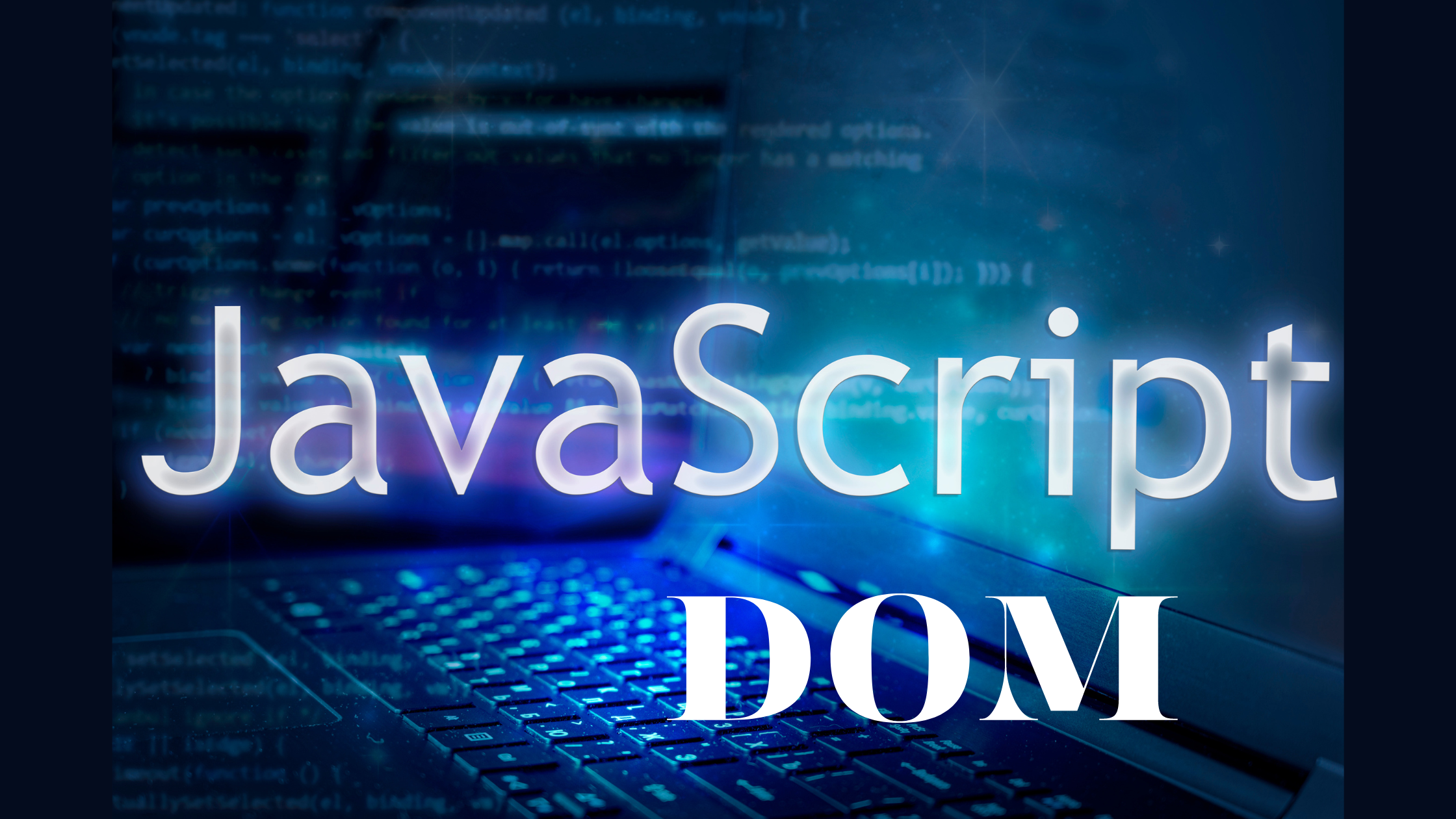
Discover the power of JavaScript's Document Object Model (DOM) in this comprehensive introduction. Learn how to manipulate web pages dynamically, interact with elements, and create engaging user experiences using DOM manipulation techniques.
Introduction
The JavaScript DOM (Document Object Model) is a powerful and essential concept in web development. It allows JavaScript to interact with HTML and XML documents, enabling dynamic, interactive, and responsive web pages. Let's take a closer look at the JavaScript DOM and how it works.
When a web page is loaded in a browser, the browser's rendering engine creates the DOM representation of that page. The DOM consists of various objects, each representing an element in the web page. These elements can be tags, attributes, text, or even comments. Each object in the DOM tree is known as a "node," and the relationship between nodes corresponds to the hierarchical structure of the HTML document.
What Is the DOM
The DOM is a programming interface provided by web browsers when a web page is loaded. It creates a structured representation of the web page as a hierarchical tree of objects.
Each element in the HTML document, such as headings, paragraphs, images, forms, etc., is represented as a "node" in the DOM tree.
The DOM allows JavaScript to easily access, modify, and manipulate these nodes, providing a way to dynamically change the web page's content, structure, and style.
// Get the elements we need
const greetingElement = document.getElementById('greeting');
const changeButton = document.getElementById('changeButton');
// Function to change the greeting
function changeGreeting() {
greetingElement.textContent = 'Hello, JavaScript!';
}
// Add event listener to the button
changeButton.addEventListener('click', changeGreeting);
In this example, when the "Change Greeting" button is clicked, the changeGreeting function is executed, which updates the text content of the h1 element with the new greeting.
Accessing DOM Elements
To access elements in the DOM, you can use various methods provided by JavaScript,
JavaScript can access DOM elements using various methods, such as
document.getElementById(), document.querySelector(), and
document.getElementsByClassName().
getElementById() retrieves an element using its unique id attribute.
querySelector() lets you select elements based on CSS selectors.
getElementsByClassName() returns a collection of elements with a specific class name.
Let's take an example where we have an HTML page with a button and a paragraph:
HTML:
<!DOCTYPE html>
<html>
<head>
<title>DOM Example</title>
</head>
<body>
<button id="myButton">Click Me!</button>
<p id="myParagraph">This is a paragraph.</p>
<script src="script.js"></script>
</body>
</html>
JavaScript: (script.js):
// Accessing elements by ID
const myButton = document.getElementById('myButton');
const myParagraph = document.getElementById('myParagraph');
console.log(myButton); // Output: <button id="myButton">Click 11Me!</button>
console.log(myParagraph); // Output: <p id="myParagraph">This is a paragraph.</p>
Manipulating DOM Elements
JavaScript can modify HTML elements' content, attributes, and styles, allowing dynamic updates based on user interactions or other events.
Now, let's manipulate the paragraph element by changing its content and style:
JavaScript (script.js)
// Accessing the paragraph element
const myParagraph = document.getElementById('myParagraph');
// Modifying the content
myParagraph.textContent = 'New text for the paragraph.';
// Changing the style
myParagraph.style.color = 'blue';
myParagraph.style.fontSize = '18px';
Event Handling
The DOM enables JavaScript to respond to various events triggered by the user, such as clicks, keypresses, mouse movements, etc.
You can add event listeners to DOM elements to respond to user interactions. Let's add an event listener to the button so that when it's clicked, the paragraph text changes.
JavaScript (script.js)
// Accessing elements
const myButton = document.getElementById('myButton');
const myParagraph = document.getElementById('myParagraph');
// Event handling function
function changeText() {
myParagraph.textContent = 'Text changed after button click!';
myParagraph.style.color = 'red';
}
// Adding the event listener
myButton.addEventListener('click', changeText);
Creating and Removing Elements
We can add new elements to the DOM or remove existing ones. Let's create a new paragraph and append it to the body:
JavaScript can create new elements using document.createElement() and add them to the DOM using appendChild() or other insertion methods
JavaScript (script.js):
// Creating a new paragraph element
const newParagraph = document.createElement('p');
newParagraph.textContent = 'This is a new paragraph added dynamically.';
// Appending the new paragraph to the body
document.body.appendChild(newParagraph);
Query Selector
The querySelector method allows you to select elements based on CSS selectors, similar to how you select elements in CSS:
JavaScript (script.js):
// Accessing elements using querySelector
const myButton = document.querySelector('#myButton'); // Selects the button with ID 'button'
const myParagraph = document.querySelector('p'); // Selects the first <p> element on the page
These examples demonstrate the basics of JavaScript DOM manipulation.
With the DOM, you can create dynamic and interactive web pages, respond to user actions, and change the content and appearance of elements on the fly.
The DOM provides a powerful way to create interactive and dynamic web pages. It acts as an intermediary between the web page's content and the scripting language, enabling seamless communication and manipulation of its elements. It's important to note that while the DOM is typically associated with JavaScript in the context of web browsers, other programming languages can also interact with the DOM in different environments (e.g., server-side JavaScript with Node.js.
Conclusion
The DOM is a fundamental concept in web development, and mastering it will enable you to build more sophisticated and engaging web applications.
Understanding JavaScript DOM is crucial for building modern, interactive web applications. It enables developers to create dynamic content, handle user interactions, and update web pages without requiring a full reload.
The DOM provides a powerful way to create interactive and dynamic web pages. It acts as an intermediary between the web page's content and the scripting language, enabling seamless communication and manipulation of its elements. It's important to note that while the DOM is typically associated with JavaScript in the context of web browsers, other programming languages can also interact with the DOM in different environments (e.g., server-side JavaScript with Node.js).
Remember that the DOM is specific to web browsers and provides an interface for client-side JavaScript. For server-side JavaScript environments like Node.js, a different object model (e.g., JSDOM) is used to simulate a browser-like environment to work with the DOM
Subscribe to my newsletter
Read articles from Okafor Johnson directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
